一、注解基本信息
1.声明bean的注解
Spring注解描述@Component组件,没有明确的角色@Service在业务逻辑层使用(service层)@Repository标注在 DAO 类上,这是因为该注解的作用不只是将类识别为Bean,同时它还能将所标注的类中抛出的数据访问异常封装为 Spring 的数据访问异常类型。 Spring本身提供了一个丰富的并且是与具体的数据访问技术无关的数据访问异常结构,用于封装不同的持久层框架抛出的异常,使得异常独立于底层的框架
@Controller定义文档的主体2.注入bean的注解
Spring注解描述@AutowiredSpring提供的工具(由Spring的依赖注入工具(BeanPostProcessor、BeanFactoryPostProcessor)自动注入。)
它可以对类成员变量、方法及构造函数进行标注,完成自动装配的工作。 通过 @Autowired的使用来消除 set ,get方法
@Inject由JSR-330提供@Resource由JSR-250提供3.java配置类相关注解
Spring注解描述@Configuration声明当前类为配置类,相当于xml形式的Spring配置(类上)@Bean注解在方法上,声明当前方法的返回值为一个bean,替代xml中的方式(方法上)@Configuration声明当前类为配置类,其中内部组合了@Component注解,表明这个类是一个bean(类上)@ComponentScan用于对Component进行扫描,相当于xml中的context:component-scan(类上)@WishlyConfiguration为@Configuration与@ComponentScan的组合注解,可以替代这两个注解4.切面(AOP)相关注解
Spring注解描述@Aspect声明一个切面(类上),使用@After、@Before、@Around定义建言(advice),可直接将拦截规则(切点)作为参数。@After在方法执行之后执行(方法上)@Before在方法执行之前执行(方法上)@Around在方法执行之前与之后执行(方法上)@PointCut声明切点, 在java配置类中使用@EnableAspectJAutoProxy注解开启Spring对AspectJ代理的支持(类上)
https://www.cnblogs.com/liaojie970/p/7883687.html
5.@Bean的属性支持
Spring注解描述@Scope设置Spring容器如何新建Bean实例(方法上,得有@Bean)
其设置类型包括:Singleton (单例,一个Spring容器中只有一个bean实例,默认模式),
Protetype (每次调用新建一个bean),
Request (web项目中,给每个http request新建一个bean),
Session (web项目中,给每个http session新建一个bean),
GlobalSession(给每一个 global http session新建一个Bean实例)@StepScope在Spring Batch中还有涉及@PostConstruct由JSR-250提供,在构造函数执行完之后执行,等价于xml配置文件中bean的initMethod@PreDestory由JSR-250提供,在Bean销毁之前执行,等价于xml配置文件中bean的destroyMethod6.@Value注解
@Value 为属性注入值(属性上)
7.环境切换
Spring注解描述@Profile通过设定Environment的ActiveProfiles来设定当前context需要使用的配置环境。(类或方法上)@ConditionalSpring4中可以使用此注解定义条件话的bean,通过实现Condition接口,并重写matches方法,从而决定该bean是否被实例化。(方法上)8.异步相关
Spring注解描述@EnableAsync配置类中,通过此注解开启对异步任务的支持,叙事性AsyncConfigurer接口(类上)@Async在实际执行的bean方法使用该注解来申明其是一个异步任务(方法上或类上所有的方法都将异步,需要@EnableAsync开启异步任务)9.定时任务相关
Spring注解描述@EnableScheduling在配置类上使用,开启计划任务的支持(类上)@Scheduled来申明这是一个任务,包括cron,fixDelay,fixRate等类型(方法上,需先开启计划任务的支持)10.@Enable*注解说明
这些注解主要用来开启对xxx的支持。
Spring注解描述@EnableAspectJAutoProxy开启对AspectJ自动代理的支持@EnableAsync开启异步方法的支持@EnableScheduling开启计划任务的支持@EnableWebMvc开启Web MVC的配置支持@EnableConfigurationProperties开启对@ConfigurationProperties注解配置Bean的支持@EnableJpaRepositories开启对SpringData JPA Repository的支持@EnableTransactionManagement开启注解式事务的支持@EnableCaching开启注解式的缓存支持11.测试相关注解
Spring注解描述@RunWith运行器,Spring中通常用于对JUnit的支持@ContextConfiguration用来加载配置ApplicationContext,其中classes属性用来加载配置类
二、纯注解开发
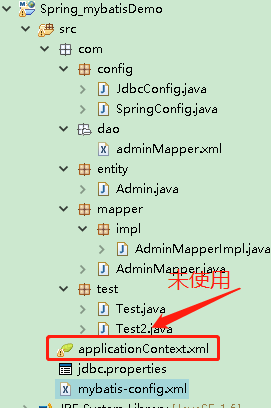
1、pom.xml
  - <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
- <modelVersion>4.0.0</modelVersion>
- <groupId>Spring_mybatisDemo</groupId>
- <artifactId>Spring_mybatisDemo</artifactId>
- <version>0.0.1-SNAPSHOT</version>
- <packaging>war</packaging>
- <name />
- <description />
- <dependencies>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-beans</artifactId>
- <version>4.1.7.RELEASE</version>
- </dependency>
-
-
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-context</artifactId>
- <version>4.1.7.RELEASE</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-jdbc</artifactId>
- <version>4.1.7.RELEASE</version>
- </dependency>
-
- <dependency>
- <groupId>org.mybatis</groupId>
- <artifactId>mybatis</artifactId>
- <version>3.2.3</version>
- </dependency>
- <dependency>
- <groupId>org.mybatis</groupId>
- <artifactId>mybatis-spring</artifactId>
- <version>1.2.0</version>
- </dependency>
-
- <dependency>
- <groupId>c3p0</groupId>
- <artifactId>c3p0</artifactId>
- <version>0.9.1.2</version>
- </dependency>
- <dependency>
- <groupId>mysql</groupId>
- <artifactId>mysql-connector-java</artifactId>
- <version>5.1.39</version>
- </dependency>
- </dependencies>
- <build>
- <sourceDirectory>${basedir}/src</sourceDirectory>
- <outputDirectory>${basedir}/WebRoot/WEB-INF/classes</outputDirectory>
- <resources>
- <resource>
- <directory>${basedir}/src</directory>
- <excludes>
- <exclude>**/*.java</exclude>
- </excludes>
- </resource>
- </resources>
- <plugins>
- <plugin>
- <artifactId>maven-war-plugin</artifactId>
- <configuration>
- <webappDirectory>${basedir}/WebRoot</webappDirectory>
- <warSourceDirectory>${basedir}/WebRoot</warSourceDirectory>
- </configuration>
- </plugin>
- <plugin>
- <artifactId>maven-compiler-plugin</artifactId>
- <configuration>
- <source>1.6</source>
- <target>1.6</target>
- </configuration>
- </plugin>
- </plugins>
- </build>
- </project>
复制代码 View Code2、配置类
SpringConfig.java- package com.config;
- import org.springframework.context.annotation.Bean;
- import org.springframework.context.annotation.ComponentScan;
- import org.springframework.context.annotation.Configuration;
- import org.springframework.context.annotation.Import;
- import com.mapper.AdminMapper;
- import com.mapper.impl.AdminMapperImpl;
- @Configuration
- @ComponentScan("com.dao")
- @Import(JdbcConfig.class)
- public class SpringConfig {
- @Bean
- public AdminMapper AdminMapper1(){
- return new AdminMapperImpl();
- }
- }
复制代码 JdbcConfig.java- package com.config;
- import java.io.IOException;
- import org.apache.ibatis.session.SqlSessionFactory;
- import org.mybatis.spring.SqlSessionFactoryBean;
- import org.mybatis.spring.SqlSessionTemplate;
- import org.springframework.beans.factory.annotation.Value;
- import org.springframework.context.annotation.Bean;
- import org.springframework.core.io.ClassPathResource;
- import org.springframework.core.io.Resource;
- import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
- import org.springframework.core.io.support.ResourcePatternResolver;
- import com.mchange.v2.c3p0.ComboPooledDataSource;
- //@Configuration
- public class JdbcConfig {
- @Value("jdbc:mysql://localhost:3306/jrbac")
- private String jdbcUrl;
- @Value("com.mysql.jdbc.Driver")
- private String driverClass;
- @Value("jrbac")
- private String user;
- @Value("123456")
- private String password;
-
-
- @Bean
- public ComboPooledDataSource dataSource() throws Exception {
- ComboPooledDataSource dataSource = new ComboPooledDataSource();
- dataSource.setJdbcUrl(jdbcUrl);
- dataSource.setDriverClass(driverClass);
- dataSource.setUser(user);
- dataSource.setPassword(password);
- return dataSource;
- }
-
- @Bean
- public SqlSessionFactoryBean sqlSessionFactory(ComboPooledDataSource dataSource) throws IOException
- {
- SqlSessionFactoryBean sqlSessionFactory = new SqlSessionFactoryBean();
- sqlSessionFactory.setDataSource(dataSource);
- sqlSessionFactory.setConfigLocation(new ClassPathResource("mybatis-config.xml"));
- ResourcePatternResolver resourceResolver = new PathMatchingResourcePatternResolver();
- Resource[] resources = resourceResolver.getResources("classpath:com/dao/*.xml");
- sqlSessionFactory.setMapperLocations(resources);
- return sqlSessionFactory;
- }
-
- @Bean
- public SqlSessionTemplate sqlSession(SqlSessionFactory sqlSessionFactory) throws Exception {
-
- return new SqlSessionTemplate(sqlSessionFactory);
- }
- }
复制代码 AdminMapperImpl.java- package com.mapper.impl;
- import java.util.List;
- import javax.annotation.Resource;
- import org.mybatis.spring.SqlSessionTemplate;
- import org.springframework.stereotype.Component;
- import com.entity.Admin;
- import com.mapper.AdminMapper;
- @Component(value = "AdminMapper1")
- public class AdminMapperImpl implements AdminMapper{
- @Resource(name = "sqlSession")
- private SqlSessionTemplate sqlSession;
-
- public void setSqlSession(SqlSessionTemplate sqlSession) {
- this.sqlSession = sqlSession;
- }
- @Override
- public List<Admin> selectAdmin() {
- AdminMapper mapper = sqlSession.getMapper(AdminMapper.class);
- return mapper.selectAdmin();
- }
- }
复制代码 AdminMapper.java- package com.mapper;
- import java.util.List;
- import com.entity.Admin;
- public interface AdminMapper {
- List<Admin> selectAdmin();
- }
复制代码 adminMapper.xml- <?xml version="1.0" encoding="UTF-8" ?>
- <!DOCTYPE mapper
- PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
- "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
- <mapper namespace="com.mapper.AdminMapper">
- <select id="selectAdmin" resultType="com.entity.Admin">
- select * from j_admin
- </select>
- </mapper>
复制代码 Test2.java- package com.test;
- import java.util.List;
- import org.springframework.context.ApplicationContext;
- import org.springframework.context.annotation.AnnotationConfigApplicationContext;
- import com.config.SpringConfig;
- import com.entity.Admin;
- import com.mapper.AdminMapper;
- public class Test2 {
- public static void main(String[] args) {
- ApplicationContext context = new AnnotationConfigApplicationContext(SpringConfig.class);
- AdminMapper adminMapper = (AdminMapper) context.getBean("AdminMapper1");
- List<Admin> admin = adminMapper.selectAdmin();
- for(Admin i : admin){
- System.out.println(i);
- }
- }
- }
复制代码
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |