权限类
主要用途:用户登录了,某个接口可能只有超级管理员才能访问,普通用户不能访问
案列:出版社的所有接口,必须登录,而且是超级管理员才能访问
分析步骤- 第一步:写一个类,继承BasePermission
- 第二步:重写has_permission方法
- 第三步:在方法校验用户时候有权限(request.user就是当前登录用户)
- 第四步:如果有权限,返回True,没有权限,返回FALSE
- 第五步:self.message 是给前端的提示信息
- 第六步:局部使用,全局使用,局部禁用
复制代码 model.py- class User(models.Model):
- username = models.CharField(max_length=32)
- password = models.CharField(max_length=32)
- user_type = models.IntegerField(default=3, choices=((1, '超级管理员'), (2, '普通用户'), (3, '2b用户')))
- def __str__(self):
- return self.username
复制代码 permission.py- from rest_framework.permissions import BasePermission
- class UserTypePermission(BasePermission):
- def has_permission(self, request, view):
- # 只有超级管理员有权限
- if request.user.user_type == 1:
- return True # 有权限
- else:
- """
- self.message = '普通用户和2b用户都没有权限'
- self.message = '您是:%s 用户,您没有权限'%request.user.get_user_type_display()
- """
- return False # 没有权限
- """
- self.message = '普通用户和2b用户都没有权限'
- 返回给前端的提示是什么样
- self.message = '您是:%s 用户,您没有权限'%request.user.get_user_type_display()
- 使用了choice后,user.user_type 拿到的是数字类型,想变成字符串 user.get_user_type_display()
- """
复制代码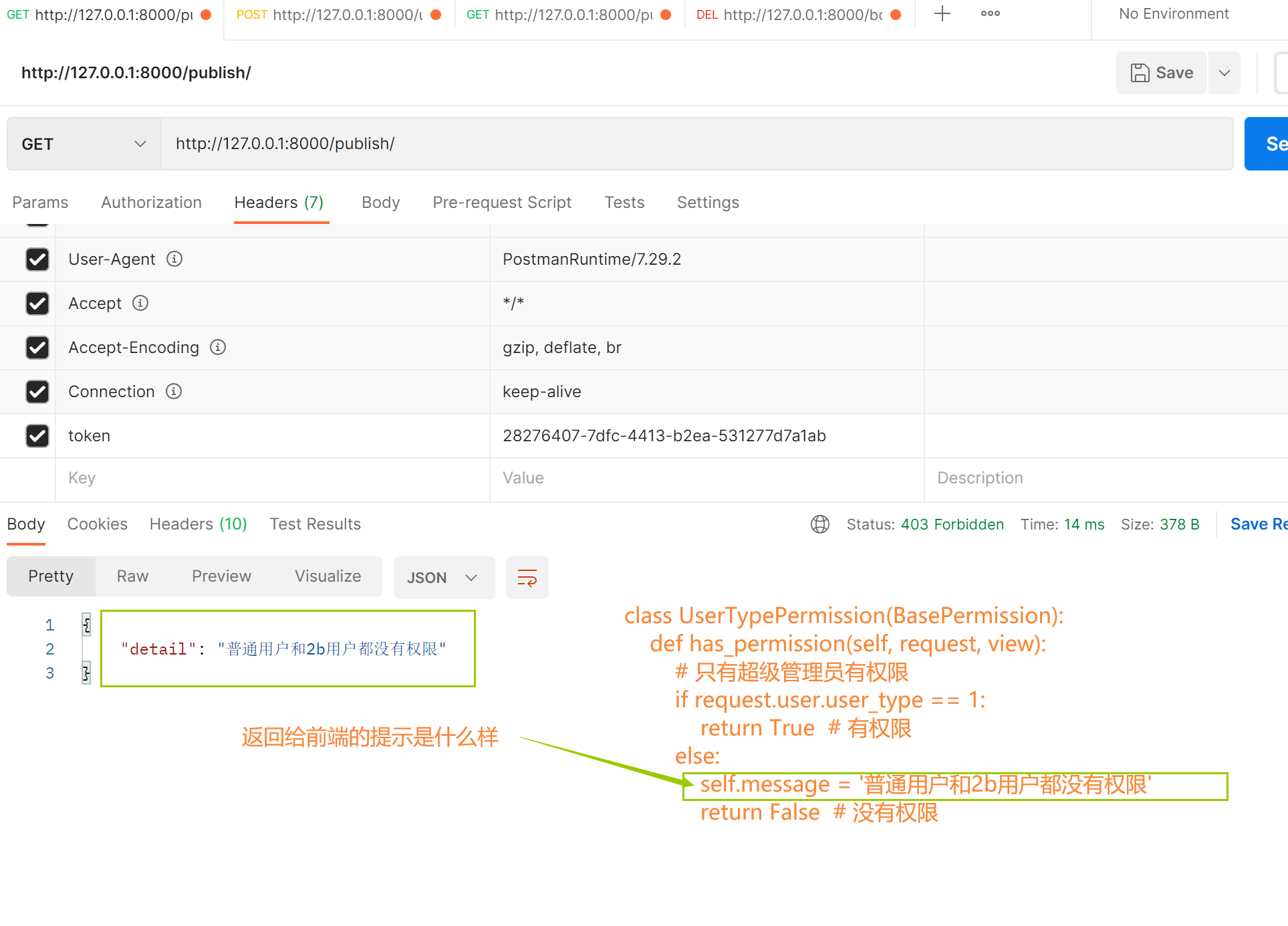
view.py- # 导入我们所写的那个权限文件
- from .permission import UserTypePermission
- # 要验证必须要登录,下面的这种方式是局部权限使用
- class PublishView(ViewSetMixin, ListCreateAPIView):
- # 登录验证
- authentication_classes = [LoginAuth, ]
- # 权限验证
- permission_classes = [UserTypePermission, ]
- queryset = Publish.objects.all()
- serializer_class = PublishSerializer
- class PublishDetailView(ViewSetMixin, RetrieveUpdateDestroyAPIView):
- # 登录验证
- authentication_classes = [LoginAuth, ]
- # 权限验证
- permission_classes = [UserTypePermission, ]
- queryset = Publish.objects.all()
- serializer_class = PublishSerializer
复制代码 settings.py- 全局权限验证:(要在setting.py文件中配置)
- 全局验证需要注意的是在登录的时候需要添加局部禁用
- permission_classes = []
- authentication_classes = []
- REST_FRAMEWORK = {
- # 'DEFAULT_AUTHENTICATION_CLASSES': ['app01.auth.LoginAuth', ]
- 'DEFAULT_PERMISSION_CLASSES': ['app01.permission.UserTypePermission', ]
- }
- # 全局的加上以后局部的就可以注释掉了
复制代码 频率类
认证,权限都通过以后,我们可以限制某个接口的访问频率,防止有人恶意攻击网站,一般根据ip或者用户限制
自定义频率类
案例:无论是否登录和是否有权限,都要限制访问的频率,比如一分钟访问3次
分析步骤:- 第一步:写一个类:继承SimpleRateThrottle
- 第二步:重写get_cache_key,返回唯一的字符串,会以这个字符串做频率限制
- 第三步:写一个类属性scope=‘随便写’,必须要跟配置文件对象
- 第四步:配置文件中写
- 'DEFAULT_THROTTLE_RATES': {
- '随意写': '3/m' # 3/h 3/s 3/d
- }
- 第五步:局部配置,全局配置,局部禁用
复制代码 throttling.py(SimpleRateThrottle)- from rest_framework.throttling import BaseThrottle, SimpleRateThrottle
- # 我们继承SimpleRateThrottle去写,而不是继承BaseThrottle去写
- class TimeThrottling(SimpleRateThrottle):
- # 类属性,这个类属性可以随意命名,但要跟配置文件对应
- scope = 'throttling'
-
- def get_cache_key(self, request, view):
- """
- # 返回什么,频率就以什么做限制
- # 可以通过用户id限制
- # 可以通过ip地址限制
- """
- return request.META.get('REMOTE_ADDR')
复制代码 局部配置- class PublishView(ViewSetMixin, ListCreateAPIView):
- authentication_classes = [LoginAuth, ]
- # permission_classes = [UserTypePermission, ]
- # 局部频率验证,每分钟只能访问五次
- throttle_classes = [TimeThrottling, ]
- queryset = Publish.objects.all()
- serializer_class = PublishSerializer
- class PublishDetailView(ViewSetMixin, RetrieveUpdateDestroyAPIView):
- authentication_classes = [LoginAuth, ]
- # permission_classes = [UserTypePermission, ]
- # 局部频率验证,每分钟只能访问五次
- throttle_classes = [TimeThrottling, ]
- queryset = Publish.objects.all()
- serializer_class = PublishSerializer
复制代码 全局配置- REST_FRAMEWORK = {
- 'DEFAULT_THROTTLE_CLASSES': ['app01.throttling.TimeThrottling', ],
- 'DEFAULT_THROTTLE_RATES': {
- 'throttling': '5/m' # 一分钟访问5次
- }
- }
复制代码 内置频率类
限制未登录用户- # 全局使用 限制未登录用户1分钟访问5次
- REST_FRAMEWORK = {
- 'DEFAULT_THROTTLE_CLASSES': (
- 'rest_framework.throttling.AnonRateThrottle',
- ),
- 'DEFAULT_THROTTLE_RATES': {
- 'anon': '3/m',
- }
- }
- #views.py
- from rest_framework.permissions import IsAdminUser
- from rest_framework.authentication import SessionAuthentication,BasicAuthentication
- class TestView4(APIView):
- authentication_classes=[]
- permission_classes = []
- def get(self,request,*args,**kwargs):
- return Response('我是未登录用户')
- # 局部使用
- from rest_framework.permissions import IsAdminUser
- from rest_framework.authentication import SessionAuthentication,BasicAuthentication
- from rest_framework.throttling import AnonRateThrottle
- class TestView5(APIView):
- authentication_classes=[]
- permission_classes = []
- throttle_classes = [AnonRateThrottle]
- def get(self,request,*args,**kwargs):
- return Response('我是未登录用户,TestView5')
复制代码 限制登录用户的访问频次- 全局:在setting中
- 'DEFAULT_THROTTLE_CLASSES': (
- 'rest_framework.throttling.AnonRateThrottle',
- 'rest_framework.throttling.UserRateThrottle'
- ),
- 'DEFAULT_THROTTLE_RATES': {
- 'user': '10/m',
- 'anon': '5/m',
- }
-
- 局部配置:
- 在视图类中配一个就行
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |