1、行转列
源数据:
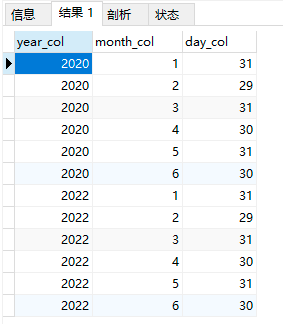
目标数据:
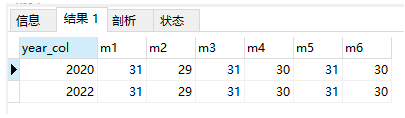
数据准备- -- 建表插入数据
- drop table if exists time_temp;
- create table if not exists time_temp(
- `year_col` int not null comment '年份',
- `month_col` int not null comment '月份',
- `day_col` int not null comment '天数'
- )engine = innodb default charset = utf8;
- insert into time_temp values
- (2020,1,31),
- (2020,2,29),
- (2020,3,31),
- (2020,4,30),
- (2020,5,31),
- (2020,6,30);
- insert into time_temp values
- (2022,1,31),
- (2022,2,29),
- (2022,3,31),
- (2022,4,30),
- (2022,5,31),
- (2022,6,30);
复制代码 1.1 方式1 分组+子查询
- -- 将数据根据年份分组,然后在进行子查询通过月份查出对应的天数;
- select t.year_col,
- (select t1.day_col from time_temp t1 where t1.month_col = 1 and t1.year_col = t.year_col) 'm1',
- (select t1.day_col from time_temp t1 where t1.month_col = 2 and t1.year_col = t.year_col) 'm2',
- (select t1.day_col from time_temp t1 where t1.month_col = 3 and t1.year_col = t.year_col) 'm3',
- (select t1.day_col from time_temp t1 where t1.month_col = 4 and t1.year_col = t.year_col) 'm4',
- (select t1.day_col from time_temp t1 where t1.month_col = 5 and t1.year_col = t.year_col) 'm5',
- (select t1.day_col from time_temp t1 where t1.month_col = 6 and t1.year_col = t.year_col) 'm6'
- from time_temp t
- group by t.year_col;
复制代码 1.2 方式2:分组+case when
- -- 先根据年份分组,在根据case when .. then ... 条件判断 输入出指定列的信息
- select t.year_col,
- min(case when t.month_col = 1 then t.day_col end) 'm1',
- min(case when t.month_col = 2 then t.day_col end) 'm2',
- min(case when t.month_col = 3 then t.day_col end) 'm3',
- min(case when t.month_col = 4 then t.day_col end) 'm4',
- min(case when t.month_col = 5 then t.day_col end) 'm5',
- min(case when t.month_col = 6 then t.day_col end) 'm6'
- from time_temp t
- group by t.year_col;
复制代码 2、删除重复数据
思路:先查询出需要保留的数据,然后删除其他的数据;- -- ====================删除重复数据=========================
- DROP TABLE IF EXISTS `results_temp`;
- CREATE TABLE `results_temp`(
- `id` int primary key auto_increment comment '主键',
- `stu_no` int NOT NULL COMMENT '学号',
- `subj_no` int NOT NULL COMMENT '课程编号',
- `exam_date` datetime NOT NULL COMMENT '考试时间',
- `stu_result` int NOT NULL COMMENT '考试成绩'
- ) ENGINE = InnoDB CHARACTER SET = utf8 COLLATE = utf8_general_ci COMMENT = '成绩表临时' ROW_FORMAT = Dynamic;
- -- 将另外一张表的数据插入到此表中(也可以用其他方式插数据,这里时为了方便) 插入两次,让数据重复
- insert into results_temp (stu_no,subj_no,exam_date,stu_result)
- select stu_no,subj_no,exam_date,stu_result
- from results
- where subj_no = 1;
- -- 查询数据,每个学生的同一门课程的成绩有两个,或者多个
- select * from results_temp
- order by stu_no desc;
复制代码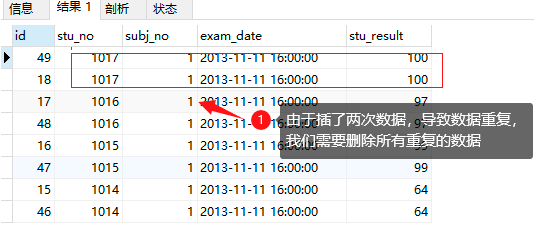
解决方法:筛选出我们需要的数据,其他数据删除;- -- 剔除重复的学生成绩,只保留一份
- -- 我们要保留的数据
- select min(id) from results_temp
- group by stu_no;
- delete from results_temp
- where id not in( -- 除了我们要保留的数据其他数据都删除
- select * from(
- select min(id) from results_temp -- 我们要保留的数据
- group by stu_no
- ) rt
- );
复制代码 再次执行SQL重复数据删除成功- select * from results_temp
- order by stu_no desc;
复制代码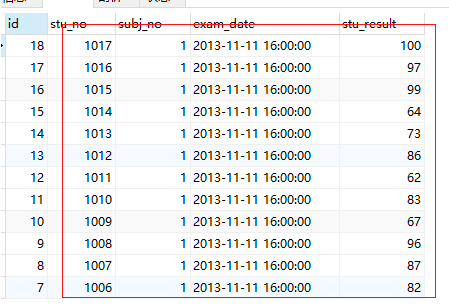
3、如果一张表,没有id自增主键,实现自定义一个序号
实现思路:通过定义一个变量,查询到一行数据就对变量 +1;
使用@关键字创建“用户变量”;
mysql中变量不用事前申明,在用的时候直接用“@变量名”。
第一种用法:set @num=1; 或set @num:=1;
第二种用法:select @num:=1; 也可以把字段的值赋值给变量 select @num:=字段名 from 表名 where ……
注意上面两种赋值符号,使用set时可以用 = 或 := ,但是使用select时必须用 :=
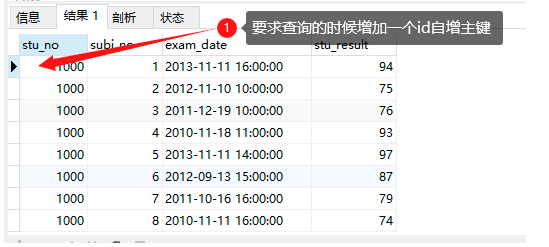
SQL实现- select @rownum:=@rownum + 1 'id',stu_no,stu_result -- @rownum:=@rownum + 1 每查询出一条数据就对变量 @rownum 加一
- from results,
- (select @rownum:= 0) rowss -- 声明:前面要使用 @rownum 要在这里(form后面)先声明并赋值为0 @rownum:= 0 ,前面才可以使用
- where subj_no = 2
- order by stu_no desc;
复制代码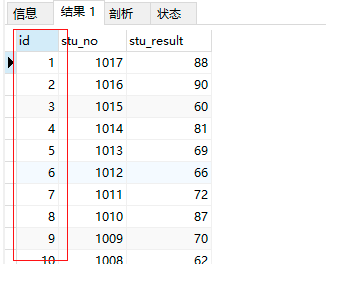
4、约束
4.1 主键约束 primary key
4.1.1 创建表和约束
- -- 主键约束
- create table employees_temp1(
- emp_id int primary key,
- emp_name varchar(50)
- )engine = innodb charset = utf8;
复制代码 4.1.2 主键约束特点1:非空
- insert into employees_temp1 values (null,'张三'); -- 添加一条数据,主键为空
复制代码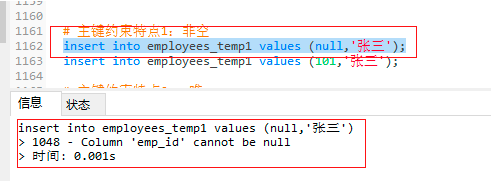
4.1.3 主键约束特点2: 唯一
- insert into employees_temp1 values (101,'张三');
- insert into employees_temp1 values (101,'张三'); -- 插入两个相同的数据
复制代码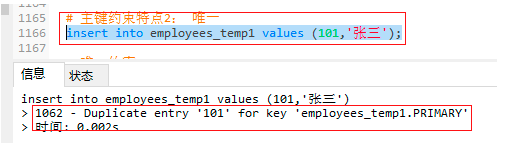
4.2 唯一约束 unique
4.2.1 创建表和唯一约束
- -- 唯一约束,
- create table employees_temp2(
- emp_id int primary key,
- emp_name varchar(50),
- emp_tel char(11) unique -- 使用列级别声明
- )engine = innodb charset = utf8;
复制代码 4.2.2 唯一约束特点1:没有非空约束非空
- -- 唯一约束特点1:没有非空约束非空
- insert into employees_temp2 values (101,'张三',null); -- 可以插入null值
- insert into employees_temp2 values (102,'李四',null);
复制代码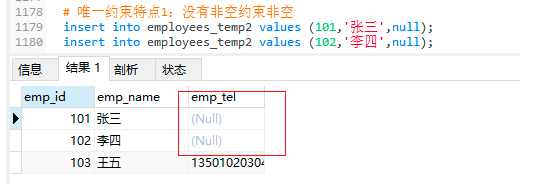
4.2.3 唯一约束特点2:可以保证值的唯一性
- -- 唯一约束特点2:可以保证值的唯一性
- insert into employees_temp2 values (103,'王五','13501020304');
- insert into employees_temp2 values (104,'刘六','13501020304'); -- 手机号不能相同
复制代码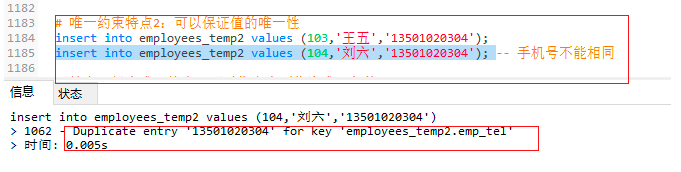
4.2.4 组合唯一约束
- -- 补充:组合唯一约束,可以指定多列作为唯一条件
- create table employees_temp3(
- emp_id int primary key,
- emp_name varchar(50),
- emp_nick char(11),
- -- 使用表级别声明,真实姓名和昵称的组合唯一
- constraint uk_emp_name_nick unique(emp_name,emp_nick)
- )engine = innodb charset = utf8;
- -- 多列唯一约束,可以保证多列值组合起来,保证值的唯一性,但是单列值,不保证唯一
- insert into employees_temp3 values (101,'王五','小五');
- insert into employees_temp3 values (102,'王五','大五');
- insert into employees_temp3 values (104,'王五','大五'); -- 不可以
- insert into employees_temp3 values (103,'王六','大五');
复制代码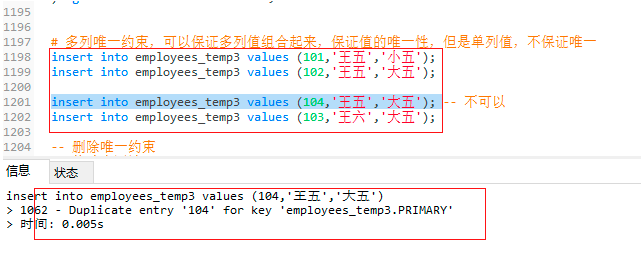
4.2.5 删除唯一约束
- -- 修改表语法
- -- alter table 表名 drop 约束名
- alter table employees_temp3 drop index uk_emp_name_nick;
- -- drop 语法
- -- drop index 约束名 on 表名
- drop index uk_emp_name_nick on employees_tem
复制代码 4.3 外键约束 delete时的级联删除和级联置空
4.3.1 级联删除 on delete cascade
- -- 级联删除
- -- 创建部门表
- drop table if exists departments_temp1;
- create table departments_temp1(
- dept_id int primary key,
- dept_name varchar(50)
- )engine = innodb charset = utf8;
- -- 插入部门数据
- insert into departments_temp1 values(100,'研发部'),(200,'市场部')
- -- 创建员工表 和外键约束
- drop table if exists employees_temp4;
- create table employees_temp4(
- emp_id int primary key,
- emp_name varchar(50),
- emp_nick char(11),
- dept_id int,
-
- -- 使用表级声明,增加部门编号的外键约束,并指定级联删除
- constraint fk_emp_dept_id foreign key (dept_id)
- references departments_temp1(dept_id)
- on delete cascade
- )engine = innodb charset = utf8;
- -- 插入员工数据
- insert into employees_temp4 values (101,'王五','小五',100);
- insert into employees_temp4 values (102,'李四','小四',200);
复制代码 查询数据:- select * from employees_temp4;
- select * from departments_temp1;
复制代码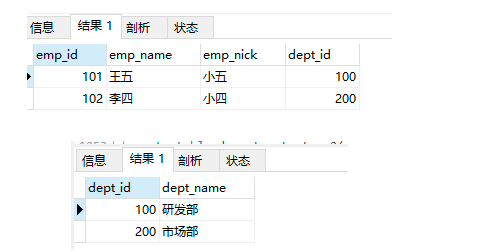 - -- 当设置外键属性为级联删除时,删除部门表中的数据,自动将所有关联表中的外键数据,一并删除delete from departments_temp1 where dept_id = 100;-- 再次查询数据:select * from employees_temp4;
- select * from departments_temp1;-- 部门删除后,该部门的数据也被删除了
复制代码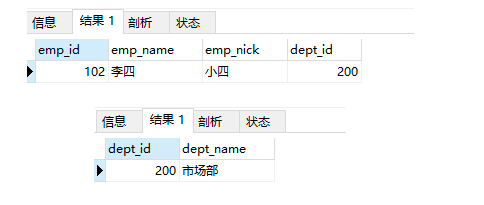
4.3.2 级联删除置空 on delete set null
- -- 级联置空
- -- 创建部门表
- drop table if exists departments_temp2;
- create table departments_temp2(
- dept_id int primary key,
- dept_name varchar(50)
- )engine = innodb charset = utf8;
- -- 插入部门数据
- insert into departments_temp2 values(100,'研发部'),(200,'市场部')
- -- 创建员工表和外键约束
- drop table if exists employees_temp5;
- create table employees_temp5(
- emp_id int primary key,
- emp_name varchar(50),
- emp_nick char(11),
- dept_id int,
-
- -- 使用表级声明,增加部门编号的外键约束,并指定级联删除
- constraint fk_null_emp_dept_id foreign key (dept_id)
- references departments_temp2(dept_id)
- on delete set null
- )engine = innodb charset = utf8;
- -- 插入员工数据
- insert into employees_temp5 values (101,'王五','小五',100);
- insert into employees_temp5 values (102,'李四','小四',200)
复制代码 查询数据:- select * from employees_temp5;
- select * from departments_temp2;
复制代码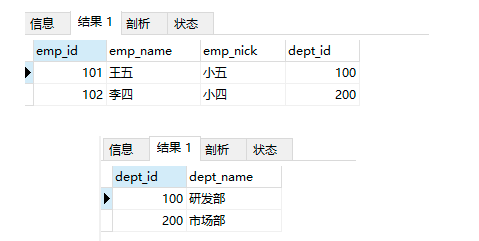 - -- 当设置外键属性为级联置空时,删除部门表中的数据,自动将所有关联表中的外键数据,一并置空delete from departments_temp2 where dept_id = 200;select * from employees_temp5;
- select * from departments_temp2;-- 部门被删除后,该部门的数据被置空
复制代码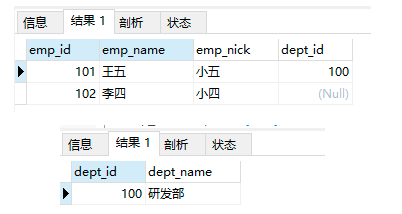
4.4 外键约束 update时的级联更新和级联置空
4.4.1 级联更新 on update cascade
- -- -- ================ update 的级联删除和级联置空==========
- drop table if exists departments_temp1_2;
- create table departments_temp1_2(
- dept_id int primary key,
- dept_name varchar(50)
- )engine = innodb charset = utf8;
- insert into departments_temp1_2 values(100,'研发部'),(200,'市场部')
- drop table if exists employees_temp4_2;
- create table employees_temp4_2(
- emp_id int primary key,
- emp_name varchar(50),
- emp_nick char(11),
- dept_id int,
-
- # 使用表级声明,真实姓名和昵称是组合唯一
- constraint uk_emp_name_nick unique(emp_name,emp_nick),
- -- 使用表级声明,增加部门编号的外键约束,并指定级联更行修改
- constraint fk_emp_dept_id_update foreign key (dept_id)
- references departments_temp1_2(dept_id)
- on update cascade -- 更新部门表中的数据,自动将所有关联表中的外键数据,一并更新
- )engine = innodb charset = utf8;
- insert into employees_temp4_2 values (101,'王五','小五',100);
- insert into employees_temp4_2 values (102,'李四','小四',200);
复制代码 查询数据:- select * from employees_temp4_2;
- select * from departments_temp1_2;
复制代码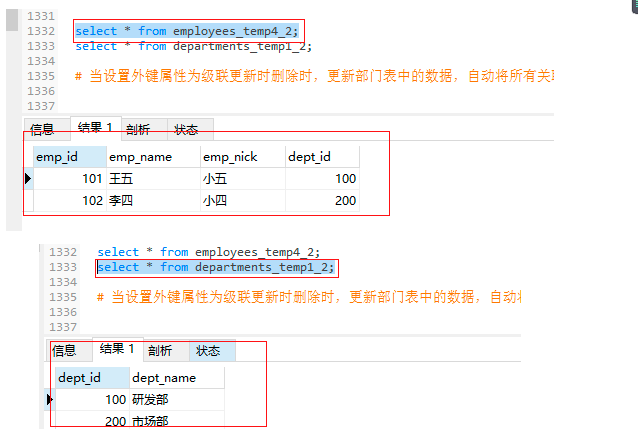
部门表数据更新:- -- 当设置外键属性为级联更新时删除时,更新部门表中的数据,自动将所有关联表中的外键数据,一并更新update departments_temp1_2 set dept_id = 111 where dept_id = 100;-- 再次查询数据select * from employees_temp4_2;
- select * from departments_temp1_2;
复制代码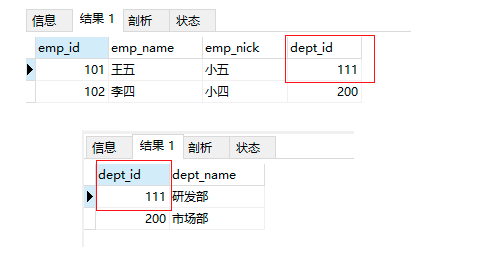
4.4.2 级联更新置空
- -- ==================update 级联更新置空========================
- drop table if exists departments_temp2_2;
- create table departments_temp2_2(
- dept_id int primary key,
- dept_name varchar(50)
- )engine = innodb charset = utf8;
- insert into departments_temp2_2 values(100,'研发部'),(200,'市场部')
- drop table if exists employees_temp5_2;
- create table employees_temp5_2(
- emp_id int primary key,
- emp_name varchar(50),
- emp_nick char(11),
- dept_id int,
-
- -- 使用表级声明,增加部门编号的外键约束,并指定级联更新置空
- constraint fk_emp_dept_id_update2 foreign key (dept_id)
- references departments_temp2_2(dept_id)
- on update set null
- )engine = innodb charset = utf8;
- select * from employees_temp5_2;
- select * from departments_temp2_2;
复制代码 查询数据:- insert into employees_temp5_2 values (101,'王五','小五',100);
- insert into employees_temp5_2 values (102,'李四','小四',200);
复制代码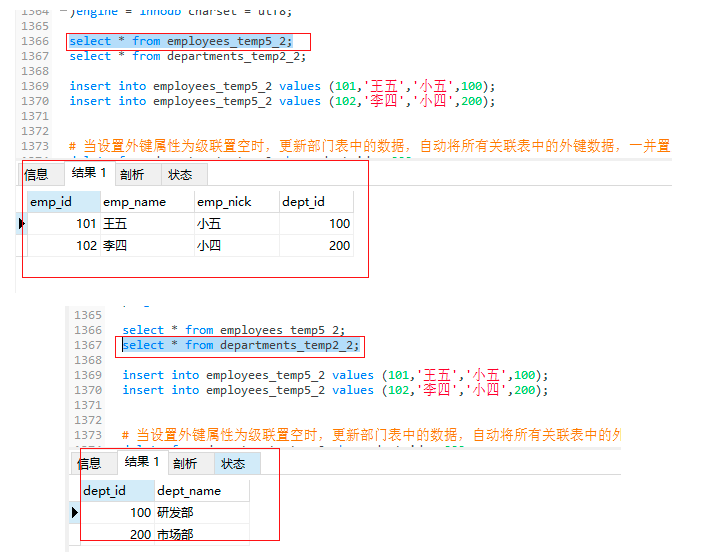
部门表数据更新- -- 当设置外键属性为级联置空时,更新部门表中的数据,自动将所有关联表中的外键数据,一并置空
- update departments_temp2_2 set dept_id = 111 where dept_id = 100;
- -- 再次查询数据
- select * from employees_temp5_2;
- select * from departments_temp2_2;
复制代码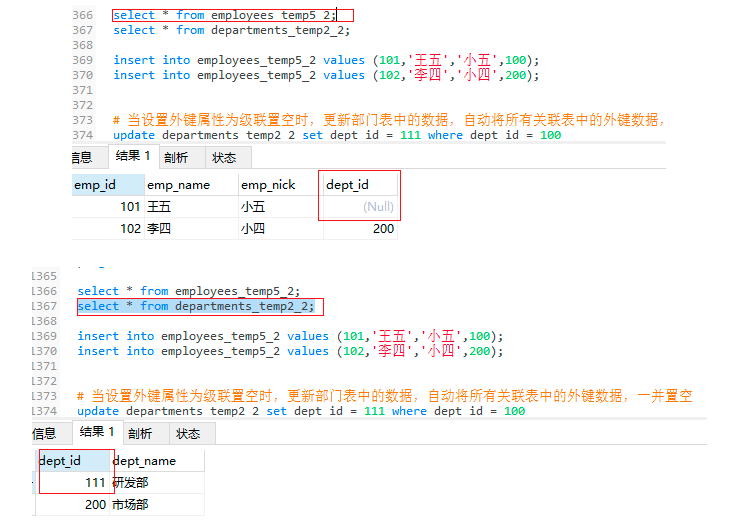
4.5 非空约束
- -- 非空约束
- drop table if exists employees_temp6;
- create table employees_temp6(
- emp_id int primary key,
- emp_name varchar(50),
- emp_nick char(11),
- dept_id int not null,
-
- -- 使用表级声明,真实姓名和昵称是组合唯一
- constraint uk_emp_name_nick unique(emp_name,emp_nick)
- )engine = innodb charset = utf8;
- -- 增加非空约束列,插入数据时,必须保证该列有效值,或者默认值,但不能为null
- insert into employees_temp6 values (101,'王五','小五',100);
- insert into employees_temp6 values (102,'李四','小四',null); -- 不能插入,因为 dept_id 设置了不能为空
复制代码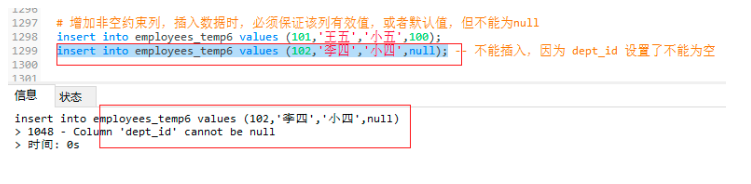
5、索引
5.1分类
- 主键索引(主键约束) primary key
- 唯一索引(唯一约束) unique
- 普通索引 index/key
- 全文索引fulltext (存储引擎必须时MyISAM)
5.2 作用
为了提高数据库的查询效率(SQL执行性能) ,底层索引算法是B+树(BTree);
5.3 建议
索引的创建和管理是数据库负责,开发人员无权干涉,原因:查询数据是否走索引,是数据库决定,底层算法觉得走索引查询效率高就走索引,如果觉得不走索引查询效率高,就不走索引,在写SQL语句时,尽量要避免索引失效(SQL调优);
5.4 注意
1.不是索引越多越好,数据库底层要管理索引,也需要耗费资源和性能(数据库性能会下降);
2.如果当前列数据重复率较高,比如性别,不建议使用索引;
3.如果当前列内容,经常改变,不建议使用索引,因为数据频繁修改要频繁的维护索引,性能会下降;
4.小数据量的表也不推荐索引,因为小表的查询效率本身就很快;
5.5 强调
一般索引都是加在where,order by 等子句经常设计的列字段,提高查询性能;
主键索引和唯一索引,对应列查询数据效率高;
5.6 建表时添加索引
- -- 普通索引的创建1,建表时添加
- drop table if exists employees_temp7;
- create table employees_temp7(
- emp_id int primary key,
- emp_name varchar(50),
- index index_emp_name (emp_name)
- )engine = innodb charset = utf8;
复制代码 5.7 建表后添加索引
- -- 普通索引的创建2,建表后添加
- drop table if exists employees_temp8;
- create table employees_temp8(
- emp_id int primary key,
- emp_name varchar(50)
- )engine = innodb charset = utf8;
- -- 使用修改表语法,添加索引
- alter table employees_temp8 add index index_emp_name_new(emp_name);
复制代码 5.8 查看表的索引
- -- 查看表的索引语法
- show index from employees_temp7;
- show index from employees_temp8;
复制代码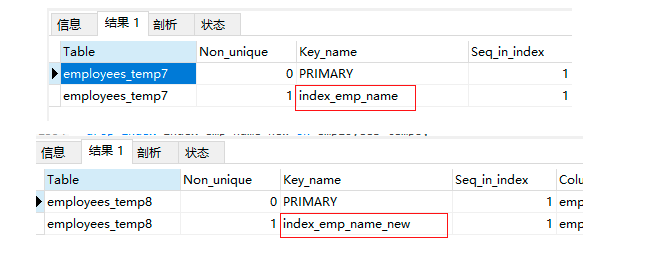
5.9 删除索引
- -- 删除索引1
- alter table employees_temp7 drop index index_emp_name;
- show index from employees_temp7;
复制代码 - -- 删除索引2
- drop index index_emp_name_new on employees_temp8;
- show index from employees_temp8;
复制代码
5.10 分析执行语句的执行性能
- -- 分析执行语句的执行性能
- -- 查看SQL语句的执行计划,通过分析执行计划结果,优化SQL语句,提示查询性能
- -- 使用 explain select 语句,可以看SQL是全表查询还是走了索引等
- -- 先把索引添加回来
- alter table employees_temp8 add index index_emp_name_new(emp_name);
- explain select * from employees_temp8;
复制代码
5.10 全文索引
- -- 全文索引
- -- 快速进行全表数据的定位,是使用与MyISAM存储引擎表,而且只适用于char,varchar,text等数据类型
- drop table if exists employees_temp9;
- create table employees_temp9(
- emp_id int primary key,
- emp_name varchar(50),
- fulltext findex_emp_name(emp_name)
- )engine = myisam charset = utf8;
复制代码 6、存储过程
6.1 带入参存储过程
- -- 作用:可以进行程序编写,实现整个业务逻辑单元的多条SQL语句的批量执行;比如:插入表10W数据
- -- 带入参的存储过程
- -- delimiter // 将MySQL结束符号更改为 // ,其他符号也可以
- delimiter //
- create procedure query_employee_by_id(in empId int)
- begin
- select * from employees_temp6 where emp_id = empId;
- end//
- -- 调用存储过程
- call query_employee_by_id(101);
复制代码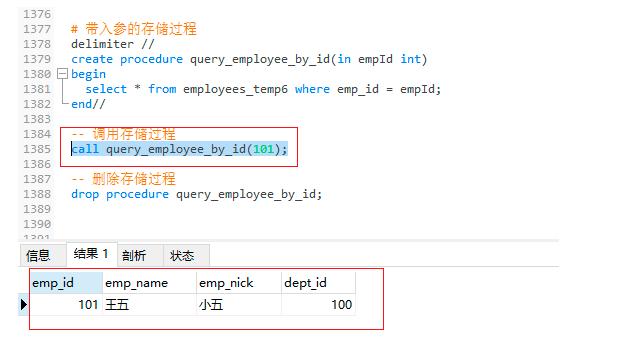 - -- 删除存储过程
- drop procedure query_employee_by_id;
复制代码 6.2 带出参存储过程
- -- 带出参的存储过程
- delimiter //
- create procedure query_employee_by_count(out empNum int)
- begin
- select count(1) into empNum from employees_temp6;
- end//
- -- 调用
- -- 定义变量,接收存储过程的结果
- set @empNum = 0;
- -- 调用出参村塾过程
- call query_employee_by_count(@empNum);
- -- 获取存储过程结果
- select @empNum from dual;
复制代码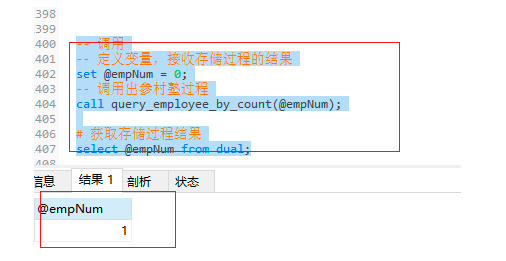
6.3 自定义存储过程
- -- 自定义存储过程,实现出入一个数值,并计算该值内的所有奇数之和,并输出结果
- delimiter //
- create procedure sum_odd(in num int)
- begin
- declare i int; -- 先定义,后赋值
- declare sums int;
- set i = 0;
- set sums = 0;
- -- declare i int default 0; -- 定义后直接赋默认值
- -- declare sums int default 0;
- while i <= num do
- if i % 2 = 1 then
- set sums = sums + i;
- end if;
- set i = i + 1;
- end while;
-
- -- 输出结果
- select sums from dual;
- end
- //
- -- 调用存储过程,查看结果
- call sum_odd(100);
- -- 恢复MySQL默认的分隔符 注意在最后一定要执行一遍整个语句
- delimiter ;
复制代码 11.1.1 备份整个数据库
- -- 简单案例,当对指定表删除数据时,自动将该条删除的数据备份
- drop table if exists employees_temp10;
- create table employees_temp10(
- emp_id int primary key,
- emp_name varchar(50),
- emp_time datetime
- )engine = innodb charset = utf8;
- insert into employees_temp10 values (101,'王五',now());
- drop table if exists employees_temp10_his;
- create table employees_temp10_his(
- emp_id int primary key,
- emp_name varchar(50),
- emp_time datetime
- )engine = innodb charset = utf8;
- -- 自定义触发器
- -- NEW 和 OLD 含义:代表触发器所在表中,当对数据操作时,触发触发器的那条数据
- -- 对于insert触发事件:NEW 表示插入后的新数据
- -- 对于update触发事件:NEW 表示修改后的数据,OLD表示被修改前的原数据
- -- 对于delete出发时间:OLD 表示被删除前的数据
- -- 语法:NEW/OLD.表中的列名
- delimiter //
- create trigger backup_employees_temp10_delete
- after delete
- on employees_temp10
- for each row
- begin
- insert into employees_temp10_his(emp_id,emp_name,emp_time)
- value (OLD.emp_id,OLD.emp_name,OLD.emp_time);
- end
- //
- delimiter ;
- -- 删除employees_temp10 中的数据
- delete from employees_temp10 where emp_id = 101;
- -- 查询employees_temp10 和历史表 employees_temp10_his
- select * from employees_temp10;
- select * from employees_temp10_his;
复制代码 11.1.2 备份整个数据库,插入数据语句前 增加列名指定 -c
- -- 快速建表,直接将查询的数据建成一张表
- -- crate table table_name (select_SQL)
- create table employees_temp11(select * from employees where department_id in(50,60));
- create table employees_temp12(select * from employees where department_id in(60,70));
- -- 两张表有重复的数据 department_id = 60
复制代码 11.1.3 备份单张表
- -- union 连接:union前的那个SQL语句,不能是分号结尾
- -- 查询结果连接,会自动去重,相同的数据只保留一份
- -- 结果51条,50号部门45条,50号部门5条,70号部门1条,执行自动去重
- select department_id,employee_id
- from employees_temp11
- union -- 查询的数据会去重
- select department_id,employee_id
- from employees_temp12;
复制代码 11.1.4 备份多张表
- -- 结果56条,50号部门45条,50号部门5条,70号部门1条,执行连接,不会自动去重,相同的数据任然会保留
- select department_id,employee_id
- from employees_temp11
- union all --查询到的数据不会去重
- select department_id,employee_id
- from employees_temp12;
复制代码 11.2.5 备份多个数据库
- -- 连接两条SQL语句,查询结果列,上下列个数要统一,否则会报错,也可以写*(表结构统一)
- select department_id,department_id,Last_name
- from employees_temp11
- union all
- select department_id,department_id,hire_date
- from employees_temp12;
- -- 别名处理
- -- 如果第一个SQL语句的结果集使用了别名处理,自动作用到连接的后面结果集,但要单独写在后面就没有效果
- select department_id,department_id '部门编号',Last_name -- 有效果
- from employees_temp11
- union all
- select department_id,department_id,hire_date
- from employees_temp12;
- select department_id,department_id,Last_name
- from employees_temp11
- union all
- select department_id,department_id '部门编号',hire_date -- 无效果
- from employees_temp12;
- -- 连接查询,默认是按照查询结构第一列升序排序,也可以自定义
- select employee_id,department_id '部门编号'
- from employees_temp11
- union all
- select employee_id,department_id
- from employees_temp12 order by employee_id desc;
复制代码 11.2.6 备份所有数据库
- -- 创建视图
- -- 普通视图和复杂视图
- -- 创建视图语法:
- -- create or replace [{undefined | merge | temptable}]
- -- view view_name [coll_list]
- -- as
- -- select_SQL
- -- 创建视图1:查询50号部门的数据
- create or replace view employee_view1
- as
- select employee_id,last_name,salary,department_id
- from employees
- where department_id = 50;
复制代码 11.2 数据恢复
数据恢复:前提,先备份数据文件;
11.2.1 source命令
- -- 查询视图
- select * from employee_view1;
复制代码 11.2.2 mysql指令
- -- 查看视图的内容结构
- desc employee_view2;
复制代码 11.2.3 多数据备份
- -- 视图中的数据,不是固定的,实际上还是查询的基础表的数据,所以基础表的数据发生改变,视图的数据也会改变
- select * from employee_view1 where last_name = 'Fripp';
- -- 修改基础表:employees,将Fripp的salary,从8200更改为9000
- update employees set salary = 9000 where last_name = 'Fripp';
- -- 视图中的数据,由于是源于基础表,跟基本表是有关系的,所以修改视图,就是修改源表
- select * from employee_view1 where last_name = 'Fripp';
- -- 修改视图 employee_view1 ,将Fripp的工资从9000更改为12000
- update employees set salary = 12000 where last_name = 'Fripp';
- -- 删除也是同理,删除视图中的数,源表中的数据也会删除
- -- 删除最低工资的Olson删除
- delete from employee_view1 where last_name = 'Olson';
- select * from employees where last_name = 'Olson';
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |