Python中有多线程的支持。Python的threading模块提供了多线程编程的基本工具。在下面,我将列举一些基础的多线程用法和一些高级用法,并提供相应的源代码,其中包含中文注释。
基础用法:
创建和启动线程
- import threading
- import time
- # 定义一个简单的线程类
- class MyThread(threading.Thread):
- def run(self):
- for _ in range(5):
- print(threading.current_thread().name, "is running")
- time.sleep(1)
- # 创建两个线程实例
- thread1 = MyThread(name="Thread-1")
- thread2 = MyThread(name="Thread-2")
- # 启动线程
- thread1.start()
- thread2.start()
- # 主线程等待所有子线程结束
- thread1.join()
- thread2.join()
- print("Main thread exiting")
复制代码 线程同步 - 使用锁
- import threading
- # 共享资源
- counter = 0
- # 创建锁
- counter_lock = threading.Lock()
- # 定义一个简单的线程类
- class MyThread(threading.Thread):
- def run(self):
- global counter
- for _ in range(5):
- with counter_lock: # 使用锁保护临界区
- counter += 1
- print(threading.current_thread().name, "Counter:", counter)
- # 创建两个线程实例
- thread1 = MyThread(name="Thread-1")
- thread2 = MyThread(name="Thread-2")
- # 启动线程
- thread1.start()
- thread2.start()
- # 主线程等待所有子线程结束
- thread1.join()
- thread2.join()
- print("Main thread exiting")
复制代码 高级用法:
使用线程池
- import concurrent.futures
- import time
- # 定义一个简单的任务函数
- def task(name):
- print(f"{name} is running")
- time.sleep(2)
- return f"{name} is done"
- # 使用线程池
- with concurrent.futures.ThreadPoolExecutor(max_workers=3) as executor:
- # 提交任务给线程池
- future_to_name = {executor.submit(task, f"Thread-{i}"): f"Thread-{i}" for i in range(5)}
- # 获取任务结果
- for future in concurrent.futures.as_completed(future_to_name):
- name = future_to_name[future]
- try:
- result = future.result()
- print(f"{name}: {result}")
- except Exception as e:
- print(f"{name}: {e}")
复制代码 使用Condition进行线程间通信
- import threading
- import time
- # 共享资源
- shared_resource = None
- # 创建条件变量
- condition = threading.Condition()
- # 定义一个写线程
- class WriterThread(threading.Thread):
- def run(self):
- global shared_resource
- for _ in range(5):
- with condition:
- shared_resource = "Write data"
- print("Writer wrote:", shared_resource)
- condition.notify() # 通知等待的线程
- condition.wait() # 等待其他线程通知
- # 定义一个读线程
- class ReaderThread(threading.Thread):
- def run(self):
- global shared_resource
- for _ in range(5):
- with condition:
- while shared_resource is None:
- condition.wait() # 等待写线程通知
- print("Reader read:", shared_resource)
- shared_resource = None
- condition.notify() # 通知写线程
- # 创建写线程和读线程
- writer_thread = WriterThread()
- reader_thread = ReaderThread()
- # 启动线程
- writer_thread.start()
- reader_thread.start()
- # 主线程等待所有子线程结束
- writer_thread.join()
- reader_thread.join()
- print("Main thread exiting")
复制代码 这些例子涵盖了一些基础和高级的多线程用法。请注意,在Python中由于全局解释器锁(GIL)的存在,多线程并不能充分利用多核处理器。如果需要充分利用多核处理器,可以考虑使用multiprocessing模块进行多进程编程。
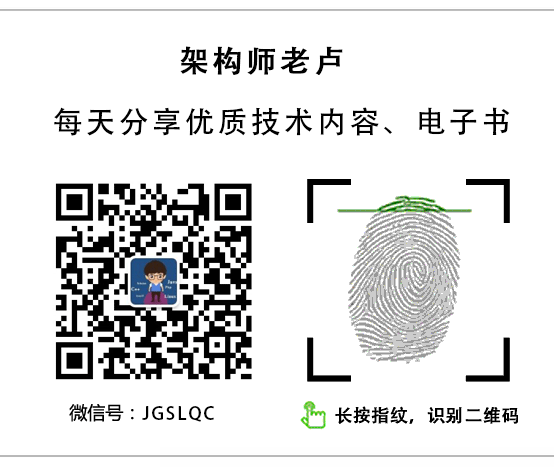
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |