让我们使用所学知识来开辟一个简单的购物车
(记得暴露属性和方法!!!)
起首来看一下最基本的一个html框架
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <title>实战小项目:购物车</title>
-
- <style>
- body {
- font-family: Arial, sans-serif;
- }
- .cart-item {
- width: 50%;
- margin-bottom: 15px;
- padding: 10px;
- border: 2px solid gray;
- border-radius: 10px;
- background-color: #ddd;
- }
- .buttons {
- margin-top: 5px;
- }
- .buttons button {
- padding: 5px 10px;
- margin-right: 5px;
- font-size: 16px;
- cursor: pointer;
- border: none;
- border-radius: 3px;
- background-color: pink;
- }
- .buttons input {
- width: 25px;
- }
- .buttons button:hover {
- background-color: yellow;
- }
- .quantity {
- font-size: 18px;
- font-weight: bold;
- margin-left: 10px;
- }
- h1, h2 {
- color: #333;
- }
- </style>
- </head>
- <body>
- <div id="app">
- <h1>实战小项目:购物车</h1>
-
- <!-- 提示:可以使用v-for指令,假设有n个品类,则生成n个商品项-->
- <div class="cart-item">
- <div class="buttons">
- <span>苹果 </span>
- <button>-</button>
- <span class="quantity">1 </span>
- <button>+</button>
- <p>
- 请输入价格:
- <input type="text"/> 元/斤 <br>
- 单价:
- 1 元/斤
- </p>
- </div>
- </div>
-
- <!-- 提示:可以用计算属性或数据变动侦听器,跟踪商品数和单价的变化,进而求出总数和总价-->
- <h3>商品总数: <ins> 1 </ins> 件</h3>
- <h3>商品总价: <ins> 1 </ins> 元</h3>
-
- </div>
-
- <script type="module">
- import { createApp, reactive, computed } from './vue.esm-browser.js'
- createApp({
- setup() {
-
- // 1.定义属性:存储商品的(响应式)数组
-
- // 2.定义方法:增加商品数
-
- // 3.定义方法:减少商品数
-
- // 4.定义方法:计算商品总数
-
- // 5.定义方法:计算商品总价
-
- // 6.暴露属性和方法
- }
- }).mount('#app');
- </script>
- </body>
- </html>
复制代码 结果如下:
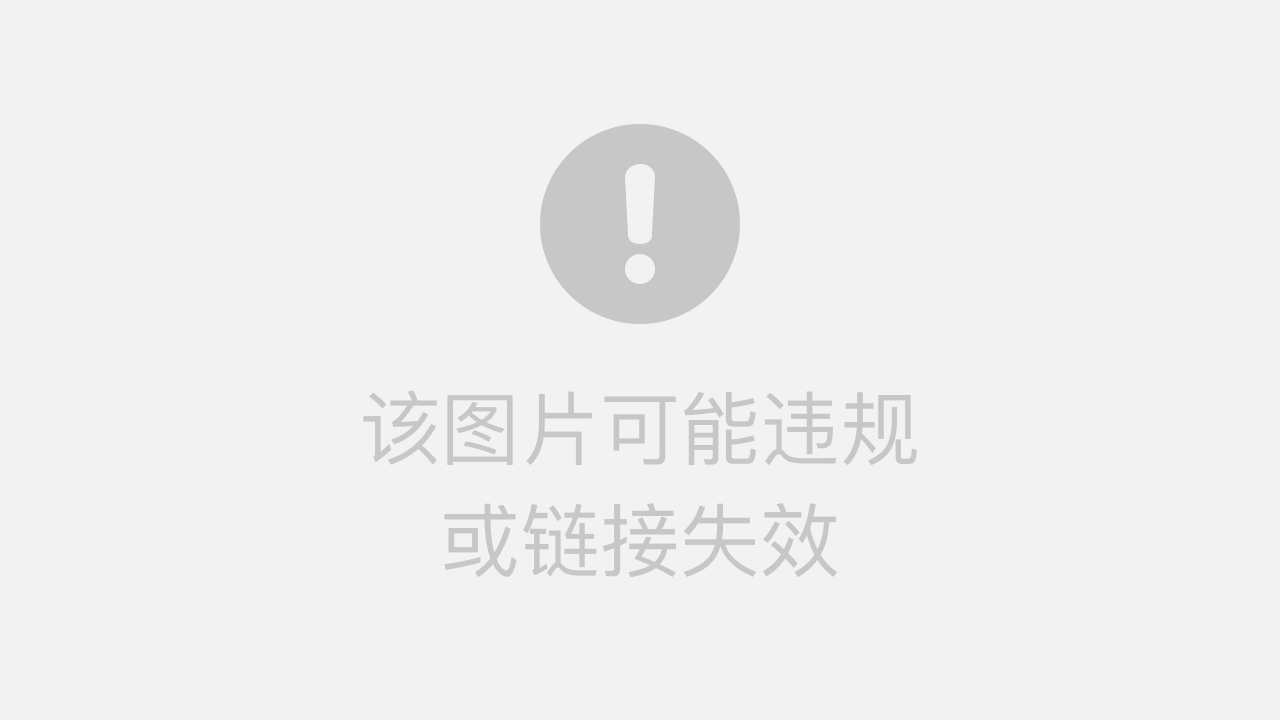
目前是一个静态的一个网页
起首界说属性:存储商品的(响应式)数组(使用v-for遍历)
- <div class="cart-item" v-for="(item,index) in cartItems">
- const cartItems = reactive([
- { name: '苹果', quantity: 1, unit_price: 1 },
- { name: '香蕉', quantity: 1, unit_price: 1 },
- { name: '菠萝', quantity: 1, unit_price: 1 },
-
- ]);
复制代码
我们可以发现我们已经做出了三个链接,但是名字都是苹果,这个时候我们添加一个插值即可
- <span>{{item.name}} </span>
复制代码
那么我们如何控制商品的增长和淘汰呢
我们只必要界说一个函数 而且使用v-on的方法绑定即可,事件触发为点击
留意:在做淘汰按钮时,我们要通过if判定限定一下商品数,否则会出现负数
- <button v-on:click = "pre(index)">-</button>
- <span class="quantity">{{cartItems[index].quantity}} </span>
- <button v-on:click = "next(index)">+</button>
- // 2.定义方法:增加商品数
- const next = (index) =>{
- cartItems[index].quantity ++;
-
-
- }
- // 3.定义方法:减少商品数
- const pre = (index) => {
- if ( cartItems[index].quantity>1) {
- cartItems[index].quantity --;
- }
-
- }
复制代码
接着我们要计算商品的总数,那么该如何计算呢
可以用计算属性或数据变更侦听器,跟踪商品数和单价的变化,进而求出总数和总价
- <h3>商品总数: <ins> {{totalItem()}} </ins> 件</h3>
- // 4.定义方法:计算商品总数
- const totalItem = () =>{
- let total_items = 0 ;
- for(const item of cartItems){
- total_items +=item.quantity;
-
- }
- return total_items
- }
-
复制代码
计算完总数,我们就该来计算总价了,同理可得使用computer计算属性,界说一个变量,在函数内里写一个for遍历即可(输入框内里属于写一个双向绑定v-model从而实现单价变化总价随着变化)
- <input type="text" v-model="cartItems[index].unit_price"> 元/斤 <br>
- <h3>商品总价: <ins> {{totalprice}} </ins> 元</h3>
- // 5.定义方法:计算商品总价
- const totalprice = computed(()=>
- {
- let total_price = 0;
- for(const money of cartItems){
- total_price += money.unit_price*money.quantity;
- }
- return total_price
- })
-
复制代码
如许我们一个简单的购物车便开辟成功啦
完备代码如下:
- <!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>实战小项目:购物车</title> <style> body { font-family: Arial, sans-serif; } .cart-item { width: 50%; margin-bottom: 15px; padding: 10px; border: 2px solid gray; border-radius: 10px; background-color: #ddd; } .buttons { margin-top: 5px; } .buttons button { padding: 5px 10px; margin-right: 5px; font-size: 16px; cursor: pointer; border: none; border-radius: 3px; background-color: pink; } .buttons input { width: 25px; } .buttons button:hover { background-color: yellow; } .quantity { font-size: 18px; font-weight: bold; margin-left: 10px; } h1, h2 { color: #333; } </style></head><body> <div id="app"> <h1>实战小项目:购物车</h1> <!-- 提示:可以使用v-for指令,假设有n个品类,则天生n个商品项--> <div class="cart-item" v-for="(item,index) in cartItems"> <div class="buttons"> <span>{{item.name}} </span> <button v-on:click = "pre(index)">-</button> <span class="quantity">{{cartItems[index].quantity}} </span> <button v-on:click = "next(index)">+</button> <p> 请输入价格: <input type="text" v-model="cartItems[index].unit_price"> 元/斤 <br> 单价: 1 元/斤 </p> </div> </div> <!-- 提示:可以用计算属性或数据变更侦听器,跟踪商品数和单价的变化,进而求出总数和总价--> <h3>商品总数: <ins> {{totalItem()}} </ins> 件</h3> <h3>商品总价: <ins> {{totalprice}}</ins> 元</h3> </div> <script type="module"> import { createApp, reactive, computed } from './vue.esm-browser.js' createApp({ setup() { // 1.界说属性:存储商品的(响应式)数组 const cartItems = reactive([ { name: '苹果', quantity: 1, unit_price: 1 }, { name: '香蕉', quantity: 1, unit_price: 1 }, { name: '菠萝', quantity: 1, unit_price: 1 }, ]); // 2.界说方法:增长商品数 const next = (index) =>{ cartItems[index].quantity ++; } // 3.界说方法:淘汰商品数 const pre = (index) => { if ( cartItems[index].quantity>1) { cartItems[index].quantity --; } } // 4.界说方法:计算商品总数 const totalItem = () =>{ let total_items = 0 ; for(const item of cartItems){ total_items +=item.quantity; } return total_items } // 5.界说方法:计算商品总价 const totalprice = computed(()=> { let total_price = 0; for(const money of cartItems){ total_price += money.unit_price*money.quantity; } return total_price }) // 6.暴露属性和方法 return { cartItems, pre, next, totalItem, totalprice, }; }, }).mount('#app'); </script></body></html>
复制代码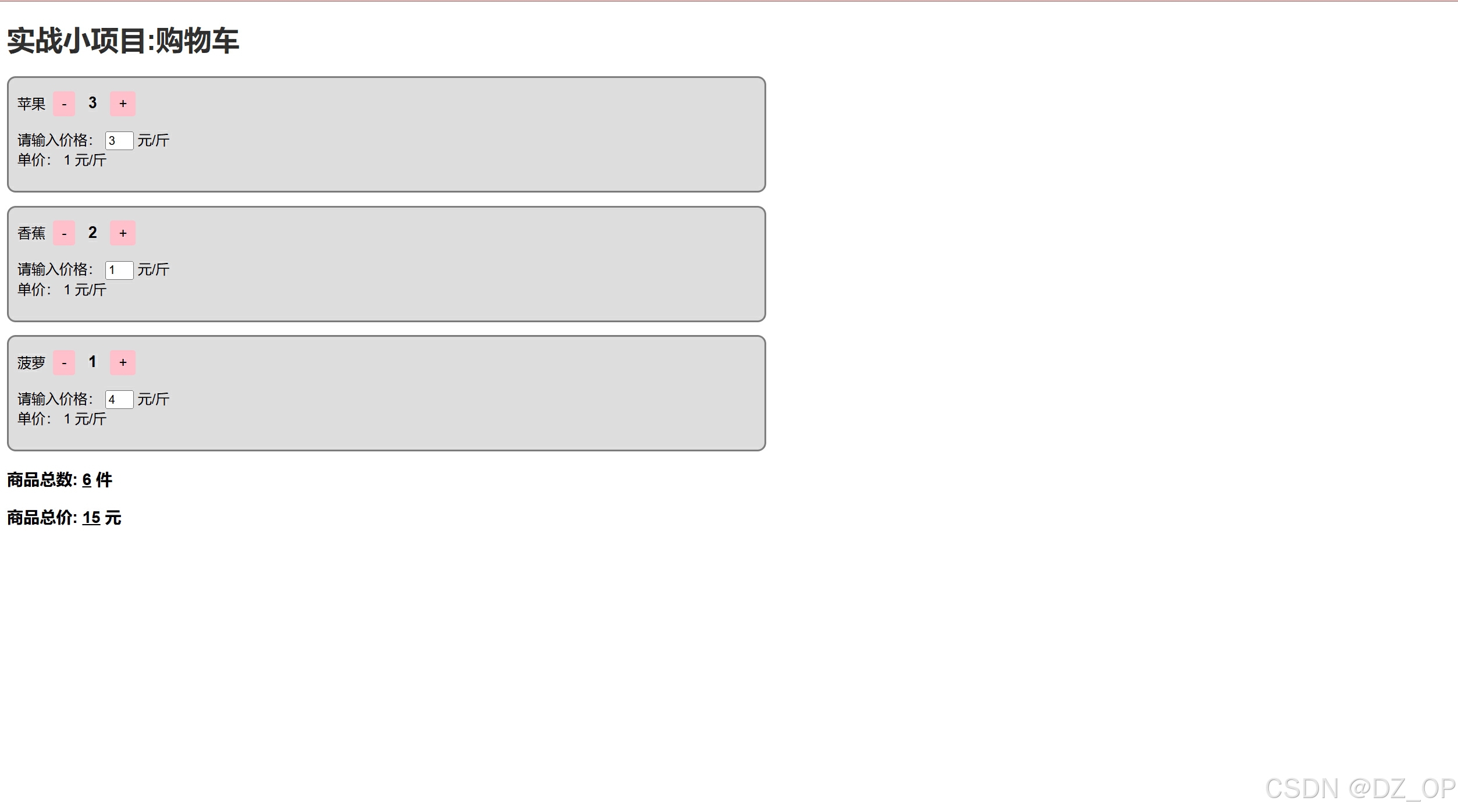
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |