参考:(17条消息) 手把手搭建一个完整的javaweb项目(适合新手)_心歌技术的博客-CSDN博客_javaweb项目完整案例
补充项目结构的细节,进行了一点修改,修改为学生信息管理系统
以下是搭建过程:
1.项目结构
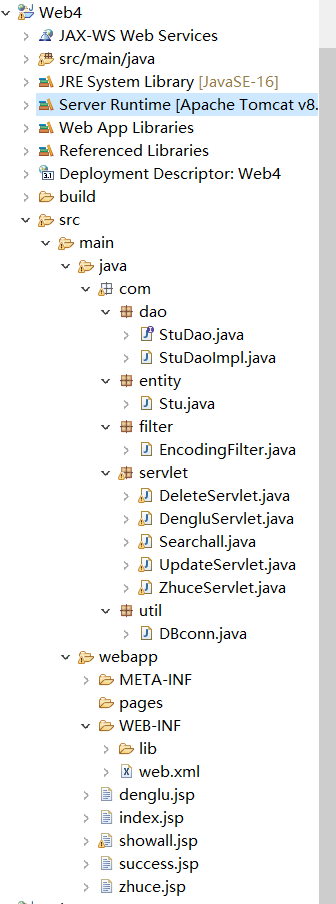
2.数据库结构
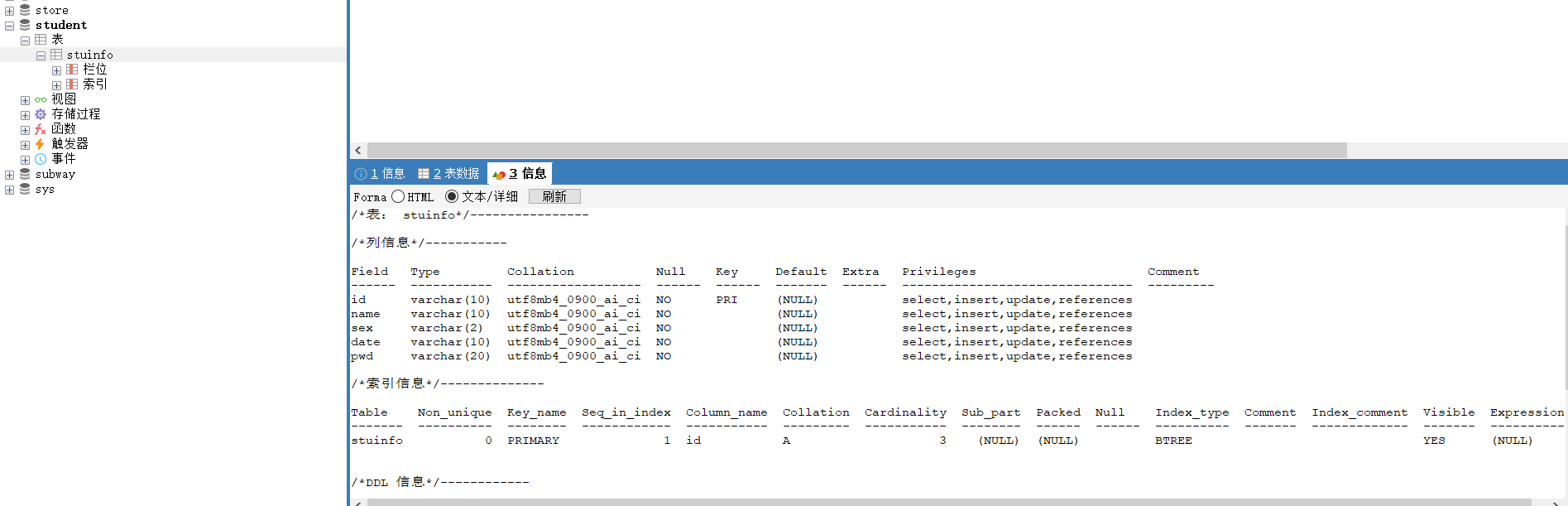
3.代码部分
com.dao.StuDao.java- package com.dao;
- import java.util.List;
- import com.entity.Stu;
- public interface StuDao {
- public boolean login(String id,String pwd);
- public boolean register(Stu stu);
- public List<Stu> getStuAll();
- public boolean delete(String id);
- public boolean update(String id,String name,String sex,String date,String pwd);
- }
复制代码 com.dao.StuDaoImpl.java- package com.dao;
- import java.sql.ResultSet;
- import java.sql.SQLException;
- import java.util.ArrayList;
- import java.util.List;
-
- import com.entity.Stu;
- import com.util.DBconn;
- public class StuDaoImpl implements StuDao{
-
- public boolean register(Stu stu) {
- boolean flag = false;
- DBconn.init();
- int i = DBconn.addUpdDel("INSERT INTO stuinfo(id,name,sex,date,pwd)" + "values('"+stu.getId()+"','"+stu.getName()+"','" +stu.getSex()+"','"+ stu.getDate()+"','"+stu.getPwd()+"')");
- if(i>0) {
- flag = true;
- }
- DBconn.closeConn();
- return flag;
- }
-
-
- public boolean login(String id,String pwd) {
- boolean flag = false;
- try {
- DBconn.init();
- ResultSet rs = DBconn.selectSql("SELECT * FROM stuinfo WHERE id='"+id+"'and pwd='"+pwd+"'");
- while(rs.next()) {
- if(rs.getString("id").equals(id) && rs.getString("pwd").equals(pwd)) {
- flag = true;
- }
-
- }
- DBconn.closeConn();
- }catch(SQLException e) {
- e.printStackTrace();
- }
- return flag;
- }
-
- public List<Stu> getStuAll(){
- List<Stu> list = new ArrayList<Stu>();
- try {
- DBconn.init();
- ResultSet rs = DBconn.selectSql("SELECT * FROM stuinfo");
- while(rs.next()) {
- Stu stu = new Stu();
- stu.setId(rs.getString("id"));
- stu.setName(rs.getString("name"));
- stu.setSex(rs.getString("sex"));
- stu.setDate(rs.getString("date"));
- stu.setPwd(rs.getString("pwd"));
- list.add(stu);
- }
- DBconn.closeConn();
- return list;
- }catch(SQLException e) {
- e.printStackTrace();
- }
- return null;
- }
-
- public boolean update(String id,String name,String sex,String date,String pwd) {
- boolean flag = false;
- DBconn.init();
- String sql = "UPDATE stuinfo set name ="
- +
- " '"+name+"',sex ='"+sex
- +"',date ='"+date
- +"',pwd ='"+pwd+"'WHERE id ="+id;
- int i = DBconn.addUpdDel(sql);
- if(i>0) {
- flag=true;
- }
- DBconn.closeConn();
- return flag;
- }
-
- public boolean delete(String id) {
- boolean flag = false;
- DBconn.init();
- String sql = "DELETE FROM stuinfo WHERE id ="+id;
- int i = DBconn.addUpdDel(sql);
- if(i>0){
- flag = true;
- }
- DBconn.closeConn();
- return flag;
- }
- }
复制代码 com.entity.Stu.java- package com.entity;
- public class Stu {
- private String id;
- private String name;
- private String sex;
- private String date;
- private String pwd;
-
- public String getId() {
- return id;
- }
- public void setId(String id) {
- this.id = id;
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- public String getSex() {
- return sex;
- }
- public void setSex(String sex) {
- this.sex = sex;
- }
- public String getDate() {
- return date;
- }
- public void setDate(String date) {
- this.date = date;
- }
- public String getPwd() {
- return pwd;
- }
- public void setPwd(String pwd) {
- this.pwd = pwd;
- }
-
-
- }
复制代码 com.filter.EncodingFilter.java- package com.filter;
- import java.io.IOException;
-
- import javax.servlet.Filter;
- import javax.servlet.FilterChain;
- import javax.servlet.FilterConfig;
- import javax.servlet.ServletException;
- import javax.servlet.ServletRequest;
- import javax.servlet.ServletResponse;
-
- public class EncodingFilter implements Filter{
- public EncodingFilter(){
- System.out.println("过滤器构造");
- }
- public void destroy() {
- System.out.println("过滤器销毁");
- }
- public void doFilter(ServletRequest request, ServletResponse response,FilterChain chain) throws IOException, ServletException {
- request.setCharacterEncoding("utf-8"); //将编码改为utf-8
- response.setContentType("text/html;charset=utf-8");
- chain.doFilter(request, response);
- }
-
- public void init(FilterConfig arg0) throws ServletException {
- System.out.println("过滤器初始化");
- }
-
- }
复制代码 com.servket.DeleteServlet.java- package com.servlet;
-
- import java.io.IOException;
- import java.io.PrintWriter;
-
- import javax.servlet.ServletException;
- import javax.servlet.ServletRequest;
- import javax.servlet.ServletResponse;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
-
- import com.dao.StuDao;
- import com.dao.StuDaoImpl;
-
- public class DeleteServlet extends HttpServlet {
- @Override
- public void doGet(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- doPost(request, response);
- }
- @Override
- public void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
-
- String id = request.getParameter("id");
-
- StuDao ud = new StuDaoImpl();
-
- if(ud.delete(id)){
- request.setAttribute("xiaoxi", "删除成功");
- request.getRequestDispatcher("/Searchall").forward(request, response);
- }else{
- response.sendRedirect("index.jsp");
- }
- }
-
-
- }
复制代码 com.servlet.DengluServlet.java- package com.servlet;
-
- import java.io.IOException;
- import java.io.PrintWriter;
-
- import javax.servlet.ServletException;
- import javax.servlet.ServletRequest;
- import javax.servlet.ServletResponse;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
-
- import com.dao.StuDao;
- import com.dao.StuDaoImpl;
-
- public class DengluServlet extends HttpServlet { //需要继承HttpServlet 并重写doGet doPost方法
-
- @Override
- public void doGet(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- doPost(request, response); //将信息使用doPost方法执行 对应jsp页面中的form表单中的method
- }
- @Override
- public void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
-
- String id = request.getParameter("id"); //得到jsp页面传过来的参数
- String pwd = request.getParameter("pwd");
-
-
- StuDao ud = new StuDaoImpl();
-
- if(ud.login(id, pwd)){
- request.setAttribute("xiaoxi", "欢迎用户"+id); //向request域中放置信息
- request.getRequestDispatcher("/success.jsp").forward(request, response);//转发到成功页面
- }else{
- response.sendRedirect("index.jsp"); //重定向到首页
- }
- }
-
-
- }
复制代码 com.servlet.Searchall.java- package com.servlet;
-
- import java.io.IOException;
- import java.io.PrintWriter;
- import java.util.List;
-
- import javax.servlet.ServletException;
- import javax.servlet.ServletRequest;
- import javax.servlet.ServletResponse;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
-
- import com.dao.StuDao;
- import com.dao.StuDaoImpl;
- import com.entity.Stu;
-
- public class Searchall extends HttpServlet {
- @Override
- public void doGet(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- doPost(request, response);
- }
- @Override
- public void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
-
- StuDao ud = new StuDaoImpl();
- List<Stu> stuAll = ud.getStuAll();
- request.setAttribute("stuAll", stuAll);
- request.getRequestDispatcher("/showall.jsp").forward(request, response);
- }
-
- }
复制代码
com.servlet.UpdateServlet.java- package com.servlet;
-
- import java.io.IOException;
- import java.io.PrintWriter;
-
- import javax.servlet.ServletException;
- import javax.servlet.ServletRequest;
- import javax.servlet.ServletResponse;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
-
- import com.dao.StuDao;
- import com.dao.StuDaoImpl;
- import com.entity.Stu;
-
- public class UpdateServlet extends HttpServlet {
- @Override
- public void doGet(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- doPost(request, response);
- }
- @Override
- public void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
-
- String id = request.getParameter("id");
- String name = request.getParameter("name");
- String sex = request.getParameter("sex");
- String date = request.getParameter("date");
- String pwd = request.getParameter("pwd");
-
- System.out.println("------------------------------------"+id);
-
- StuDao ud = new StuDaoImpl();
-
- if(ud.update(id, name, sex, date, pwd)){
- request.setAttribute("xiaoxi", "更新成功");
- request.getRequestDispatcher("/Searchall").forward(request, response);
- }else{
- response.sendRedirect("index.jsp");
- }
- }
-
-
- }
复制代码
com.servlet.ZhuceServlet.java- package com.servlet;
-
- import java.io.IOException;
- import java.io.PrintWriter;
-
- import javax.servlet.ServletException;
- import javax.servlet.ServletRequest;
- import javax.servlet.ServletResponse;
- import javax.servlet.http.HttpServlet;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- import com.dao.StuDao;
- import com.dao.StuDaoImpl;
- import com.entity.Stu;
-
- public class ZhuceServlet extends HttpServlet {
- @Override
- public void doGet(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
- doPost(request, response);
- }
- @Override
- public void doPost(HttpServletRequest request, HttpServletResponse response)
- throws ServletException, IOException {
-
- String id = request.getParameter("id");
- String name = request.getParameter("name"); //获取jsp页面传过来的参数
- String sex = request.getParameter("sex");
- String date = request.getParameter("date");
- String pwd = request.getParameter("pwd");
-
-
-
-
- Stu stu = new Stu(); //实例化一个对象,组装属性
- stu.setId(id);
- stu.setName(name);
- stu.setSex(sex);
- stu.setDate(date);
- stu.setPwd(pwd);
- StuDao ud = new StuDaoImpl();
-
-
- if(ud.register(stu)){
- request.setAttribute("username", name); //向request域中放置参数
- //request.setAttribute("xiaoxi", "注册成功");
- request.getRequestDispatcher("/denglu.jsp").forward(request, response); //转发到登录页面
- }else{
-
- response.sendRedirect("index.jsp");//重定向到首页
- }
- }
-
- }
复制代码
com.util.DBconn.java- package com.util;
-
- import java.sql.*;
-
- public class DBconn {
- static String url = "jdbc:mysql://localhost:3306/student?useunicuee=true& characterEncoding=utf8";
- static String username = "root";
- static String password = "123456";
- static Connection conn = null;
- static ResultSet rs = null;
- static PreparedStatement ps =null;
- public static void init(){
- try {
- Class.forName("com.mysql.jdbc.Driver");
- conn = DriverManager.getConnection(url,username,password);
- } catch (Exception e) {
- System.out.println("init [SQL驱动程序初始化失败!]");
- e.printStackTrace();
- }
- }
- public static int addUpdDel(String sql){
- int i = 0;
- try {
- PreparedStatement ps = conn.prepareStatement(sql);
- i = ps.executeUpdate();
- } catch (SQLException e) {
- System.out.println("sql数据库增删改异常");
- e.printStackTrace();
- }
-
- return i;
- }
- public static ResultSet selectSql(String sql){
- try {
- ps = conn.prepareStatement(sql);
- rs = ps.executeQuery(sql);
- } catch (SQLException e) {
- System.out.println("sql数据库查询异常");
- e.printStackTrace();
- }
- return rs;
- }
- public static void closeConn(){
- try {
- conn.close();
- } catch (SQLException e) {
- System.out.println("sql数据库关闭异常");
- e.printStackTrace();
- }
- }
- }
复制代码
web.xml- <?xml version="1.0" encoding="UTF-8"?>
- <web-app version="3.1" xmlns="http://xmlns.jcp.org/xml/ns/javaee"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd">
- <display-name></display-name>
-
- <filter>
- <filter-name>EncodingFilter</filter-name>
- <filter-class>com.filter.EncodingFilter</filter-class>
- </filter>
- <filter-mapping>
- <filter-name>EncodingFilter</filter-name>
- <url-pattern>/*</url-pattern>
- </filter-mapping>
-
-
-
- <servlet>
- <servlet-name>DengluServlet</servlet-name>
- <servlet-class>com.servlet.DengluServlet</servlet-class>
- </servlet>
- <servlet>
- <servlet-name>ZhuceServlet</servlet-name>
- <servlet-class>com.servlet.ZhuceServlet</servlet-class>
- </servlet>
- <servlet>
- <servlet-name>Searchall</servlet-name>
- <servlet-class>com.servlet.Searchall</servlet-class>
- </servlet>
- <servlet>
- <servlet-name>DeleteServlet</servlet-name>
- <servlet-class>com.servlet.DeleteServlet</servlet-class>
- </servlet>
- <servlet>
- <servlet-name>UpdateServlet</servlet-name>
- <servlet-class>com.servlet.UpdateServlet</servlet-class>
- </servlet>
-
-
-
- <servlet-mapping>
- <servlet-name>DengluServlet</servlet-name>
- <url-pattern>/DengluServlet</url-pattern>
- </servlet-mapping>
- <servlet-mapping>
- <servlet-name>ZhuceServlet</servlet-name>
- <url-pattern>/ZhuceServlet</url-pattern>
- </servlet-mapping>
- <servlet-mapping>
- <servlet-name>Searchall</servlet-name>
- <url-pattern>/Searchall</url-pattern>
- </servlet-mapping>
- <servlet-mapping>
- <servlet-name>DeleteServlet</servlet-name>
- <url-pattern>/DeleteServlet</url-pattern>
- </servlet-mapping>
- <servlet-mapping>
- <servlet-name>UpdateServlet</servlet-name>
- <url-pattern>/UpdateServlet</url-pattern>
- </servlet-mapping>
-
-
-
- <welcome-file-list>
-
- <welcome-file>denglu.jsp</welcome-file>
- <welcome-file>zhuce.jsp</welcome-file>
- <welcome-file>index.jsp</welcome-file>
- <welcome-file>success.jsp</welcome-file>
- </welcome-file-list>
- </web-app>
复制代码
denglu.jsp- <%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
- <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
- <html>
- <head>
- <title>登录注册页面</title>
- </head>
- <body >
- <p>用户登录</p>
- <form action="DengluServlet" method="post" style="padding-top:-700px;">
- 用户名:<input type="text" name="id" value=""><br><br>
- 密码: <input type="password" name="pwd" value=""><br><br>
- <input type="submit"value="登录"name="denglu"><input type="reset"value="重置"><br>
- </form>
- <form action="zhuce.jsp">
- <input type="submit"value="新用户注册">
- </form>
-
- </body>
- </html>
复制代码
index.jsp- <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
- <%
- String path = request.getContextPath();
- String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
- %>
- <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
- <html>
- <head>
- <title>My JSP 'index.jsp' starting page</title>
- </head>
- <body>
- <h1>失敗</h1>
- </body>
- </html>
复制代码
showall.jsp- <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
- <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
- <%
- String path = request.getContextPath();
- String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
- %>
- <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
- <html>
- <head>
- <base href="<%=basePath%>">
- <title>所有用户页面</title>
- </head>
-
- <body>
- <h1>${xiaoxi}</h1>
- <table width="600" border="1" cellpadding="0" >
- <tr>
- <th>用户名</th>
- <th>姓名</th>
- <th>性别</th>
- <th>生日</th>
- <th>密码</th>
-
- </tr>
- <c:forEach var="U" items="${stuAll}" >
- <form action="UpdateServlet" method="post">
-
- <tr>
- <td><input type="text" value="${U.id}" name="id" ></td>
- <td><input type="text" value="${U.name}" name="name"></td>
- <td><input type="text" value="${U.sex}" name="sex"></td>
- <td><input type="text" value="${U.date}" date="date"></td>
- <td><input type="text" value="${U.pwd}" name="pwd"></td>
-
-
- <td><a target="_blank" href="https://www.cnblogs.com/DeleteServlet?id=${U.id}">删除</a> <input type="submit" value="更新"/></td>
- </tr>
- </form>
- </c:forEach>
- </table>
- </body>
- </html>
复制代码
success.jsp- <%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
- <%
- String path = request.getContextPath();
- String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
- %>
- <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
- <html>
- <head>
- <title>My JSP 'success.jsp' starting page</title>
- </head>
- <body>
- ${xiaoxi} <br>
- <a target="_blank" href="https://www.cnblogs.com/Searchall">查看所有用户</a>
- </body>
- </html>
复制代码
zhuce.jsp- <%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
- <%
- String path = request.getContextPath();
- String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
- %>
- <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
- <html>
- <head>
- <title>My JSP 'BB.jsp' starting page</title>
- </head>
- <body >
- <form action="ZhuceServlet"method="post" style="padding-top:-700px;">
-
- 输入用户名:
- <input name="id" type="text"><br><br>
-
- 输入姓名:
- <input name="name" type="text"><br><br>
-
- 选择性别:
- <input type="radio" name="sex" value="男"checked>男
- <input type="radio" name="sex"value="女">女<br><br>
-
- 输入生日:
- <input name="date" type="text"><br><br>
- 输入密码:
- <input name="pwd" type="password"><br><br>
- <input type="reset"value="重置"><input type="submit"value="注册">
- </form>
- </body>
- </html>
复制代码
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |