序列化就是将对象实例的状态存储到存储媒介的过程。
序列化实现对对象信息的永久性存储。
反序列化就是读取文件的过程
序列化和反序列化的实现步骤(json序列化):
- (1)使用 JsonSerializer.Serialize(student); 返回一个Json字符串
- 引入System.Text.Json.JsonSerializer 提供了一个非泛型版本
- 将一个对象序列化为 JSON 字符串,可以使用 JsonSerializer.Serialize<T> 方法;
- 将 JSON 字符串反序列化为对象,则可以使用 JsonSerializer.Deserialize<T> 方法。 进行反序列化时,指定泛型类型是须要的
- Student student = JsonSerializer.Deserialize<Student>(json);
序列化单个对象
示例:如下图,实现单个对象的序列化与反序列化
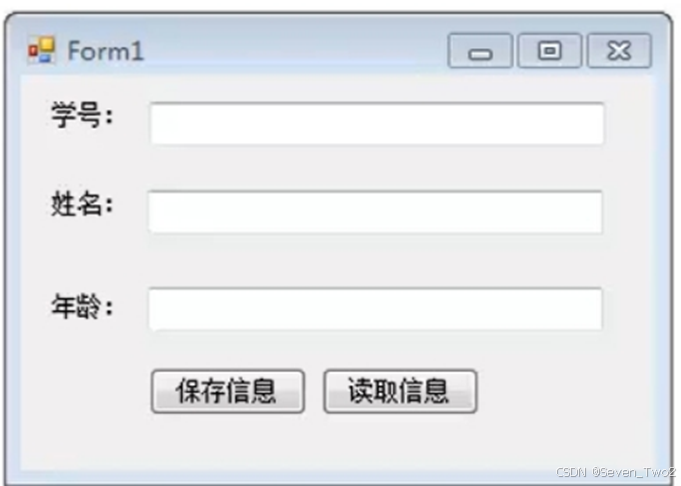
输入学生信息,点击“保存信息"按钮,将学生信息永久序列化保存到电脑上。
关闭程序后,在启动程序,可以将当地序列化文件读取,将信息显示在界面的文本框中。
其中,学号,姓名,年龄文本框的name分别为txtNo,txtName,txtAge。
- internal class Student //学生类
- {
- public string StuNum { get; set; }
- public string StuName { get; set; }
- public int StuAge { get; set; }
- }
- private void btnSave_Click(object sender, EventArgs e)
- {
- Student student = new Student();
- student.StuNum = this.textNo.Text;
- student.StuName = this.textName.Text;
- student.StuAge = int.Parse(this.textAge.Text);
-
- string json = JsonSerializer.Serialize(student);
- FileStream fs = new FileStream("stu.json", FileMode.Create, FileAccess.ReadWrite);
- StreamWriter sw = new StreamWriter(fs);
- sw.Write(json);
- sw.Close();
- fs.Close();
- MessageBox.Show("序列化成功");
- }
- //***************************************************************************************************************
- private void btnReade_Click(object sender, EventArgs e)
- {
- FileStream fs = new FileStream("stu.json", FileMode.Open, FileAccess.ReadWrite);
- StreamReader sr = new StreamReader(fs);
- //读取整个文件内容
- string json = sr.ReadToEnd();
- //关闭流
- sr.Close();
- fs.Close();
- //反序列化Json字符串为Student对象
- Student student = JsonSerializer.Deserialize<Student>(json);
- //使用Student对象信息
- this.textNo.Text = student.StuNum;
- this.textName.Text = student.StuName;
- this.textAge.Text =student.StuAge.ToString();
- MessageBox.Show("**反序列化成功**");
- }
- }
复制代码 序列化聚集
示例:如下图,实现聚集的序列化
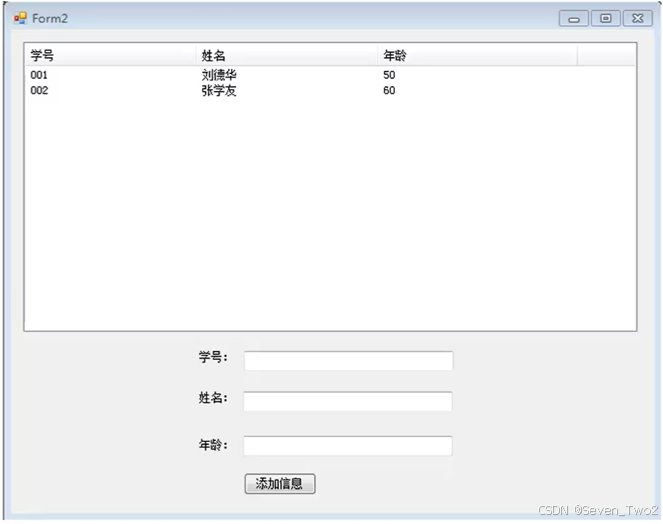
打开窗体,主动从序列化文件中读取信息,并在listView列表上进行展示。
输入学生信息,点击“添加信息”按钮,添加学生,而且序列化学生列表,刷新ListView列表数据。
- List<Student> list = new List<Student>();//创建集合存储对象
- private void Form3_Load(object sender, EventArgs e)
- {
- bindDate();
- }
- public void bindDate()
- {
- if (!File.Exists("list.json"))
- {
- return;
- }
- FileStream fs = new FileStream("list.json", FileMode.Open, FileAccess.Read);
- //使用JsonSerializer 将Json字符串反序列为 Student对象的列表
- list = JsonSerializer.Deserialize<List<Student>>(fs);
- fs.Close();
- this.listView1.Items.Clear(); // 清空listView1中的所有项
- foreach (Student stu in list)
- { // 为每个Student对象创建一个ListViewItem,并设置其主项为学生编号
- ListViewItem item = new ListViewItem(stu.StuNum);
- item.SubItems.Add(stu.StuName);
- item.SubItems.Add(stu.StuAge.ToString());
- this.listView1.Items.Add(item);
- }
- }
- private void btnAdd_Click(object sender, EventArgs e)
- {
- Student student = new Student();
- student.StuNum = this.textNo.Text;
- student.StuName = this.textName.Text;
- student.StuAge = int.Parse(this.textAge.Text);
- list.Add(student);
- //将Student列表序列化为一个Json类型的字符串
- string json = JsonSerializer.Serialize(list);
- FileStream fs = new FileStream("list.json", FileMode.Create, FileAccess.Write);
- StreamWriter sw = new StreamWriter(fs);
- sw.Write(json);
- sw.Close();
- fs.Close();
- MessageBox.Show("保存成功");
- bindDate(); //刷新listView数据列表
- }
- }
复制代码
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |