实现逻辑
1、后端功能:随机生成验证码图片,并把交给前端、接收用户输入,验证用户输入的验证码是否正确、
2、前端功能:显示验证码,提供输入框供用户输入他们看到的验证码,把用户输入的数据交给后端,接收后端返回的验证结果
前后端交互接口
后端必要完成的两个工作:1、生成验证码,2、校验验证码的正确性
接口定义:
1、生成验证码
请求url:/captcha/getCaptcha
相应:返回验证码的图片
2、校验验证码的正确性
请求url:/captcha/check
请求参数:用户输入的验证码captcha
相应:验证码正确返回true,错误返回false
前端代码
index.html
展示效果:
用户点击图片之后,可以更新验证码图片
代码如下:
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="utf-8">
- <title>验证码</title>
- <style>
- body {
- font-family: 'Arial', sans-serif;
- background-color: #f7f7f7;
- margin: 0;
- padding: 0;
- display: flex;
- justify-content: center;
- align-items: center;
- height: 100vh;
- }
- #container {
- text-align: center;
- background: white;
- padding: 50px;
- border-radius: 8px;
- box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
- }
- h1 {
- color: #333;
- font-size: 2em;
- margin-bottom: 20px;
- }
- #inputCaptcha {
- height: 40px;
- margin-right: 10px;
- vertical-align: middle;
- border: 1px solid #ddd;
- border-radius: 4px;
- padding: 0 10px;
- }
- #verificationCodeImg {
- vertical-align: middle;
- border: 1px solid #ddd;
- cursor: pointer;
- transition: transform 0.2s;
- }
- #verificationCodeImg:hover {
- transform: scale(1.05);
- }
- #checkCaptcha {
- height: 40px;
- width: 120px;
- background-color: #5cb85c;
- color: white;
- border: none;
- border-radius: 4px;
- cursor: pointer;
- transition: background-color 0.2s;
- }
- #checkCaptcha:hover {
- background-color: #4cae4c;
- }
- </style>
- </head>
- <body>
- <div id="container">
- <h1>输入验证码</h1>
- <div id="confirm">
- <input type="text" name="inputCaptcha" id="inputCaptcha">
- <img id="verificationCodeImg" src="http://127.0.0.1:8080/captcha/getCaptcha" style="cursor: pointer;"
- title="看不清?换一张"/>
- <input type="button" value="提交" id="checkCaptcha">
- </div>
- </div>
- <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
- <script>
- $("#verificationCodeImg").click(function () {
- $(this).hide().attr('src', 'http://127.0.0.1:8080/captcha/getCaptcha?dt=' + new Date().getTime()).fadeIn();
- });
- $("#checkCaptcha").click(function () {
- $.ajax({
- type: "post",
- url: "captcha/check",
- data: {
- captcha: $("#inputCaptcha").val()
- },
- success: function (result) {
- if (result) {
- location.href = "success.html"
- } else {
- alert("验证码错误")
- }
- }
- });
- });
- </script>
- </body>
- </html>
复制代码 success.html,当用户验证码输入正确时展示的内容
展示效果:
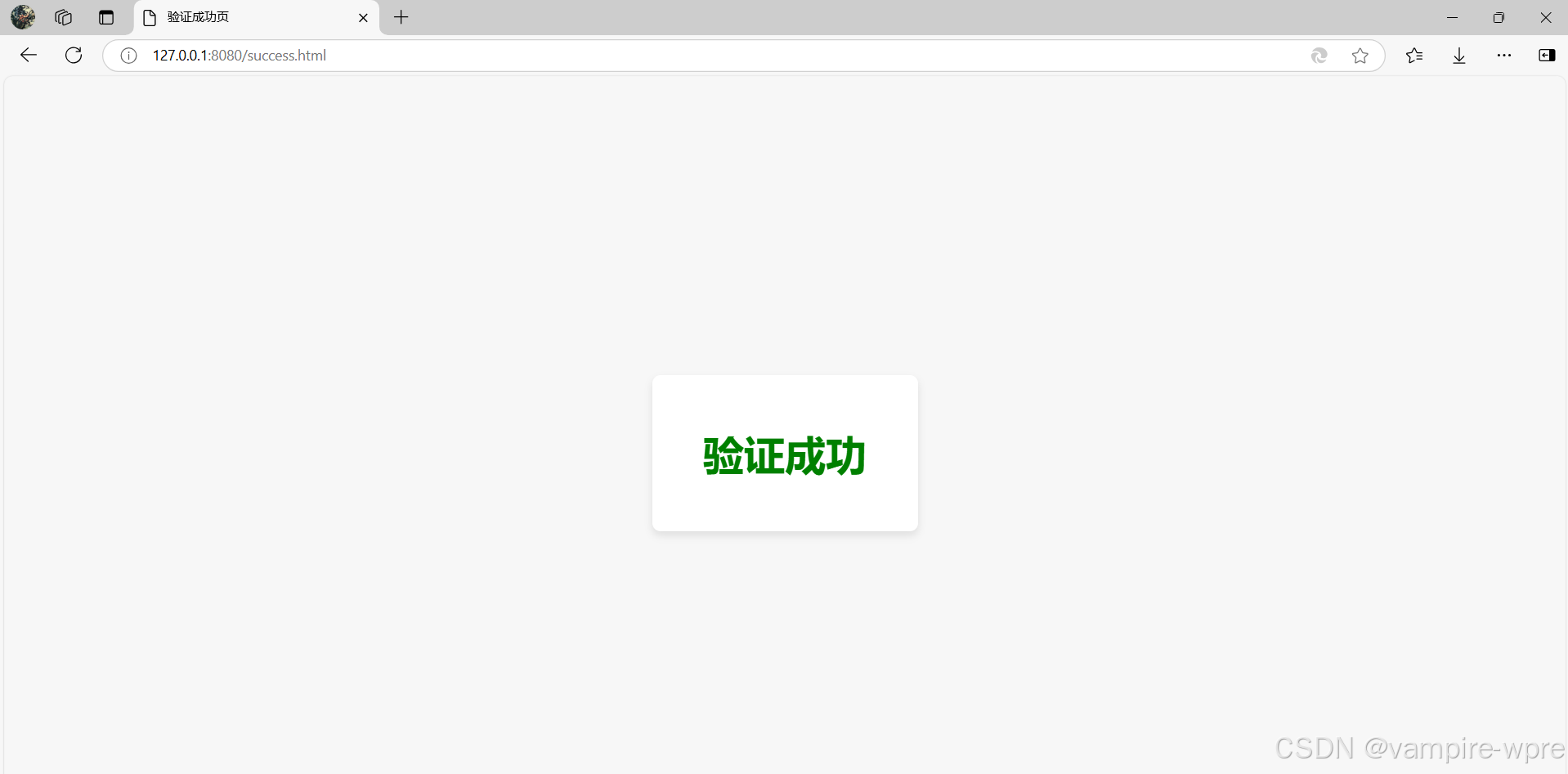
代码如下:
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <title>验证成功页</title>
- <style>
- body {
- font-family: 'Arial', sans-serif;
- background-color: #f7f7f7;
- margin: 0;
- padding: 0;
- display: flex;
- justify-content: center;
- align-items: center;
- height: 100vh;
- }
- .container {
- text-align: center;
- background: white;
- padding: 50px;
- border-radius: 8px;
- box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
- }
- h1 {
- color: green;
- font-size: 2.5em;
- margin: 0;
- }
- p {
- color: blue;
- font-size: 1.2em;
- margin-top: 20px;
- }
- </style>
- </head>
- <body>
- <div class="container">
- <h1>验证成功</h1>
- </div>
- </body>
- </html>
复制代码 后端代码
结构如下:
在pom.xml中添加依赖:
- <dependency>
- <groupId>cn.hutool</groupId>
- <artifactId>hutool-captcha</artifactId>
- <version>5.8.26</version>
- </dependency>
复制代码 CaptchaController类:
- @RestController
- @RequestMapping("/captcha")
- public class CaptchaController {
- //注入
- @Autowired
- private CaptchaProperties captchaProperties;
- @Value("${captcha.session.key}")
- private String key;
- @Value("${captcha.session.datetime}")
- private String datetime;
- @RequestMapping("/getCaptcha")
- public void getCaptcha(HttpServletResponse response, HttpSession session) throws IOException {
- //定义图形验证码的长和宽
- LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(captchaProperties.getWidth(), captchaProperties.getHeight());
- String code = lineCaptcha.getCode();
- //设置session,把验证码信息存储到session中
- session.setAttribute(key, code);
- session.setAttribute(datetime, new Date());
- //验证码写入浏览器
- lineCaptcha.write(response.getOutputStream());
- //设置ContentType
- response.setContentType("image/jpeg");
- //设置编码
- response.setCharacterEncoding("utf8");
- //防止浏览器缓存验证码图片
- response.setHeader("Pragma", "No-cache");
- }
- @RequestMapping("/check")
- public boolean check(String captcha, HttpSession session) {
- String code = (String) session.getAttribute(key);
- if (!StringUtils.hasLength(captcha)) {
- return false;
- }
- //从session中获取时间
- Date date = (Date) session.getAttribute(datetime);
- if (date == null || System.currentTimeMillis() - date.getTime() > 60 * 1000) {
- //session为null,或者session过期(超过一分钟就算过期)
- //System.currentTimeMillis() - date.getTime(): 当前时间-请求时间
- return false;
- }
- //不区分大小写
- return captcha.equalsIgnoreCase(code);
- }
- }
复制代码 CaptchaProperties类:
- @ConfigurationProperties(prefix = "captcha")
- @Configuration
- @Data
- public class CaptchaProperties {
- private Integer height;
- private Integer width;
- }
复制代码 MySession类:
- @Data
- public class MySession {
- private String key;
- private String datetime;
- }
复制代码 配置文件 application.yml:
- captcha:
- height: 50
- width: 150
- session:
- key: CaptchaCode
- datetime: CaptchaDate
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。
|