下载
https://www.wxwidgets.org/downloads/
下载压缩包即可
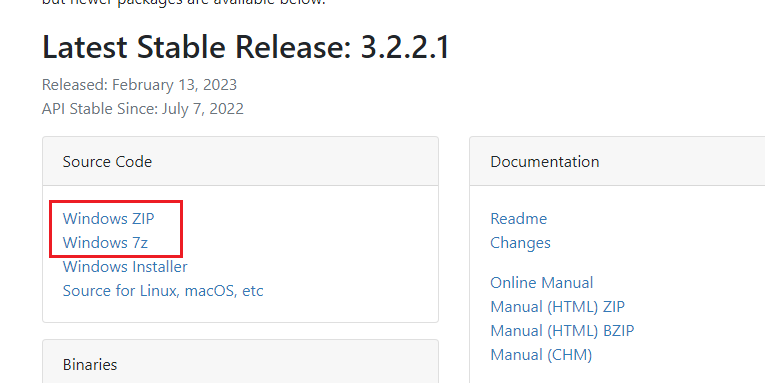
编译
打开 build\msw 目录下的 sln 文件
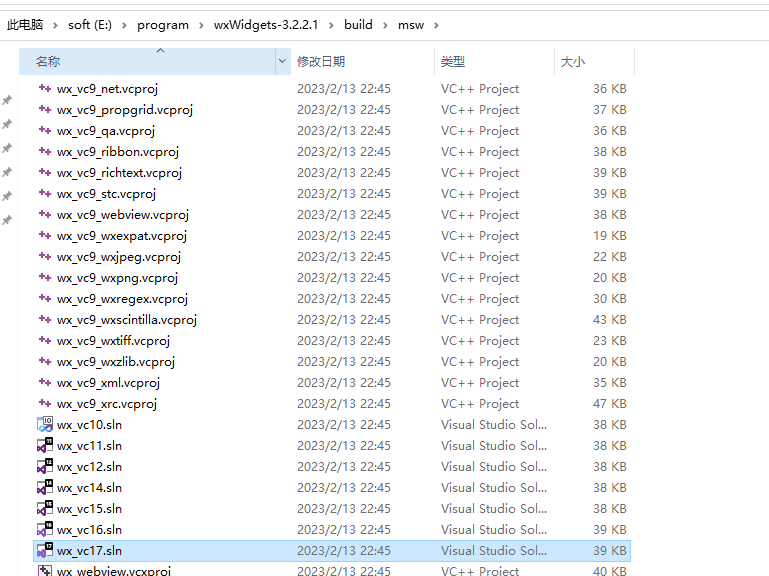
vs发布版本与vc版本对应关系: vs发布版本与vc版本对应关系
vs发布包版本vc版本Visual Studio 2003VC7Visual Studio 2005VC8Visual Studio 2008VC9Visual Studio 2010VC10Visual Studio 2012VC11Visual Studio 2013VC12Visual Studio 2015VC14Visual Studio 2017VC15Visual Studio 2019VC16Visual Studio 2022VC17生成->批生成
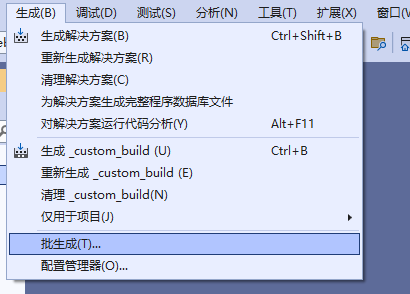
全选->重新生成
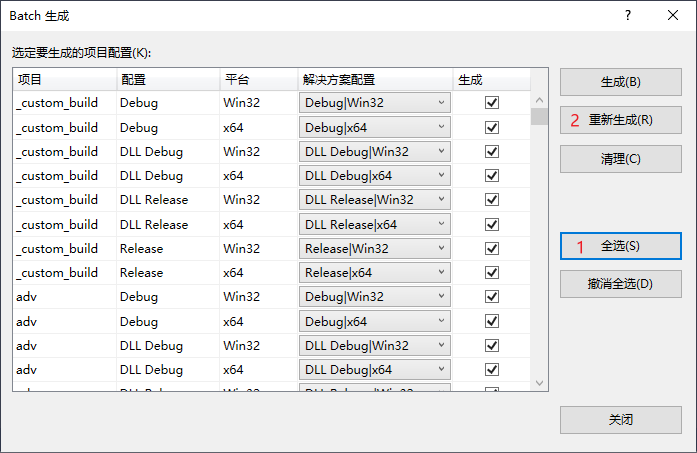
大约需要 30 分钟
新建 hello world 前的准备
新建一个目录, 将 include 目录和lib目录复制过去
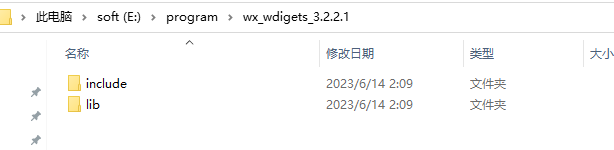
配置环境变量 wx_win, 值为include与lib所在目录: E:\program\wx_wdigets_3.2.2.1
hello world
editor config
- [*]
- guidelines = 120
- [*.{cpp,c,h}]
- charset = charset = utf-16le
- indent_style = space
- indent_size = 4
- end_of_line = lf
- trim_trailing_whitespace = true
- insert_final_newline = true
- tab_width = 4
复制代码 代码
- // wxWidgets "Hello World" Program
- // For compilers that support precompilation, includes "wx/wx.h".
- #define WXUSINGDLL
- // #define __WXMSW__
- // #define _UNICODE
- #include <wx/wxprec.h>
- #ifndef WX_PRECOMP
- #include <wx/wx.h>
- #endif
- class MyApp : public wxApp
- {
- public:
- virtual bool OnInit();
- };
- class MyFrame : public wxFrame
- {
- public:
- MyFrame();
- private:
- void OnHello(wxCommandEvent& event);
- void OnExit(wxCommandEvent& event);
- void OnAbout(wxCommandEvent& event);
- };
- enum
- {
- ID_Hello = 1
- };
- wxIMPLEMENT_APP(MyApp);
- bool MyApp::OnInit()
- {
- MyFrame* frame = new MyFrame();
- frame->Show(true);
- return true;
- }
- MyFrame::MyFrame()
- : wxFrame(NULL, wxID_ANY, "第一个窗口")
- {
- wxMenu* menuFile = new wxMenu;
- menuFile->Append(ID_Hello, "&你好...\tCtrl-H",
- "此菜单的提示文本显示在状态栏");
- menuFile->AppendSeparator();
- menuFile->Append(wxID_EXIT);
- wxMenu* menuHelp = new wxMenu;
- menuHelp->Append(wxID_ABOUT);
- wxMenuBar* menuBar = new wxMenuBar;
- menuBar->Append(menuFile, "文件(&F)");
- menuBar->Append(menuHelp, "帮助(&H)");
- SetMenuBar(menuBar);
- CreateStatusBar();
- SetStatusText("Welcome to wxWidgets!");
- Bind(wxEVT_MENU, &MyFrame::OnHello, this, ID_Hello);
- Bind(wxEVT_MENU, &MyFrame::OnAbout, this, wxID_ABOUT);
- Bind(wxEVT_MENU, &MyFrame::OnExit, this, wxID_EXIT);
- }
- void MyFrame::OnExit(wxCommandEvent& event)
- {
- Close(true);
- }
- void MyFrame::OnAbout(wxCommandEvent& event)
- {
- wxMessageBox("This is a wxWidgets Hello World example",
- "About Hello World", wxOK | wxICON_INFORMATION);
- }
- void MyFrame::OnHello(wxCommandEvent& event)
- {
- wxLogMessage("Hello world from wxWidgets!");
- }
复制代码 项目属性设置
修改输出目录与中间目录
所有配置, 所有平台
$(SolutionDir)$(ProjectName)\$(Platform)\$(Configuration)\
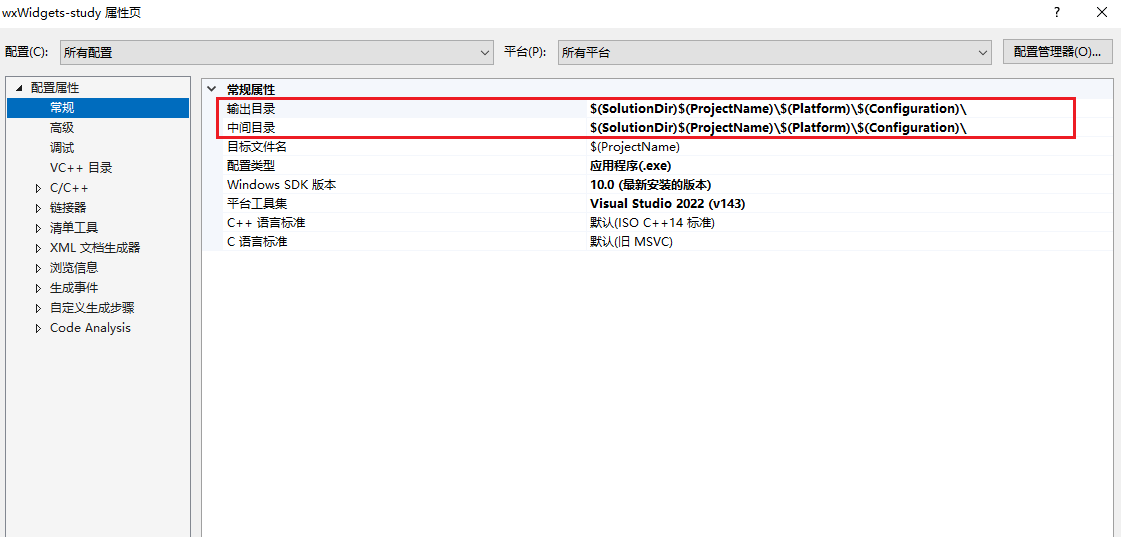
添加头文件目录
所有配置, 所有平台
- $(wx_win)\include\msvc
- $(wx_win)\include
复制代码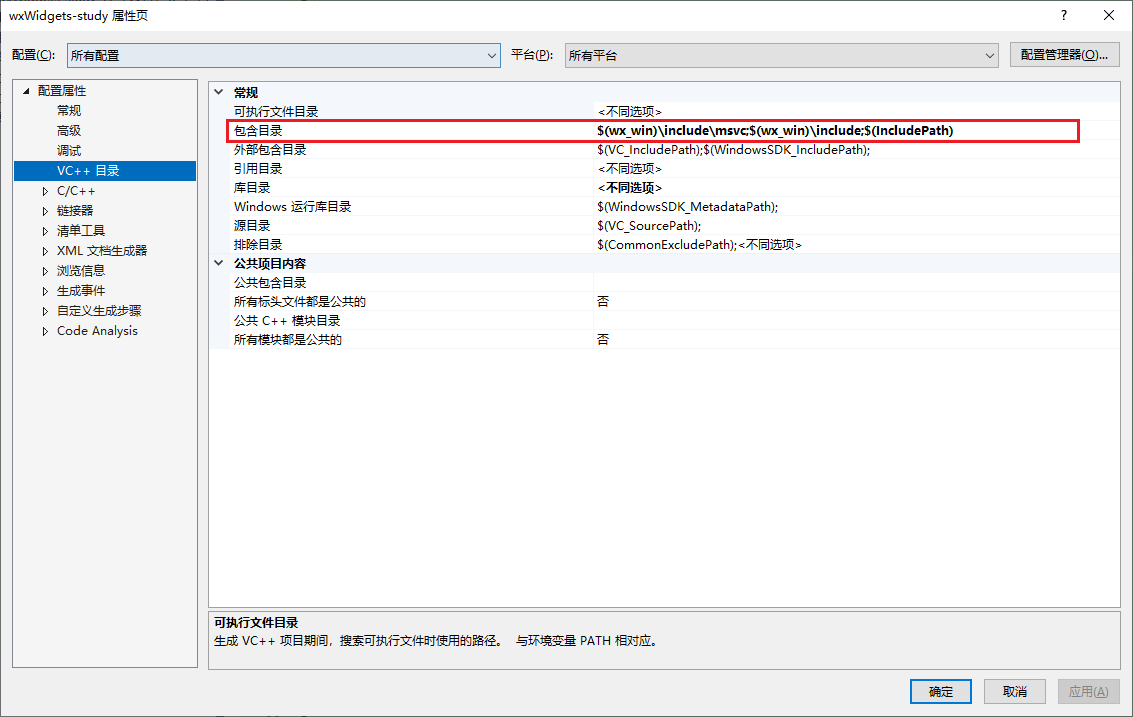
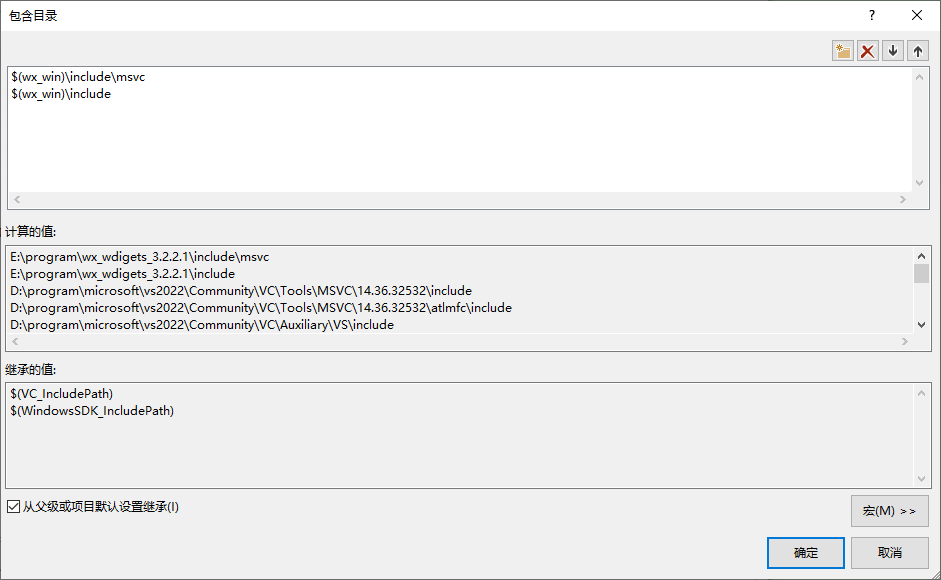
添加库目录
注意, win32与x64 不同
win32
$(wx_win)\lib\vc_dll
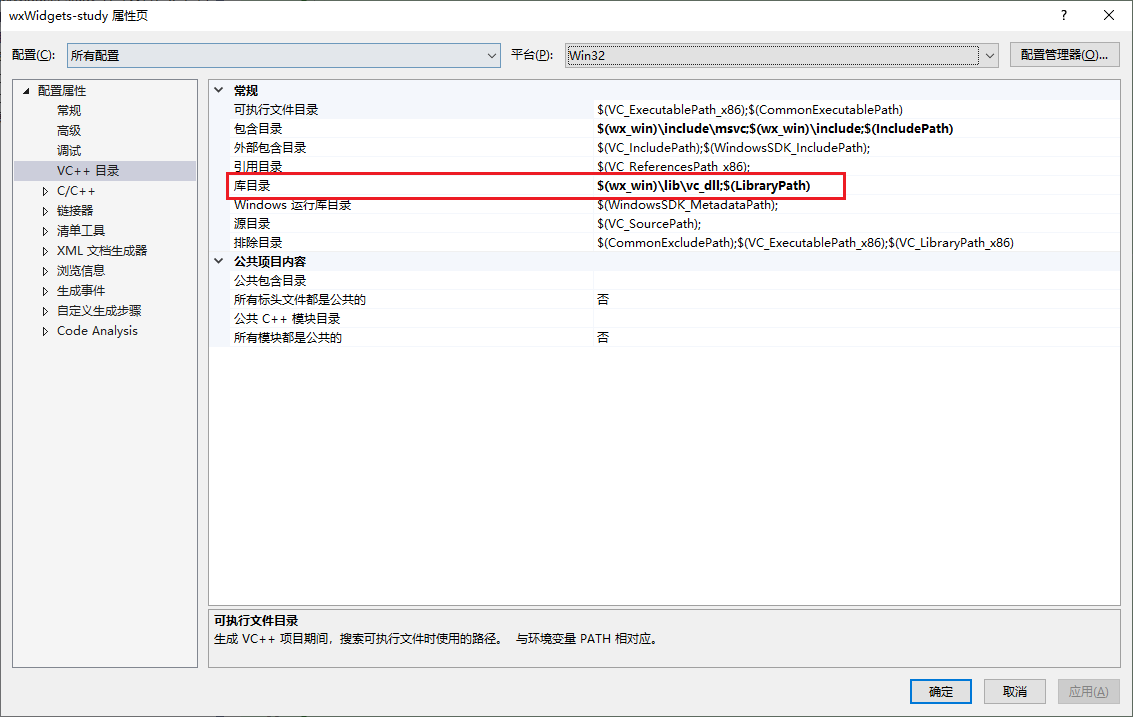
x64
$(wx_win)\lib\vc_x64_dll
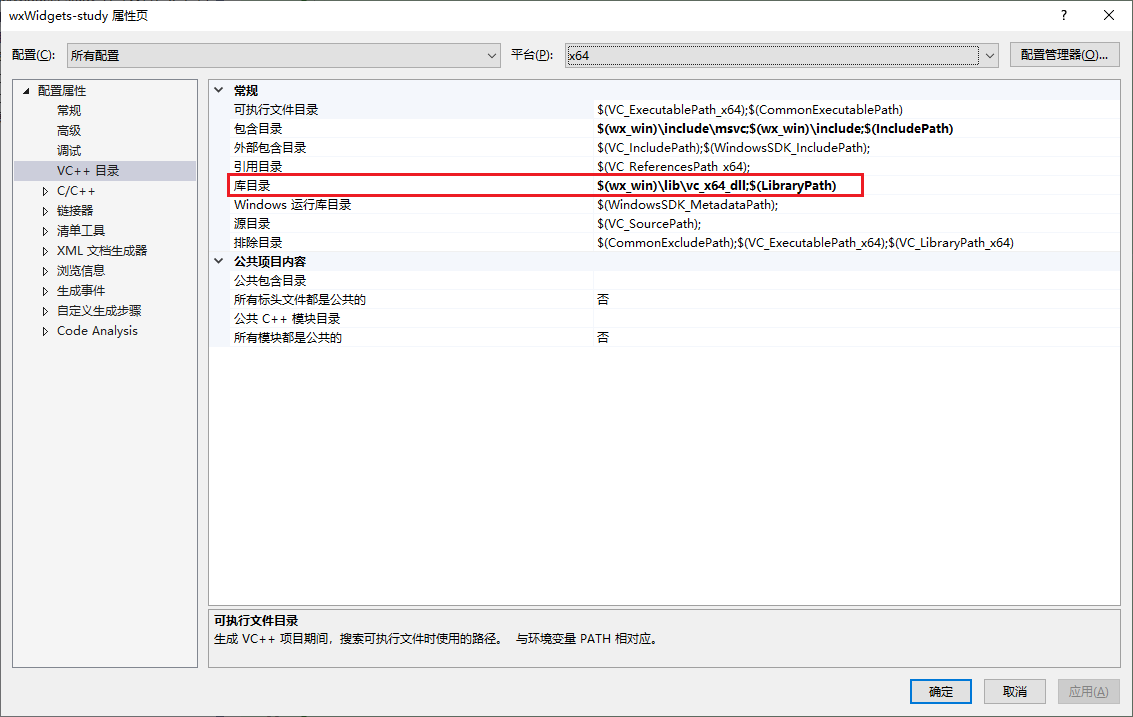
修改子系统
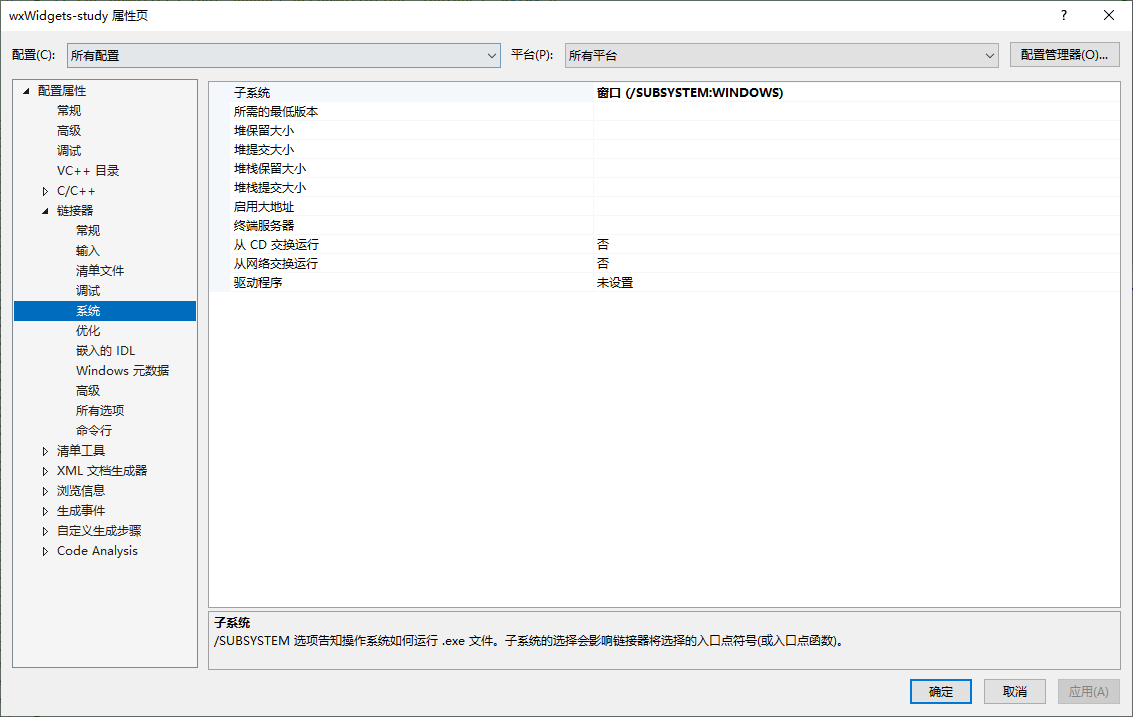
dll 文件的复制
因为使用的时动态库形式, 所以运行时需要动态库文件, 添加生成事件
所有配置, 所有平台
用于 MSBuild 命令和属性的常用宏
python $(SolutionDir)$(ProjectName)\copylib.py $(Platform) $(Configuration) $(SolutionDir)$(ProjectName)
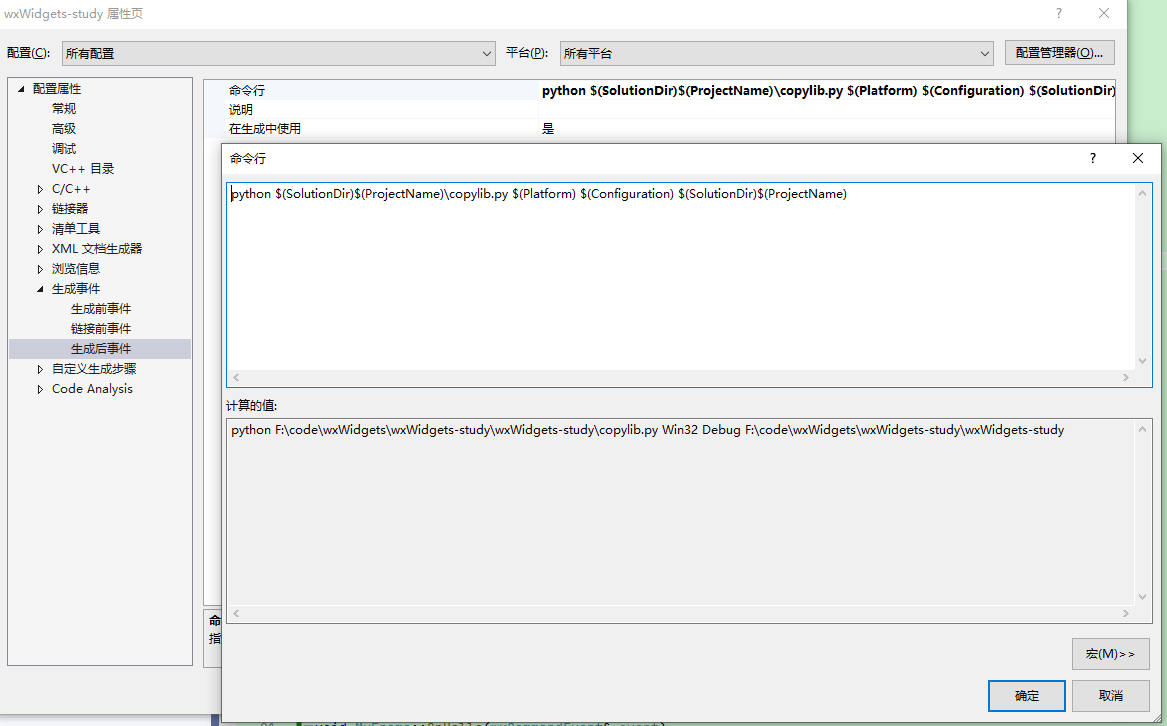
python 脚本- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- import sys
- import os
- from shutil import copyfile
- wx_env_name='wx_win'
- dll_dict={
- # 'wxbase322u':'net',
- 'wxbase322u':'',
- # 'wxbase322u':'xml',
- # 'wxmsw322u':'adv',
- # 'wxmsw322u':'aui',
- 'wxmsw322u':'core',
- # 'wxmsw322u':'gl',
- # 'wxmsw322u':'html',
- # 'wxmsw322u':'media',
- # 'wxmsw322u':'propgrid',
- # 'wxmsw322u':'qa',
- # 'wxmsw322u':'ribbon',
- # 'wxmsw322u':'richtext',
- # 'wxmsw322u':'stc',
- # 'wxmsw322u':'webview',
- # 'wxmsw322u':'xrc'
- }
- if __name__ == "__main__":
- platform = sys.argv[1]
- configuration = sys.argv[2]
- proj_dir = sys.argv[3]
- wx_path = os.getenv(wx_env_name)
- # print('wx_path:',wx_path)
- # print('platform:', platform)
- # print('configuration:',configuration)
- # print('proj_dir:',proj_dir)
- for key,value in dll_dict.items():
- dll_name = key
- if 'Debug' == configuration:
- dll_name = dll_name + 'd'
- if 0 == len(value):
- dll_name = dll_name + '_vc_'
- else:
- dll_name = dll_name + '_' + value + '_vc_'
- if 'x64' == platform:
- dll_name = dll_name + 'x64_'
- dll_name = dll_name + 'custom.dll'
- # print('dll_name:',dll_name)
-
- source_dll = wx_path + os.path.sep + 'lib'
- if 'x64' == platform:
- source_dll = source_dll + os.path.sep + 'vc_x64_dll' + os.path.sep + dll_name
- else:
- source_dll = source_dll + os.path.sep + 'vc_dll' + os.path.sep + dll_name
- # print('source_dll',source_dll)
- target_dll = proj_dir + os.path.sep + platform + os.path.sep + configuration + os.path.sep + dll_name
- # print('target_dll',target_dll)
- print(source_dll + ' => ' + target_dll)
- if not os.path.exists(target_dll):
- copyfile(source_dll, target_dll)
复制代码 测试效果
- 删除与解决方案 .sln 同级的构建目录, 比如 x64等, 保持目录清晰干净
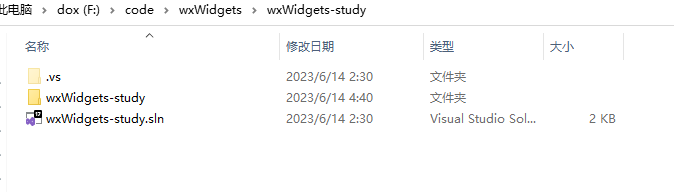
分别运行 Debug x86 , Debug x64 , Release x86 , Release x64 验证效果
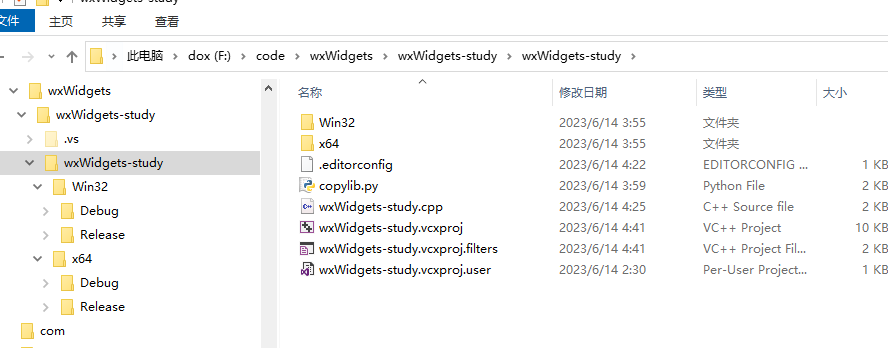
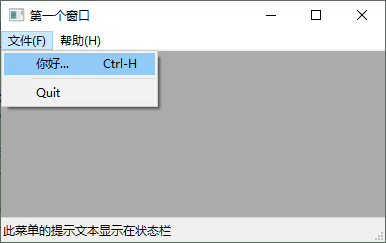
项目模板
用Visual Studio2019自定义项目模板
将 .editorconfig与copylib.py添加到项目
添加->现有项
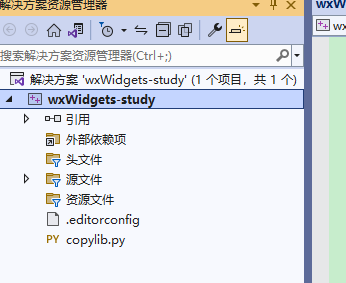
注意:
- 生成的模板文件位于: C:\Users\用户名\Documents\Visual Studio 2022\My Exported Templates
- 实际使用的模板文件在: C:\Users\用户名\Documents\Visual Studio 2022\Templates\ProjectTemplates
- 可能编辑模板之后不生效, 需要删除缓存: C:\Users\用户名\AppData\Local\Microsoft\VisualStudio\vs版本号\ComponentModelCache\Microsoft.VisualStudio.Default.cache
效果
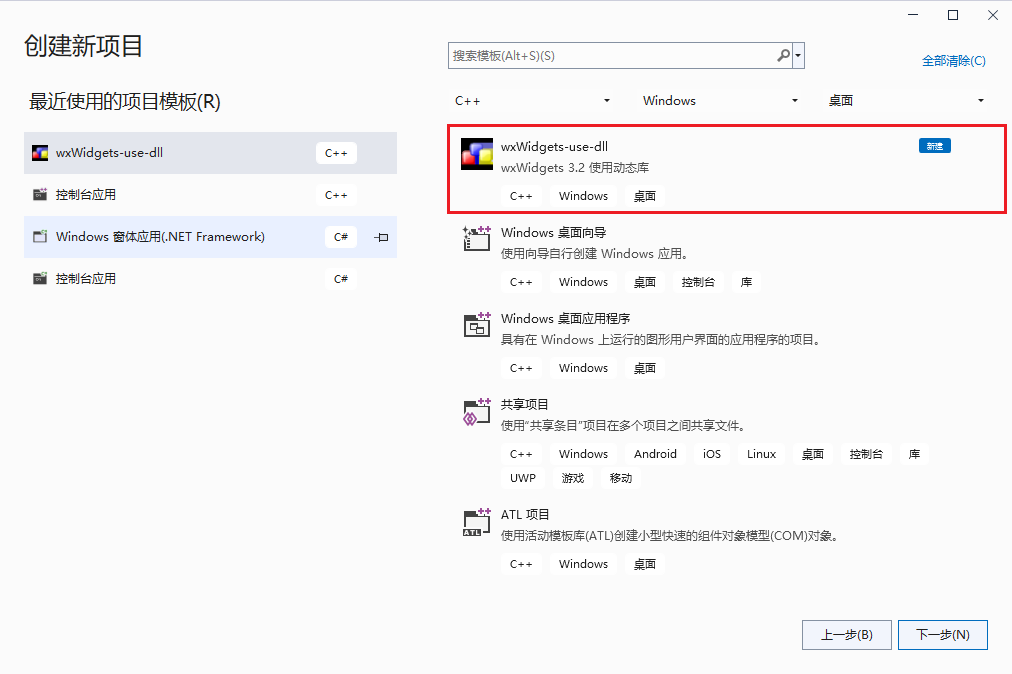
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |