什么是线性表
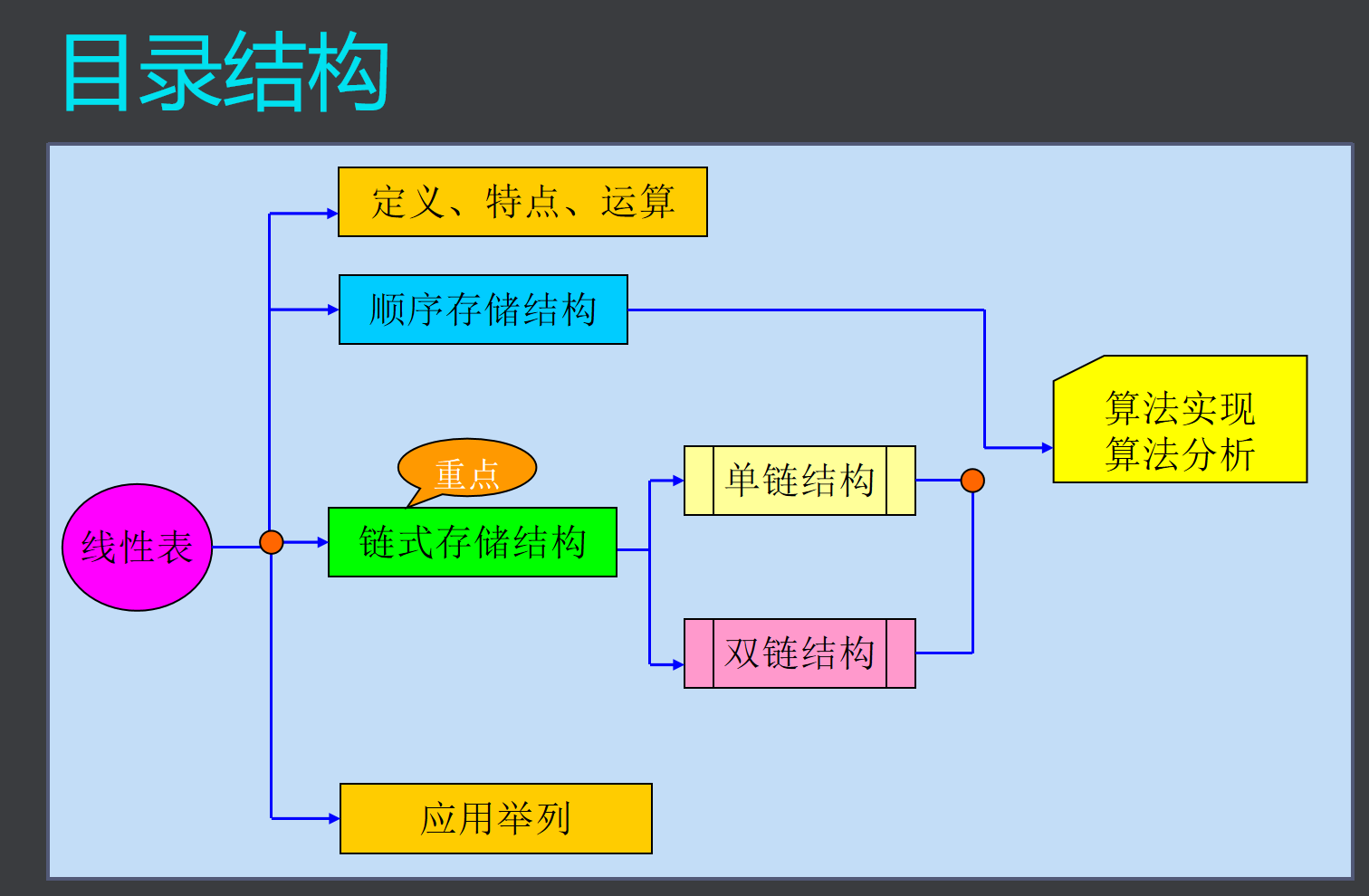
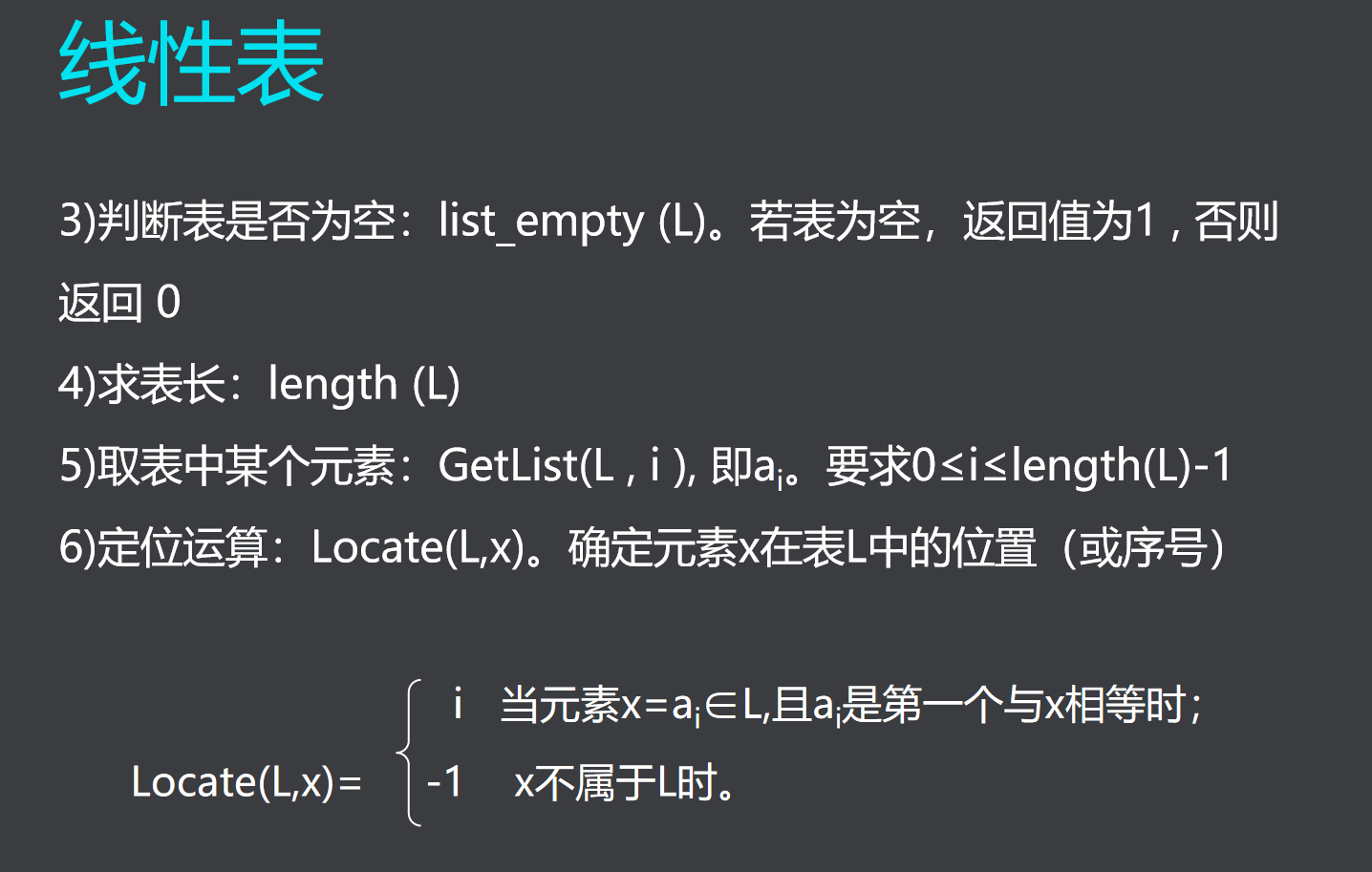
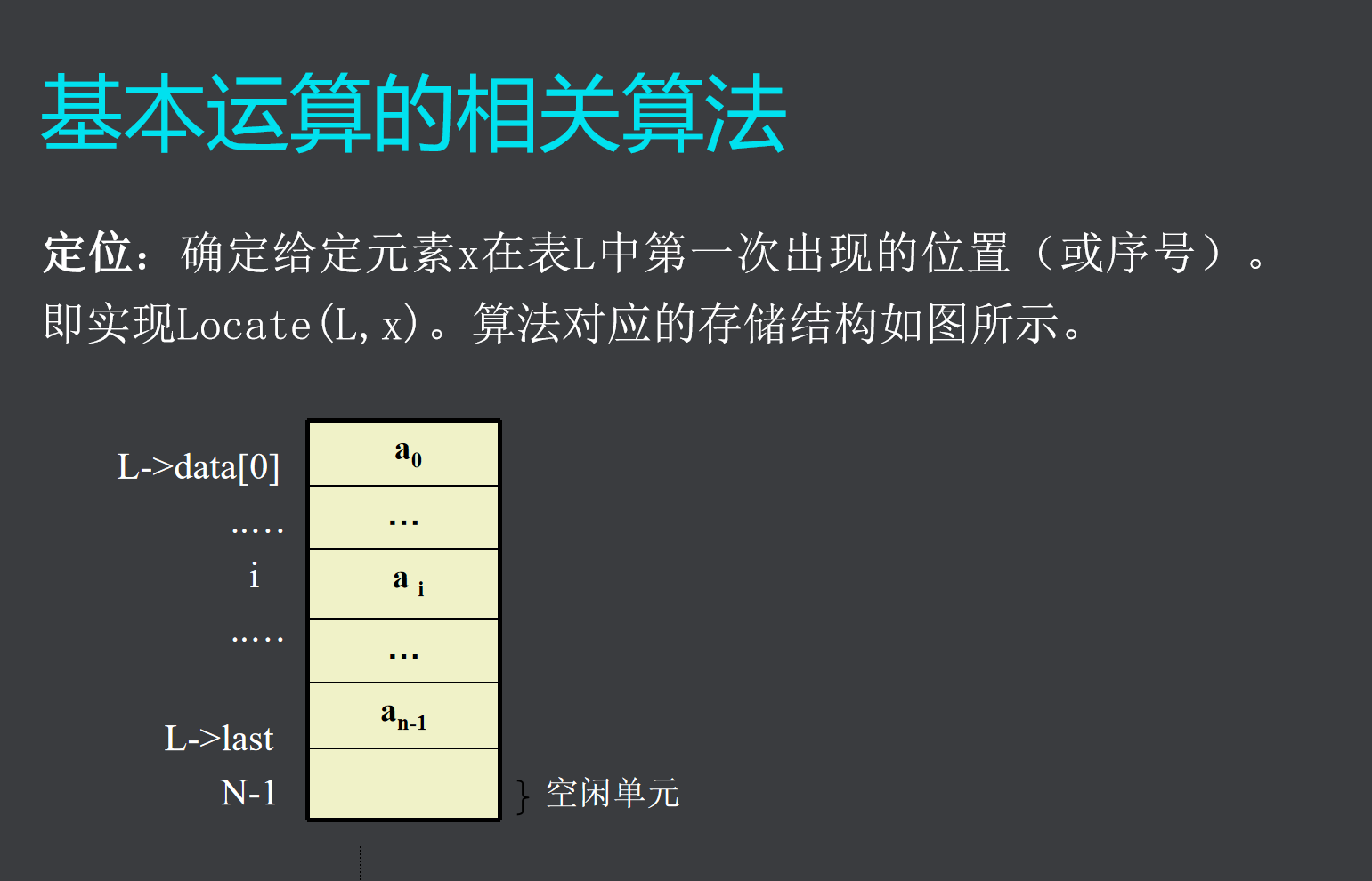
线性表的插入元素
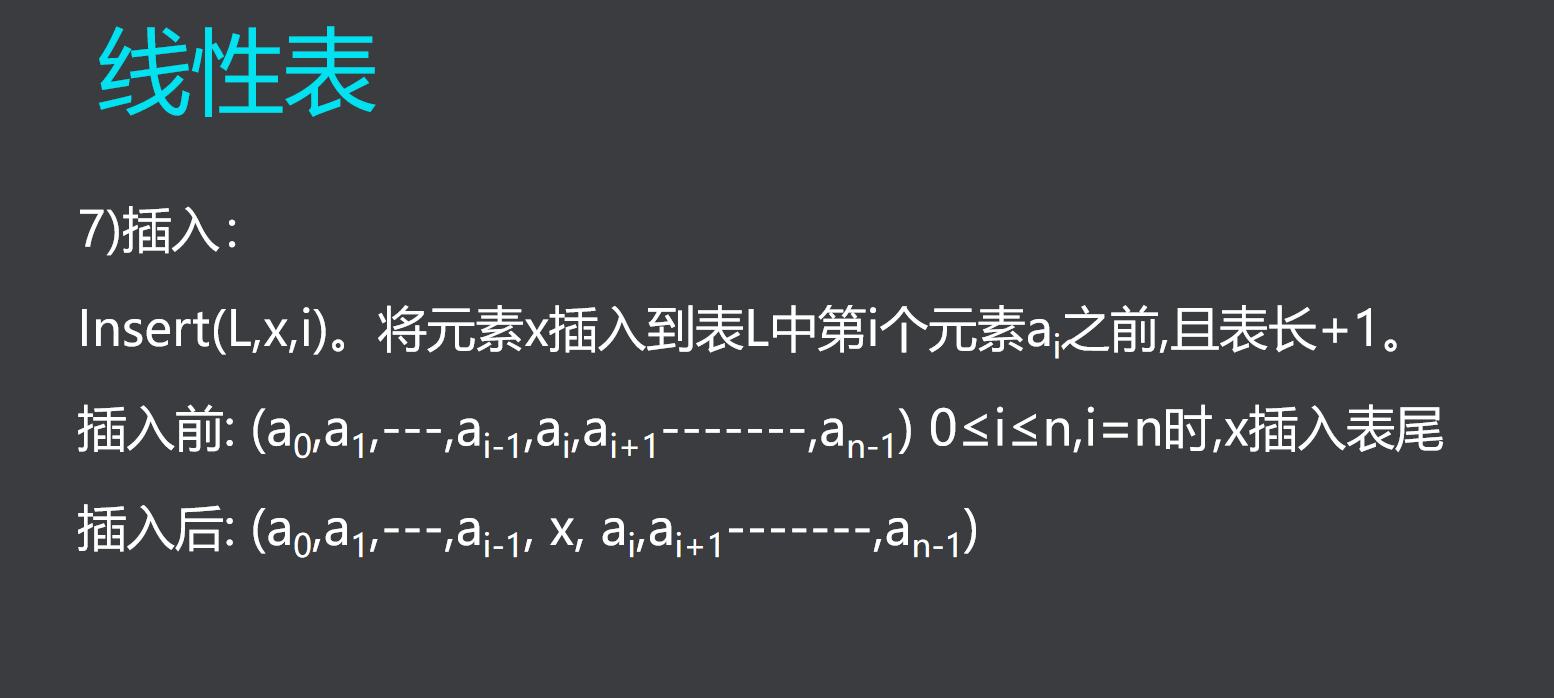
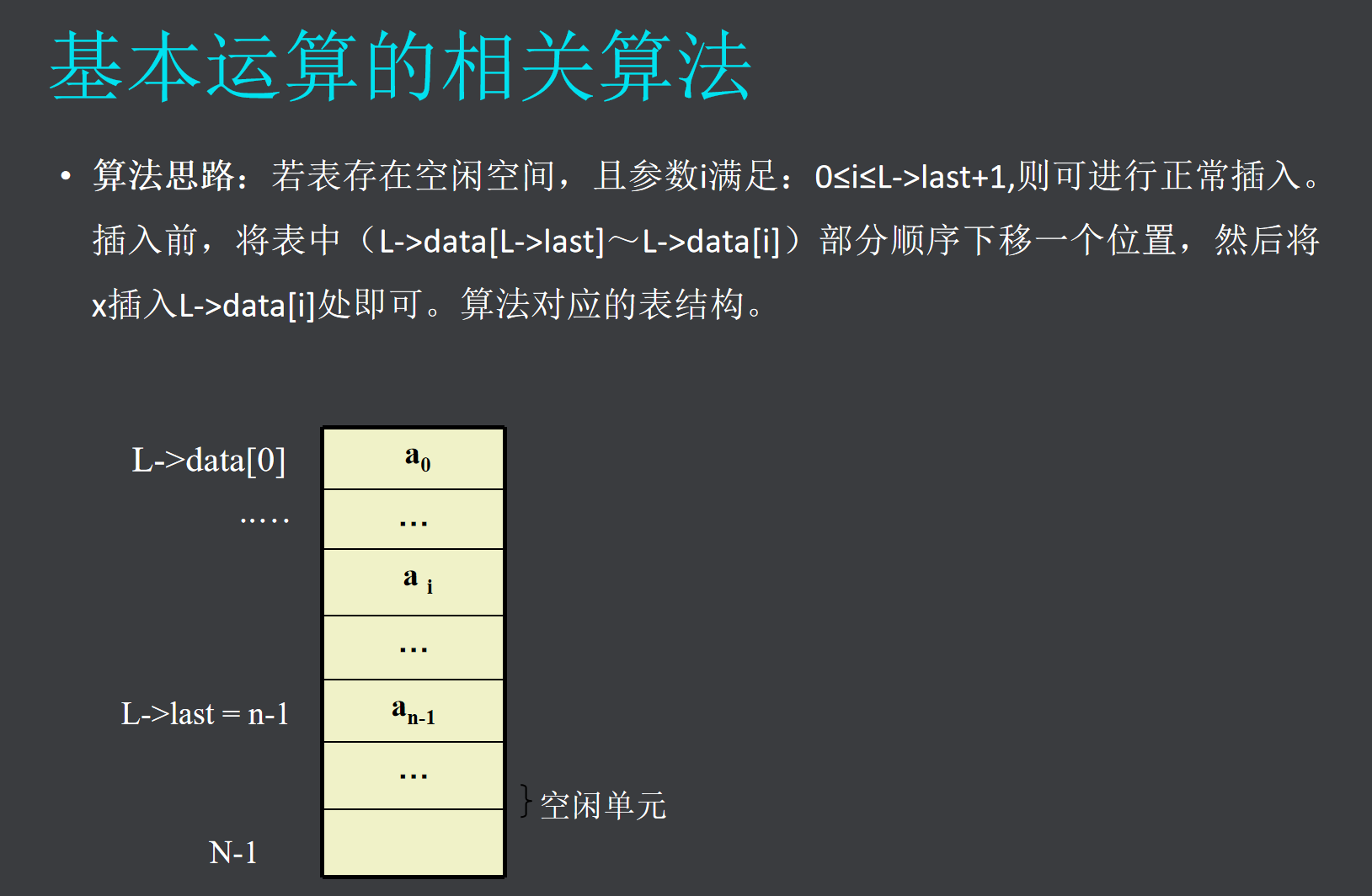
线性表的删除元素
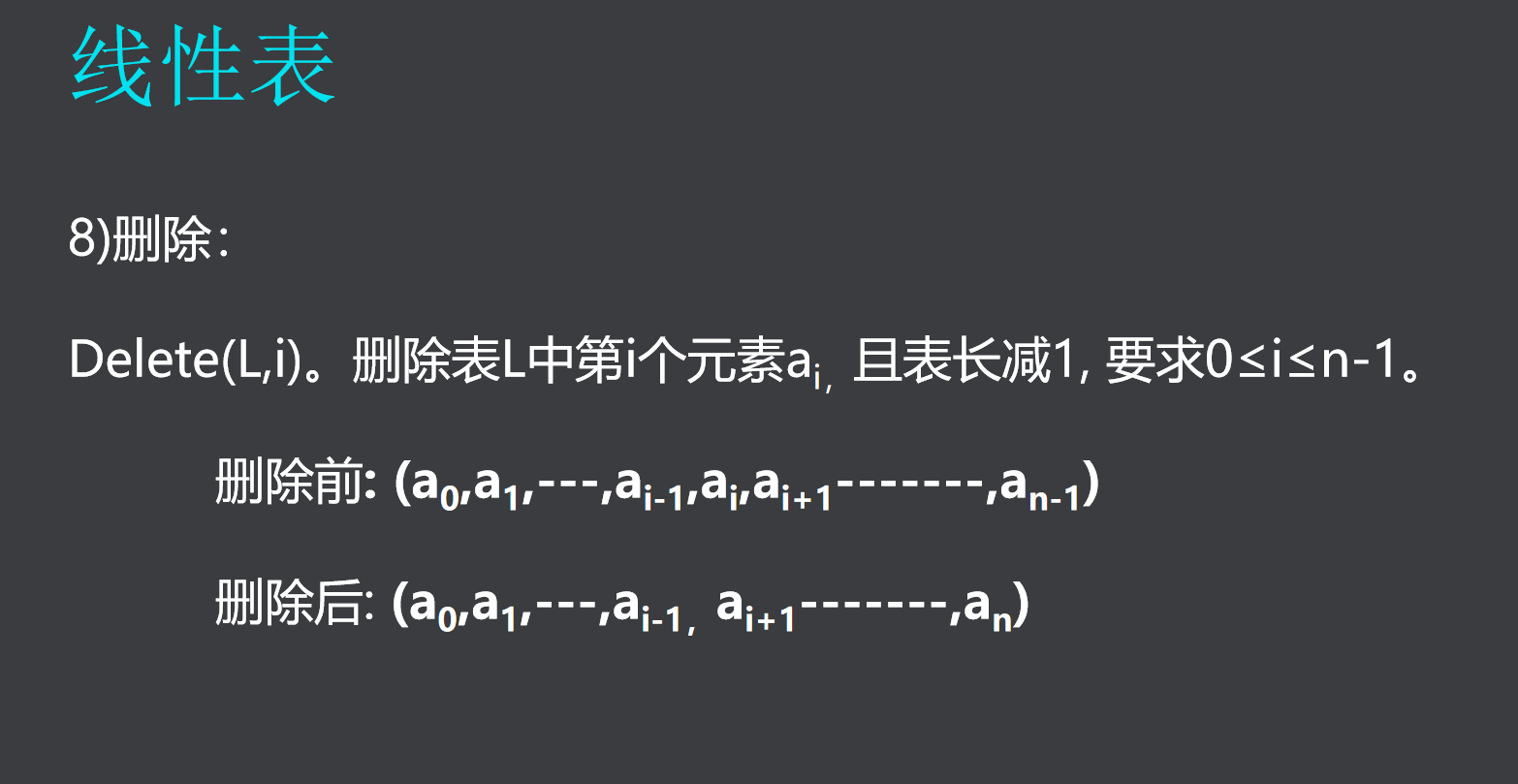
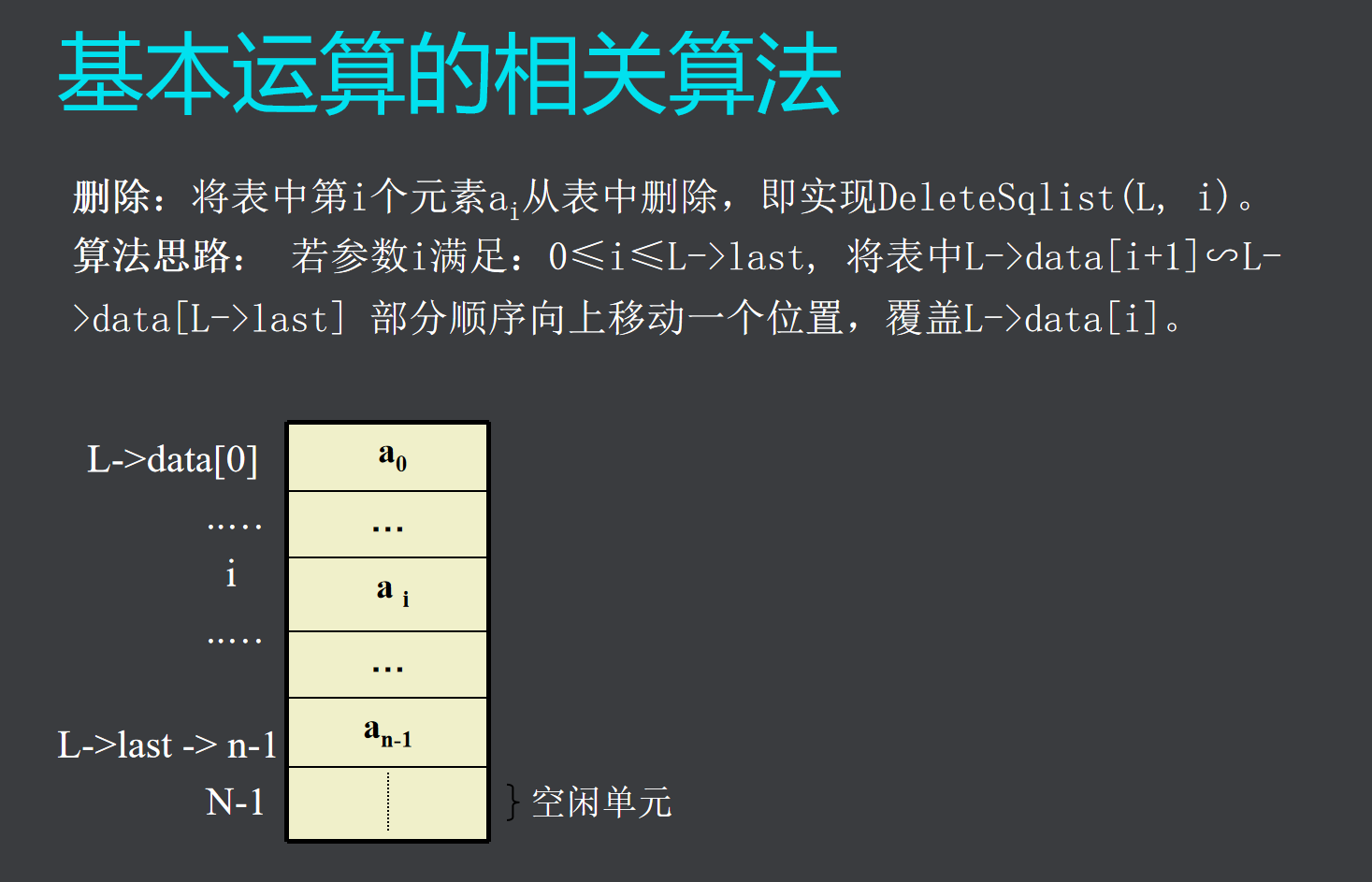
线性表顺序存储的缺点
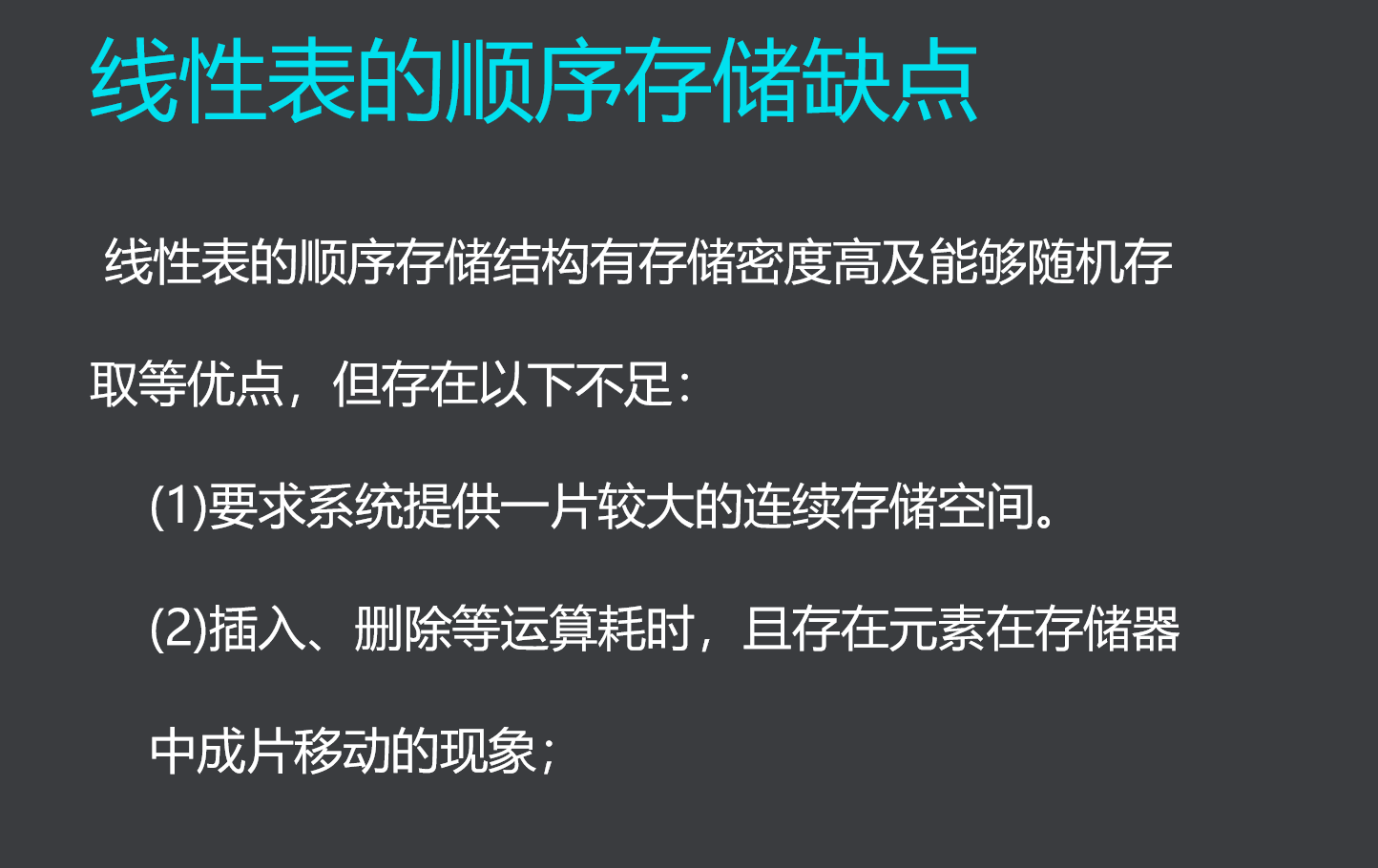
线性表的特点
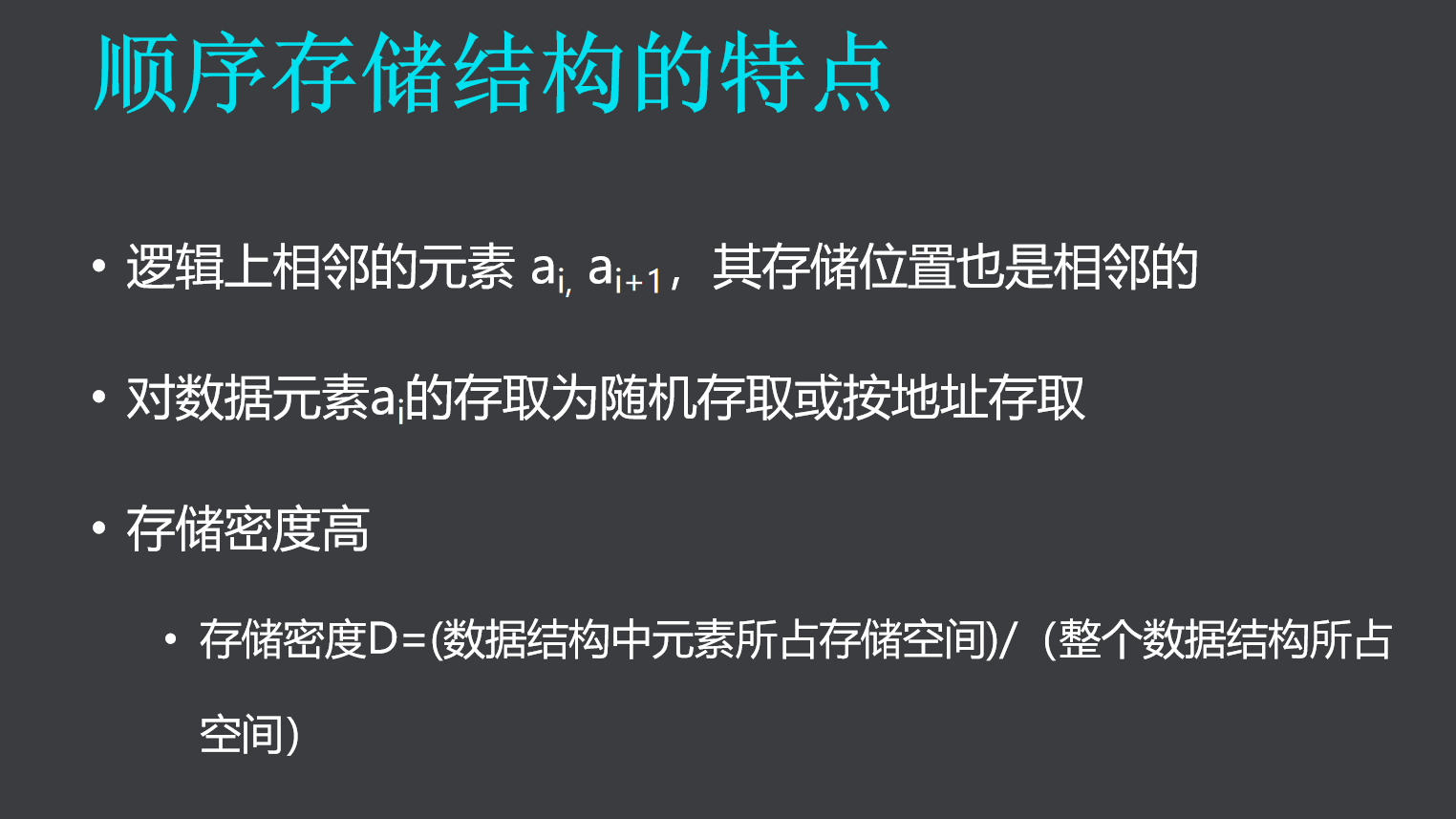
1.线性表的实例
首先我们创建3个文件,分别如下:
liner_data
--sqlist.c
--sqlist.h
--test.c- sqlist.h
- // .h文件中定位数据的结构以及函数的方法
- typedef int data_t;
- #define N 128 //定义一个宏
- typedef struct {
- data_t data[N];
- int last;
- } sqlist, *sqlink;
- sqlink list_create();
- int list_clear(sqlink L);
- int list_delete(sqlink L);
- int list_empty(sqlink L);
- int list_length(sqlink L);
- int list_locate(sqlink L, data_t value);
- int list_insert(sqlink L, data_t value, int pos);
- int list_show(sqlink L);
复制代码 下面编写sqlist.c文件:函数实现的功能- //
- // Created by Lenovo on 2023/9/9.
- //
- #include <stdio.h>
- #include <stdlib.h>
- #include <string.h>
- #include "sqlist.h"
- sqlink list_create(){
- // malloc
- sqlink L;
- L = (sqlink)malloc(sizeof(sqlist));
- if(L == NULL){
- printf("list malloc failed\n");
- return L;
- }
- // initialize
- memset(L, 0, sizeof(sqlist)); // 向数组中除了最后一个,其他全部初始化为0
- L->last = -1;
- return L;
- }
- int list_clear(sqlink L){
- /*
- * @return: 0-success -1-failed
- */
- if(L == NULL){
- return -1;
- }
- memset(L, 0, sizeof(sqlist));
- L->last = -1;
- return 0;
- }
- int list_delete(sqlink L){
- if(L==NULL)
- return -1;
- free(L); // 删除堆内存
- L=NULL;
- return 0;
- }
- /*
- * list_empty: Is list empty?
- * para L: list
- * @return: 1-empty 0-not empty
- */
- int list_empty(sqlink L){
- if(L->last == -1)
- return 1;
- else
- return 0;
- }
- int list_length(sqlink L){
- if(L==NULL)
- return -1;
- return (L->last+1);
- }
- int list_locate(sqlink L, data_t value){
- return 0;
- }
- int list_insert(sqlink L, data_t value, int pos){
- int i;
- // 判断是否满了full?
- if(L->last == N-1){
- printf("list is full\n");
- return -1;
- }
- // check para 0<=pos<=last+1 [0, last+1]
- if(pos<0 || pos>L->last+1){
- printf("Pos is invalid\n");
- return -1;
- }
- //move
- for (i=L->last; i>=pos; i--){
- L->data[i+1] = L->data[i];
- }
- // update value last
- L->data[pos] = value;
- L->last++;
- return 0;
- }
- int list_show(sqlink L){
- int i;
- if (L==NULL)
- return -1;
- if(L->last == -1)
- printf("list is empty\n");
- for(i=0; i<=L->last; i++){
- printf("%d ", L->data[i]);
- }
- puts(""); // 自动换行
- return 0;
- }
复制代码 test.c文件:main函数的执行入口- //
- // Created by Lenovo on 2023/9/9.
- //
- #include <stdio.h>
- #include "sqlist.h"
- void test_insert(){
- sqlink L;
- L=list_create();
- if (L==NULL)
- return;
- list_insert(L, 10, 0);
- list_insert(L, 20, 0);
- list_insert(L, 30, 0);
- list_insert(L, 40, 0);
- list_insert(L, 50, 0);
- list_show(L);
- list_insert(L, 100, -1000);
- list_show(L);
- list_delete(L);
- }
- int main(){
- test_insert();
- return 0;
- }
复制代码 2.执行步骤
2.1 使用gcc进行编译
c语言程序编译的过程如下:
预编译-编译-汇编-连接
汇编:gcc -c sqlist.c -o sqlist.o
gcc -c test.c -o test.o
连接:可执行文件:gcc sqlist.o test.o -o test
以上3步可直接等价于:gcc *.c -o test
程序运行成功:
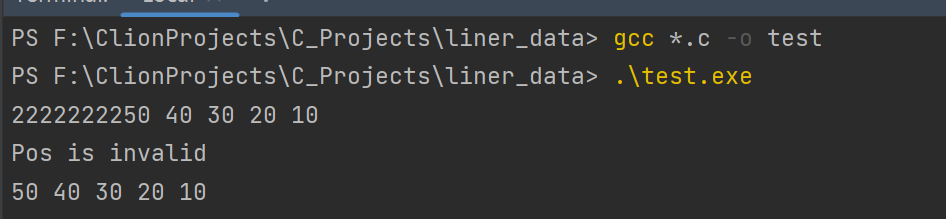
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |