技能支持:JAVA、JSP
服务器:TOMCAT 7.0.86
编程软件:IntelliJ IDEA 2021.1.3 x64
全部文件展示
网页实现功能截图
主页
注册
登录
购物车主页
修改功能
修改成功
添加商品功能
添加成功
添加进入购物车功能
付出功能
付出过的历史清单账单
全部代码
dao->StudentDAO

- package dao;
- import entiy.Product;
- import entiy.Student;
- import entiy.Total;
- import util.DBUtil;
- import java.sql.Connection;
- import java.sql.PreparedStatement;
- import java.sql.ResultSet;
- import java.sql.SQLException;
- import java.util.ArrayList;
- import java.util.List;
- public class StudentDAO {
- public List<Student> findAll() throws Exception {
- List<Student> students = new ArrayList<Student>();
- Connection conn = null;
- PreparedStatement prep = null;
- ResultSet rst = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement("select * from users");
- rst = prep.executeQuery();
- while (rst.next()) {
- int id = rst.getInt("id");
- String name = rst.getString("name");
- int idname = rst.getInt("idname");
- String pd = rst.getString("pd");
- Student e1 = new Student();
- e1.setName(name);
- e1.setIdname(idname);
- e1.setPd(pd);
- students.add(e1);
- }
- } catch (Exception e) {
- e.printStackTrace();
- throw e;
- } finally {
- DBUtil.close(conn);
- }
- return students;
- }
- public List<Product> findAllgoods() throws Exception {
- List<Product> products = new ArrayList<Product>();
- Connection conn = null;
- PreparedStatement prep = null;
- ResultSet rst = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement("select * from goods");
- rst = prep.executeQuery();
- while (rst.next()){
- int id = rst.getInt("id");
- String name = rst.getString("name");
- double price = rst.getDouble("price");
- Product e1 = new Product();
- e1.setId(id);
- e1.setName(name);
- e1.setPrice(price);
- products.add(e1);
- }
- } catch (Exception e) {
- e.printStackTrace();
- throw e;
- }finally {
- DBUtil.close(conn);
- }
- return products;
- }
- public List<Total> findtotals() throws Exception {
- List<Total> totals = new ArrayList<Total>();
- Connection conn = null;
- PreparedStatement prep = null;
- ResultSet rst = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement("select * from Total");
- rst = prep.executeQuery();
- while (rst.next()){
- int id = rst.getInt("id");
- double total = rst.getDouble("total");
- Total e1 = new Total();
- System.out.println(e1);
- e1.setId(id);
- e1.setTotal(total);
- totals.add(e1);
- }
- } catch (Exception e) {
- e.printStackTrace();
- throw e;
- }finally {
- DBUtil.close(conn);
- }
- return totals;
- }
- public void save(Student e) throws Exception {
- Connection conn = null;
- PreparedStatement prep = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement(
- "INSERT INTO users (name, idname, pd) VALUES (?, ?, ?)");
- prep.setString(1, e.getName());
- prep.setInt(2, e.getIdname());
- prep.setString(3, e.getPd());
- prep.executeUpdate();
- } catch (Exception e1) {
- e1.printStackTrace();
- throw e1;
- } finally {
- // Close PreparedStatement and Connection
- if (prep != null) {
- try {
- prep.close();
- } catch (SQLException e2) {
- e2.printStackTrace();
- }
- }
- DBUtil.close(conn);
- }
- }
- public void savegoods(Product e) throws Exception {
- Connection conn = null;
- PreparedStatement prep = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement(
- "INSERT INTO goods (name,price) VALUES (?, ?)");
- prep.setString(1, e.getName());
- prep.setDouble(2, e.getPrice());
- prep.executeUpdate();
- } catch (SQLException e1) {
- // Handle specific SQL exceptions or log them for debugging
- e1.printStackTrace();
- throw new Exception("Failed to save goods: " + e1.getMessage(), e1);
- } finally {
- // Close PreparedStatement and Connection in finally block
- if (prep != null) {
- try {
- prep.close();
- } catch (SQLException e2) {
- e2.printStackTrace();
- }
- }
- DBUtil.close(conn);
- }
- }
- public void delete(int id) throws Exception {
- Connection conn =null;
- PreparedStatement prep = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement("delete from emp where id="+id+"");
- prep.executeUpdate();
- }catch (Exception e){
- e.printStackTrace();
- throw e;
- }finally {
- DBUtil.close(conn);
- }
- }
- //根据ID查询商品信息(修改商品信息第一步)
- public Product findById(int id) throws Exception{
- Connection conn = null;
- PreparedStatement prep = null;
- ResultSet rst = null;
- Product e =new Product();
- try {
- conn =DBUtil.getConnection();
- System.out.println(conn);
- prep = conn.prepareStatement(
- "select * from goods where id="+id+"");
- System.out.println(id);
- rst = prep.executeQuery();
- if(rst.next()){
- int id1 = rst.getInt("id");
- String name = rst.getString("name");
- Double price = rst.getDouble("price");
- e.setId(id1);
- e.setName(name);
- e.setPrice(price);
- }
- }catch (Exception e1){
- e1.printStackTrace();
- throw e1;
- }finally {
- DBUtil.close(conn);
- }
- return e;
- }
- public void Update(int id,String name,double price) throws Exception {
- Connection connection = DBUtil.getConnection();
- PreparedStatement preparedStatement = connection.prepareStatement("update goods set name=?,price=? where id =?;");
- preparedStatement.setString(1,name);
- preparedStatement.setDouble(2,price);
- preparedStatement.setInt(3,id);
- int i = preparedStatement.executeUpdate();
- connection.close();
- }
- public void totalprice(Total e) throws Exception {
- Connection conn = null;
- PreparedStatement prep = null;
- try {
- conn = DBUtil.getConnection();
- prep = conn.prepareStatement(
- "INSERT INTO Total (total) VALUES (?)");
- prep.setDouble(1, e.getTotal());
- prep.executeUpdate();
- } catch (Exception e1) {
- e1.printStackTrace();
- throw e1;
- } finally {
- // Close PreparedStatement and Connection
- if (prep != null) {
- try {
- prep.close();
- } catch (SQLException e2) {
- e2.printStackTrace();
- }
- }
- DBUtil.close(conn);
- }
- }
- public static void main(String[] args) throws Exception {
- /* StudentDAO dao = new StudentDAO();
- Student e = new Student();
- e.setName("ww");
- e.setIdname(Integer.parseInt("123"));
- e.setPd("123");
- dao.save(e);
- System.out.println(e);
- */
- }
- }
复制代码 entity->CarItem -> roduct -> Student ->Total
- package entiy;
- // 可选的购物车条目类
- public class CarItem {
- private Product product;
- private int quantity;
- // 其他属性
- // 构造方法、getter和setter方法
- public CarItem(Product product, int quantity /*, 其他属性 */) {
- this.product = product;
- this.quantity = quantity;
- // 初始化其他属性
- }
- public Product getProduct() {
- return product;
- }
- public void setProduct(Product product) {
- this.product = product;
- }
- public int getQuantity() {
- return quantity;
- }
- public void setQuantity(int quantity) {
- this.quantity = quantity;
- }
- @Override
- public String toString() {
- return "CarItem{" +
- "product=" + product +
- ", quantity=" + quantity +
- '}';
- }
- }
复制代码- package entiy;
- public class Product {
- private int id;
- private String name;
- private double price;
- // 其他商品属性,如颜色、库存等
- // 构造方法、getter和setter方法
- public int getId() {
- return id;
- }
- public void setId(int id) {
- this.id = id;
- }
- public Product(/*, 其他属性 */) {
- this.name = name;
- this.price = price;
- // 初始化其他属性
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- public double getPrice() { return price; }
- public void setPrice(double price) {
- this.price = price;
- }
- @Override
- public String toString() {
- return "Product{" +
- "id=" + id +
- ", name='" + name + '\'' +
- ", price=" + price +
- '}';
- }
- }
复制代码- package entiy;
- public class Student {
- private String name;
- private int idname;
- private String pd;
- public String getName(){
- return name;
- }
- public void setName(String name){
- this.name=name;
- }
- public int getIdname(){
- return idname;
- }
- public void setIdname(int idname){
- this.idname = idname;
- }
- public String getPd(){
- return pd;
- }
- public void setPd(String pd){
- this.pd = pd;
- }
- @Override
- public String toString(){
- return "Student{"+
- "name="+name+
- "idname="+idname+
- ",pd="+pd+
- '}';
- }
- }
复制代码- package entiy;
- public class Total {
- private double total;
- private int id;
- public void setId(int id) {
- this.id = id;
- }
- public int getId() {
- return id;
- }
- public Total(/*, 其他属性 */) {
- this.total = total;
- this.id = id;
- // 初始化其他属性
- }
- public void setTotal(double total) {
- this.total = total;
- }
- public double getTotal() {
- return total;
- }
- @Override
- public String toString() {
- return "Total{" +
- "total=" + total +
- ", id=" + id +
- '}';
- }
- }
复制代码 Servlet包下的许多服务
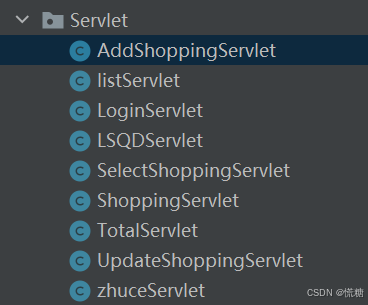 以下按顺序分列
- package Servlet;
- import dao.StudentDAO;
- import entiy.Product;
- import entiy.Student;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- import java.io.PrintWriter;
- public class AddShoppingServlet extends HttpServlet {
- public void service(HttpServletRequest request,
- HttpServletResponse response)
- throws ServletException, IOException {
- request.setCharacterEncoding("utf-8");
- //读取参数
- String name = request.getParameter("name");
- String price = request.getParameter("price");
- response.setContentType("text/html;charset=utf-8");
- PrintWriter out=response.getWriter();
- try {
- StudentDAO dao = new StudentDAO();
- //创建商品对象
- Product e1 = new Product();
- e1.setName(name);
- e1.setPrice(Double.parseDouble(price));
- System.out.println(e1.getName());
- System.out.println(e1.getPrice());
- dao.savegoods(e1);
- //重定向到主页面
- response.sendRedirect("list");
- } catch (Exception e) {
- e.printStackTrace();
- out.println("系统繁忙,请稍后再试!");
- }
- out.close();
- }
- }
复制代码
- package Servlet;
- import dao.StudentDAO;
- import entiy.Product;
- import javax.servlet.RequestDispatcher;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- import java.io.PrintWriter;
- import java.util.List;
- public class listServlet extends HttpServlet {
- public void service(HttpServletRequest request,
- HttpServletResponse response)
- throws ServletException, IOException {
- response.setContentType("text/html;charset=utf-8");
- PrintWriter out = response.getWriter();
- try {
- StudentDAO dao = new StudentDAO();
- List<Product> products = dao.findAllgoods();
- request.setAttribute("goods",products);
- RequestDispatcher rd = request.getRequestDispatcher("shopping.jsp");
- rd.forward(request,response);
- } catch (Exception e) {
- e.printStackTrace();
- out.println("系统繁忙,请稍后再试!");
- }
- }
- }
复制代码- package Servlet;
- import java.io.*;
- import java.sql.*;
- import javax.servlet.*;
- import javax.servlet.http.*;
- public class LoginServlet extends HttpServlet {
- // 数据库连接信息(这里假设你使用MySQL数据库)
- private String jdbcURL = "jdbc:mysql://localhost:3306/sdjyy";
- private String jdbcUsername = "root";
- private String jdbcPassword = "asd123";
- protected void doPost(
- HttpServletRequest request,
- HttpServletResponse response) throws ServletException, IOException {
- // 获取登录页面提交的账号和密码
- String username = request.getParameter("idname");
- String password = request.getParameter("pd");
- System.out.println(username);
- System.out.println(password);
- // 假设这里是你的登录逻辑,可以是数据库验证等
- boolean isValidUser = false;
- try {
- isValidUser = checkUser(username, password);
- } catch (Exception e) {
- e.printStackTrace();
- }
- // 这里进行账号密码验证的逻辑,比如查询数据库
- // 注意:这里应该使用 PreparedStatement 或其他安全方式来避免 SQL 注入攻击
- // 示例中简单输出账号密码到控制台
- System.out.println("Username: " + username);
- System.out.println("Password: " + password);
- if (isValidUser) {
- // 登录成功,重定向到商品页面
- HttpSession session = request.getSession();
- session.setAttribute("username", username);
- response.sendRedirect("list");
- } else {
- // 登录失败,可以返回到登录页面或者给出错误信息
- response.sendRedirect("denglu.jsp?error=1"); // 假设带有错误参数
- }
- }
- // 模拟用户验证,实际情况应根据你的业务逻辑实现
- private boolean checkUser(String username, String password) throws Exception {
- try {
- // 加载数据库驱动程序
- Class.forName("com.mysql.jdbc.Driver");
- // 连接数据库
- Connection connection = DriverManager.getConnection(jdbcURL, jdbcUsername, jdbcPassword);
- // 查询语句
- String sql = "SELECT * FROM users WHERE idname = ? AND pd = ?";
- PreparedStatement statement = connection.prepareStatement(sql);
- statement.setString(1, username);
- statement.setString(2, password);
- System.out.println(username);
- System.out.println(password);
- // 执行查询
- ResultSet result = statement.executeQuery();
- // 如果查询到结果集,说明用户名和密码匹配
- if (result.next()) {
- return true;
- }
- // 关闭连接
- connection.close();
- } catch (SQLException | ClassNotFoundException e) {
- e.printStackTrace();
- }
- // 如果没有查询到结果或者出现异常,则验证失败
- return false;
- }
- }
复制代码
- package Servlet;
- import dao.StudentDAO;
- import entiy.Total;
- import javax.servlet.RequestDispatcher;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- import java.io.PrintWriter;
- import java.util.List;
- public class LSQDServlet extends HttpServlet {
- public void service(HttpServletRequest request,
- HttpServletResponse response)
- throws ServletException, IOException {
- response.setContentType("text/html;charset=utf-8");
- PrintWriter out = response.getWriter();
- try {
- StudentDAO dao = new StudentDAO();
- List<Total> totals = dao.findtotals();
- request.setAttribute("total",totals);
- RequestDispatcher rd = request.getRequestDispatcher("Checkout.jsp");
- rd.forward(request,response);
- } catch (Exception e) {
- e.printStackTrace();
- out.println("系统繁忙,请稍后再试!");
- }
- }
- }
复制代码- package Servlet;
- import dao.StudentDAO;
- import entiy.Product;
- import javax.servlet.RequestDispatcher;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- import java.io.PrintWriter;
- public class SelectShoppingServlet extends HttpServlet {
- public void service(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException {
- request.setCharacterEncoding("utf-8");
- response.setContentType("text/html;charset=utf-8");
- StudentDAO studentDAO = new StudentDAO();
- String id = request.getParameter("id");
- System.out.println(id);
- PrintWriter writer = response.getWriter();
- try {
- Product product = studentDAO.findById(Integer.parseInt(id));
- request.setAttribute("goods",product );
- RequestDispatcher rd = request.getRequestDispatcher("Update.jsp");
- rd.forward(request,response);
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- }
- }
复制代码- package Servlet;
- import javax.servlet.*;
- import javax.servlet.http.*;
- import java.io.*;
- import java.sql.*;
- public class ShoppingServlet extends HttpServlet {
- // 数据库连接信息(这里假设你使用MySQL数据库)
- private String jdbcURL = "jdbc:mysql://localhost:3306/sdjyy";
- private String jdbcUsername = "root";
- private String jdbcPassword = "asd123";
- protected void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- HttpSession session = request.getSession();
- String idname = (String) session.getAttribute("idname");
- if (idname != null) {
- // 如果成功获取到 idname,可以继续处理逻辑
- // 在这里可以根据 idname 进行数据库查询或者其他业务逻辑
- Connection conn = null;
- PreparedStatement stmt = null;
- ResultSet rs = null;
- try {
- conn = DriverManager.getConnection(jdbcURL, jdbcUsername, jdbcPassword);
- String sql = "SELECT * FROM users WHERE idname = ?";
- stmt = conn.prepareStatement(sql);
- stmt.setString(1, idname);
- rs = stmt.executeQuery();
- if (rs.next()) {
- // 从结果集中获取其他信息或者执行其他操作
- String username = rs.getString("idname");
- System.out.println("Username retrieved: " + username);
- // 将用户名存储到 session 中(如果需要的话)
- session.setAttribute("username", username);
- // 转发到 shopping.jsp 页面或者其他需要的页面
- RequestDispatcher dispatcher = request.getRequestDispatcher("/shopping.jsp");
- dispatcher.forward(request, response);
- } else {
- // 如果未能从数据库中找到对应的记录,处理逻辑(例如跳转到错误页面)
- response.sendRedirect("/denglu.jsp");
- }
- } catch (SQLException se) {
- se.printStackTrace();
- response.getWriter().println("数据库连接错误");
- } finally {
- // 关闭资源
- try {
- if (rs != null) rs.close();
- if (stmt != null) stmt.close();
- if (conn != null) conn.close();
- } catch (SQLException se) {
- se.printStackTrace();
- }
- }
- } else {
- // 如果未能成功取 idname,处理逻辑(例如跳转到登录页面或者错误页面)
- response.sendRedirect("/denglu.jsp");
- }
- }
- }
复制代码- package Servlet;
- import dao.StudentDAO;
- import entiy.Product;
- import entiy.Total;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- import java.io.PrintWriter;
- public class TotalServlet extends HttpServlet {
- public void service(HttpServletRequest request,
- HttpServletResponse response)
- throws ServletException, IOException {
- request.setCharacterEncoding("utf-8");
- String total = request.getParameter("total");
- response.setContentType("text/html;charset=utf-8");
- PrintWriter out=response.getWriter();
- try {
- StudentDAO dao = new StudentDAO();
- Total e1 = new Total();
- e1.setTotal(Double.parseDouble(total));
- System.out.println(e1.getTotal());
- dao.totalprice(e1);
- response.sendRedirect("list");
- } catch (Exception e) {
- e.printStackTrace();
- out.println("系统繁忙,请稍后再试!");
- }
- out.close();
- }
- }
复制代码- package Servlet;
- import dao.StudentDAO;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- public class UpdateShoppingServlet extends HttpServlet {
- public void service(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException {
- StudentDAO productDAO = new StudentDAO();
- request.setCharacterEncoding("utf-8");
- response.setContentType("text/html;charset=utf-8");
- // Get parameters from request
- String idStr = request.getParameter("id");
- String name = request.getParameter("name");
- String priceStr = request.getParameter("price");
- // Validate parameters
- if (idStr == null || name == null || priceStr == null || idStr.isEmpty() || name.isEmpty() || priceStr.isEmpty()) {
- response.getWriter().println("Invalid parameters. Please provide all fields.");
- return;
- }
- try {
- // Parse parameters
- int id = Integer.parseInt(idStr);
- double price = Double.parseDouble(priceStr);
- // Update product in DAO
- productDAO.Update(id, name, price);
- // Redirect to list page after successful update
- response.sendRedirect("list");
- } catch (NumberFormatException e) {
- // Handle if id or price is not a valid number
- response.getWriter().println("Invalid id or price format. Please enter valid numbers.");
- } catch (Exception e) {
- // Handle other exceptions
- throw new ServletException("Error updating product", e);
- }
- }
- }
复制代码- package Servlet;
- import dao.StudentDAO;
- import entiy.Student;
- import javax.servlet.ServletException;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import java.io.IOException;
- import java.io.PrintWriter;
- public class zhuceServlet extends HttpServlet {
- public void service(HttpServletRequest request,
- HttpServletResponse response)
- throws ServletException, IOException {
- request.setCharacterEncoding("utf-8");
- String name = request.getParameter("name");
- String idname = request.getParameter("idname");
- String pd = request.getParameter("pd");
- response.setContentType("text/html;charset=utf-8");
- PrintWriter out = response.getWriter();
- try {
- if (name == null || idname == null || pd == null ||
- name.isEmpty() || idname.isEmpty() || pd.isEmpty()) {
- throw new IllegalArgumentException("参数不能为空");
- }
- StudentDAO dao = new StudentDAO();
- Student e1 = new Student();
- e1.setName(name);
- e1.setIdname(Integer.parseInt(idname));
- e1.setPd(pd);
- dao.save(e1);
- response.sendRedirect("denglu.jsp");
- } catch (NumberFormatException e) {
- out.println("ID必须是数字");
- } catch (IllegalArgumentException e) {
- out.println(e.getMessage());
- } catch (Exception e) {
- e.printStackTrace();
- out.println("系统繁忙,请稍后再试!");
- } finally {
- out.close();
- }
- }
- }
复制代码 util->DBUtil
- package util;
- import java.sql.Connection;
- import java.sql.DriverManager;
- import java.sql.SQLException;
- public class DBUtil {
- public static Connection getConnection() throws Exception {
- Connection conn = null;
- try {
- Class.forName("com.mysql.jdbc.Driver");
- conn = DriverManager.getConnection(
- "jdbc:mysql://localhost:3306/sdjyy?" +
- "useUnicode=true&characterEncoding=utf8","root","asd123"
- );
- } catch (Exception e) {
- e.printStackTrace();
- throw e;
- }
- return conn;
- }
- public static void close(Connection conn){
- if(conn!=null){
- try {
- conn.close();
- }catch (SQLException e){
- e.printStackTrace();
- }
- }
- }
- public static void main(String[] args)throws Exception{
- Connection conn = getConnection();
- System.out.println(conn);
- }
- }
复制代码
web.xml
- <?xml version="1.0" encoding="UTF-8"?>
- <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
- version="4.0">
- <servlet>
- <servlet-name>zhuce</servlet-name>
- <servlet-class>Servlet.zhuceServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>zhuce</servlet-name>
- <url-pattern>/zhuce</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>Login</servlet-name>
- <servlet-class>Servlet.LoginServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>Login</servlet-name>
- <url-pattern>/Login</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>Shopping</servlet-name>
- <servlet-class>Servlet.ShoppingServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>Shopping</servlet-name>
- <url-pattern>/Shopping</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>list</servlet-name>
- <servlet-class>Servlet.listServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>list</servlet-name>
- <url-pattern>/list</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>add</servlet-name>
- <servlet-class>Servlet.AddShoppingServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>add</servlet-name>
- <url-pattern>/add</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>load</servlet-name>
- <servlet-class>Servlet.SelectShoppingServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>load</servlet-name>
- <url-pattern>/load</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>update</servlet-name>
- <servlet-class>Servlet.UpdateShoppingServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>update</servlet-name>
- <url-pattern>/update</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>total</servlet-name>
- <servlet-class>Servlet.TotalServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>total</servlet-name>
- <url-pattern>/total</url-pattern>
- </servlet-mapping>
- <servlet>
- <servlet-name>LSQD</servlet-name>
- <servlet-class>Servlet.LSQDServlet</servlet-class>
- </servlet>
- <servlet-mapping>
- <servlet-name>LSQD</servlet-name>
- <url-pattern>/LSQD</url-pattern>
- </servlet-mapping>
- </web-app>
复制代码 JSP代码
AddShopping.jsp
- <%@page contentType="text/html;charset=utf-8" pageEncoding="utf-8" %>
- <%@page import="java.util.*,java.text.*,dao.*" %>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <title>Add Product</title>
- <link rel="stylesheet" href="css/style.css">
- <style>
- /* 这里可以添加页面特定的 CSS 样式 */
- body {
- font-family: Arial, sans-serif;
- background-color: #f0f0f0;
- margin: 0;
- padding: 0;
- }
- #wrap {
- width: 80%;
- margin: 0 auto;
- background-color: #fff;
- box-shadow: 0 0 10px rgba(0,0,0,0.1);
- }
- header {
- background-color: #333;
- color: #fff;
- padding: 10px;
- text-align: center;
- }
- #content {
- padding: 20px;
- }
- .form_table {
- width: 100%;
- }
- .form_table td {
- padding: 10px;
- }
- .inputgri {
- width: 100%;
- padding: 8px;
- border: 1px solid #ccc;
- border-radius: 4px;
- box-sizing: border-box;
- font-size: 14px;
- }
- .button {
- padding: 10px 20px;
- background-color: #4CAF50;
- color: white;
- border: none;
- border-radius: 4px;
- cursor: pointer;
- font-size: 14px;
- }
- .button:hover {
- background-color: #45a049;
- }
- #footer {
- background-color: #333;
- color: #fff;
- text-align: center;
- padding: 10px;
- }
- </style>
- </head>
- <body>
- <div id="wrap">
- <div id="content">
- <h1>添加商品:</h1>
- <form action="add" method="post">
- <table class="form_table">
- <tr>
- <td align="right">Name:</td>
- <td><input type="text" class="inputgri" name="name" /></td>
- </tr>
- <tr>
- <td align="right">Price:</td>
- <td><input type="text" class="inputgri" name="price" /></td>
- </tr>
- </table>
- <p><input type="submit" class="button" value="确认添加" /></p>
- </form>
- </div>
- <footer id="footer">
- sdjyw@126.com
- </footer>
- </div>
- </body>
- </html>
复制代码 Checkoust.jsp
- <%@ page import="entiy.Total" %>
- <%@ page import="java.util.*" %>
- <%@ page import="java.text.SimpleDateFormat" %>
- <%@page contentType="text/html;charset=utf-8" pageEncoding="utf-8" %>
- <html>
- <head>
- <title>Title</title>
- <style>
- .table {
- width: 100%;
- border-collapse: collapse;
- }
- .table_header {
- background-color: lightgray;
- }
- .table td, .table th {
- border: 1px solid black;
- padding: 8px;
- }
- .button {
- padding: 10px 20px;
- background-color: #4CAF50;
- color: white;
- border: none;
- text-align: center;
- text-decoration: none;
- display: inline-block;
- font-size: 16px;
- cursor: pointer;
- }
- </style>
- </head>
- <body>
- <h1>
- 查看您的历史清单
- </h1>
- <h2>
- <p>
- <%
- Date date = new Date();
- SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
- %>
- <%=sdf.format(date)%>
- <br />
- </p >
- </h2>
- <table class="table">
- <tr class="table_header">
- <td>
- ID
- </td>
- <td>
- 消费金额
- </td>
- </tr>
- <%
- List<Total> totals = (List<Total>) request.getAttribute("total");
- if (totals != null && !totals.isEmpty()) {
- for (int i = 0; i < totals.size(); i++) {
- Total e = totals.get(i);
- %>
- <tr>
- <td><%= e.getId() %></td>
- <td><%= e.getTotal() %></td>
- </tr>
- <%
- }
- } else {
- %>
- <tr>
- <td colspan="2">没有找到消费金额记录。</td>
- </tr>
- <%
- }
- %>
- </table>
- <p>
- <input type="button" class="button" value="返回主页面" onclick="location='list'"/>
- </p >
- </div>
- </div>
- <div id="footer">
- <div id="footer_bg">
- sdjyy@444.com
- </div>
- </div>
- </div>
- </body>
- </html>
复制代码 denglu.jsp
- <%@page contentType="text/html;charset=utf-8" pageEncoding="UTF-8" %>
- <%@page import="java.util.*,java.text.*,entiy.*" %>
- <!DOCTYPE html>
- <html>
- <head>
- <title>交易网登录</title>
- <script>
- // 检查是否有错误信息参数,如果有则显示弹框提示
- <% if ("1".equals(request.getParameter("error"))) { %>
- window.onload = function() {
- alert("您的账号或密码输入错误,请重试。");
- }
- <% } %>
- </script>
- <style>
- h1{
- font-size: 40px;
- color: blanchedalmond;
- text-align: center;
- font-family: 'Courier New', Courier, monospace;
- font-style: italic;
- }
- </style>
- </head>
- <body>
- <h1 name="top">交易网</h1>
- <table align="center" cellspacing="0">
- <tr>
- <td>
- <table cellpadding="0">
- <tr>
- <!-- 使用相对路径引用图片 -->
- <td><img src="img/3.jpg"></td>
- </tr>
- <tr>
- <td>
- <ul>
- <li>全面支持iPhone/iPad及Android等系统</li>
- <li>客户端、手机与网页,实现发送、阅读邮件立即同步普通登录手机号登录</li>
- </ul>
- </td>
- </tr>
- </table>
- </td>
- <td>
- <table border="1" cellspacing="0">
- <form action="Login" method="post">
- <tr>
- <td>
- <table cellpadding="30">
- <tr>
- <td colspan="2" align="center"><strong>登录</strong></td>
- </tr>
- <tr>
- <td>昵称:
- <input type="text" id="name" name="name">
- </td>
- </tr>
- <tr>
- <td>账号:
- <input type="text" id="idname" name="idname">
- </td>
- </tr>
- <tr>
- <td>密码:
- <input type="password" id="pd" name="pd">
- </td>
- </tr>
- <tr>
- <td align="center" colspan="2">
- <input type="submit" value="登录" onclick="f1();" />
- <!-- 注册按钮改用正确的 <a> 标签 -->
- <a href="zhuce.jsp"><input type="button" value="注册" onclick="f2();"></a>
- </td>
- </tr>
- </table>
- </td>
- </tr>
- </form>
- </table>
- </td>
- </tr>
- <tr>
- <td align="right">关于交易网</td>
- </tr>
- </table>
- </body>
- </html>
复制代码 index.jsp
- <%--
- Created by IntelliJ IDEA.
- User: NIL
- Date: 2024/7/17
- Time: 9:10
- To change this template use File | Settings | File Templates.
- --%>
- <%@ page contentType="text/html;charset=UTF-8" language="java" %>
- <html>
- <head>
- <title>$Title$</title>
- </head>
- <body>
- $END$
- </body>
- </html>
复制代码 shopping.jsp
Update.jsp
- <%@ page import="entiy.Product" %>
- <%@ page contentType="text/html;charset=UTF-8" language="java" %>
- <!DOCTYPE html>
- <html>
- <head>
- <title>修改商品信息</title>
- <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
- <link rel="stylesheet" type="text/css"
- href="css/style.css" />
- </head>
- <body>
- <div id="wrap">
- <div id="top_content">
- <div id="header">
- <div id="rightheader">
- </div>
- <div id="topheader">
- <h1 id="title">
- 商品修改
- </h1>
- </div>
- <div id="navigation">
- </div>
- </div>
- <div id="content">
- <p id="whereami">
- </p>
- <h1>
- 修改商品信息:
- </h1>
- <form action="update" method="post">
- <%
- Product product = (Product) request.getAttribute("goods");
- System.out.println(product.getId());
- %>
- <table cellpadding="0" cellspacing="0" border="0"
- class="form_table">
- <tr>
- <td valign="middle" align="right">
- 商品:
- </td>
- <td valign="middle" align="left">
- <input type="text" class="inputgri" name="name" value="<%=product.getName()%>" />
- </td>
- </tr>
- <tr>
- <td valign="middle" align="right">
- 价格:
- </td>
- <td valign="middle" align="left">
- <input type="text" class="inputgri" name="price" value="<%=product.getPrice()%>" />
- <input type="hidden" name="id" value="<%=product.getId()%>">
- </td>
- </tr>
- </table>
- <p>
- <input type="submit" class="button" value="提交" />
- </p>
- </form>
- </div>
- </div>
- <div id="footer">
- <div id="footer_bg">
- ABC@126.com
- </div>
- </div>
- </div>
- </body>
- </html>
复制代码 zhuce.jsp
- <%@page contentType="text/html;charset=utf-8" pageEncoding="UTF-8" %>
- <%@page import="java.util.*,java.text.*,entiy.*" %>
- <!DOCTYPE html>
- <html>
- <head>
- <title>交易网注册</title>
- <script>
- function f2(){
- alert("确认注册?");
- }
- </script>
- <style>
- h1 {
- font-size: 40px;
- color: blanchedalmond;
- text-align: center;
- font-family: 'Courier New', Courier, monospace;
- font-style: italic;
- }
- </style>
- </head>
- <body>
- <h1 name="top">交易网</h1>
- <table align="center" cellspacing="0">
- <tr>
- <td>
- <table border="1" cellspacing="0">
- <form action="zhuce" method="post">
- <tr>
- <td>
- <table cellpadding="30">
- <tr>
- <td colspan="2" align="center"><strong>注册</strong></td>
- </tr>
- <tr>
- <td>昵称:
- <input type="text" id="name" name="name">
- </td>
- </tr>
- <tr>
- <td>帐号:
- <input type="text" id="idname" name="idname">(输入九位以内整数字)
- </td>
- </tr>
- <tr>
- <td>密码:
- <input type="password" id="pd" name="pd">
- </td>
- </tr>
- <tr>
- <td align="center" colspan="2">
- <input type="submit" value="注册" onclick="f2();">
- </td>
- </tr>
- </table>
- </td>
- </tr>
- </form>
- </table>
- </td>
- </tr>
- <tr>
- <td align="right">关于交易网</td>
- </tr>
- </table>
- </body>
- </html>
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |