目录:
(1)前台用户体系-阿里云OSS先容
(2)阿里云oss代码入门案例
(3)上传文件到阿里云oss-接口开发
(4)用户认证-接口开发
(5)用户认证-前端整合
(1)前台用户体系-阿里云OSS先容
扫码登录后显示用户的昵称,点击下面会显示一些下拉列表,下面完成这些功能
实名认证:在里面申请某些事情,注册某些软件,需要用到某些功能,需要做一个实名认证,比如说填写你的信息,上传身份证号等等,进行认证,提交之后由平台考核,考核通过之后才能进行预约挂号操作
挂号订单:通过它可以看到当天用户曾经挂了哪些 号,号是什么样的信息
就诊人管理:在挂号的时间可以填写由谁挂号,在注册用户里面可以添加多个就诊人,比如说把父母添加进去,帮他们挂号
先做实名认证功能:需要先相识一个技术:阿里云oss
做实名认证的时间需要,上传一些信息,比如说一个头像,上传身份证的正面,反面信息,上传内容需要存储到服务器里面,阿里云oss就是一个存储服务器,是阿里云为我们提供的一个文件存储体系,方便我们存储,实现文件的上传和下载功能。
用户认证需要上传证件图片、首页轮播也需要上传图片,因此我们要做文件服务,阿里云oss是一个很好的分布式文件服务体系,所以我们只需要集成阿里云oss即可
1、开通“对象存储OSS”服务
(1)申请阿里云账号
(2)实名认证
(3)开通“对象存储OSS”服务
(4)进入管理控制台
1.1创建Bucket
选择:标准存储、公共读、不开通
1.2上传默认头像
创建文件夹avatar,上传默认的用户头像
:
搜索:
创建Bucket:点击Bucket列表:
点击创建Bucket:新用户标准存储免费一段时间
点击确定:
查察这个:下面有Bucket域名
测试以下存储:点击文件管理
点击上传文件:
把一个图片拖沓到这里:
点击上传文件:
点击详情:
在浏览器输入地址:
直接下载了这个图片:
上面的操作我是通过控制台操作的,在实际的项目中通过代码实现,写代码需要用到 accesskeys:
使用当前账户的accessKeys里面的id和秘钥
(2)阿里云oss代码入门案例
查察资助文档:
新建 一个Module:
在这个pom中引入依靠:
- <?xml version="1.0" encoding="UTF-8"?>
- <project xmlns="http://maven.apache.org/POM/4.0.0"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
- <parent>
- <artifactId>service</artifactId>
- <groupId>com.atguigu</groupId>
- <version>0.0.1-SNAPSHOT</version>
- </parent>
- <modelVersion>4.0.0</modelVersion>
- <artifactId>service_oss</artifactId>
- <dependencies>
- <!-- 阿里云oss依赖 -->
- <dependency>
- <groupId>com.aliyun.oss</groupId>
- <artifactId>aliyun-sdk-oss</artifactId>
- </dependency>
- <!-- 日期工具栏依赖 -->
- <dependency>
- <groupId>joda-time</groupId>
- <artifactId>joda-time</artifactId>
- </dependency>
- </dependencies>
- </project>
复制代码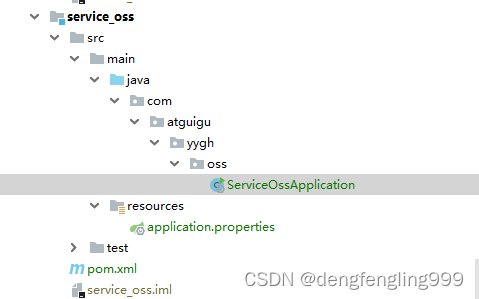
创建设置文件:application.properties:
- # 服务端口
- server.port=8205
- # 服务名
- spring.application.name=service-oss
- #返回json的全局时间格式
- spring.jackson.date-format=yyyy-MM-dd HH:mm:ss
- spring.jackson.time-zone=GMT+8
- # nacos服务地址
- spring.cloud.nacos.discovery.server-addr=127.0.0.1:8848
- #阿里云存储的配置
- aliyun.oss.endpoint=http://oss-cn-beijing.aliyuncs.com
- aliyun.oss.accessKeyId=LTAI5tF2Ej79XRwCiYbiNRyR
- aliyun.oss.secret=zvSy5n0sifBKZEmD77glgqbOcuiAp8
复制代码 创建启动类:
- package com.atguigu.yygh.oss;
- import org.springframework.boot.SpringApplication;
- import org.springframework.boot.autoconfigure.SpringBootApplication;
- import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
- import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
- import org.springframework.context.annotation.ComponentScan;
- @SpringBootApplication(exclude = DataSourceAutoConfiguration.class)//取消数据源自动配置,配置文件中没有配置数据库的配置,所以这里需要取消加载数据库的配置
- @EnableDiscoveryClient
- @ComponentScan(basePackages = {"com.atguigu"}) //配置相同路径可以使用Swagger文档了
- public class ServiceOssApplication {
- public static void main(String[] args) {
- SpringApplication.run(ServiceOssApplication.class, args);
- }
- }
复制代码 设置网关:
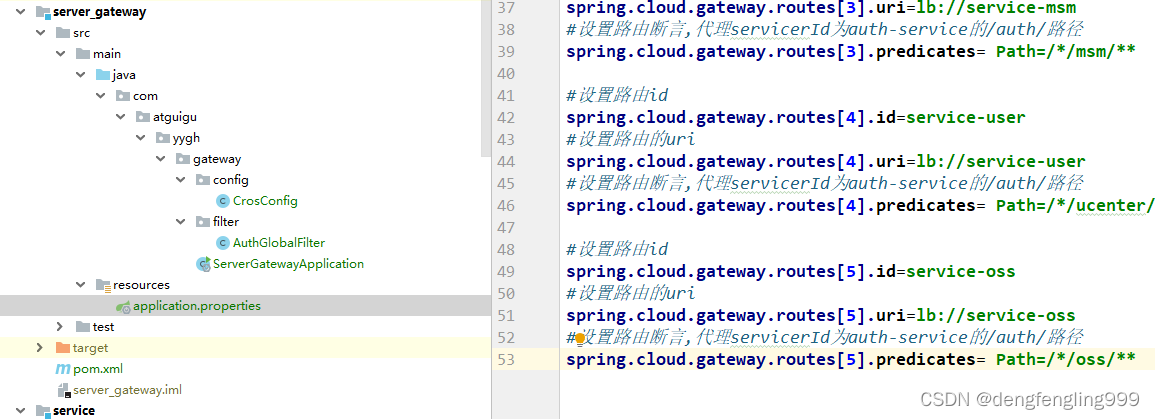
编写测试代码:
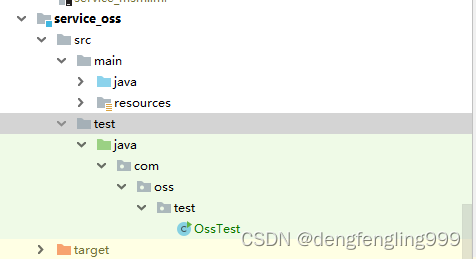
使用java代码创建bucket:
- package com.oss.test;
- import com.aliyun.oss.OSS;
- import com.aliyun.oss.OSSClientBuilder;
- import com.aliyun.oss.model.CannedAccessControlList;
- public class OssTest {
- public static void main(String[] args) {
- // Endpoint以华东1(杭州)为例,其它Region请按实际情况填写。
- String endpoint = "https://oss-cn-beijing.aliyuncs.com";
- // 阿里云账号AccessKey拥有所有API的访问权限,风险很高。强烈建议您创建并使用RAM用户进行API访问或日常运维,请登录RAM控制台创建RAM用户。
- String accessKeyId = "LTAI5tF2Ej79XRwCiYbiNRyR";
- String accessKeySecret = "zvSy5n0sifBKZEmD77glgqbOcuiAp8";
- // 填写Bucket名称,例如examplebucket。
- String bucketName = "yygh-tetss";
- // 创建OSSClient实例。
- OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
- // 创建存储空间。
- ossClient.createBucket(bucketName);
- // 设置存储空间的读写权限。例如将examplebucket的读写权限ACL设置为公有
- ossClient.setBucketAcl(bucketName, CannedAccessControlList.PublicRead);
- //关闭ossClient
- ossClient.shutdown();
- }
- }
复制代码
查察阿里云页面:乐成创建yygh-tetss名称的Bucket:
(3)上传文件到阿里云oss-接口开发
查察阿里云文档:上传文件:
使用文件流作为我们的上传代码:
创建controller:
- package com.atguigu.yygh.oss.controller;
- import com.atguigu.yygh.common.result.Result;
- import com.atguigu.yygh.oss.service.FileService;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.web.bind.annotation.PostMapping;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RestController;
- import org.springframework.web.multipart.MultipartFile;
- @RestController
- @RequestMapping("/api/oss/file")
- public class FileApiController {
- @Autowired
- private FileService fileService;
- //上传文件到阿里云oss
- @PostMapping("fileUpload")
- public Result fileUpload(MultipartFile file) {
- //获取上传文件
- String url = fileService.upload(file);
- return Result.ok(url);
- }
- }
复制代码 创建service:
- package com.atguigu.yygh.oss.service;
- import org.springframework.web.multipart.MultipartFile;
- public interface FileService {
- //上传文件到阿里云oss
- String upload(MultipartFile file);
- }
复制代码 在配之类中添加:
添加工具类,获取这些数据:
- package com.atguigu.yygh.oss.utils;
- import org.springframework.beans.factory.InitializingBean;
- import org.springframework.beans.factory.annotation.Value;
- import org.springframework.stereotype.Component;
- @Component
- public class ConstantOssPropertiesUtils implements InitializingBean {
- @Value("${aliyun.oss.endpoint}")
- private String endpoint;
- @Value("${aliyun.oss.accessKeyId}")
- private String accessKeyId;
- @Value("${aliyun.oss.secret}")
- private String secret;
- @Value("${aliyun.oss.bucket}")
- private String bucket;
- public static String EDNPOINT;
- public static String ACCESS_KEY_ID;
- public static String SECRECT;
- public static String BUCKET;
- @Override
- public void afterPropertiesSet() throws Exception {
- EDNPOINT=endpoint;
- ACCESS_KEY_ID=accessKeyId;
- SECRECT=secret;
- BUCKET=bucket;
- }
- }
复制代码 实现类:FileServiceImpl
- package com.atguigu.yygh.oss.service.impl;
- import com.aliyun.oss.OSS;
- import com.aliyun.oss.OSSClientBuilder;
- import com.atguigu.yygh.oss.service.FileService;
- import com.atguigu.yygh.oss.utils.ConstantOssPropertiesUtils;
- import org.joda.time.DateTime;
- import org.springframework.stereotype.Service;
- import org.springframework.web.multipart.MultipartFile;
- import java.io.IOException;
- import java.io.InputStream;
- import java.util.UUID;
- @Service
- public class FileServiceImpl implements FileService {
- //上传文件到阿里云oss
- @Override
- public String upload(MultipartFile file) {
- String endpoint = ConstantOssPropertiesUtils.EDNPOINT;//工具类中的地域节点
- String accessKeyId = ConstantOssPropertiesUtils.ACCESS_KEY_ID;//工具类中的access-key
- String accessKeySecret = ConstantOssPropertiesUtils.SECRECT;//工具类中的secrect
- String bucketName = ConstantOssPropertiesUtils.BUCKET;//工具类上的指定的Bucket存储地址
- try {
- // 创建OSSClient实例。
- OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
- // 上传文件流。
- InputStream inputStream = file.getInputStream();
- String fileName = file.getOriginalFilename();
- //生成随机唯一值,使用uuid,添加到文件名称里面,防止相同文件名导致文件覆盖,因为用户上传的文件名可能会一致
- String uuid = UUID.randomUUID().toString().replaceAll("-",""); //把生成的uuid里面的-替换掉
- fileName = uuid+fileName;
- //按照当前日期,创建文件夹2021/02/02/,上传到创建的文件夹里面, 结果形式为:2021/02/02/uuid01.jpg。
- //因为前面引入了依赖joda-time,所以转日期可以直接.toString("yyyy/MM/dd")
- String timeUrl = new DateTime().toString("yyyy/MM/dd");
- fileName = timeUrl+"/"+fileName;
- //调用方法实现上传
- ossClient.putObject(bucketName, fileName, inputStream);
- // 关闭OSSClient。
- ossClient.shutdown();
- //上传之后文件路径 格式要和阿里云上面的一致
- // https://yygh-atguigu.oss-cn-beijing.aliyuncs.com/uuid01.jpg
- String url = "https://"+bucketName+"."+endpoint+"/"+fileName;
- //返回
- return url;
- } catch (IOException e) {
- e.printStackTrace();
- return null;
- }
- }
- }
复制代码 使用swaggert文档进行测试:
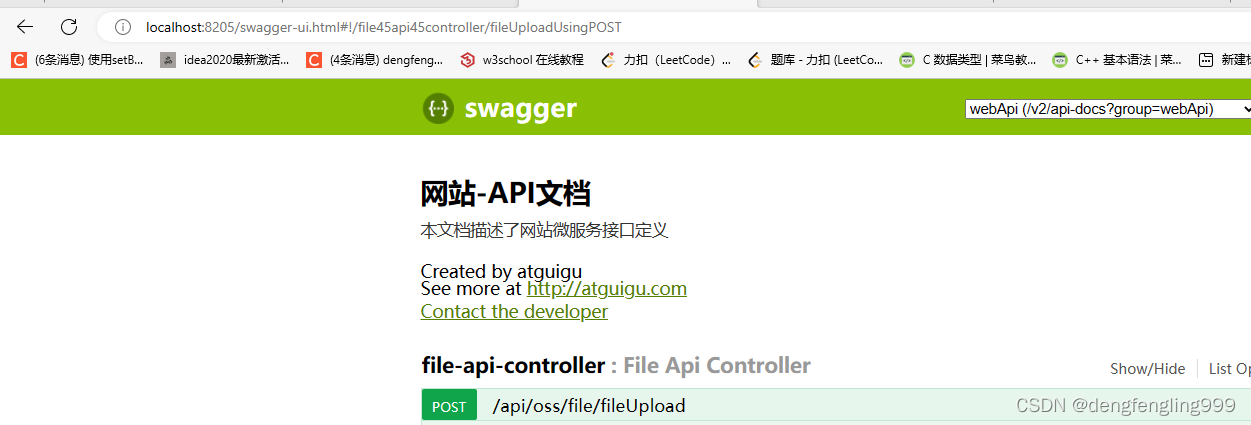
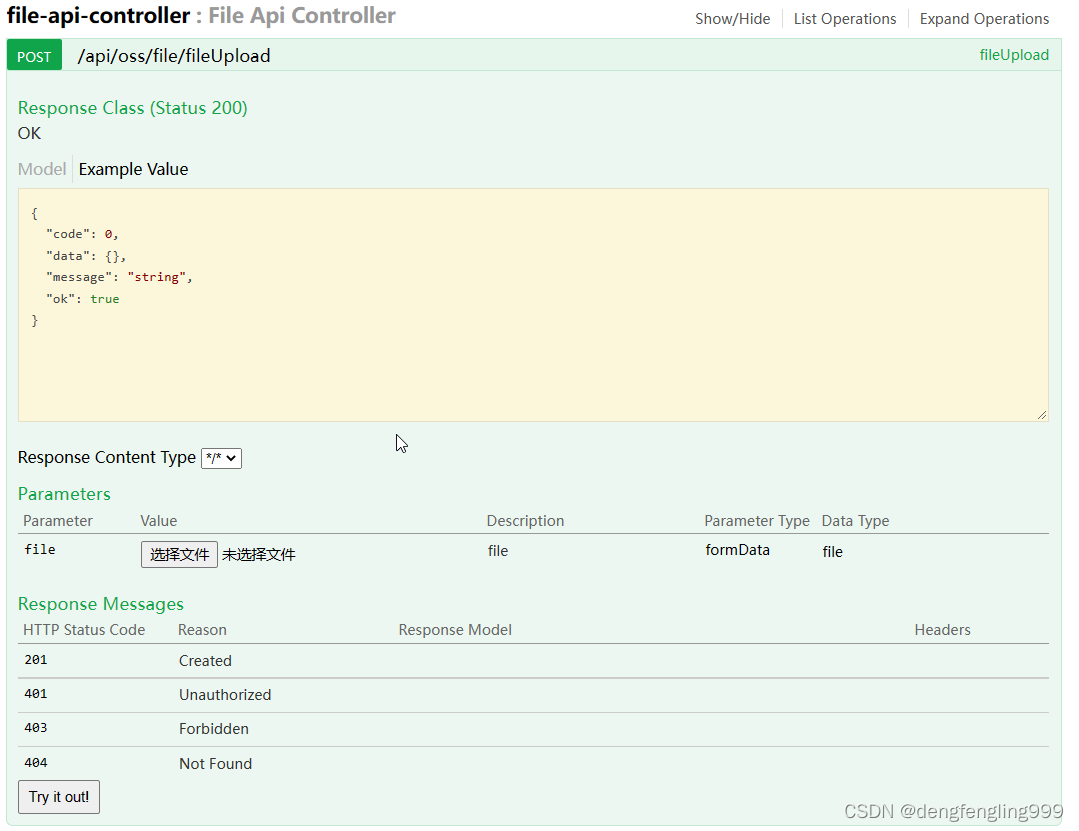 选择文件上传:
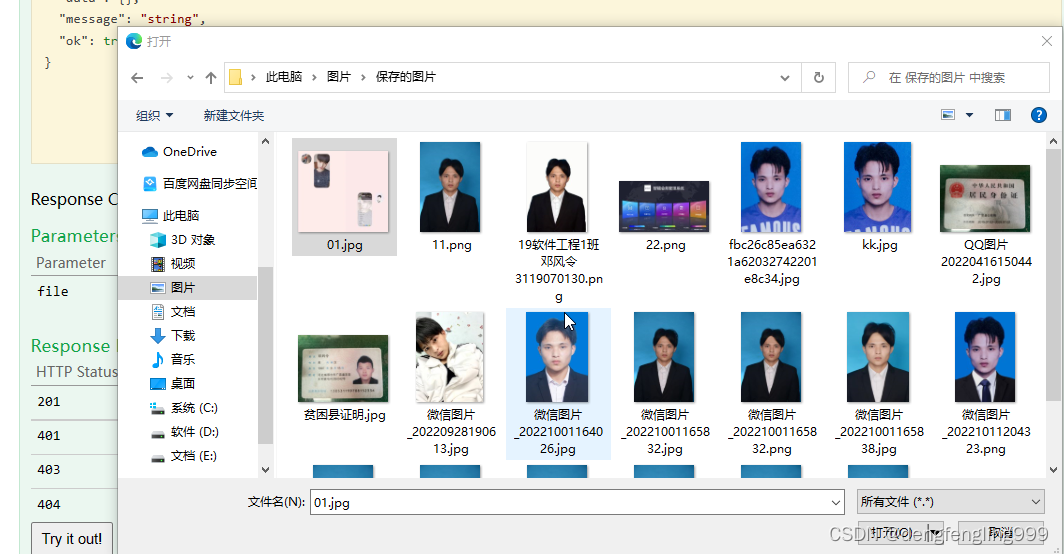
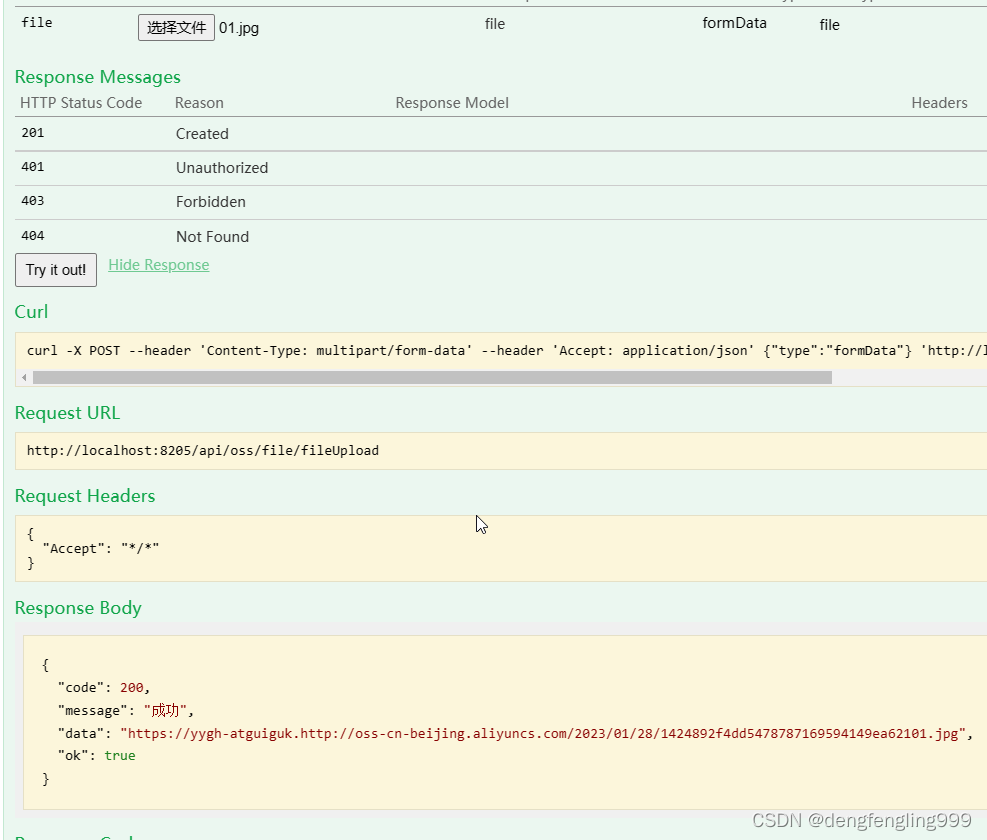 在Bucket中乐成上传:
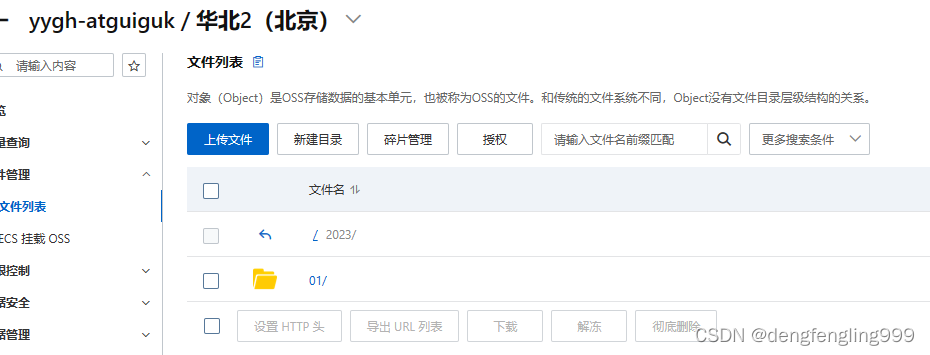
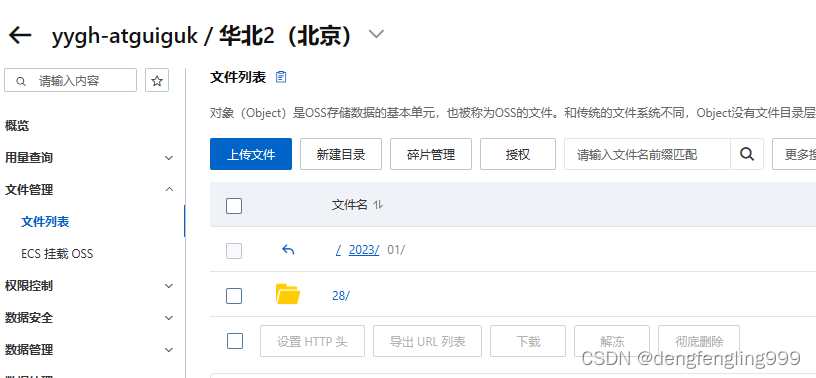
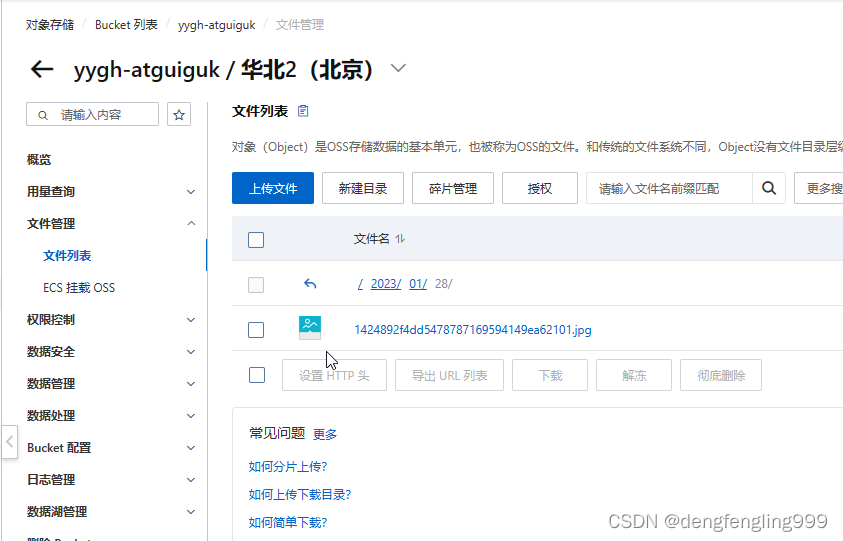
(4)用户认证-接口开发
需求分析
用户登录乐成后都要进行身份认证,认证通事后才可以预约挂号
认证过程:用户填写信息(姓名、证件类型、证件号码和证件照片)==> 平台审批
用户认证设计接口:
起首创建一个工具类,获取用户的信息,这个工具类,在别的地方可能会用到,所以放在common-utils模块中:
创建utils:
- package com.atguigu.yygh.common.utils;
- import com.atguigu.yygh.common.helper.JwtHelper;
- import javax.servlet.http.HttpServletRequest;
- //获取当前用户信息工具类
- public class AuthContextHolder {
- //获取当前用户id
- public static Long getUserId(HttpServletRequest request) {
- //从header获取token
- String token = request.getHeader("token");
- //jwt从token获取userid
- Long userId = JwtHelper.getUserId(token);
- return userId;
- }
- //获取当前用户名称
- public static String getUserName(HttpServletRequest request) {
- //从header获取token
- String token = request.getHeader("token");
- //jwt从token获取userid
- String userName = JwtHelper.getUserName(token);
- return userName;
- }
- }
复制代码 用户认证的操作在service-user中进行编写:
创建认证的实体类:封装的条件
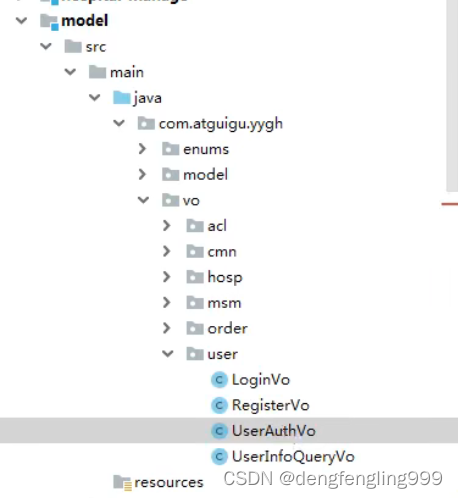
UserAuthVo :
- package com.atguigu.yygh.vo.user;
- import com.baomidou.mybatisplus.annotation.TableField;
- import io.swagger.annotations.ApiModel;
- import io.swagger.annotations.ApiModelProperty;
- import lombok.Data;
- @Data
- @ApiModel(description="会员认证对象")
- public class UserAuthVo {
- @ApiModelProperty(value = "用户姓名")
- @TableField("name")
- private String name;
- @ApiModelProperty(value = "证件类型")
- @TableField("certificates_type")
- private String certificatesType;
- @ApiModelProperty(value = "证件编号")
- @TableField("certificates_no")
- private String certificatesNo;
- @ApiModelProperty(value = "证件路径")
- @TableField("certificates_url")
- private String certificatesUrl;
- }
复制代码 在UserInfoApiController:继续添加接口访问:用户认证接口:
- //用户认证接口
- @PostMapping("auth/userAuth")
- public Result userAuth(@RequestBody UserAuthVo userAuthVo, HttpServletRequest request) {
- //其实就是往user_info补充添加一些认证数据进去,根据id查出记录会修改记录
- //传递两个参数,第一个参数用户id,第二个参数认证数据vo对象
- userInfoService.userAuth(AuthContextHolder.getUserId(request),userAuthVo);
- return Result.ok();
- }
复制代码 service接口:UserInfoService
- package com.atguigu.yygh.user.service;
- import com.atguigu.yygh.model.user.UserInfo;
- import com.atguigu.yygh.vo.user.LoginVo;
- import com.atguigu.yygh.vo.user.UserAuthVo;
- import com.baomidou.mybatisplus.extension.service.IService;
- import java.util.Map;
- public interface UserInfoService extends IService<UserInfo> {
- //用户手机登录接口
- Map<String, Object> login(LoginVo loginVo);
- //根据openid判断数据库是否存在微信的扫描人信息
- UserInfo selectWxInfoOpenId(String openid);
- //用户认证接口
- void userAuth(Long userId, UserAuthVo userAuthVo);
- }
复制代码 实现类:UserInfoService Impl:
- //用户认证接口
- @Override
- public void userAuth(Long userId, UserAuthVo userAuthVo) {
- //刚开始数据库中有这条用户信息,只是信息不完善,需要先把他查询出来,在进行更新
- //根据用户id查询用户信息
- UserInfo userInfo = baseMapper.selectById(userId);
- //设置认证信息
- //认证人姓名
- userInfo.setName(userAuthVo.getName());
- //其他认证信息
- userInfo.setCertificatesType(userAuthVo.getCertificatesType());
- userInfo.setCertificatesNo(userAuthVo.getCertificatesNo());
- userInfo.setCertificatesUrl(userAuthVo.getCertificatesUrl());
- userInfo.setAuthStatus(AuthStatusEnum.AUTH_RUN.getStatus());
- //进行信息更新
- baseMapper.updateById(userInfo);
- }
复制代码 就是更新表中字段的其他信息:

在UserInfoApiController:中继续添加用去用户信息接口
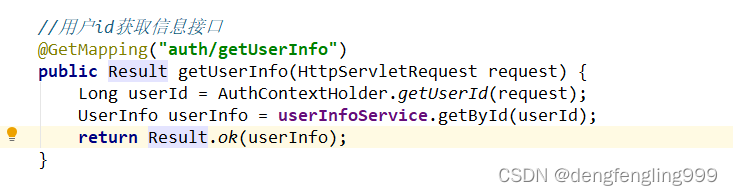
- package com.atguigu.yygh.user.controller;import com.atguigu.yygh.common.result.Result;import com.atguigu.yygh.common.utils.AuthContextHolder;import com.atguigu.yygh.model.user.UserInfo;import com.atguigu.yygh.user.service.UserInfoService;import com.atguigu.yygh.vo.user.LoginVo;import com.atguigu.yygh.vo.user.UserAuthVo;import io.swagger.annotations.ApiOperation;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.*;import javax.servlet.http.HttpServletRequest;import java.util.Map;@RestController@RequestMapping("/api/user")public class UserInfoApiController { @Autowired private UserInfoService userInfoService; //用户手机登录接口 @ApiOperation(value = "用户登录") @PostMapping("login") public Result login(@RequestBody LoginVo loginVo) { Map<String, Object> info = userInfoService.login(loginVo); return Result.ok(info); } //用户认证接口
- @PostMapping("auth/userAuth")
- public Result userAuth(@RequestBody UserAuthVo userAuthVo, HttpServletRequest request) {
- //其实就是往user_info补充添加一些认证数据进去,根据id查出记录会修改记录
- //传递两个参数,第一个参数用户id,第二个参数认证数据vo对象
- userInfoService.userAuth(AuthContextHolder.getUserId(request),userAuthVo);
- return Result.ok();
- } //用户id获取信息接口 @GetMapping("auth/getUserInfo") public Result getUserInfo(HttpServletRequest request) { Long userId = AuthContextHolder.getUserId(request); UserInfo userInfo = userInfoService.getById(userId); return Result.ok(userInfo); }}
复制代码 (5)用户认证-前端整合
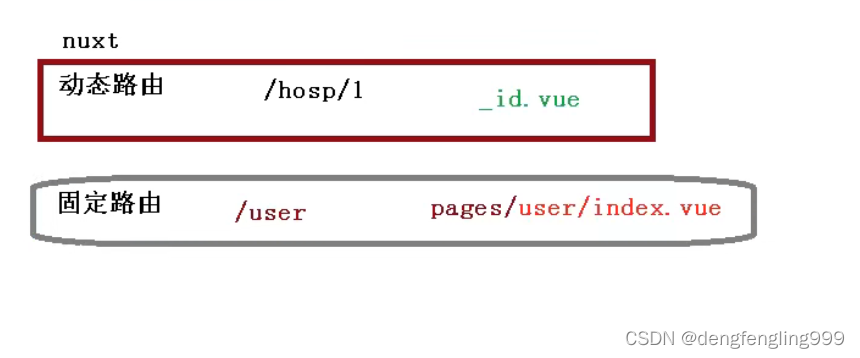
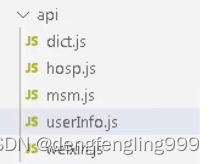
在userInfo.js中继续添加两个路径 :
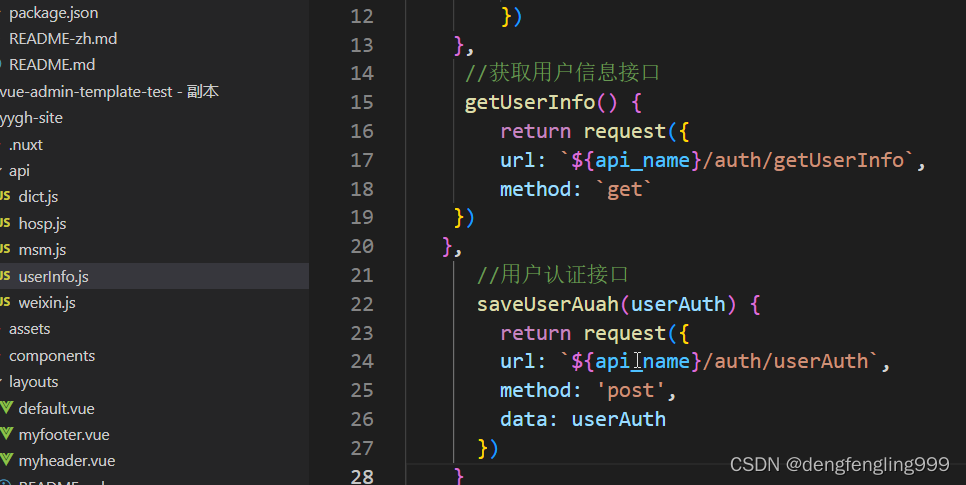
在page目录下创建
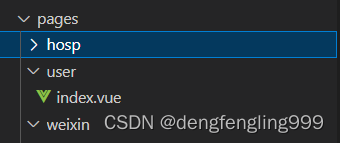
- <template>
- <!-- header -->
- <div class="nav-container page-component">
- <!--左侧导航 #start -->
- <div class="nav left-nav">
- <div class="nav-item selected">
- <span
- class="v-link selected dark"
- onclick="javascript:window.location='/user'"
- >实名认证
- </span>
- </div>
- <div class="nav-item">
- <span
- class="v-link selected dark"
- onclick="javascript:window.location='/order'"
- >
- 挂号订单
- </span>
- </div>
- <div class="nav-item">
- <span
- class="v-link clickable dark"
- onclick="javascript:window.location='/patient'"
- >
- 就诊人管理
- </span>
- </div>
- <div class="nav-item">
- <span class="v-link clickable dark"> 修改账号信息 </span>
- </div>
- <div class="nav-item">
- <span class="v-link clickable dark"> 意见反馈 </span>
- </div>
- </div>
- <!-- 左侧导航 #end -->
- <!-- 右侧内容 #start -->
- <div class="page-container">
- <div>
- <div class="title">实名认证</div>
- <div class="status-bar">
- <div class="status-wrapper">
- <span class="iconfont"></span>{{ userInfo.param.authStatusString }}
- </div>
- </div>
- <div class="tips">
- <span class="iconfont"></span>
- 完成实名认证后才能添加就诊人,正常进行挂号,为了不影响后续步骤,建议提前实名认证。
- </div>
- <div class="form-wrapper" v-if="userInfo.authStatus == 0">
- <div>
- <el-form
- :model="userAuah"
- label-width="110px"
- label-position="left"
- >
- <el-form-item prop="name" label="姓名:" class="form-normal">
- <div class="name-input">
- <el-input
- v-model="userAuah.name"
- placeholder="请输入联系人姓名全称"
- class="input v-input"
- />
- </div>
- </el-form-item>
- <el-form-item prop="certificatesType" label="证件类型:">
- <el-select
- v-model="userAuah.certificatesType"
- placeholder="请选择证件类型"
- class="v-select patient-select"
- >
- <el-option
- v-for="item in certificatesTypeList"
- :key="item.value"
- :label="item.name"
- :value="item.name"
- >
- </el-option>
- </el-select>
- </el-form-item>
- <el-form-item prop="certificatesNo" label="证件号码:">
- <el-input
- v-model="userAuah.certificatesNo"
- placeholder="请输入联系人证件号码"
- class="input v-input"
- />
- </el-form-item>
- <el-form-item prop="name" label="上传证件:">
- <div class="upload-wrapper">
- <div class="avatar-uploader">
- <el-upload
- class="avatar-uploader"
- :action="fileUrl"
- :show-file-list="false"
- :on-success="onUploadSuccess"
- >
- <div class="upload-inner-wrapper">
- <img
- v-if="userAuah.certificatesUrl"
- :src="userAuah.certificatesUrl"
- class="avatar"
- />
- <i v-else class="el-icon-plus avatar-uploader-icon"></i>
- <div v-if="!userAuah.certificatesUrl" class="text">
- 上传证件合照
- </div>
- </div>
- </el-upload>
- </div>
- <img
- src="//img.114yygh.com/static/web/auth_example.png"
- class="example"
- />
- </div>
- </el-form-item>
- </el-form>
- <div class="bottom-wrapper">
- <div class="button-wrapper">
- <div class="v-button" @click="saveUserAuah()">
- {{ submitBnt }}
- </div>
- </div>
- </div>
- </div>
- </div>
- <div class="context-container" v-if="userInfo.authStatus != 0">
- <div>
- <el-form
- :model="formData"
- label-width="110px"
- label-position="right"
- >
- <el-form-item prop="name" label="姓名:" class="form-normal">
- <div class="name-input">
- {{ userInfo.name }}
- </div>
- </el-form-item>
- <el-form-item prop="name" label="证件类型:">
- {{ userInfo.certificatesType }}
- </el-form-item>
- <el-form-item prop="name" label="证件号码:">
- {{ userInfo.certificatesNo }}
- </el-form-item>
- </el-form>
- </div>
- </div>
- </div>
- </div>
- <!-- 右侧内容 #end -->
- <!-- 登录弹出框 -->
- </div>
- <!-- footer -->
- </template>
-
- <script>
- import "~/assets/css/hospital_personal.css";
- import "~/assets/css/hospital.css";
- import "~/assets/css/personal.css";
- import dictApi from "@/api/dict";
- import userInfoApi from "@/api/userInfo";
- const defaultForm = {
- name: "",
- certificatesType: "", //证件类型
- certificatesNo: "", //证件编号
- certificatesUrl: "", //
- };
- export default {
- data() {
- return {
- userAuah: defaultForm,
- certificatesTypeList: [],
- fileUrl: "http://localhost/api/oss/file/fileUpload",
- userInfo: {
- param: {},
- },
- submitBnt: "提交",
- };
- },
- created() {
- this.init();
- },
- methods: {
- init() {
- this.getUserInfo(); //得到用户信息
- this.getDict(); //得到字典信息
- },
- //得到用户信息
- getUserInfo() {
- userInfoApi.getUserInfo().then((response) => {
- this.userInfo = response.data;
- });
- },
- //认证操作方法
- saveUserAuah() {
- if (this.submitBnt == "正在提交...") {
- this.$message.info("重复提交");
- return;
- }
- this.submitBnt = "正在提交...";
- userInfoApi
- .saveUserAuah(this.userAuah)
- .then((response) => {
- this.$message.success("提交成功");
- window.location.reload();
- })
- .catch((e) => {
- this.submitBnt = "提交";
- });
- },
- getDict() {
- dictApi.findByDictCode("CertificatesType").then((response) => {
- this.certificatesTypeList = response.data;
- });
- },
- onUploadSuccess(response, file) {
- if (response.code !== 200) {
- this.$message.error("上传失败");
- return;
- }
- // 填充上传文件列表
- this.userAuah.certificatesUrl = file.response.data;
- },
- },
- };
- </script>
- <style>
- .header-wrapper .title {
- font-size: 16px;
- margin-top: 0;
- }
- .content-wrapper {
- margin-left: 0;
- }
- .patient-card .el-card__header .detail {
- font-size: 14px;
- }
- .page-container .title {
- letter-spacing: 1px;
- font-weight: 700;
- color: #333;
- font-size: 16px;
- margin-top: 0;
- margin-bottom: 20px;
- }
- .page-container .tips {
- width: 100%;
- padding-left: 0;
- }
- .page-container .form-wrapper {
- padding-left: 92px;
- width: 580px;
- }
- .form-normal {
- height: 40px;
- }
- .bottom-wrapper {
- width: 100%;
- padding: 0;
- margin-top: 0;
- }
- </style>
复制代码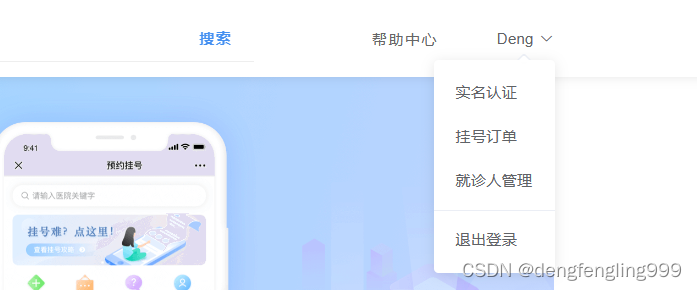
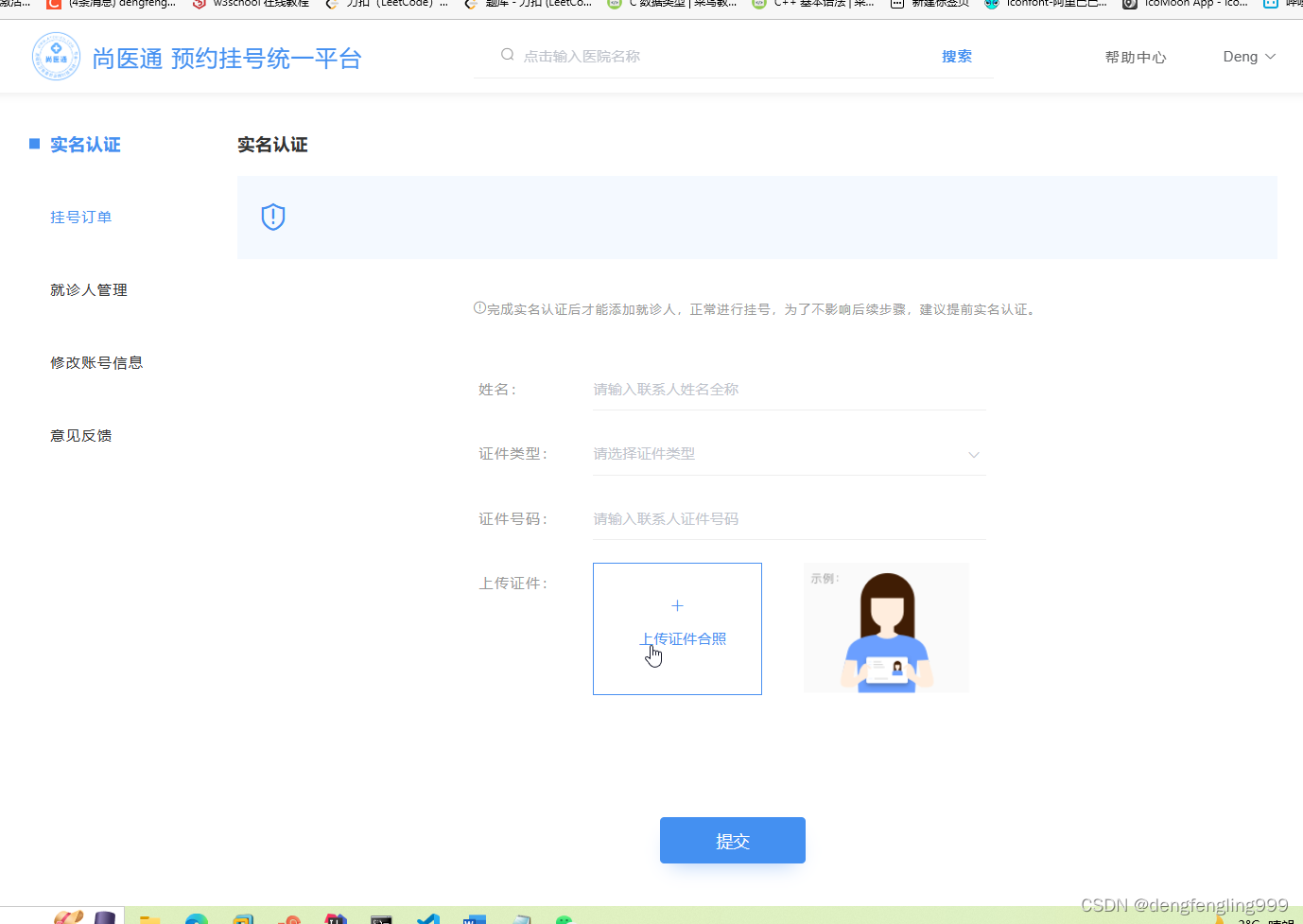
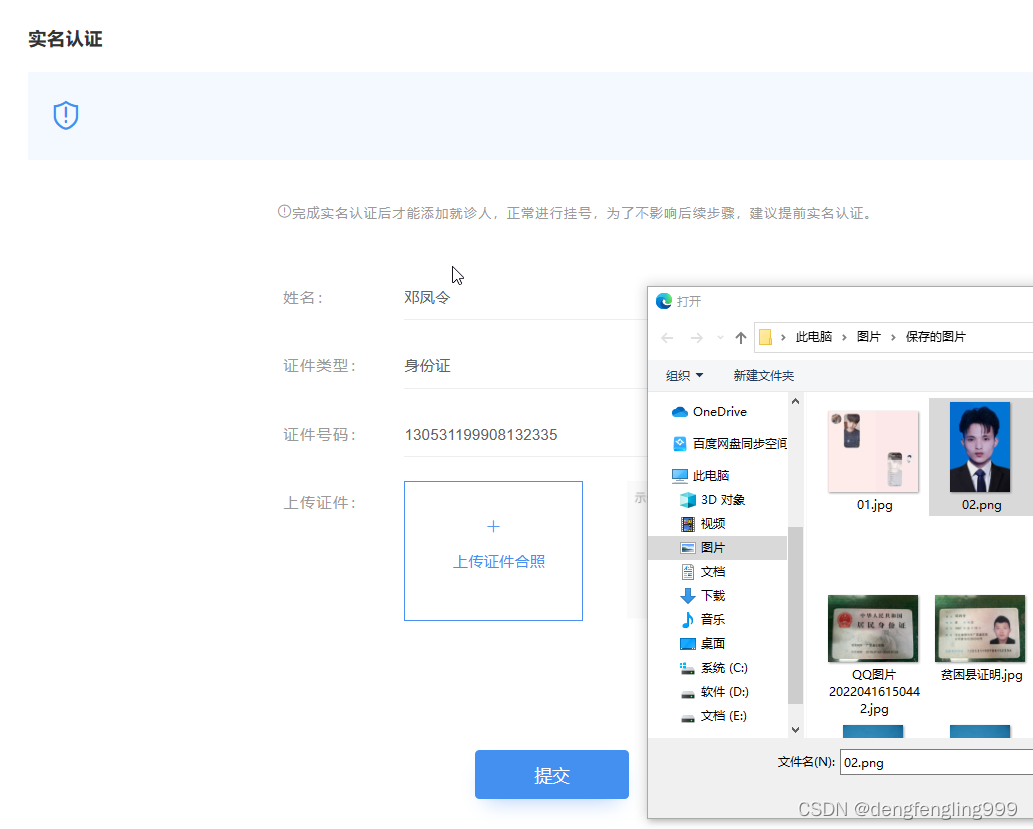
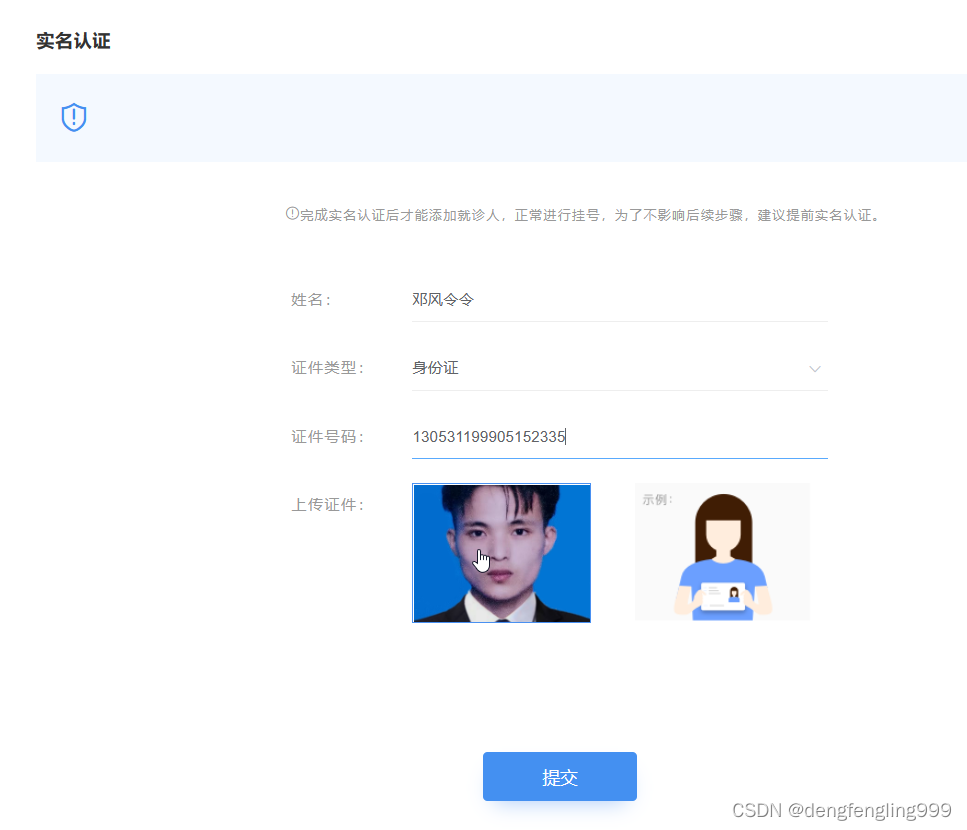
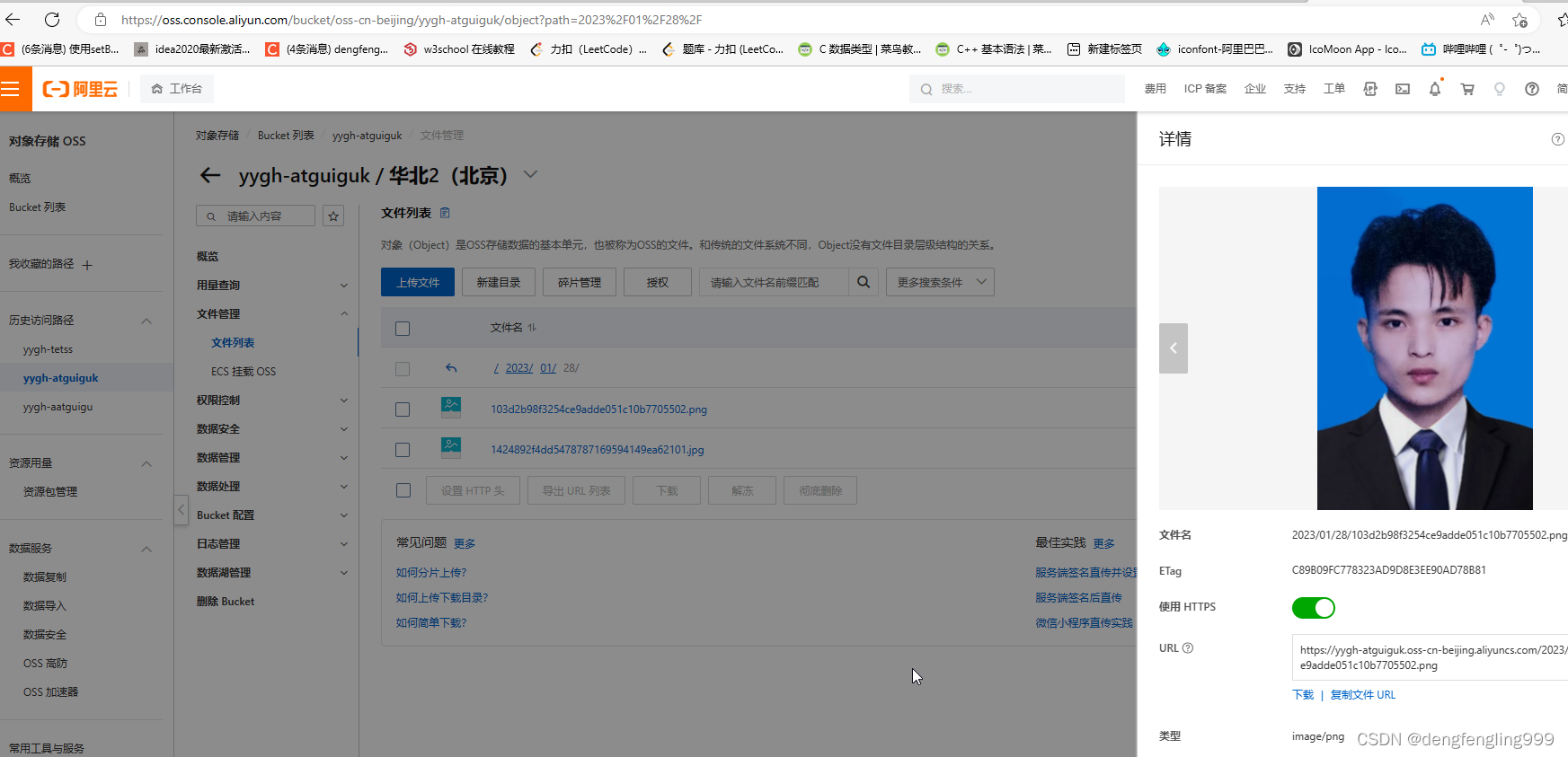
点击提交:
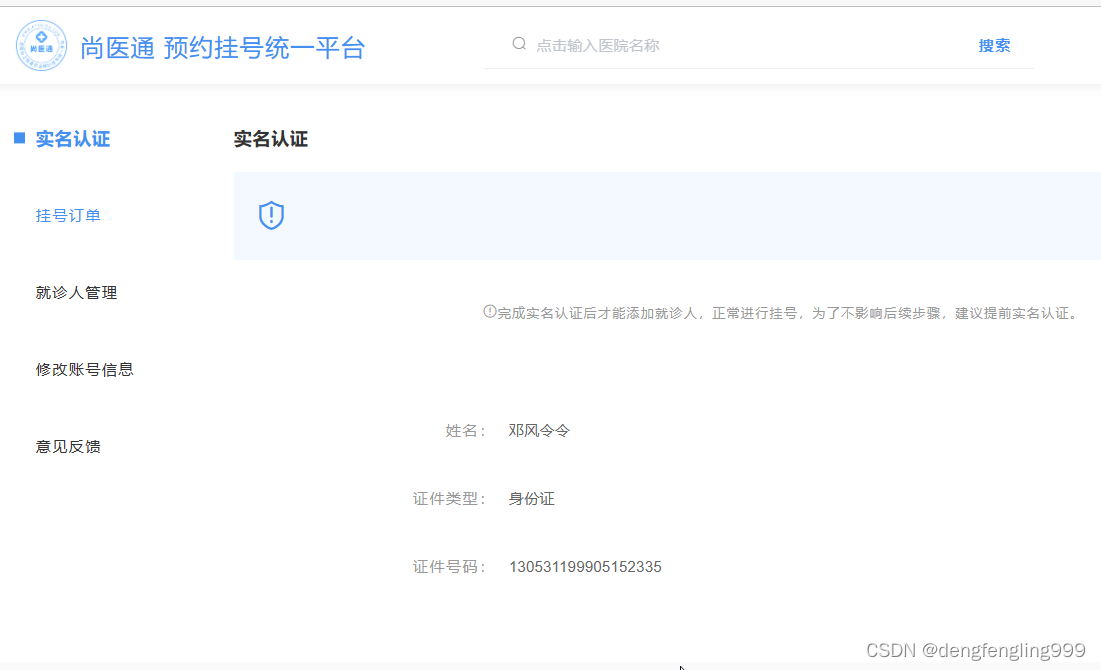
数据库中:user_info:中的登录的用户数据乐成进行了认证,数据进行了更新

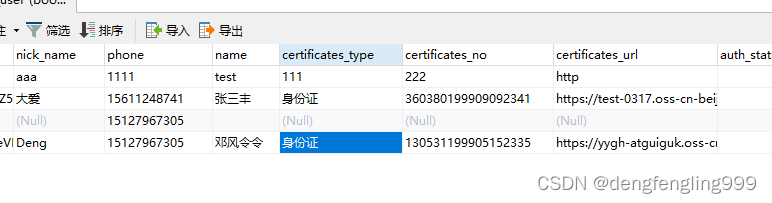
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |