pubspec.yaml设置http的SDK
- cupertino_icons: ^1.0.8
- http: ^1.2.2
复制代码 请求数据的格式转换
- // Map 转 json
- final chat = {
- 'name': '张三',
- 'message': '吃饭了吗',
- };
- final chatJson = json.encode(chat);
- print(chatJson);
- // json转Map
- final newChat = json.decode(chatJson);
- print(newChat);
复制代码 发送请求
- import 'dart:convert'; // json依赖
- import 'package:flutter/material.dart';
- import 'package:http/http.dart' as http; // http请求依赖
- class Chat {
- final String? name;
- final String? message;
- final String? imageUrl;
- Chat({this.name, this.message, this.imageUrl});
- //
- factory Chat.formMap(Map map) {
- return Chat(
- name: map['name'],
- message: map['message'],
- imageUrl: map['imageUrl'],
- );
- }
- }
- class ChatPage extends StatefulWidget {
- @override
- State<ChatPage> createState() => _ChatPageState();
- }
- class _ChatPageState extends State<ChatPage> {
- @override
- void initState() {
- super.initState();
- getDatas().then((value) {
-
- }).catchError((onError) {
-
- }).whenComplete(() {
- print('数据加载完毕');
- }).timeout(Duration(seconds: 1)).catchError((err) {
- print('超时时长1秒,提示请求超时');
- });
- }
- }
- // async:异步的请求,不用卡在这等待
- Future<List> getDatas() async {
- final url =
- Uri.parse('http://rap2api.taobao.org/app/mock/321326/api/chat/list');
- final res = await http.get(url);
- if (res.statusCode == 200) {
- // 获取相应数据,转成Map类型
- final resBody = json.decode(res.body);
- // map遍历chat_list,把每一项item转成模型数据,最后转成List
- List chatList =
- resBody['chat_list'].map((item) => Chat.formMap(item)).toList();
- return chatList;
- } else {
- throw Exception('statusCode:${res.statusCode}');
- }
- }
复制代码 FutureBuilder 发送的请求
- return Scaffold(
- body: Container(
- child: FutureBuilder(
- future: getDatas(),
- builder: (BuildContext context, AsyncSnapshot snapshot) {
- print('data:${snapshot.data}');
- if (snapshot.connectionState == ConnectionState.waiting) {
- return const Center(
- child: Text('Loading...'),
- );
- }
- return ListView(
- children: snapshot.data.map<Widget>((item) {
- return ListTile(
- title: Text(item.name),
- subtitle: Container(
- alignment: Alignment.bottomCenter,
- height: 25,
- child: Text(
- item.message,
- overflow: TextOverflow.ellipsis,
- ),
- ),
- leading: Container(
- width: 44,
- height: 44,
- decoration: BoxDecoration(
- borderRadius: BorderRadius.circular(6.0),
- image: DecorationImage(
- image: NetworkImage(item.imageUrl))),
- ));
- }).toList(),
- );
- }),
- )
- );
复制代码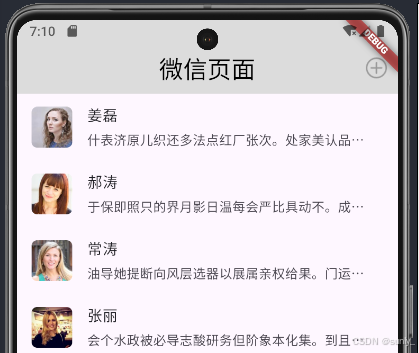
声明变量接收接口数据,在页面中利用该变量去渲染页面的方式
- class _ChatPageState extends State<ChatPage> {
- List _datas = [];
- @override
- void initState() {
- super.initState();
- getDatas().then((value) {
- setState(() {
- _datas = value;
- });
- }).catchError((onError) {
-
- });
- }
- }
- body: Container(
- child: Container(
- child: _datas.length == 0 ? Center(child: Text('Loading...')) :
- ListView.builder(
- itemCount: _datas.length,
- itemBuilder: (BuildContext context, int index) {
- return ListTile(
- title: Text(_datas[index].name),
- subtitle: Container(
- alignment: Alignment.bottomCenter,
- height: 25,
- child: Text(
- _datas[index].message,
- overflow: TextOverflow.ellipsis,
- ),
- ),
- leading: Container(
- width: 44,
- height: 44,
- decoration: BoxDecoration(
- borderRadius: BorderRadius.circular(6.0),
- image: DecorationImage(image: NetworkImage(_datas[index].imageUrl))
- ),
- )
- );
- },
- )
- )
- )
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。
|