success.h
- #ifndef SUCCESS_H
- #define SUCCESS_H
- #include <QWidget>
- namespace Ui {
- class success;
- }
- class success : public QWidget
- {
- Q_OBJECT
- public:
- explicit success(QWidget *parent = nullptr);
- ~success();
- public slots:
- void my_login_slot();
- private slots:
- void on_pushButton_clicked();
- private:
- Ui::success *ui;
- };
- #endif // SUCCESS_H
复制代码 widget.h
- #ifndef WIDGET_H
- #define WIDGET_H
- #include <QWidget>
- #include<QMessageBox>
- #include<QLabel>
- QT_BEGIN_NAMESPACE
- namespace Ui { class Widget; }
- QT_END_NAMESPACE
- class Widget : public QWidget
- {
- Q_OBJECT
- public:
- Widget(QWidget *parent = nullptr);
- ~Widget();
- public slots:
- void my_cancel_slot();
- signals:
- void my_login_signal();
- void my_cl_signal();
- private slots:
- void on_pushButton_clicked();
- void on_pushButton_2_clicked();
- private:
- Ui::Widget *ui;
- };
- #endif // WIDGET_H
复制代码 mani.cpp
- #include "widget.h"
- #include "success.h"
- #include <QApplication>
- int main(int argc, char *argv[])
- {
- QApplication a(argc, argv);
- Widget w;
- w.show();
- success login;
- QObject::connect(&w,&Widget::my_login_signal,&login,&success::my_login_slot);
- return a.exec();
- }
复制代码 success.cpp
- #include "success.h"
- #include "ui_success.h"
- success::success(QWidget *parent) :
- QWidget(parent),
- ui(new Ui::success)
- {
- ui->setupUi(this);
- this->setWindowFlag(Qt::FramelessWindowHint);
- this->setAttribute(Qt::WA_TranslucentBackground);
- }
- success::~success()
- {
- delete ui;
- }
- void success::my_login_slot()
- {
- this->show();
- }
- void success::on_pushButton_clicked()
- {
- this->close();
- }
复制代码 widget.cpp
- #include "widget.h"
- #include "ui_widget.h"
- Widget::Widget(QWidget *parent)
- : QWidget(parent)
- , ui(new Ui::Widget)
- {
- ui->setupUi(this);
- this->setWindowFlag(Qt::FramelessWindowHint);
- this->setAttribute(Qt::WA_TranslucentBackground);
- ui->lineEdit_2->setEchoMode(QLineEdit::Password);
- connect(ui->btn2,SIGNAL(clicked()),this,SLOT(my_cancel_slot()));
- }
- Widget::~Widget()
- {
- delete ui;
- }
- void Widget::my_cancel_slot()
- {
- this->close();
- }
- void Widget::on_pushButton_clicked()
- {
- if(ui->lineEdit->text()=="admin"&&ui->lineEdit_2->text()=="123456")
- {
- int ret=QMessageBox::information(this,
- "登陆",
- "登陆成功",
- QMessageBox::Ok,
- QMessageBox::Ok);
- if(ret==QMessageBox::Ok)
- {
- emit my_login_signal();
- this->close();
- }
- }else
- {
- QMessageBox msg(QMessageBox::Critical,
- "错误",
- "账号和密码不匹配,是否重新登陆",
- QMessageBox::Yes|QMessageBox::No,
- this);
- int ret=msg.exec();
- if(ret==QMessageBox::Yes)
- {
- ui->lineEdit->setText("");
- ui->lineEdit_2->setText("");
- }else if (ret==QMessageBox::No)
- {
- this->close();
- }
- }
- }
- void Widget::on_pushButton_2_clicked()
- {
- QMessageBox msg(QMessageBox::Question,
- "退出",
- "您是否要退出登陆",
- QMessageBox::Yes|QMessageBox::No,
- this);
- int ret=msg.exec();
- if(ret==QMessageBox::Yes)
- {
- this->close();
- }
- // else if (ret==QMessageBox::No)
- // {
- // }
- }
复制代码 输入账号密码正确的情况:
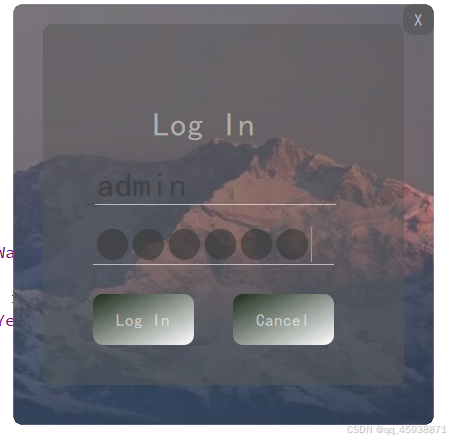
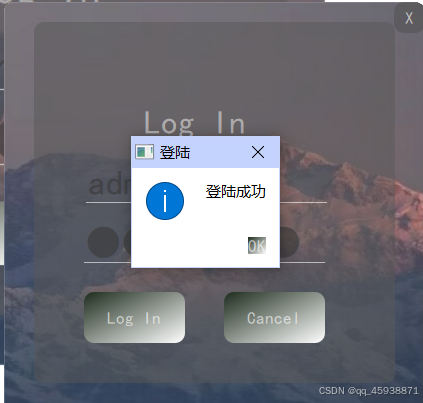
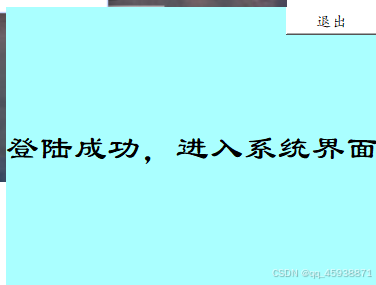
输入账号密码错误的情况:
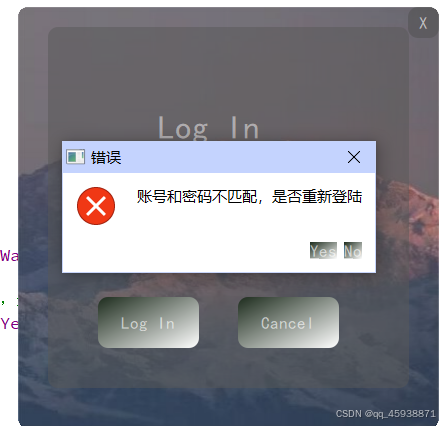
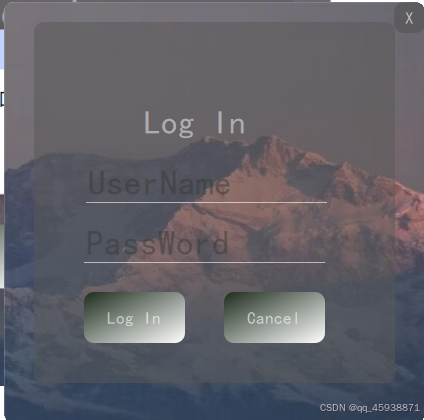
点击Cancel按钮:
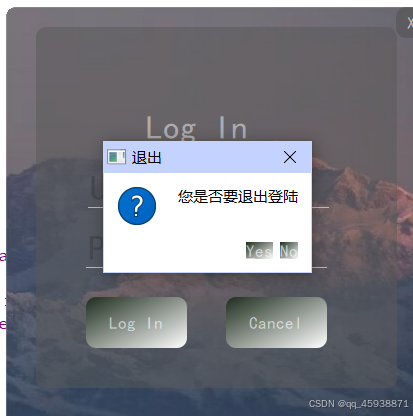
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |