1、在idea中配置database 连接数据库,用来在idea中编写sql脚本操作数据库
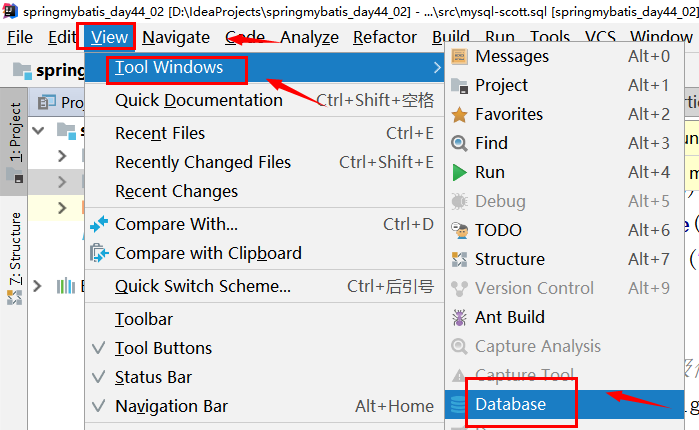
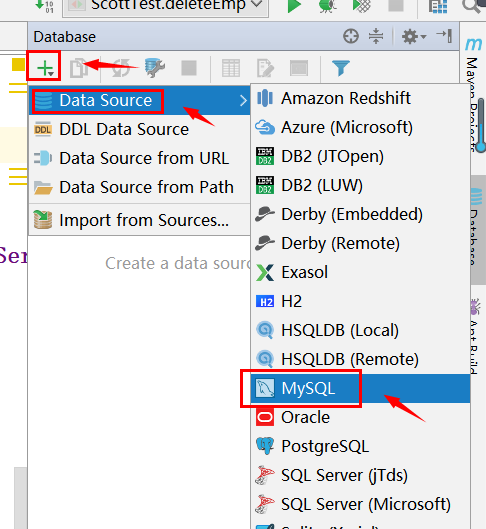
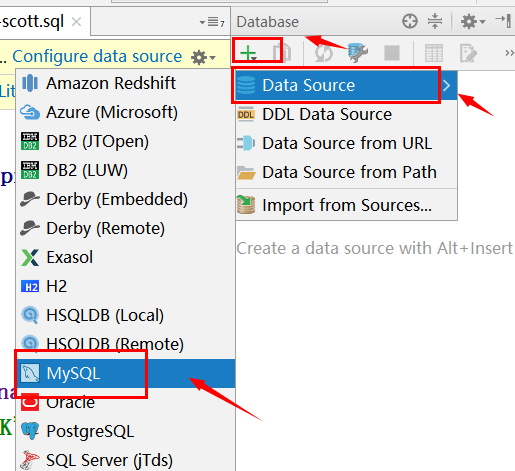 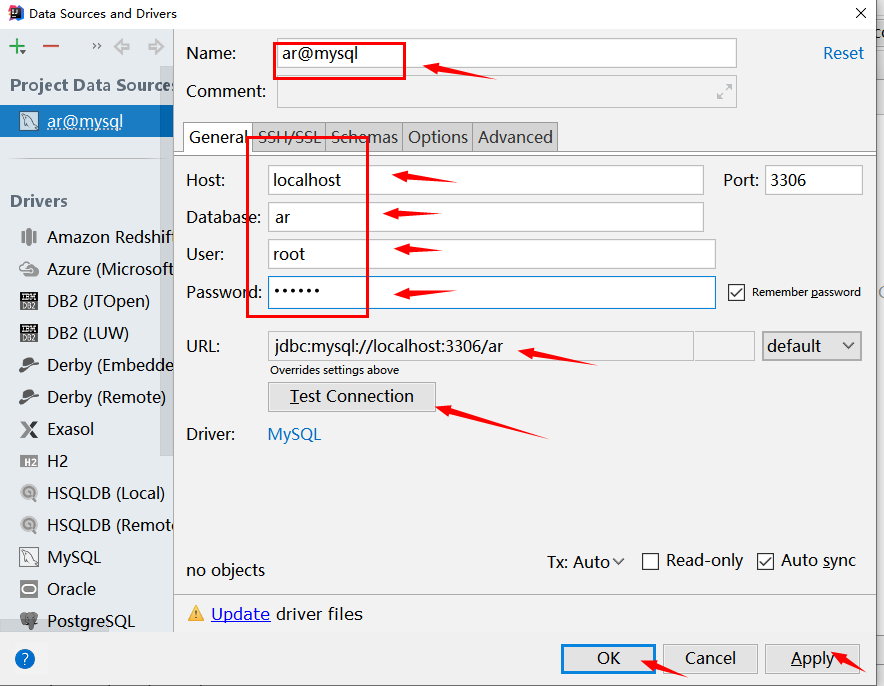
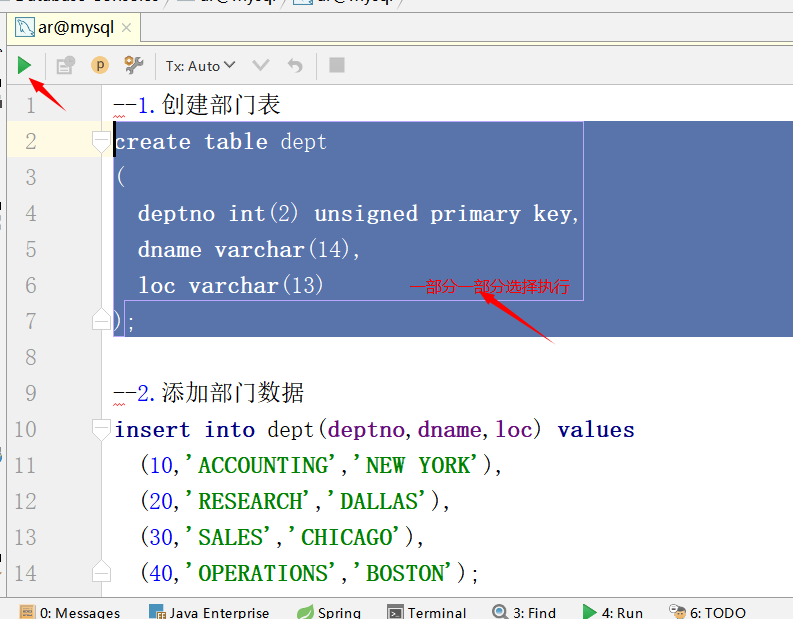
2、sql详细脚本如下:
  - 1 --1.创建部门表
- 2 create table dept
- 3 (
- 4 deptno int(2) unsigned primary key,
- 5 dname varchar(14),
- 6 loc varchar(13)
- 7 );
- 8
- 9 --2.添加部门数据
- 10 insert into dept(deptno,dname,loc) values
- 11 (10,'ACCOUNTING','NEW YORK'),
- 12 (20,'RESEARCH','DALLAS'),
- 13 (30,'SALES','CHICAGO'),
- 14 (40,'OPERATIONS','BOSTON');
- 15
- 16 --3.创建员工表
- 17 create table emp
- 18 (
- 19 empno int(4) unsigned primary key,
- 20 ename varchar(10),
- 21 job varchar(9),
- 22 mgr int(4),
- 23 hiredate date,
- 24 sal double(7,2),
- 25 comm double(7,2),
- 26 deptno int(2) references dept(deptno)
- 27 );
- 28
- 29 --4.插入数据员工数据
- 30 insert into emp(empno,ename,job,mgr,hiredate,sal,comm,deptno) values
- 31 (7369,'SMITH','CLERK',7902,'1980-12-17',800,null,20),
- 32 (7499,'ALLEN','SALESMAN',7698,'1981-02-20',1600,300,30),
- 33 (7521,'WARD','SALESMAN',7698,'1981-02-22',1250,500,30),
- 34 (7566,'JONES','MANAGER',7839,'1981-04-02',2975,null,20),
- 35 (7654,'MARTIN','SALESMAN',7698,'1981-09-28',1250,1400,30),
- 36 (7698,'BLAKE','MANAGER',7839,'1981-05-01',2850,null,30),
- 37 (7782,'BLAKE','MANAGER',7839,'1981-06-09',2450,null,10),
- 38 (7788,'SCOTT','ANALYST',7566,'1987-04-19',3000,null,20),
- 39 (7839,'KING','PRESIDENT',null,'1981-11-17',5000,null,10),
- 40 (7844,'TURNER','SALESMAN',7698,'1981-09-08',1500,0,30),
- 41 (7876,'ADAMS','CLERK',7788,'1987-05-23',1100,null,20),
- 42 (7900,'JAMES','CLERK',7698,'1981-12-03',950,null,30),
- 43 (7902,'FORD','ANALYST',7566,'1981-12-03',3000,null,20),
- 44 (7934,'MILLER','CLERK',7782,'1982-01-23',1300,null,10);
- 45
- 46
- 47 --3.员工福利表
- 48 create table bonus
- 49 (
- 50 ename varchar(10),
- 51 job varchar(9),
- 52 sal double(7,2),
- 53 comm double(7,2)
- 54 );
- 55
- 56 --4.工资等级表
- 57 create table salgrade
- 58 (
- 59 grade int(10),
- 60 losal double(7,2),
- 61 hisal double(7,2)
- 62 );
- 63
- 64 --5.插入工资等级信息
- 65 insert into salgrade(grade,losal,hisal) values
- 66 (1,700,1200),
- 67 (2,1201,1400),
- 68 (3,1401,2000),
- 69 (4,2001,3000),
- 70 (5,3001,9999);
复制代码 scott-mysql.sql3、创建项目,项目结构如下:
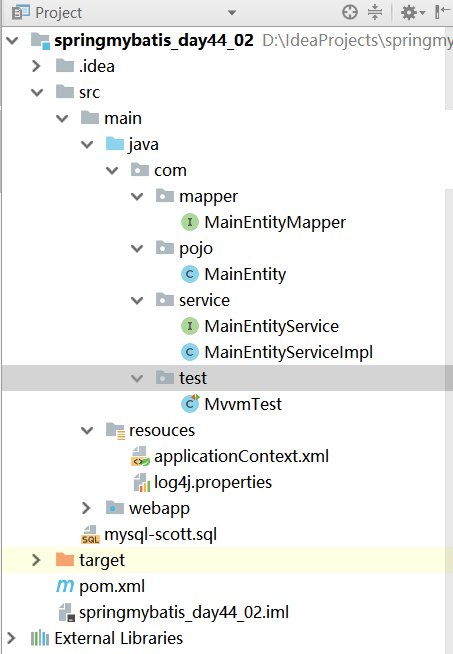
4、在pojo包下创建MainEntity.java实体类
  - 1 package com.pojo;
- 2 /**
- 3 * 承接首页数据的持久化类
- 4 * 用来接受sql查询的结果
- 5 * 且将数据传输到首页显示
- 6 */
- 7 public class MainEntity {
- 8 //因为多方外键列的值和一方主键列的值是一样的,如果有需要则显示多方外键列字段,不写一方主键列
- 9 //先写多方字段
- 10 private Integer empno;
- 11 private String ename;
- 12 private String job;
- 13 private Double sal;
- 14 private Integer deptno;
- 15
- 16 private Double maxsal;
- 17 private Double minsal;
- 18 //再写一方字段
- 19 private String dname;
- 20
- 21 public MainEntity() {
- 22 }
- 23
- 24 public MainEntity(Integer empno, String ename, String job, Double sal, Integer deptno, String dname) {
- 25 this.empno = empno;
- 26 this.ename = ename;
- 27 this.job = job;
- 28 this.sal = sal;
- 29 this.deptno = deptno;
- 30 this.dname = dname;
- 31 }
- 32
- 33 public MainEntity(Integer empno, String ename, String job, Double sal, Integer deptno, String dname, Double minsal,Double maxsal) {
- 34 this.empno = empno;
- 35 this.ename = ename;
- 36 this.job = job;
- 37 this.sal = sal;
- 38 this.deptno = deptno;
- 39 this.maxsal = maxsal;
- 40 this.minsal = minsal;
- 41 this.dname = dname;
- 42 }
- 43
- 44 public Integer getEmpno() {
- 45 return empno;
- 46 }
- 47
- 48 public void setEmpno(Integer empno) {
- 49 this.empno = empno;
- 50 }
- 51
- 52 public String getEname() {
- 53 return ename;
- 54 }
- 55
- 56 public void setEname(String ename) {
- 57 this.ename = ename;
- 58 }
- 59
- 60 public String getJob() {
- 61 return job;
- 62 }
- 63
- 64 public void setJob(String job) {
- 65 this.job = job;
- 66 }
- 67
- 68 public Double getSal() {
- 69 return sal;
- 70 }
- 71
- 72 public void setSal(Double sal) {
- 73 this.sal = sal;
- 74 }
- 75
- 76 public Integer getDeptno() {
- 77 return deptno;
- 78 }
- 79
- 80 public void setDeptno(Integer deptno) {
- 81 this.deptno = deptno;
- 82 }
- 83
- 84 public String getDname() {
- 85 return dname;
- 86 }
- 87
- 88 public void setDname(String dname) {
- 89 this.dname = dname;
- 90 }
- 91
- 92 public Double getMaxsal() {
- 93 return maxsal;
- 94 }
- 95
- 96 public void setMaxsal(Double maxsal) {
- 97 this.maxsal = maxsal;
- 98 }
- 99
- 100 public Double getMinsal() {
- 101 return minsal;
- 102 }
- 103
- 104 public void setMinsal(Double minsal) {
- 105 this.minsal = minsal;
- 106 }
- 107
- 108 @Override
- 109 public String toString() {
- 110 return "MainEntity{" +
- 111 "empno=" + empno +
- 112 ", ename='" + ename + '\'' +
- 113 ", job='" + job + '\'' +
- 114 ", sal=" + sal +
- 115 ", deptno=" + deptno +
- 116 ", maxsal=" + maxsal +
- 117 ", minsal=" + minsal +
- 118 ", dname='" + dname + '\'' +
- 119 '}';
- 120 }
- 121 }
复制代码 MainEntity.java5、在mapper包下创建MainEntityMapper.java映射接口
  - 1 package com.mapper;
- 2
- 3 import com.pojo.MainEntity;
- 4 import org.apache.ibatis.annotations.Delete;
- 5 import org.apache.ibatis.annotations.Insert;
- 6 import org.apache.ibatis.annotations.Select;
- 7 import org.apache.ibatis.annotations.Update;
- 8
- 9 import java.util.List;
- 10
- 11 public interface MainEntityMapper {
- 12 // mybatis注解的动态sql的要写在script标签内,且开始标签前不能有空格
- 13 @Select("")
- 29 public List<MainEntity> selectData(MainEntity mainEntity);
- 30
- 31
- 32 @Select("select d.dname,e.empno,e.ename,e.job,e.sal,e.deptno from emp e,dept d where e.deptno=d.deptno")
- 33 public List<MainEntity> selectMainData();
- 34
- 35 @Select("select d.dname,e.empno,e.ename,e.job,e.sal,e.deptno from emp e,dept d where e.deptno=d.deptno and e.empno=#{primarykey}")
- 36 public MainEntity selectByInfoData(Integer primarykey);
- 37
- 38 @Insert("insert into emp(empno,ename,job,sal,deptno) values(#{empno},#{ename},#{job},#{sal},#{deptno})")
- 39 public int insertData(MainEntity mainEntity);
- 40
- 41 @Update("update emp set ename=#{ename},job=#{job},sal=#{sal},deptno=#{deptno} where empno=#{empno}")
- 42 public int updateData(MainEntity mainEntity);
- 43
- 44 @Delete("delete from emp where empno=#{primarykey}")
- 45 public int deleteData(Integer primarykey);
- 46
- 47 }
复制代码 MainEntityMapper.java6、在service包下创建MainEntityService.java业务层接口
  - 1 package com.service;
- 2
- 3 import com.pojo.MainEntity;
- 4 import org.apache.ibatis.annotations.Delete;
- 5 import org.apache.ibatis.annotations.Insert;
- 6 import org.apache.ibatis.annotations.Select;
- 7 import org.apache.ibatis.annotations.Update;
- 8
- 9 import java.util.List;
- 10
- 11 public interface MainEntityService {
- 12
- 13 public List<MainEntity> show(MainEntity mainEntity);
- 14
- 15 public int add(MainEntity mainEntity);
- 16
- 17 public int edit(MainEntity mainEntity);
- 18
- 19 public int del(Integer primarykey);
- 20
- 21 }
复制代码 MainEntityService.java7、在service包下创建MainEntityServiceImpl.java业务层接口实现类
  - 1 package com.service;
- 2
- 3 import com.mapper.MainEntityMapper;
- 4 import com.pojo.MainEntity;
- 5
- 6 import java.util.List;
- 7
- 8 public class MainEntityServiceImpl implements MainEntityService {
- 9 private MainEntityMapper mapper;
- 10
- 11 public MainEntityMapper getMapper() {
- 12 return mapper;
- 13 }
- 14
- 15 public void setMapper(MainEntityMapper mapper) {
- 16 this.mapper = mapper;
- 17 }
- 18
- 19 @Override
- 20 public List<MainEntity> show(MainEntity mainEntity) {
- 21 return mapper.selectData(mainEntity);
- 22 }
- 23
- 24 @Override
- 25 public int add(MainEntity mainEntity) {
- 26 return mapper.insertData(mainEntity);
- 27 }
- 28
- 29 @Override
- 30 public int edit(MainEntity mainEntity) {
- 31 return mapper.updateData(mainEntity);
- 32 }
- 33
- 34 @Override
- 35 public int del(Integer primarykey) {
- 36 return mapper.deleteData(primarykey);
- 37 }
- 38 }
复制代码 MainEntityServiceImpl .java8、在resouces下创建日志记录文件log4j.properties文件
  - 1 log4j.rootLogger=DEBUG, Console
- 2 #Console
- 3 log4j.appender.Console=org.apache.log4j.ConsoleAppender
- 4 log4j.appender.Console.layout=org.apache.log4j.PatternLayout
- 5 log4j.appender.Console.layout.ConversionPattern=%d [%t] %-5p [%c] - %m%n
- 6
- 7 log4j.logger.org.apache=INFO
- 8 log4j.logger.java.sql.ResultSet=INFO
- 9 log4j.logger.java.sql.Connection=DEBUG
- 10 log4j.logger.java.sql.Statement=DEBUG
- 11 log4j.logger.java.sql.PreparedStatement=DEBUG
复制代码 log4j.properties9、在resouces下创建spring的applicationContext.xml文件
  - 1 <?xml version="1.0" encoding="UTF-8"?>
- 2 <beans xmlns="http://www.springframework.org/schema/beans"
- 3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- 4 xmlns:aop="http://www.springframework.org/schema/aop"
- 5 xmlns:context="http://www.springframework.org/schema/context"
- 6 xmlns:tx="http://www.springframework.org/schema/tx"
- 7 xmlns:mvc="http://www.springframework.org/schema/mvc"
- 8 xsi:schemaLocation="http://www.springframework.org/schema/beans
- 9 http://www.springframework.org/schema/beans/spring-beans.xsd
- 10 http://www.springframework.org/schema/aop
- 11 http://www.springframework.org/schema/aop/spring-aop.xsd
- 12 http://www.springframework.org/schema/context
- 13 http://www.springframework.org/schema/context/spring-context.xsd
- 14 http://www.springframework.org/schema/tx
- 15 http://www.springframework.org/schema/tx/spring-tx.xsd
- 16 http://www.springframework.org/schema/mvc
- 17 http://www.springframework.org/schema/mvc/spring-mvc.xsd
- 18 ">
- 19
- 20 <bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
- 21 <property name="driverClassName" value="com.mysql.jdbc.Driver"/>
- 22 <property name="url" value="jdbc:mysql://localhost:3306/ar"/>
- 23 <property name="username" value="root"/>
- 24 <property name="password" value="123456"/>
- 25 </bean>
- 26
- 27 <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
- 28 <property name="dataSource" ref="dataSource"/>
- 29 </bean>
- 30
- 31 <tx:annotation-driven transaction-manager="transactionManager"/>
- 32
- 33 <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
- 34 <property name="dataSource" ref="dataSource"/>
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43 </bean>
- 44
- 45
- 46
- 47
- 48 <bean id="sqlSessionTemplate" class="org.mybatis.spring.SqlSessionTemplate">
- 49 <constructor-arg ref="sqlSessionFactory"/>
- 50 </bean>
- 51
- 52
- 53 <bean id="mainEntityMapper" class="org.mybatis.spring.mapper.MapperFactoryBean">
- 54 <property name="sqlSessionTemplate" ref="sqlSessionTemplate"/>
- 55 <property name="mapperInterface" value="com.mapper.MainEntityMapper"/>
- 56 </bean>
- 57
- 58
- 59 <bean id="mainEntityService" class="com.service.MainEntityServiceImpl">
- 60 <property name="mapper" ref="mainEntityMapper"/>
- 61 </bean>
- 62 </beans>
复制代码 applicationContext.xml10、在test包下创建MvvmTest.java测试类
  - 1 package com.test;
- 2
- 3 import com.pojo.MainEntity;
- 4 import com.service.MainEntityService;
- 5 import org.junit.Test;
- 6 import org.springframework.context.ApplicationContext;
- 7 import org.springframework.context.support.ClassPathXmlApplicationContext;
- 8
- 9 import java.util.List;
- 10
- 11 public class MvvmTest {
- 12 //加载spring的xml文件
- 13 ApplicationContext ac=new ClassPathXmlApplicationContext("applicationContext.xml");
- 14 //在spring的xml文件中获取service的bean
- 15 MainEntityService service=(MainEntityService)ac.getBean("mainEntityService");
- 16 // 测试查询所有
- 17 @Test
- 18 public void selectAll(){
- 19 List<MainEntity> list= service.show(new MainEntity());
- 20 for (MainEntity m:list) {
- 21 System.out.println(m);
- 22 }
- 23 }
- 24
- 25 @Test
- 26 public void selectData(){
- 27 MainEntity entity=new MainEntity();
- 28 // entity.setEmpno(7788);
- 29 // entity.setEname("SCOTT");
- 30 // entity.setJob("ANALYST");
- 31 // entity.setDeptno(10);
- 32 entity.setMinsal(800.00);
- 33 entity.setMaxsal(1300.00);
- 34
- 35 List<MainEntity> list= service.show(entity);
- 36 for (MainEntity m:list) {
- 37 System.out.println(m);
- 38 }
- 39 }
- 40
- 41
- 42
- 43 @Test
- 44 public void insertData(){
- 45 MainEntity entity=new MainEntity(1,"holly","教学",12.00,10,"xxx");
- 46 System.out.println(service.add(entity)>0?"add success":"add fail");
- 47 }
- 48 @Test
- 49 public void updateData(){
- 50 MainEntity entity=new MainEntity(1,"holly","教学",16.00,10,"xxx");
- 51 System.out.println(service.edit(entity)>0?"update success":"update fail");
- 52 }
- 53 @Test
- 54 public void deleteData(){
- 55 System.out.println(service.del(1)>0?"delete success":"delete fail");
- 56 }
- 57
- 58
- 59 }
复制代码 MvvmTest .java11、运行其中一个?
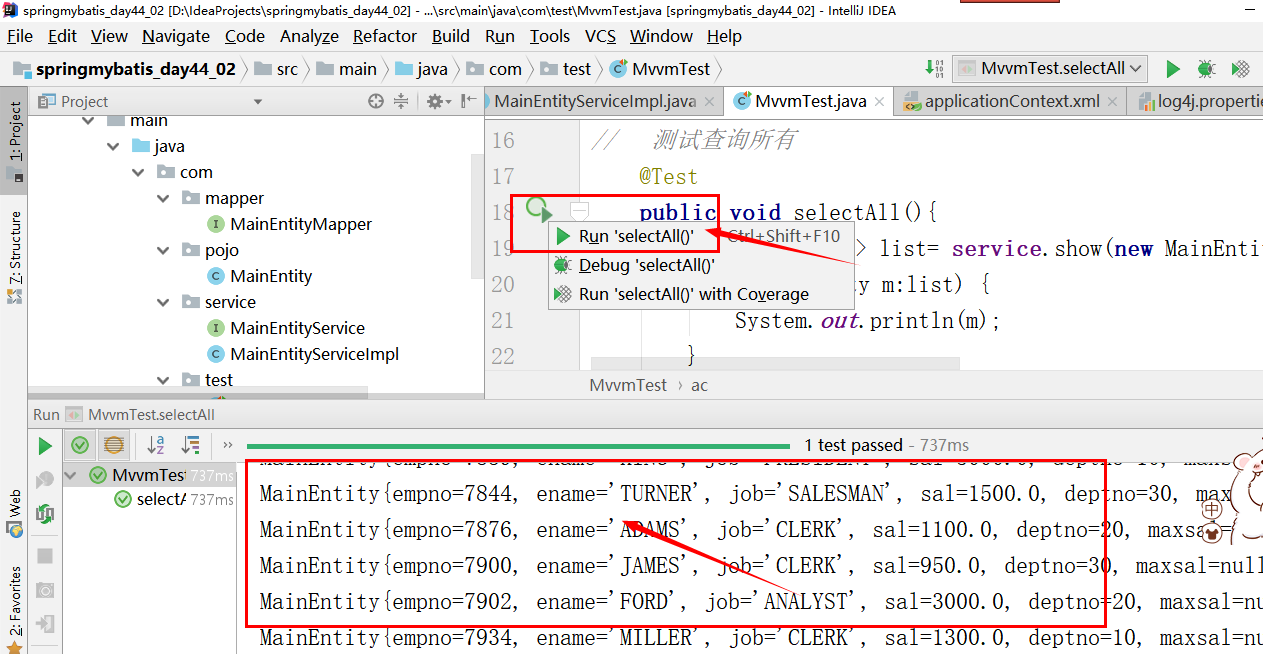
此文章为原创,转载请注明出处!需要本案例源码,理论讲解视频,代码操作视频的,请私信联系作者!
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |