马上注册,结交更多好友,享用更多功能,让你轻松玩转社区。
您需要 登录 才可以下载或查看,没有账号?立即注册
x
实现颜色的随时调整,追加橡皮擦功能
widget.h
- #ifndef WIDGET_H
- #define WIDGET_H
- #include <QWidget>
- #include <QColor>
- #include <QPoint>
- #include <QVector>
- #include <QMouseEvent>
- #include <QPainter>
- #include <QColorDialog>
- // 自定义 Line 类,存储线段的起点、终点、颜色和宽度
- class Line {
- public:
- Line(const QPoint& start, const QPoint& end, const QColor& color, int width)
- : start(start), end(end), color(color), width(width) {}
- QPoint start;
- QPoint end;
- QColor color;
- int width;
- };
- QT_BEGIN_NAMESPACE
- namespace Ui { class Widget; }
- QT_END_NAMESPACE
- class Widget : public QWidget
- {
- Q_OBJECT
- public:
- Widget(QWidget *parent = nullptr);
- ~Widget();
- protected:
- void paintEvent(QPaintEvent *event) override; // 重写绘图事件
- void mouseMoveEvent(QMouseEvent *event) override; // 重写鼠标移动事件
- void mousePressEvent(QMouseEvent *event) override; // 重写鼠标按下事件
- void mouseReleaseEvent(QMouseEvent *event) override; // 重写鼠标释放事件
- private slots:
- void on_pushButton_clicked(); // 打开调色板
- void on_pushButton_2_clicked(); // 设置宽度为1
- void on_pushButton_3_clicked(); // 设置宽度为5
- void on_pushButton_4_clicked(); // 设置宽度为10
- void on_pushButton_5_clicked(); // 切换到画笔模式
- void on_pushButton_6_clicked(); // 切换到橡皮擦模式
- private:
- Ui::Widget *ui;
- QVector<Line> lines; // 存储所有线段的容器
- QPoint start; // 线段起点
- QPoint end; // 线段终点
- QColor color; // 当前颜色
- int width; // 当前宽度
- bool isEraserMode; // 是否处于橡皮擦模式
- };
- #endif // WIDGET_H
复制代码 widget.cpp
- #include "widget.h"
- #include "ui_widget.h"
- Widget::Widget(QWidget *parent)
- : QWidget(parent)
- , ui(new Ui::Widget)
- {
- ui->setupUi(this);
- color = Qt::black; // 默认颜色
- width = 1; // 默认宽度
- isEraserMode = false; // 默认是画笔模式
- }
- Widget::~Widget()
- {
- delete ui;
- }
- void Widget::paintEvent(QPaintEvent *event)
- {
- QPainter painter(this);
- // 遍历所有线段并绘制
- for (const auto& line : lines) {
- QPen pen(line.color, line.width);
- painter.setPen(pen);
- painter.drawLine(line.start, line.end);
- }
- }
- void Widget::mouseMoveEvent(QMouseEvent *event)
- {
- if (event->buttons() & Qt::LeftButton) {
- end = event->pos();
- QColor currentColor = isEraserMode ? Qt::white : color; // 橡皮擦模式下使用背景颜色
- lines.append(Line(start, end, currentColor, width)); // 保存线段信息
- start = end;
- update(); // 触发重绘
- }
- }
- void Widget::mousePressEvent(QMouseEvent *event)
- {
- if (event->button() == Qt::LeftButton) {
- start = event->pos();
- }
- }
- void Widget::mouseReleaseEvent(QMouseEvent *event)
- {
- if (event->button() == Qt::LeftButton) {
- end = event->pos();
- QColor currentColor = isEraserMode ? Qt::white : color; // 橡皮擦模式下使用背景颜色
- lines.append(Line(start, end, currentColor, width)); // 保存线段信息
- update(); // 触发重绘
- }
- }
- // 打开调色板
- void Widget::on_pushButton_clicked()
- {
- color = QColorDialog::getColor(Qt::black, this, "选择颜色");
- }
- // 设置宽度为1
- void Widget::on_pushButton_2_clicked()
- {
- width = 1;
- }
- // 设置宽度为5
- void Widget::on_pushButton_3_clicked()
- {
- width = 5;
- }
- // 设置宽度为10
- void Widget::on_pushButton_4_clicked()
- {
- width = 10;
- }
- // 切换到画笔模式
- void Widget::on_pushButton_5_clicked()
- {
- isEraserMode = false; // 设置为画笔模式
- ui->pushButton_5->setStyleSheet("background-color: lightgray;"); // 高亮画笔模式按钮
- ui->pushButton_6->setStyleSheet(""); // 取消橡皮擦模式按钮的高亮
- }
- // 切换到橡皮擦模式
- void Widget::on_pushButton_6_clicked()
- {
- isEraserMode = true; // 设置为橡皮擦模式
- ui->pushButton_6->setStyleSheet("background-color: lightgray;"); // 高亮橡皮擦模式按钮
- ui->pushButton_5->setStyleSheet(""); // 取消画笔模式按钮的高亮
- }
复制代码 运行现象
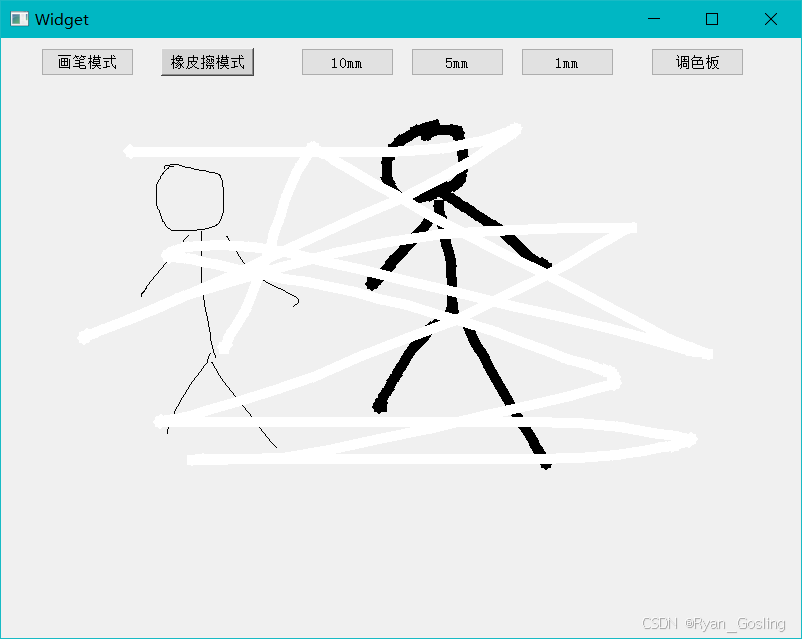
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |