1.创建
2.配置tomcat
3.创建webapp
step01,war包
step02
创建web.xml
- [/code][size=5]4.构建SpringMVC[/size]
- [indent]导入jar包
- [/indent][code] org.springframework spring-webmvc 5.3.23 javax.servlet javax.servlet-api 4.0.1 provided
复制代码web.xml配置DispacheServlet,核心拦截器
- springmvc org.springframework.web.servlet.DispatcherServlet contextConfigLocation classpath:springmvc.xml springmvc /
复制代码springmvc.xml
5.SpringIOC
创建配置文件applicationcontext.xml
- [/code][indent]配置监听器,该监听器的作用是在服务器启动的时候读ioc配置文件
- 继承在web.xml中添加
- [/indent][code] contextConfigLocation classpath:applicationcontext.xml org.springframework.web.context.ContextLoaderListener
复制代码 6.MyBatis
导入jar包
- org.mybatis mybatis 3.5.6 mysql mysql-connector-java 8.0.28 org.mybatis mybatis-spring 2.0.6 org.springframework spring-orm 5.3.23 com.alibaba druid 1.2.8
复制代码在MyConfig中创建3个bean,加入IOC
applicationcontext.xml
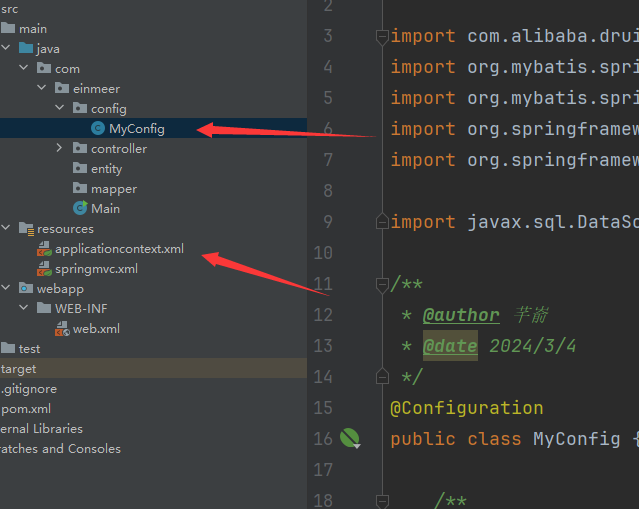 - [/code][indent]MyConfig
- [/indent][code]package com.einmeer.config;import com.alibaba.druid.pool.DruidDataSource;import org.mybatis.spring.SqlSessionFactoryBean;import org.mybatis.spring.mapper.MapperScannerConfigurer;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import javax.sql.DataSource;/*** @author 芊嵛* @date 2024/3/4*/@Configurationpublic class MyConfig { /*** 配置连接池* @return*/ @Bean DataSource getDataSource(){ DruidDataSource dataSource = new DruidDataSource(); dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver"); dataSource.setUrl("jdbc:mysql://192.168.21.130:3306/test?useUnicode=true&characterEncoding=utf8"); dataSource.setUsername("root"); dataSource.setPassword("2459689935");; dataSource.setMinIdle(8); dataSource.setMaxActive(20); return dataSource; } @Bean SqlSessionFactoryBean getSqlSessionFactory(){ SqlSessionFactoryBean bean = new SqlSessionFactoryBean(); bean.setDataSource(getDataSource()); bean.setTypeAliasesPackage("com.einmeer.entity"); return bean; } @Bean MapperScannerConfigurer getMapperScanner(){ MapperScannerConfigurer bean = new MapperScannerConfigurer(); bean.setBasePackage("com.einmeer.mapper"); return bean; }}
复制代码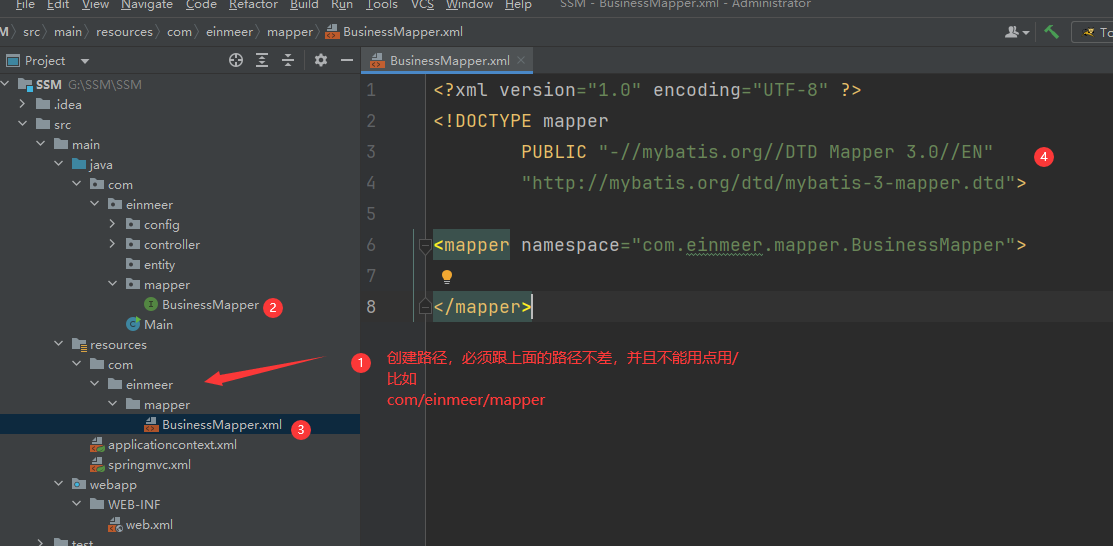
mapper.xml固定的头
- [/code][size=5]7.简化entity[/size]
- [indent]下载lombok插件
- [/indent][align=center][img]https://img2024.cnblogs.com/blog/2815669/202403/2815669-20240304233346563-767183280.png[/img][/align]
- [indent]导入jar
- [/indent][code] org.projectlombok lombok 1.18.24 provided
复制代码 8.停止到如今的配置整合
8.1MyConfig.java
- package com.einmeer.config;import com.alibaba.druid.pool.DruidDataSource;import org.mybatis.spring.SqlSessionFactoryBean;import org.mybatis.spring.mapper.MapperScannerConfigurer;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import javax.sql.DataSource;/** * @author 芊嵛 * @date 2024/3/4 */@Configurationpublic class MyConfig { /** * 配置连接池 * * @return */ @Bean DataSource getDataSource() { DruidDataSource dataSource = new DruidDataSource(); dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver"); dataSource.setUrl("jdbc:mysql://192.168.21.130:3306/test?useUnicode=true&characterEncoding=utf8"); dataSource.setUsername("root"); dataSource.setPassword("2459689935"); ; dataSource.setMinIdle(8); dataSource.setMaxActive(20); return dataSource; } @Bean SqlSessionFactoryBean getSqlSessionFactory() { SqlSessionFactoryBean bean = new SqlSessionFactoryBean(); bean.setDataSource(getDataSource()); // 包别名,不写的话,mapper中返回值类型要写全 bean.setTypeAliasesPackage("com.einmeer.entity"); return bean; } @Bean MapperScannerConfigurer getMapperScanner() { MapperScannerConfigurer bean = new MapperScannerConfigurer(); // mapper别名 bean.setBasePackage("com.einmeer.mapper"); return bean; }}
复制代码 8.2applicationcontext.xml
8.3springmvc.xml
8.4web.xml
- springmvc org.springframework.web.servlet.DispatcherServlet contextConfigLocation classpath:springmvc.xml springmvc / contextConfigLocation classpath:applicationcontext.xml org.springframework.web.context.ContextLoaderListener
复制代码 9.测试
9.1Business.java
- package com.einmeer.entity;import lombok.AllArgsConstructor;import lombok.Data;import lombok.NoArgsConstructor;import java.math.BigDecimal;/** * @author 芊嵛 * @date 2024/3/4 */@Data // get/set方法@AllArgsConstructor // 有参(全参)@NoArgsConstructor // 无参public class Business { private Integer businessId; private String businessName; private String businessAddress; private String businessExplain; private String businessImg; private Integer orderTypeId; private BigDecimal startPrice; private BigDecimal deliveryPrice; private String remarks;}
复制代码 9.2BusinessMapper.java
- package com.einmeer.mapper;import com.einmeer.entity.Business;import java.util.List;/** * @author 芊嵛 * @date 2024/3/4 */public interface BusinessMapper { // 查询所有信息 List list();}
复制代码 9.3BusinessMapper.xml
- select businessId, businessName, businessAddress, businessExplain, businessImg, orderTypeId, startPrice, deliveryPrice, remarks from business;
复制代码 9.4BusinessController
- package com.einmeer.controller;import com.einmeer.entity.Business;import com.einmeer.mapper.BusinessMapper;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import javax.annotation.Resource;import java.util.List;/** * @author 芊嵛 * @date 2024/3/4 *///返回字符串@RestController// 多一层路径@RequestMapping("/business")public class BusinessController { // 自动new,只限一次 @Resource BusinessMapper businessMapper; // 调用方法的路径 @GetMapping("/list") String list() { System.out.println(businessMapper.list()); return "index"; }}
复制代码
10.输出到页面上
停止如今能打印到控制台,要想出入到页面上需要继承配置
导入jar,转换成JSON字符串
- com.alibaba fastjson 2.0.32
复制代码springmvc.xml配置消息转换器与跨域
- application/json;charset-utf-8
复制代码BusinessController.java修改一下
- package com.einmeer.controller;import com.einmeer.entity.Business;import com.einmeer.mapper.BusinessMapper;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import javax.annotation.Resource;import java.util.List;/** * @author 芊嵛 * @date 2024/3/4 *///返回字符串@RestController// 多一层路径@RequestMapping("/business")public class BusinessController { // 自动new,只限一次 @Resource BusinessMapper businessMapper; // 调用方法的路径 @GetMapping("/list") List list() { return businessMapper.list(); }}
复制代码
11.把sql语句输出到控制窗口
导包
- org.duracloud common 7.0.0
复制代码 12.一次性插入多条数据
- INSERT INTO business ( businessName, businessAddress, businessExplain, businessImg, orderTypeId, startPrice, deliveryPrice, remarks ) VALUES (#{abusiness.businessName}, #{abusiness.businessAddress}, #{abusiness.businessExplain}, #{abusiness.businessImg}, #{abusiness.orderTypeId}, #{abusiness.startPrice}, #{abusiness.deliveryPrice}, #{abusiness.remarks} ) ;
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |