4. string类的模仿实现
首先,我们先补充一下关于编码的知识:
- int main()
- {
- char buff1[] = "abcd";
- char buff2[] = "比特";
-
- cout << sizeof(buff1) << endl;
- cout << sizeof(buff2) << endl;
- cout << buff1 << endl;
- cout << buff2 << endl;
- return 0;
- }
复制代码
假如严格按照标准的话,我们应该要实现成 basic_string ,但是这样难度太大,要思量各种编码的拷贝,以是我们就实现的轻微简单一些,不要实现成模板了。
4.1 构造 + 析构
- //string::string()
- //{
- // _str = new char[1]{ '\0' };
- // _size = 0;
- // _capacity = 0;
- //}
- string::string(const char* str)
- :_size(strlen(str))
- {
- _str = new char[_size + 1];
- _capacity = _size;
- strcpy(_str, str);
- }
- //s2(s1)
- string::string(const string& s)
- {
- _str = new char[s._capacity + 1];
- strcpy(_str, s._str);
- _size = s._size;
- _capacity = s._capacity;
- }
- string::~string()
- {
- delete[] _str;
- _str = nullptr;
- _size = _capacity = 0;
- }
复制代码 4.2 c_str
- const char* string::c_str() const
- {
- return _str;
- }
复制代码 4.3 下标遍历
- size_t string::size() const
- {
- return _size;
- }
- char& string::operator[](size_t pos)
- {
- assert(pos < _size);
- return _str[pos];
- }
复制代码- const char& string::operator[](size_t pos) const
- {
- assert(pos < _size);
- return _str[pos];
- }
复制代码 4.4 迭代器
- string::iterator string::begin()
- {
- return _str;
- }
- string::iterator string::end()
- {
- return _str + _size;
- }
复制代码
- string::const_iterator string::begin() const
- {
- return _str;
- }
- string::const_iterator string::end() const
- {
- return _str + _size;
- }
复制代码 4.5 插入
- void string::reserve(size_t n)
- {
- if (n > _capacity)
- {
- char* tmp = new char[n + 1];
- strcpy(tmp, _str);
- delete[] _str;
- _str = tmp;
- _capacity = n;
- }
- }
- void string::push_back(char ch)
- {
- if (_size == _capacity)
- {
- size_t newcapacity = 0 == _capacity ? 4 : _capacity * 2;
- reserve(newcapacity);
- }
- _str[_size] = ch;
- _str[_size + 1] = '\0';
- ++_size;
- }
- //"hello" "xxxxxxxxxxxxx"
- void string::append(const char* str)
- {
- size_t len = strlen(str);
- if (_size + len > _capacity)
- {
- reserve(_size + len);
- }
- strcpy(_str + _size, str);
- _size += len;
- }
- string& string::operator+=(char ch)
- {
- push_back(ch);
- return *this;
- }
- string& string::operator+=(const char* str)
- {
- append(str);
- return *this;
- }
复制代码 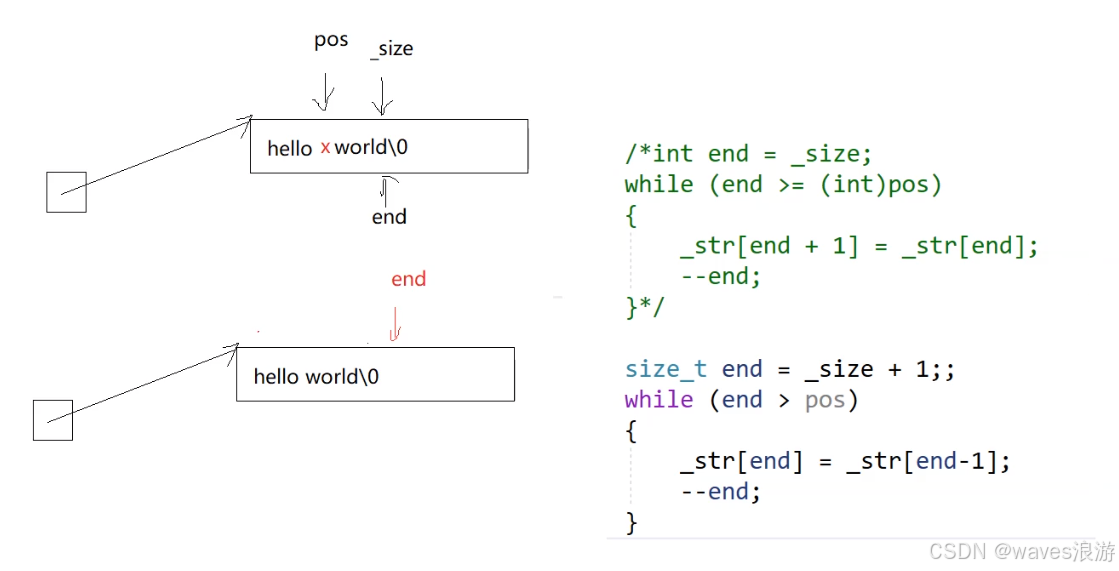
- void string::insert(size_t pos, char ch)
- {
- assert(pos <= _size);
- if (_size == _capacity)
- {
- size_t newcapacity = 0 == _capacity ? 4 : _capacity * 2;
- reserve(newcapacity);
- }
- /*int end = _size;
- while (end >= (int)pos)
- {
- _str[end + 1] = _str[end];
- --end;
- }*/
- size_t end = _size + 1;
- while (end > pos)
- {
- _str[end] = _str[end - 1];
- --end;
- }
- _str[pos] = ch;
- ++_size;
- }
复制代码
- void string::insert(size_t pos, const char* str)
- {
- assert(pos <= _size);
- size_t len = strlen(str);
- if (_size + len > _capacity)
- {
- reserve(_size + len);
- }
- /*int end = _size;
- while (end >= (int)pos)
- {
- _str[end + len] = _str[end];
- --end;
- }*/
- size_t end = _size + len;
- while (end > pos + len - 1)
- {
- _str[end] = _str[end - len];
- --end;
- }
- memcpy(_str + pos, str, len);
- _size += len;
- }
复制代码 4.6 删除
- const size_t string::npos = -1;
- void string::erase(size_t pos, size_t len)
- {
- assert(pos < _size);
- //len 大于等于后面字符个数时,有多少删多少
- if (len >= _size - pos)
- {
- _str[pos] = '\0';
- _size = pos;
- }
- else
- {
- strcpy(_str + pos, _str + pos + len);
- _size -= len;
- }
- }
复制代码 4.7 查找
- size_t string::find(char ch, size_t pos)
- {
- for (size_t i = pos; i < _size; i++)
- {
- if (_str[i] == ch)
- {
- return i;
- }
- }
- return npos;
- }
- size_t string::find(const char* str, size_t pos)
- {
- char* p = strstr(_str + pos, str);
- return p - _str;
- }
复制代码 4.8 赋值
- //s1 = s3
- //s1 = s1
- string& string::operator=(const string& s)
- {
- if (this != &s)
- {
- char* tmp = new char[s._capacity + 1];
- strcpy(tmp, s._str);
- delete[] _str;
- _str = tmp;
- _size = s._size;
- _capacity = s._capacity;
- }
- return *this;
- }
复制代码 4.9 交换
- //s1.swap(s3)
- void string::swap(string& s)
- {
- std::swap(_str, s._str);
- std::swap(_size, s._size);
- std::swap(_capacity, s._capacity);
- }
复制代码 4.10 提取子串
- string string::substr(size_t pos, size_t len)
- {
- //len大于后面剩余字符,有多少取多少
- if (len > _size - pos)
- {
- string sub(_str + pos);
- return sub;
- }
- else
- {
- string sub;
- sub.reserve(len);
- for (size_t i = 0; i < len; i++)
- {
- sub += _str[pos + i];
- }
- return sub;
- }
- }
复制代码 4.11 比力巨细
- bool string::operator<(const string& s) const
- {
- return strcmp(_str, s._str) < 0;
- }
- bool string::operator>(const string& s) const
- {
- return !(*this <= s);
- }
- bool string::operator<=(const string& s) const
- {
- return *this < s || *this == s;
- }
- bool string::operator>=(const string& s) const
- {
- return !(*this < s);
- }
- bool string::operator==(const string& s) const
- {
- return strcmp(_str, s._str) == 0;
- }
- bool string::operator!=(const string& s) const
- {
- return !(*this == s);
- }
复制代码 4.12 流插入 && 流提取
- void string::clear()
- {
- _str[0] = '\0';
- _size = 0;
- }
- //一个字符一个字符放入str里,会有很多次扩容,可以优化
- //istream& operator>> (istream& is, string& str)
- //{
- // str.clear();
- // //流提取(>>)提取不了空格和换行,istream里的函数get()可以
- // char ch = is.get();
- // while (ch != ' ' && ch != '\n')
- // {
- // str += ch;
- // ch = is.get();
- // }
- // return is;
- //}
- istream& operator>> (istream& is, string& str)
- {
- str.clear();
- char buff[128];
- int i = 0;
- char ch = is.get();
- while (ch != ' ' && ch != '\n')
- {
- buff[i++] = ch;
- // 0 - 126
- if (i == 127)
- {
- buff[i] = '\0';
- str += buff;
- i = 0;
- }
- ch = is.get();
- }
- if (i != 0)
- {
- buff[i] = '\0';
- str += buff;
- }
- return is;
- }
- ostream& operator<< (ostream& os, const string& str)
- {
- for (size_t i = 0; i < str.size(); i++)
- {
- os << str[i];
- }
- return os;
- }
复制代码 5. 现代版写法的String类
我们之前写的拷贝构造和赋值运算符重载是传统写法,其实另有现代写法:
- //现代写法(让别人干活,交换)
- //s2(s1)
- string::string(const string& s)
- {
- string tmp(s._str);
- /*std::swap(tmp._str, _str);
- std::swap(tmp._size, _size);
- std::swap(tmp._capacity, _capacity);*/
-
- //这个是我们写的string类里的交换函数
- swap(tmp);
- }
复制代码
- /*string& string::operator=(const string& s)
- {
- if (this != &s)
- {
- string tmp(s._str);
- swap(tmp);
- }
- return *this;
- }*/
- //s1 = s3
- string& string::operator=(string tmp)
- {
- swap(tmp);
- return *this;
- }
复制代码 5.1 完整代码
- //string.h
- #include <iostream>
- #include <assert.h>
- using namespace std;
- namespace bit
- {
- class string
- {
- public:
- typedef char* iterator;
- typedef const char* const_iterator;
- iterator begin();
- iterator end();
- const_iterator begin() const;
- const_iterator end() const;
- //string();
- string(const char* str = "");
- string(const string& s);
- //string& operator=(const string& s);
- string& operator=(string tmp);
- ~string();
-
- const char* c_str() const;
-
- size_t size() const;
- char& operator[](size_t pos);
- const char& operator[](size_t pos) const;
- void reserve(size_t n);
- void push_back(char ch);
- void append(const char* str);
- string& operator+=(char ch);
- string& operator+=(const char* str);
- void insert(size_t pos, char ch);
- void insert(size_t pos, const char* str);
- void erase(size_t pos = 0, size_t len = npos);
- size_t find(char ch, size_t pos = 0);
- size_t find(const char* str, size_t pos = 0);
- void swap(string& s);
- string substr(size_t pos = 0, size_t len = npos);
- bool operator<(const string& s) const;
- bool operator>(const string& s) const;
- bool operator<=(const string& s) const;
- bool operator>=(const string& s) const;
- bool operator==(const string& s) const;
- bool operator!=(const string& s) const;
- void clear();
- private:
- //char _buff[16];
- char* _str = nullptr;
- size_t _size = 0;
- size_t _capacity = 0;
- const static size_t npos;
-
- //特例,const静态成员变量只有整型可以这样声明定义(了解即可,不建议这样写)
- //const static size_t npos = -1;
-
- //不支持
- //const static double N = 2.2;
- };
- istream& operator>> (istream& is, string& str);
- ostream& operator<< (ostream& os, const string& str);
- }
复制代码- //string.cpp
- #include "string.h"
- namespace bit
- {
- const size_t string::npos = -1;
- //string::string()
- //{
- // _str = new char[1]{ '\0' };
- // _size = 0;
- // _capacity = 0;
- //}
- string::iterator string::begin()
- {
- return _str;
- }
- string::iterator string::end()
- {
- return _str + _size;
- }
- string::const_iterator string::begin() const
- {
- return _str;
- }
- string::const_iterator string::end() const
- {
- return _str + _size;
- }
- string::string(const char* str)
- :_size(strlen(str))
- {
- _str = new char[_size + 1];
- _capacity = _size;
- strcpy(_str, str);
- }
- //传统写法(实在人)
- //s2(s1)
- /*string::string(const string& s)
- {
- _str = new char[s._capacity + 1];
- strcpy(_str, s._str);
- _size = s._size;
- _capacity = s._capacity;
- }*/
- //现代写法(让别人干活,交换)
- //s2(s1)
- string::string(const string& s)
- {
- string tmp(s._str);
- /*std::swap(tmp._str, _str);
- std::swap(tmp._size, _size);
- std::swap(tmp._capacity, _capacity);*/
-
- //这个是我们写的string类里的交换函数
- swap(tmp);
- }
- //s1 = s3
- //s1 = s1
- /*string& string::operator=(const string& s)
- {
- if (this != &s)
- {
- char* tmp = new char[s._capacity + 1];
- strcpy(tmp, s._str);
- delete[] _str;
- _str = tmp;
- _size = s._size;
- _capacity = s._capacity;
- }
- return *this;
- }*/
- /*string& string::operator=(const string& s)
- {
- if (this != &s)
- {
- string tmp(s._str);
- swap(tmp);
- }
- return *this;
- }*/
-
- //s1 = s3
- string& string::operator=(string tmp)
- {
- swap(tmp);
- return *this;
- }
- string::~string()
- {
- delete[] _str;
- _str = nullptr;
- _size = _capacity = 0;
- }
- const char* string::c_str() const
- {
- return _str;
- }
- size_t string::size() const
- {
- return _size;
- }
- char& string::operator[](size_t pos)
- {
- assert(pos < _size);
- return _str[pos];
- }
- const char& string::operator[](size_t pos) const
- {
- assert(pos < _size);
- return _str[pos];
- }
- void string::reserve(size_t n)
- {
- if (n > _capacity)
- {
- char* tmp = new char[n + 1];
- strcpy(tmp, _str);
- delete[] _str;
- _str = tmp;
- _capacity = n;
- }
- }
- void string::push_back(char ch)
- {
- /*if (_size == _capacity)
- {
- size_t newcapacity = 0 == _capacity ? 4 : _capacity * 2;
- reserve(newcapacity);
- }
- _str[_size] = ch;
- _str[_size + 1] = '\0';
- ++_size;*/
- insert(_size, ch);
- }
- //"hello" "xxxxxxxxxxxxx"
- void string::append(const char* str)
- {
- /*size_t len = strlen(str);
- if (_size + len > _capacity)
- {
- reserve(_size + len);
- }
- strcpy(_str + _size, str);
- _size += len;*/
- insert(_size, str);
- }
- string& string::operator+=(char ch)
- {
- push_back(ch);
- return *this;
- }
- string& string::operator+=(const char* str)
- {
- append(str);
- return *this;
- }
- void string::insert(size_t pos, char ch)
- {
- assert(pos <= _size);
- if (_size == _capacity)
- {
- size_t newcapacity = 0 == _capacity ? 4 : _capacity * 2;
- reserve(newcapacity);
- }
- /*int end = _size;
- while (end >= (int)pos)
- {
- _str[end + 1] = _str[end];
- --end;
- }*/
- size_t end = _size + 1;
- while (end > pos)
- {
- _str[end] = _str[end - 1];
- --end;
- }
- _str[pos] = ch;
- ++_size;
- }
- void string::insert(size_t pos, const char* str)
- {
- assert(pos <= _size);
- size_t len = strlen(str);
- if (_size + len > _capacity)
- {
- reserve(_size + len);
- }
- /*int end = _size;
- while (end >= (int)pos)
- {
- _str[end + len] = _str[end];
- --end;
- }*/
- size_t end = _size + len;
- while (end > pos + len - 1)
- {
- _str[end] = _str[end - len];
- --end;
- }
- memcpy(_str + pos, str, len);
- _size += len;
- }
- void string::erase(size_t pos, size_t len)
- {
- assert(pos < _size);
- //len 大于等于后面字符个数时,有多少删多少
- if (len >= _size - pos)
- {
- _str[pos] = '\0';
- _size = pos;
- }
- else
- {
- strcpy(_str + pos, _str + pos + len);
- _size -= len;
- }
- }
- size_t string::find(char ch, size_t pos)
- {
- for (size_t i = pos; i < _size; i++)
- {
- if (_str[i] == ch)
- {
- return i;
- }
- }
- return npos;
- }
- size_t string::find(const char* str, size_t pos)
- {
- char* p = strstr(_str + pos, str);
- return p - _str;
- }
- //s1.swap(s3)
- void string::swap(string& s)
- {
- std::swap(_str, s._str);
- std::swap(_size, s._size);
- std::swap(_capacity, s._capacity);
- }
- string string::substr(size_t pos, size_t len)
- {
- //len大于后面剩余字符,有多少取多少
- if (len > _size - pos)
- {
- string sub(_str + pos);
- return sub;
- }
- else
- {
- string sub;
- sub.reserve(len);
- for (size_t i = 0; i < len; i++)
- {
- sub += _str[pos + i];
- }
- return sub;
- }
- }
- bool string::operator<(const string& s) const
- {
- return strcmp(_str, s._str) < 0;
- }
- bool string::operator>(const string& s) const
- {
- return !(*this <= s);
- }
- bool string::operator<=(const string& s) const
- {
- return *this < s || *this == s;
- }
- bool string::operator>=(const string& s) const
- {
- return !(*this < s);
- }
-
- bool string::operator==(const string& s) const
- {
- return strcmp(_str, s._str) == 0;
- }
- bool string::operator!=(const string& s) const
- {
- return !(*this == s);
- }
- void string::clear()
- {
- _str[0] = '\0';
- _size = 0;
- }
- //一个字符一个字符放入str里,会有很多次扩容,可以优化
- //istream& operator>> (istream& is, string& str)
- //{
- // str.clear();
- // //流提取(>>)提取不了空格和换行,istream里的函数get()可以
- // char ch = is.get();
- // while (ch != ' ' && ch != '\n')
- // {
- // str += ch;
- // ch = is.get();
- // }
- // return is;
- //}
- istream& operator>> (istream& is, string& str)
- {
- str.clear();
- char buff[128];
- int i = 0;
- char ch = is.get();
- while (ch != ' ' && ch != '\n')
- {
- buff[i++] = ch;
- // 0 - 126
- if (i == 127)
- {
- buff[i] = '\0';
- str += buff;
- i = 0;
- }
- ch = is.get();
- }
- if (i != 0)
- {
- buff[i] = '\0';
- str += buff;
- }
- return is;
- }
- ostream& operator<< (ostream& os, const string& str)
- {
- for (size_t i = 0; i < str.size(); i++)
- {
- os << str[i];
- }
- return os;
- }
- }
复制代码- //Test.cpp
- #include "string.h"
- namespace bit
- {
- void test_string1()
- {
- string s1("hello world");
- cout << s1.c_str() << endl;
- for (size_t i = 0; i < s1.size(); i++)
- {
- s1[i]++;
- }
- for (size_t i = 0; i < s1.size(); i++)
- {
- cout << s1[i] << " ";
- }
- cout << endl;
- //封装:统一屏蔽了底层实现细节,提供了一种简单通用的访问容器的方式
- string::iterator it1 = s1.begin();
- while (it1 != s1.end())
- {
- cout << *it1 << " ";
- ++it1;
- }
- cout << endl;
- for (auto e : s1)
- {
- cout << e << " ";
- }
- cout << endl;
- string s2;
- cout << s2.c_str() << endl;
- const string s3("xxxxxxx");
- string::const_iterator it3 = s3.begin();
- while (it3 != s3.end())
- {
- //*it3 = 'y';//err
- cout << *it3 << " ";
- ++it3;
- }
- cout << endl;
- for (size_t i = 0; i < s3.size(); i++)
- {
- //s3[i]++;
- cout << s3[i] << " ";
- }
- cout << endl;
- }
- void test_string2()
- {
- string s1("hello world");
- cout << s1.c_str() << endl;
-
- s1.push_back('x');
- cout << s1.c_str() << endl;
-
- s1.append("yyyyy");
- cout << s1.c_str() << endl;
-
- s1 += 'z';
- s1 += "mmmmmm";
- cout << s1.c_str() << endl;
- }
- void test_string3()
- {
- string s1("hello world");
- cout << s1.c_str() << endl;
- s1.insert(6, 'x');
- cout << s1.c_str() << endl;
- s1.insert(0, 'x');
- cout << s1.c_str() << endl;
-
- string s2("hello world");
- cout << s2.c_str() << endl;
- s2.insert(6, "yyy");
- cout << s2.c_str() << endl;
- s2.insert(0, "yyy");
- cout << s2.c_str() << endl;
- string s3("hello world");
- cout << s3.c_str() << endl;
- //s3.erase(6, 10);
- s3.erase(6);
- cout << s3.c_str() << endl;
- string s4("hello world");
- cout << s4.c_str() << endl;
- s4.erase(6, 3);
- cout << s4.c_str() << endl;
- }
- void test_string4()
- {
- string s1("hello world");
- cout << s1.find('o') << endl;
- cout << s1.find("wor") << endl;
- }
- void test_string5()
- {
- string s1("hello world");
- string s2(s1);
- s1[0] = 'x';
- cout << s1.c_str() << endl;
- cout << s2.c_str() << endl;
-
- string s3("yyyy");
- s1 = s3;
- cout << s1.c_str() << endl;
- cout << s3.c_str() << endl;
-
- string s4("zzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz");
- s1 = s4;
- cout << s1.c_str() << endl;
- cout << s4.c_str() << endl;
-
- s1 = s1;
- cout << s1.c_str() << endl;
- cout << s3.c_str() << endl;
-
- std::swap(s1, s3);
- cout << s1.c_str() << endl;
- cout << s3.c_str() << endl;
- s1.swap(s3);
- cout << s1.c_str() << endl;
- cout << s3.c_str() << endl;
- }
- void test_string6()
- {
- string url("https://gitee.com/ailiangshilove/cpp-class/blob/master/%E8%AF%BE%E4%BB%B6%E4%BB%A3%E7%A0%81/C++%E8%AF%BE%E4%BB%B6V6/string%E7%9A%84%E6%8E%A5%E5%8F%A3%E6%B5%8B%E8%AF%95%E5%8F%8A%E4%BD%BF%E7%94%A8/TestString.cpp");
- size_t pos1 = url.find(':');
- string url1 = url.substr(0, pos1 - 0);
- cout << url1.c_str() << endl;
- size_t pos2 = url.find('/', pos1 + 3);
- string url2 = url.substr(pos1 + 3, pos2 - (pos1 + 3));
- cout << url2.c_str() << endl;
- string url3 = url.substr(pos2 + 1);
- cout << url3.c_str() << endl;
- }
- void test_string7()
- {
- //string s1("hello world");
- //cout << s1 << endl;
-
- string s1;
- cin >> s1;
- cout << s1 << endl;
- }
- void test_string8()
- {
- string s1("hello world");
- string s2(s1);
- cout << s1 << endl;
- cout << s2 << endl;
- string s3("xxxxxxxxxxxxxxxxxxxxxxxxx");
- s1 = s3;
- cout << s1 << endl;
- cout << s3 << endl;
- }
- }
- int main()
- {
- bit::test_string8();
- return 0;
- }
复制代码 6. 写时拷贝(了解)
写时拷贝就是一种拖延症,是在浅拷贝的基础之上增长了引用计数的方式来实现的。
引用计数:用来记载资源使用者的个数。在构造时,将资源的计数给成1,每增长一个对象使用该资源,就给计数增长1,当某个对象被销毁时,先给该计数减1,然后再检查是否需要开释资源,假如计数为1,阐明该对象时资源的末了一个使用者,将该资源开释;否则就不能开释,因为另有其他对象在使用该资源。
举个例子:
- void copy_on_write()
- {
- //如果计数不等于1,再去做深拷贝
- }
- void string::push_back(char ch)
- {
- //写时拷贝
- copy_on_write();
- /*if (_size == _capacity)
- {
- size_t newcapacity = 0 == _capacity ? 4 : _capacity * 2;
- reserve(newcapacity);
- }
- _str[_size] = ch;
- _str[_size + 1] = '\0';
- ++_size;*/
- insert(_size, ch);
- }
复制代码 可以用下面的代码来看是否是用了写时拷贝:
- void test_string9()
- {
- std::string s1("hello world");
- std::string s2(s1);
- cout << (void*)s1.c_str() << endl;
- cout << (void*)s2.c_str() << endl;
- }
复制代码 Windows的VS下没有用写时拷贝,而Linux的g++就使用了写时拷贝。
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |