一、前言
在日常工作中,假如涉及到与第三方举行接口对接,有的会使用WebService的方式,这篇文章主要讲解在.NET Framework中如何调用WebService。
1.创建WebService
(1)新建项目——模板选择ASP.NET Web 应用程序
(2)选择空项目模板
(3)右击项目-添加-Web服务(ASMX)
(4)新建后会主动天生一个测试服务HelloWorld并返回执行字符串
(5)点击运行,并调用返回
二、方法一:静态引用
这种方式是通过添加静态引用的方式调用WebService
1.首先创建一个Winform程序,右击引用-添加服务引用。地址即为 运行的WebService地址,命名空间可自命名
2.计划Winform窗体,可选择工具箱button调用,TextBox入参
3.根据所需,调用WebService服务即可拿到返回参数
三、动态调用
上面使用静态引用的方式调用WebService,但是这种方式有一个缺点:假如发布的WebService地址改变,那么就要重新添加WebService的引用。假如是现有的WebService发生了改变,也要更新现有的服务引用,这需要把代码放到现场才可以。
使用动态调用WebService的方法可以解决该问题。
1.我们在配置文件里面添加配置,把WebService的地址、WebService提供的类名、要调用的方法名称,都写在配置文件里面
2.同样计划Winform界面,添加按钮,调用WebService服务
添加帮助类
- using System;
- using System.CodeDom.Compiler;
- using System.CodeDom;
- using System.Collections.Generic;
- using System.IO;
- using System.Linq;
- using System.Net;
- using System.Text;
- using System.Threading.Tasks;
- using System.Xml.Serialization;
- using System.Web;
- using System.Xml.Serialization;
- using System.Web.Caching;
- using System.Web.Services.Description;
- namespace ApiTest1
- {
- internal class WebServiceHelper
- {
- /// <summary>
- /// 生成dll文件保存到本地
- /// </summary>
- /// <param name="url">WebService地址</param>
- /// <param name="className">类名</param>
- /// <param name="methodName">方法名</param>
- /// <param name="filePath">保存dll文件的路径</param>
- public static void CreateWebServiceDLL(string url, string className, string methodName, string filePath)
- {
- // 1. 使用 WebClient 下载 WSDL 信息。
- WebClient web = new WebClient();
- Stream stream = web.OpenRead(url + "?WSDL");
- // 2. 创建和格式化 WSDL 文档。
- ServiceDescription description = ServiceDescription.Read(stream);
- //如果不存在就创建file文件夹
- if (Directory.Exists(filePath) == false)
- {
- Directory.CreateDirectory(filePath);
- }
- if (File.Exists(filePath + className + "_" + methodName + ".dll"))
- {
- //判断缓存是否过期
- var cachevalue = HttpRuntime.Cache.Get(className + "_" + methodName);
- if (cachevalue == null)
- {
- //缓存过期删除dll
- File.Delete(filePath + className + "_" + methodName + ".dll");
- }
- else
- {
- // 如果缓存没有过期直接返回
- return;
- }
- }
- // 3. 创建客户端代理代理类。
- ServiceDescriptionImporter importer = new ServiceDescriptionImporter();
- // 指定访问协议。
- importer.ProtocolName = "Soap";
- // 生成客户端代理。
- importer.Style = ServiceDescriptionImportStyle.Client;
- importer.CodeGenerationOptions = CodeGenerationOptions.GenerateProperties | CodeGenerationOptions.GenerateNewAsync;
- // 添加 WSDL 文档。
- importer.AddServiceDescription(description, null, null);
- // 4. 使用 CodeDom 编译客户端代理类。
- // 为代理类添加命名空间,缺省为全局空间。
- CodeNamespace nmspace = new CodeNamespace();
- CodeCompileUnit unit = new CodeCompileUnit();
- unit.Namespaces.Add(nmspace);
- ServiceDescriptionImportWarnings warning = importer.Import(nmspace, unit);
- CodeDomProvider provider = CodeDomProvider.CreateProvider("CSharp");
- CompilerParameters parameter = new CompilerParameters();
- parameter.GenerateExecutable = false;
- // 可以指定你所需的任何文件名。
- parameter.OutputAssembly = filePath + className + "_" + methodName + ".dll";
- parameter.ReferencedAssemblies.Add("System.dll");
- parameter.ReferencedAssemblies.Add("System.XML.dll");
- parameter.ReferencedAssemblies.Add("System.Web.Services.dll");
- parameter.ReferencedAssemblies.Add("System.Data.dll");
- // 生成dll文件,并会把WebService信息写入到dll里面
- CompilerResults result = provider.CompileAssemblyFromDom(parameter, unit);
- if (result.Errors.HasErrors)
- {
- // 显示编译错误信息
- System.Text.StringBuilder sb = new StringBuilder();
- foreach (CompilerError ce in result.Errors)
- {
- sb.Append(ce.ToString());
- sb.Append(System.Environment.NewLine);
- }
- throw new Exception(sb.ToString());
- }
- //记录缓存
- var objCache = HttpRuntime.Cache;
- // 缓存信息写入dll文件
- objCache.Insert(className + "_" + methodName, "1", null, DateTime.Now.AddMinutes(5), TimeSpan.Zero, CacheItemPriority.High, null);
- }
- /// <summary>
- /// 根据WebService的url地址获取className
- /// </summary>
- /// <param name="wsUrl">WebService的url地址</param>
- /// <returns></returns>
- //private string GetWsClassName(string wsUrl)
- //{
- // string[] parts = wsUrl.Split('/');
- // string[] pps = parts[parts.Length - 1].Split('.');
- // return pps[0];
- //}
- }
- }
复制代码 3.动态调用WebService代码:
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Configuration;
- using System.Data;
- using System.Drawing;
- using System.IO;
- using System.Linq;
- using System.Reflection;
- using System.Text;
- using System.Threading.Tasks;
- using System.Windows.Forms;
- namespace ApiTest1
- {
- public partial class Form1 : Form
- {
- public Form1()
- {
- InitializeComponent();
- }
- private void button1_Click(object sender, EventArgs e)
- {
- // 读取配置文件,获取配置信息
- string url = ConfigurationManager.AppSettings["WebServiceAddress"];
- string className = ConfigurationManager.AppSettings["ClassName"];
- string methodName = ConfigurationManager.AppSettings["MethodName"];
- string filePath = ConfigurationManager.AppSettings["FilePath"];
- // 调用WebServiceHelper
- WebServiceHelper.CreateWebServiceDLL(url, className, methodName, filePath);
- // 读取dll内容
- byte[] filedata = File.ReadAllBytes(filePath + className + "_" + methodName + ".dll");
- // 加载程序集信息
- Assembly asm = Assembly.Load(filedata);
- Type t = asm.GetType(className);
- // 创建实例
- object o = Activator.CreateInstance(t);
- MethodInfo method = t.GetMethod(methodName);
- // 参数
- string MsgCode = textBox1.Text;
- string SendXml = textBox2.Text;
- //string UserCode = textBox3.Text;
- object[] args = { MsgCode, SendXml};
- //object[] args = { "动态调用WebService" };
- // 调用访问,获取方法返回值
- string value = method.Invoke(o, args).ToString();
- //输出返回值
- MessageBox.Show($"返回值:{value}");
- }
- }
- }
复制代码 程序运行结果
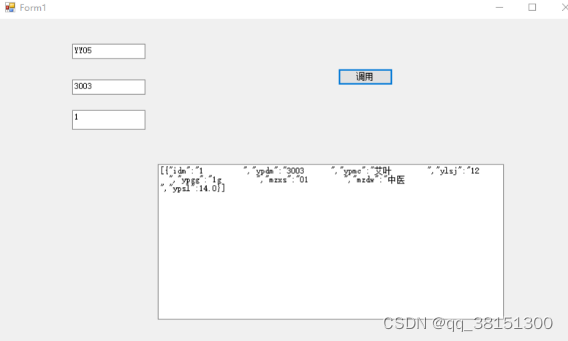
假如说类名没有提供,可以根据url来主动获取类名:
见帮助类(WebServiceHelper)中GetWsClassName 方法。
参考链接:C#调用WebService的方法先容
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |