本文章介绍一个基于java的简单酒店数据管理系统
项目介绍
该项目适用于初学java后,需要一个小练手的java web项目,该项目是只有一个酒店数据表,然后实现对该酒店增加,修改,删除和分页查询的小案例,虽然项目不是很复杂,但麻雀虽小但五脏俱全,适合于个人学习适用。
项目使用的技术架构
后端:java+SpringBoot + MyBatis-Plus
数据库:MySQL
前端:Vue + Element-ui + Axios
开发工具:idea或eclipse
更多项目请查看:项目帮
项目实现
- HotelController 定义了对酒店信息的CRUD的接口:
- @RestController
- @RequestMapping("hotel")
- public class HotelController {
- @Autowired
- private IHotelService hotelService;
- /**
- * 通过id来查询酒店数据
- * @param id 酒店id号
- * @return
- */
- @GetMapping("/{id}")
- public Hotel queryById(@PathVariable("id") Long id){
- return hotelService.getById(id);
- }
- /**
- * 分页查询数据列表出来
- * @param page 页码
- * @param size 每页的大小
- * @return
- */
- @GetMapping("/list")
- public PageResult hotelList(
- @RequestParam(value = "page", defaultValue = "1") Integer page,
- @RequestParam(value = "size", defaultValue = "1") Integer size
- ){
- Page<Hotel> result = hotelService.page(new Page<>(page, size));
- return new PageResult(result.getTotal(), result.getRecords());
- }
- /**
- * 保存酒店信息
- * @param hotel 酒店信息实体类
- */
- @PostMapping
- public void saveHotel(@RequestBody Hotel hotel){
- hotelService.save(hotel);
- }
- /**
- * 更新酒店信息
- * @param hotel 酒店信息实体类
- */
- @PutMapping()
- public void updateById(@RequestBody Hotel hotel){
- if (hotel.getId() == null) {
- throw new InvalidParameterException("id不能为空");
- }
- hotelService.updateById(hotel);
- }
- /**
- * 通过酒店id删除酒店信息
- * @param id 酒店id号
- */
- @DeleteMapping("/{id}")
- public void deleteById(@PathVariable("id") Long id) {
- hotelService.removeById(id);
- }
- }
复制代码 项目实现的效果
- 首页
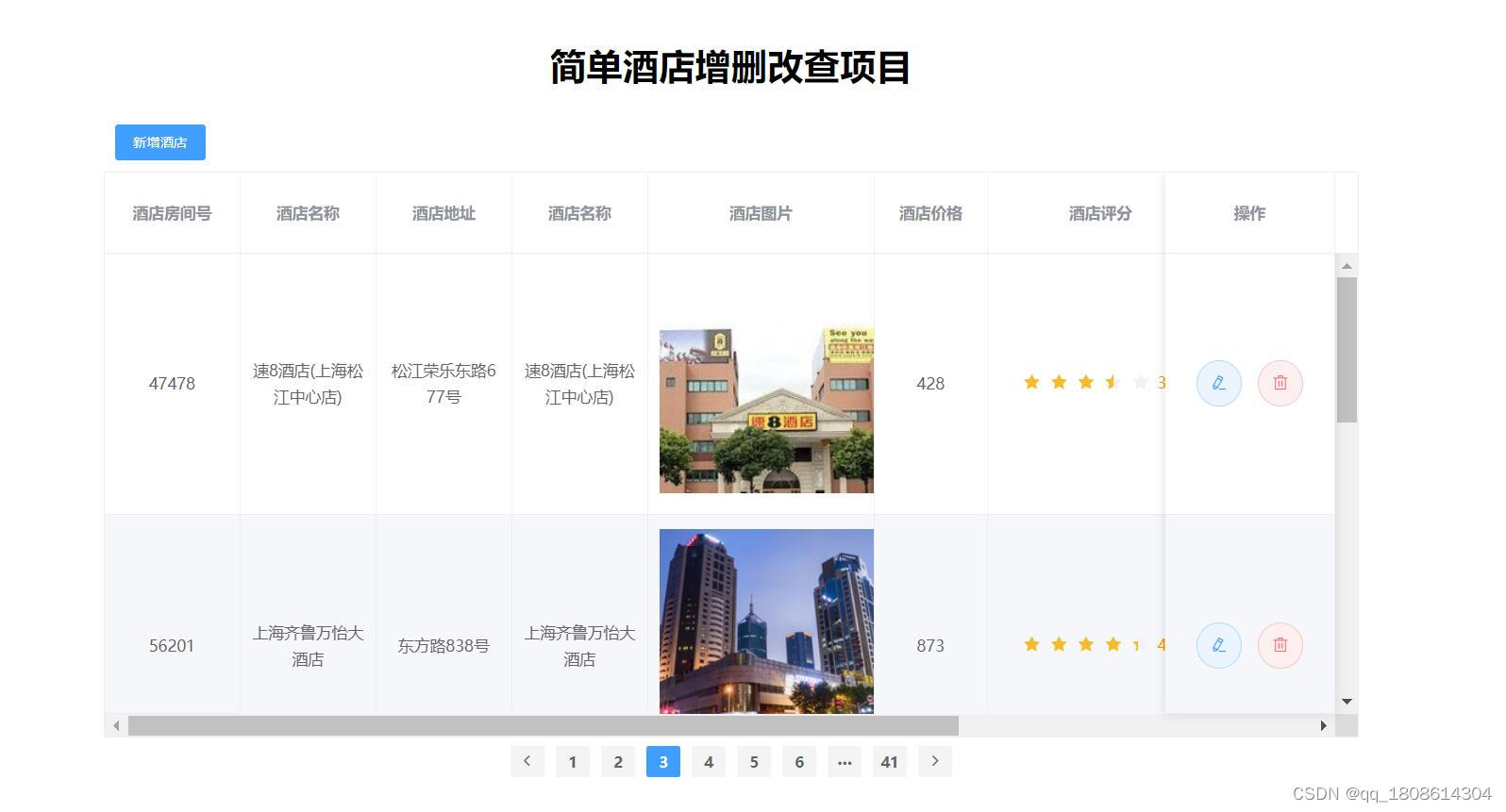
- 增加酒店信息页面
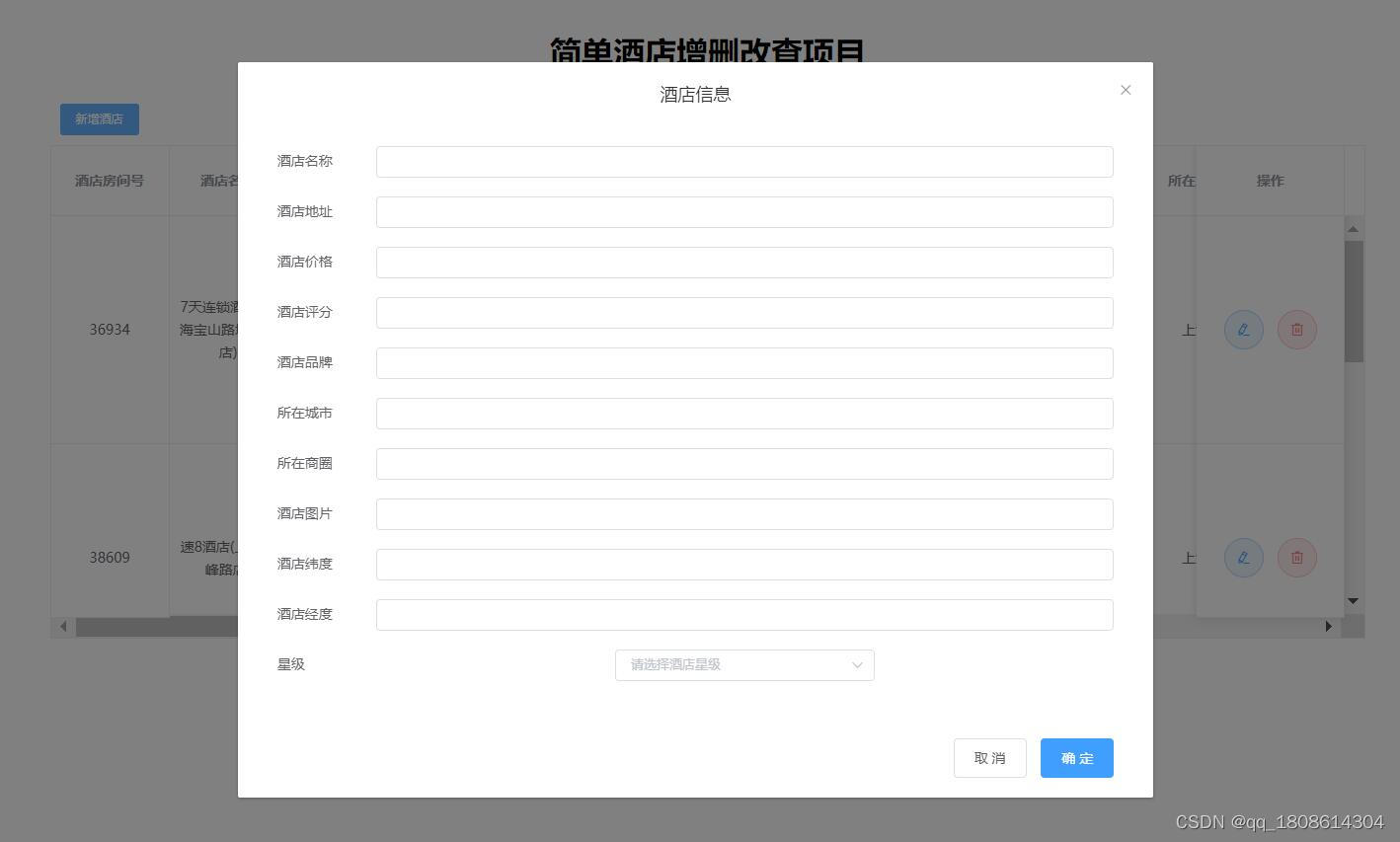
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |