一、什么是DI依赖注入
依赖关系注入 (DI) 是一个过程,通过该过程,对象仅通过构造函数参数、工厂方法的参数或在构造对象实例或从工厂方法返回后在对象实例上设置的属性来定义其依赖关系(即,使
用它们使用的其他对象)。然后,容器在创建 Bean 时注入这些依赖项。这个过程基本上是Bean本身的反函数(因此得名“控制反转”),通过使用类的直接构造或服务定位器模式来控制
其依赖项的实例化或位置。
使用 DI 原则,代码更清晰,当对象与其依赖项一起提供时,解耦更有效。该对象不查找其依赖项,也不知道依赖项的位置或类。
二、依赖注入的方式
依赖注入有三种方式:基于构造函数的依赖关系注入、利用set方法的依赖关系注入、其他的依赖关系注入。
1.基于构造函数的依赖关系注入
这个在Spring(五)的学习中已经是说过了,这里仅再简单叙述一下构造函数注入的方式。
(1)通过index索引进行注入,索引从0开始。
(2)通过传入参数名进行注入,最方便。
(3)通过传参的类型进行注入,不适用于有同类型的情况。
(4)通过bean进行注入。
2.利用set方法的依赖关系注入
通过set方法进行依赖注入是最核心的注入方式。
这个前面的学习也已经用到了,不过前面注入的都是一些简单的基本类型和String类型,这里我们再对一些复杂的类型如List、Map等进行注入方法的学习。
(1)Student和Address是我们要用到的类
Address- package com.jms.pojo;
- public class Address {
- private int id;
- private String address;
- public int getId() {
- return id;
- }
- public void setId(int id) {
- this.id = id;
- }
- public String getAddress() {
- return address;
- }
- public void setAddress(String address) {
- this.address = address;
- }
- @Override
- public String toString() {
- return "Address{" +
- "id=" + id +
- ", address='" + address + '\'' +
- '}';
- }
- }
复制代码 Student- package com.jms.pojo;
- import java.util.*;
- public class Student {
- private String name;
- private Address address;
- private String[] lesson;
- private List<String> hobbys;
- private Map<String, String> card;
- private Set<String> games;
- private String graduate;
- private Properties info;
- public String[] getLesson() {
- return lesson;
- }
- public void setLesson(String[] lesson) {
- this.lesson = lesson;
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- public Address getAddress() {
- return address;
- }
- public void setAddress(Address address) {
- this.address = address;
- }
- public List<String> getHobbys() {
- return hobbys;
- }
- public void setHobbys(List<String> hobbys) {
- this.hobbys = hobbys;
- }
- public Map<String, String> getCard() {
- return card;
- }
- public void setCard(Map<String, String> card) {
- this.card = card;
- }
- public Set<String> getGames() {
- return games;
- }
- public void setGames(Set<String> games) {
- this.games = games;
- }
- public String getGraduate() {
- return graduate;
- }
- public void setGraduate(String graduate) {
- this.graduate = graduate;
- }
- public Properties getInfo() {
- return info;
- }
- public void setInfo(Properties info) {
- this.info = info;
- }
- @Override
- public String toString() {
- return "Student{" + "\n" +
- "name='" + name + '\'' + "\n" +
- "address=" + address.toString() + "\n" +
- "lesson=" + Arrays.toString(lesson) + "\n" +
- "hobbys=" + hobbys + "\n" +
- "card=" + card + "\n" +
- "games=" + games + "\n" +
- "graduate='" + graduate + '\'' + "\n" +
- "info=" + info + "\n" +
- '}';
- }
- }
复制代码 (2)beans.xml- <bean id="student" class="com.jms.pojo.Student">
-
- <property name="name" value="jms"/>
-
- <property name="address" ref="address"/>
-
- <property name="lesson">
- <array>
- <value>C</value>
- <value>Java</value>
- <value>Python</value>
- </array>
- </property>
-
- <property name="hobbys">
- <list>
- <value>编程</value>
- <value>写作</value>
- <value>听音乐</value>
- </list>
- </property>
-
- <property name="card">
- <map>
- <entry key="学生卡" value="101010101"/>
- <entry key="饭卡" value="20202020202"/>
- <entry key="水卡" value="30303030303"/>
- </map>
- </property>
-
- <property name="games">
- <set>
- <value>game1</value>
- <value>game2</value>
- <value>game3</value>
- </set>
- </property>
-
- <property name="graduate">
- <null/>
- </property>
-
- <property name="info">
- <props>
- <prop key="学号">100001</prop>
- <prop key="姓名">啊范德萨</prop>
- <prop key="班级">计算机3班</prop>
- </props>
- </property>
- </bean>
- <bean id="address" class="com.jms.pojo.Address">
- <property name="id" value="1"/>
- <property name="address" value="world"/>
- </bean>
复制代码 (3)测试- @Test
- public void studentTest() {
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("beans.xml");
- Student student = applicationContext.getBean("student", Student.class);
- System.out.println(student);
- }
复制代码 测试结果:
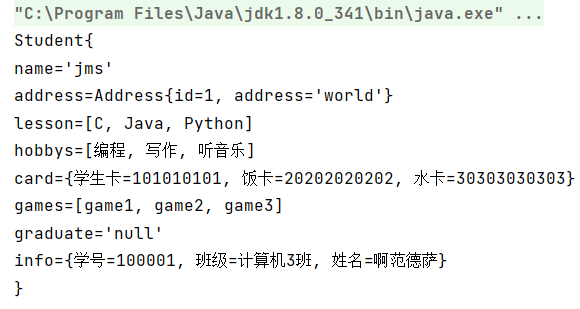
3.其他的依赖关系注入
c命名空间注入和p命名空间注入,这是官方给出的拓展的注入方式,p命名空间注入对应set注入,c命名空间注入对应有参构造注入。
使用这两种注入时都需要添加约束,以下是官方给出的使用示例:
p-namespace:- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns:p="http://www.springframework.org/schema/p"
- xsi:schemaLocation="http://www.springframework.org/schema/beans
- https://www.springframework.org/schema/beans/spring-beans.xsd">
- <bean name="john-classic" class="com.example.Person">
- <property name="name" value="John Doe"/>
- <property name="spouse" ref="jane"/>
- </bean>
- <bean name="john-modern"
- class="com.example.Person"
- p:name="John Doe"
- p:spouse-ref="jane"/>
- <bean name="jane" class="com.example.Person">
- <property name="name" value="Jane Doe"/>
- </bean>
- </beans>
复制代码 c-namespace:- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns:p="http://www.springframework.org/schema/p"
- xsi:schemaLocation="http://www.springframework.org/schema/beans
- https://www.springframework.org/schema/beans/spring-beans.xsd">
- <bean name="john-classic" class="com.example.Person">
- <property name="name" value="John Doe"/>
- <property name="spouse" ref="jane"/>
- </bean>
- <bean name="john-modern"
- class="com.example.Person"
- p:name="John Doe"
- p:spouse-ref="jane"/>
- <bean name="jane" class="com.example.Person">
- <property name="name" value="Jane Doe"/>
- </bean>
- </beans>
复制代码
(本文仅作个人学习记录用,如有纰漏敬请指正)
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |