1.先创建一个ASP.Net Web应用程序,选择Web API
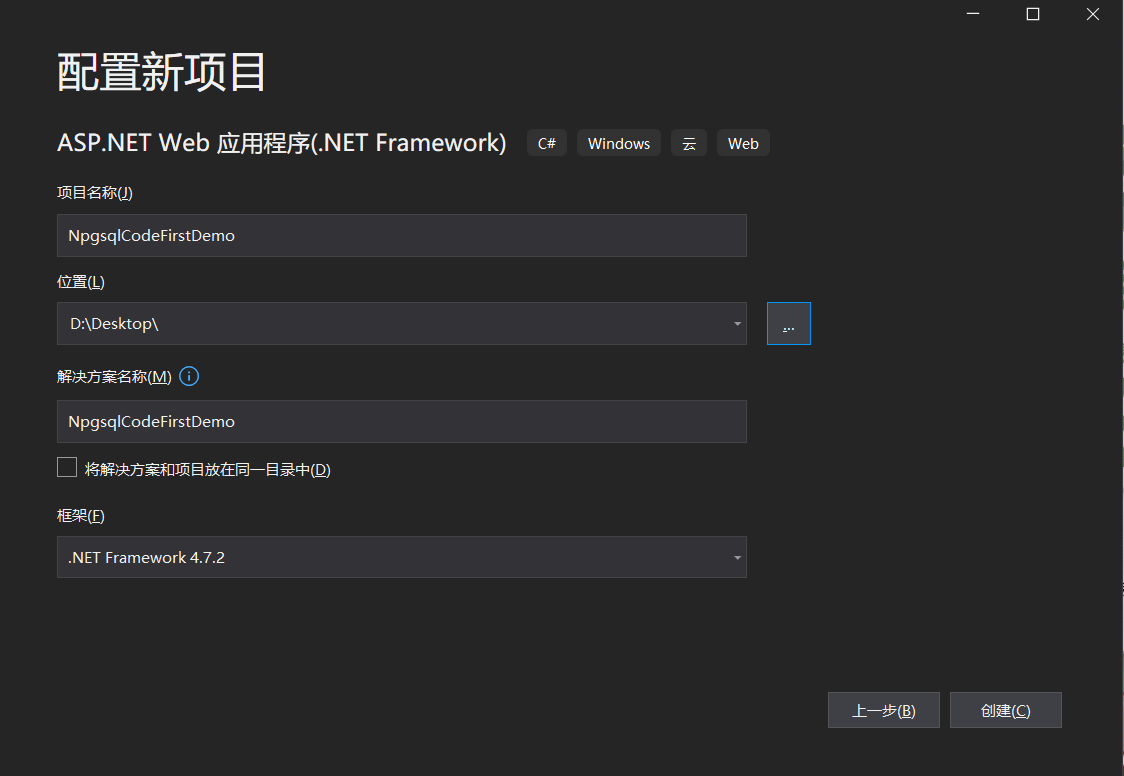
2、创建EntityLib、EF、AppService三个类库。EntityLib用于存放数据库表所对应的实体,AppService用于编写用户对实体的一些操作方法,如增删改查等操作。
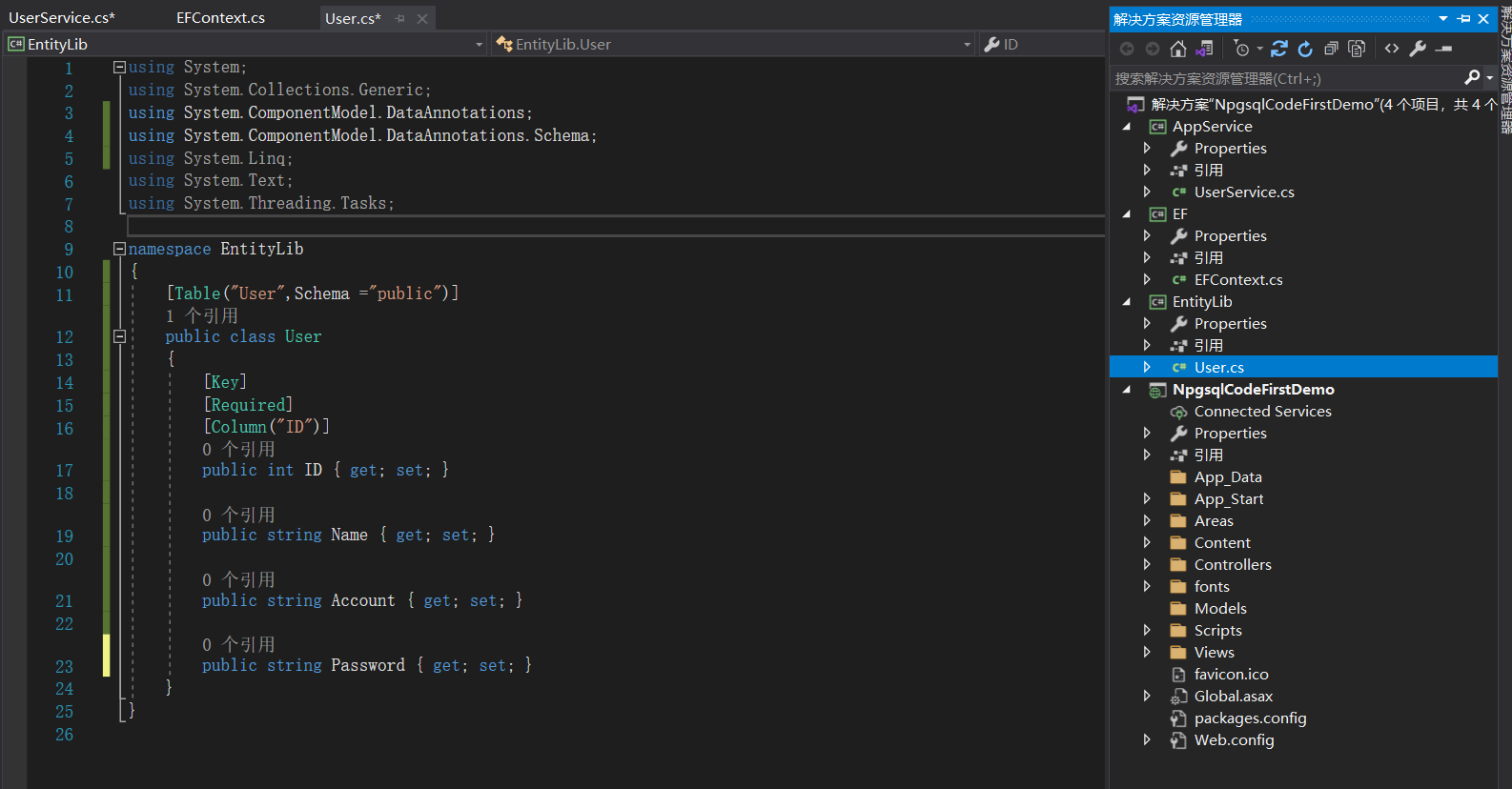
创建好所有类库之后,需要添加引用库EntityFramework6.Npgsql,右击项目中的引用———》管理NuGet程序包———》搜索EntityFramework6.Npgsql添加到项目中,这个库会自动添加EntityFramework6和Npgsql的引用。需要给EF、AppService和API都添加这个引用。添加完成之后就开始写各个类库相应的代码了。
EFContext类- public class EFContext : DbContext
- {
- public EFContext() : base("Postgresql")//连接字符串名称
- {
- }
- protected override void OnModelCreating(DbModelBuilder modelBuilder)
- {
- <connectionStrings>
- <add name="Postgresql" connectionString="PORT=502;DATABASE=postgres;HOST=10.60.140.1;PASSWORD=007;USER ID=postgres" providerName="Npgsql" />
- </connectionStrings>modelBuilder.HasDefaultSchema("public");//EF默认创建到dbo架构中,而PostgreSQL默认为public架构
- <connectionStrings>
- <add name="Postgresql" connectionString="PORT=502;DATABASE=postgres;HOST=10.60.140.1;PASSWORD=007;USER ID=postgres" providerName="Npgsql" />
- </connectionStrings>modelBuilder.Conventions.Remove<PluralizingTableNameConvention>();
- <connectionStrings>
- <add name="Postgresql" connectionString="PORT=502;DATABASE=postgres;HOST=10.60.140.1;PASSWORD=007;USER ID=postgres" providerName="Npgsql" />
- </connectionStrings>base.OnModelCreating(modelBuilder);
- }
- public DbSet<User> Users { get; set; }
- }
复制代码
User类- [Table("User",Schema ="public")]
- public class User
- {
- [Key]
- [Required]
- [Column("ID")]
- public int ID { get; set; }
- public string Name { get; set; }
- public string Account { get; set; }
- public string Password { get; set; }
- }
复制代码
AppService类完成类的编写之后,需要添加数据库连接字符串,打开API的web.config文件添加:
 - <connectionStrings>
- <add name="Postgresql" connectionString="PORT=502;DATABASE=postgres;HOST=10.60.140.1;PASSWORD=007;USER ID=postgres" providerName="Npgsql" />
- </connectionStrings>
复制代码 3.进行数据迁移,通过命令将实体类导入数据库生成相应的数据库表。先打开VS的工具,然后点击NuGet包管理器 ,选择程序包管理器控制台,默认项目改为EF,输入以下三行命令
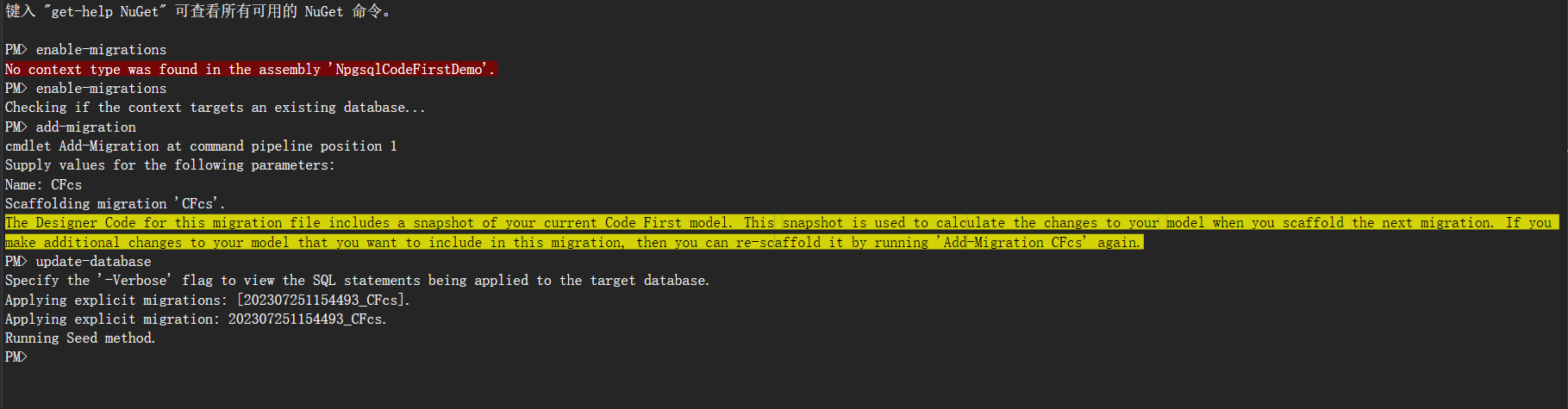
1-》enable-migrations
2-》add-migration
3-》update-database
去PostgreSQL数据库去查看发现已经生成好了数据库和对应的表了,这里因为我postgres数据库已经有User表了,所以修改了一下数据库连接字符串
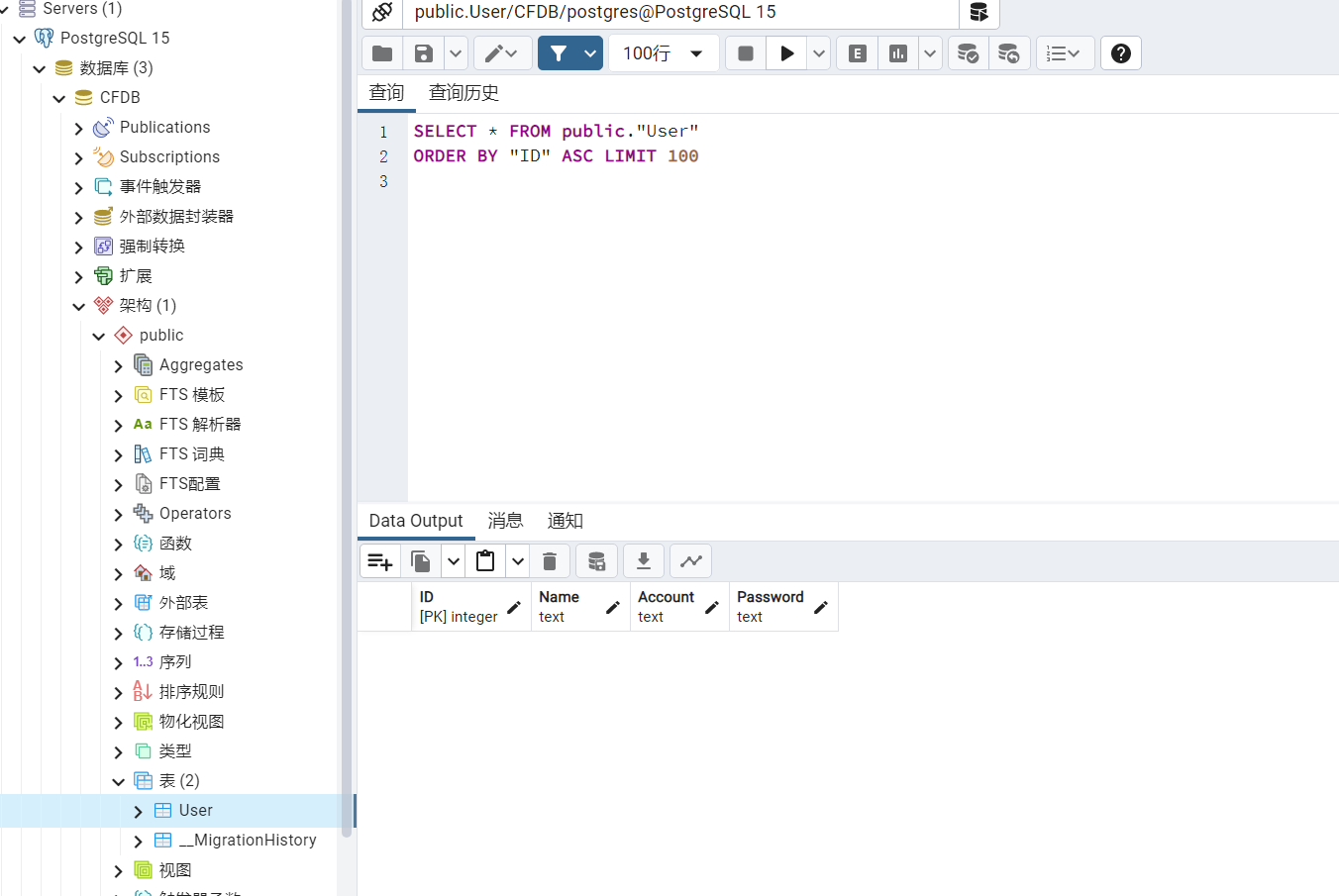
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |