WPF 实现带蒙版的 MessageBox 消息提示框
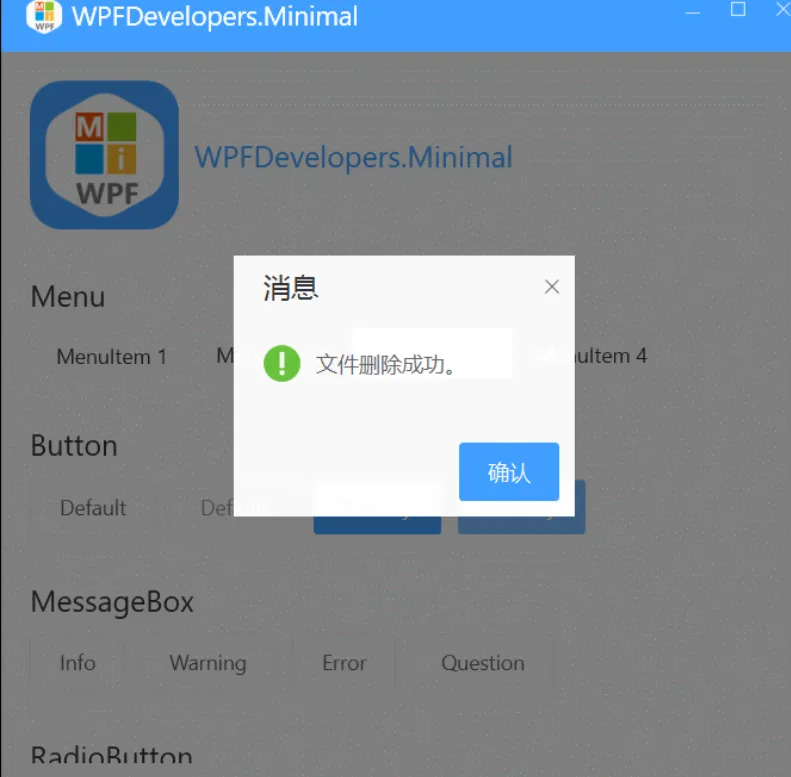
WPF 实现带蒙版的 MessageBox 消息提示框
作者:WPFDevelopersOrg
原文链接: https://github.com/WPFDevelopersOrg/WPFDevelopers.Minimal
- 框架使用大于等于.NET40;
- Visual Studio 2022;
- 项目使用 MIT 开源许可协议;
- Nuget Install-Package WPFDevelopers.Minimal 3.2.6-preview
MessageBox
- 实现MessageBox的Show五种方法;
- Show(string messageBoxText) 传入Msg参数;
- Show(string messageBoxText, string caption) 传入Msg与标题参数;
- Show(string messageBoxText, string caption, MessageBoxButton button) 传入Msg与标题、操作按钮参数;
- Show(string messageBoxText, string caption, MessageBoxImage icon) 传入Msg与标题、消息图片参数;
- Show(string messageBoxText, string caption, MessageBoxButton button, MessageBoxImage icon) 传入Msg与标题、操作按钮、消息图片参数;
- 拿到父级Window窗体的内容Content,放入一个Grid里,再在容器里放入一个半透明的Grid,最后将整个Grid赋给父级Window窗体的内容Content;
一、MessageBox.cs 代码如下;- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Windows;
- using System.Windows.Controls;
- using System.Windows.Media;
- namespace WPFDevelopers.Minimal.Controls
- {
- public static class MessageBox
- {
- public static MessageBoxResult Show(string messageBoxText)
- {
- var msg = new WPFMessageBox(messageBoxText);
- return GetWindow(msg);
- }
- public static MessageBoxResult Show(string messageBoxText, string caption)
- {
- var msg = new WPFMessageBox(messageBoxText, caption);
- return GetWindow(msg);
- }
- public static MessageBoxResult Show(string messageBoxText, string caption, MessageBoxButton button)
- {
- var msg = new WPFMessageBox(messageBoxText, caption, button);
- return GetWindow(msg);
- }
- public static MessageBoxResult Show(string messageBoxText, string caption, MessageBoxImage icon)
- {
- var msg = new WPFMessageBox(messageBoxText, caption, icon);
- return GetWindow(msg);
- }
- public static MessageBoxResult Show(string messageBoxText, string caption, MessageBoxButton button, MessageBoxImage icon)
- {
- var msg = new WPFMessageBox(messageBoxText, caption,button,icon);
- return GetWindow(msg);
- }
- static MessageBoxResult GetWindow(WPFMessageBox msg)
- {
- msg.WindowStartupLocation = WindowStartupLocation.CenterOwner;
- Window win = null;
- if (Application.Current.Windows.Count > 0)
- win = Application.Current.Windows.OfType<Window>().FirstOrDefault(o => o.IsActive);
- if (win != null)
- {
- var layer = new Grid() { Background = new SolidColorBrush(Color.FromArgb(128, 0, 0, 0)) };
- UIElement original = win.Content as UIElement;
- win.Content = null;
- var container = new Grid();
- container.Children.Add(original);
- container.Children.Add(layer);
- win.Content = container;
- msg.Owner = win;
- msg.ShowDialog();
- container.Children.Clear();
- win.Content = original;
- }
- else
- msg.Show();
- return msg.Result;
- }
- }
- }
复制代码 二、Styles.MessageBox.xaml 代码如下;- <ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
- xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
- xmlns:sys="clr-namespace:System;assembly=mscorlib"
- xmlns:wpfsc="clr-namespace:WPFDevelopers.Minimal.Controls">
-
- <ResourceDictionary.MergedDictionaries>
- <ResourceDictionary Source="../Themes/Basic/ControlBasic.xaml"/>
- <ResourceDictionary Source="../Themes/Basic/Animations.xaml"/>
- </ResourceDictionary.MergedDictionaries>
-
- </ResourceDictionary>
-
复制代码 三、WPFMessageBox.cs 代码如下;- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Text;
- using System.Windows;
- using System.Windows.Controls;
- using System.Windows.Media;
- using System.Windows.Shapes;
- namespace WPFDevelopers.Minimal.Controls
- {
- [TemplatePart(Name = TitleTemplateName, Type = typeof(TextBlock))]
- [TemplatePart(Name = CloseButtonTemplateName, Type = typeof(Button))]
- [TemplatePart(Name = MessageTemplateName, Type = typeof(TextBlock))]
- [TemplatePart(Name = ButtonCancelTemplateName, Type = typeof(Button))]
- [TemplatePart(Name = ButtonCancelTemplateName, Type = typeof(Button))]
- [TemplatePart(Name = PathTemplateName, Type = typeof(Path))]
- public sealed class WPFMessageBox : Window
- {
- private const string TitleTemplateName = "PART_Title";
- private const string CloseButtonTemplateName = "PART_CloseButton";
- private const string MessageTemplateName = "PART_Message";
- private const string ButtonCancelTemplateName = "PART_ButtonCancel";
- private const string ButtonOKTemplateName = "PART_ButtonOK";
- private const string PathTemplateName = "PART_Path";
- private string _messageString;
- private string _titleString;
- private Geometry _geometry;
- private SolidColorBrush _solidColorBrush;
- private Visibility _cancelVisibility = Visibility.Collapsed;
- private Visibility _okVisibility;
- private TextBlock _title;
- private TextBlock _message;
- private Button _closeButton;
- private Button _buttonCancel;
- private Button _buttonOK;
- private Path _path;
- static WPFMessageBox()
- {
- DefaultStyleKeyProperty.OverrideMetadata(typeof(WPFMessageBox), new FrameworkPropertyMetadata(typeof(WPFMessageBox)));
- }
- public override void OnApplyTemplate()
- {
- base.OnApplyTemplate();
- _title = GetTemplateChild(TitleTemplateName) as TextBlock;
- _message = GetTemplateChild(MessageTemplateName) as TextBlock;
- if (_title == null || _message == null)
- throw new InvalidOperationException("the title or message control is null!");
- _title.Text = _titleString;
- _message.Text = _messageString;
- _path = GetTemplateChild(PathTemplateName) as Path;
- if (_path != null)
- {
- _path.Data = _geometry;
- _path.Fill = _solidColorBrush;
- }
- _closeButton = GetTemplateChild(CloseButtonTemplateName) as Button;
- if (_closeButton != null)
- _closeButton.Click += _closeButton_Click;
- _buttonCancel = GetTemplateChild(ButtonCancelTemplateName) as Button;
- if (_buttonCancel != null)
- {
- _buttonCancel.Visibility = _cancelVisibility;
- _buttonCancel.Click += _buttonCancel_Click;
- }
- _buttonOK = GetTemplateChild(ButtonOKTemplateName) as Button;
- if (_buttonOK != null)
- {
- _buttonOK.Visibility = _okVisibility;
- _buttonOK.Click += _buttonOK_Click;
- }
- if (Owner == null)
- {
- BorderThickness = new Thickness(1);
- WindowStartupLocation = WindowStartupLocation.CenterScreen;
- }
- }
- private void _buttonOK_Click(object sender, RoutedEventArgs e)
- {
- Result = MessageBoxResult.OK;
- Close();
- }
- private void _buttonCancel_Click(object sender, RoutedEventArgs e)
- {
- Result = MessageBoxResult.Cancel;
- Close();
- }
- private void _closeButton_Click(object sender, RoutedEventArgs e)
- {
- Close();
- }
- protected override void OnClosed(EventArgs e)
- {
- base.OnClosed(e);
- if (Owner == null)
- return;
- var grid = Owner.Content as Grid;
- UIElement original = VisualTreeHelper.GetChild(grid, 0) as UIElement;
- grid.Children.Remove(original);
- Owner.Content = original;
- }
- public MessageBoxResult Result { get; set; }
- public WPFMessageBox(string message)
- {
-
- _messageString = message;
- }
- public WPFMessageBox(string message, string caption)
- {
- _titleString = caption;
- _messageString = message;
-
- }
- public WPFMessageBox(string message, string caption, MessageBoxButton button)
- {
- _titleString = caption;
- _messageString = message; ;
- }
- public WPFMessageBox(string message, string caption, MessageBoxImage image)
- {
- _titleString = caption;
- _messageString = message;
- DisplayImage(image);
- }
- public WPFMessageBox(string message, string caption, MessageBoxButton button, MessageBoxImage image)
- {
- _titleString = caption;
- _messageString = message;
- DisplayImage(image);
- DisplayButtons(button);
- }
- private void DisplayButtons(MessageBoxButton button)
- {
- switch (button)
- {
- case MessageBoxButton.OKCancel:
- case MessageBoxButton.YesNo:
- _cancelVisibility = Visibility.Visible;
- _okVisibility = Visibility.Visible;
- break;
- //case MessageBoxButton.YesNoCancel:
- // break;
- default:
- _okVisibility = Visibility.Visible;
- break;
- }
- }
- private void DisplayImage(MessageBoxImage image)
- {
- switch (image)
- {
- case MessageBoxImage.Warning:
- _geometry = Application.Current.Resources["PathWarning"] as Geometry;
- _solidColorBrush = Application.Current.Resources["WarningSolidColorBrush"] as SolidColorBrush;
- break;
- case MessageBoxImage.Error:
- _geometry = Application.Current.Resources["PathError"] as Geometry;
- _solidColorBrush = Application.Current.Resources["DangerSolidColorBrush"] as SolidColorBrush;
- break;
- case MessageBoxImage.Information:
- _geometry = Application.Current.Resources["PathWarning"] as Geometry;
- _solidColorBrush = Application.Current.Resources["SuccessSolidColorBrush"] as SolidColorBrush;
- break;
- case MessageBoxImage.Question:
- _geometry = Application.Current.Resources["PathQuestion"] as Geometry;
- _solidColorBrush = Application.Current.Resources["PrimaryNormalSolidColorBrush"] as SolidColorBrush;
- break;
- default:
- break;
- }
- }
- }
- }
复制代码 Nuget Install-Package WPFDevelopers.Minimal
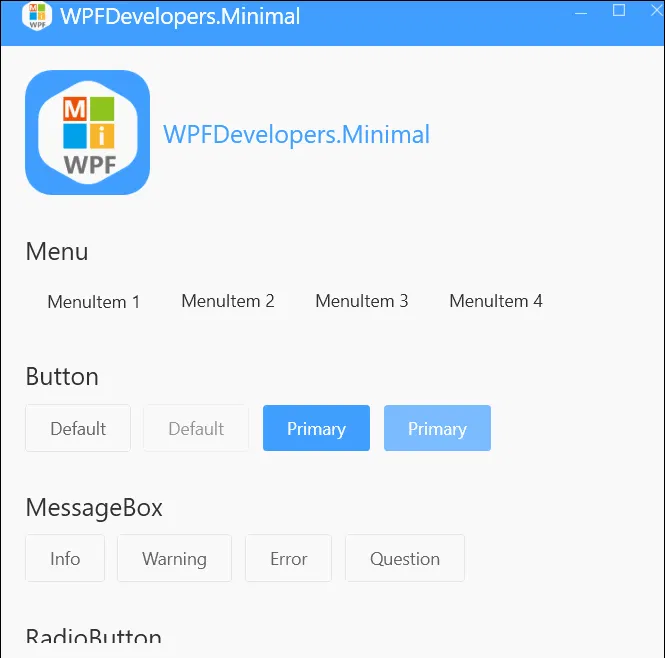
其他基础控件
1.Window
2.Button
3.CheckBox
4.ComboBox
5.DataGrid
6.DatePicker
7.Expander
8.GroupBox
9.ListBox
10.ListView
11.Menu
12.PasswordBox
13.TextBox
14.RadioButton
15.ToggleButton
16.Slider
17.TreeView
18.TabControl
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |