HarmonyOS鸿蒙体系上,file文件常用操纵记录
1.创建文件
- createFile(fileName: string, content: string): string {
- // 获取应用文件路径
- let context = getContext(this) as common.UIAbilityContext;
- let filesDirPath = context.filesDir + '/' + fileName;
- // 新建并打开文件
- let file = fs.openSync(filesDirPath, fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE);
- // 写入一段内容至文件
- let writeLen = fs.writeSync(file.fd, content);
- // 从文件读取一段内容
- let buf = new ArrayBuffer(1024);
- let readLen = fs.readSync(file.fd, buf, { offset: 0 });
- // 关闭文件
- fs.closeSync(file);
- return filesDirPath
- }
复制代码 2.将沙箱文件移动到分布式文件夹
- /**
- * 创建分布式文件
- */
- async createDistributedFile(uri: string) {
- // 获取应用文件路径
- let context = getContext(this) as common.UIAbilityContext;
- let filesDir = context.distributedFilesDir;
- let fileName = this.getFileName(uri)
- try {
- let destUriPath = filesDir + '/' + fileName
- this.tempFilePath = fileName
- //给新建的文件写入内容
- // mMediaFileUtils.writeFileContent(destUriPath,content)
- // 新建并打开文件
- let writeFile = fs.openSync(destUriPath, fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE);
- //设置文件权限
- securityLabel.setSecurityLabel(destUriPath, 's1').then(() => {
- })
- // fs.write(file.fd,content)
- //读取原文件内容
- // 读取图片为buffer
- const file = fs.openSync(uri, fs.OpenMode.READ_ONLY);
- let photoSize = fs.statSync(file.fd).size;
- let buffer = new ArrayBuffer(photoSize);
- fs.readSync(file.fd, buffer);
- fs.closeSync(file);
- //开始写入内容
- let writeLen = fs.write(writeFile.fd, buffer)
- } catch (error) {
- }
- }
复制代码 3.读取分布式文件
- /**
- * 读取分布式文件
- * @param filePath
- */
- readDistributedFile(filePath: string) {
- let context = getContext(this) as common.UIAbilityContext;
- let distributedFilesDir = context.distributedFilesDir;
- let distributedFilesDirs = fs.listFileSync(distributedFilesDir);
- for (let i = 0; i < distributedFilesDirs.length; i++) {
- let fileName = distributedFilesDir + '/' + distributedFilesDirs[i]
- this.readFile(fileName)
- }
- }
复制代码 4.读取文件内容
- /**
- * 读取文件
- * @param filePath 文件路径
- */
- readFile(filePath: string) {
- try {
- // 打开分布式目录下的文件
- let file = fs.openSync(filePath, fs.OpenMode.READ_WRITE);
- // 定义接收读取数据的缓存
- let arrayBuffer = new ArrayBuffer(4096);
- // 读取文件的内容,返回值是读取到的字节个数
- class Option {
- public offset: number = 0;
- public length: number = 0;
- }
- let option = new Option();
- option.length = arrayBuffer.byteLength;
- let num = fs.readSync(file.fd, arrayBuffer, option);
- // 打印读取到的文件数据
- let buf = buffer.from(arrayBuffer, 0, num);
- Log.info('读取的文件内容: ' + buf.toString());
- } catch (error) {
- let err: BusinessError = error as BusinessError;
- Log.error(`Failed to openSync / readSync. Code: ${err.code}, message: ${err.message}`);
- }
- }
复制代码 5.产看文件列表(沙箱和分布式文件夹)
- /**
- * 查看文件列表 沙箱文件夹 和 分布式文件夹
- */
- lookFilesList(): string {
- //沙箱文件夹
- let allFiles = ''
- let context = getContext(this) as common.UIAbilityContext;
- let filesDir = context.filesDir;
- let files = fs.listFileSync(filesDir);
- for (let i = 0; i < files.length; i++) {
- Log.info(`当前设备文件 name: ${files[i]}`);
- }
- //分布式文件夹
- let distributedFilesDir = context.distributedFilesDir;
- Log.info('context.distributedFilesDir: ' + distributedFilesDir)
- let distributedFilesDirs = fs.listFileSync(distributedFilesDir);
- if (distributedFilesDirs.length > 0) {
- for (let i = 0; i < distributedFilesDirs.length; i++) {
- Log.info(`分布式文件 name: ${distributedFilesDirs[i]}`);
- }
- }
- return allFiles;
- }
复制代码 6.删除分布式下指定文件
- /**
- * 删除分布式指定文件
- */
- deleteDistributedFile(fileName: string) {
- let context = getContext(this) as common.UIAbilityContext;
- let filePath = context.distributedFilesDir + '/' + fileName
- securityLabel.setSecurityLabel(filePath, 's1').then(() => {
- Log.info('Succeeded in setSecurityLabeling.');
- })
- try {
- fs.rmdir(filePath)
- Log.info('刪除文件成功')
- } catch (error) {
- let err: BusinessError = error as BusinessError;
- Log.error(`Failed to openSync / readSync. Code: ${err.code}, message: ${err.message}`);
- }
- }
复制代码 7. 删除当地文件
- /**
- * 删除本地文件
- */
- deleteCurrentDeviceFile() {
- let context = getContext(this) as common.UIAbilityContext;
- let filesDir = context.filesDir;
- let filesDirs = fs.listFileSync(filesDir);
- if (filesDirs.length <= 0) {
- return
- }
- let fileName = filesDirs + '/' + filesDirs[0]
- securityLabel.setSecurityLabel(fileName, 's1').then(() => {
- Log.info('Succeeded in setSecurityLabeling.');
- })
- try {
- fs.rmdir(fileName)
- Log.info('刪除文件成功')
- } catch (error) {
- let err: BusinessError = error as BusinessError;
- Log.error(`Failed to openSync / readSync. Code: ${err.code}, message: ${err.message}`);
- }
- }
复制代码 8.获取文件名
- /**
- * 获取文件名
- * @param filePath
- * @returns
- */
- getFileName(filePath: string): string {
- const parts = filePath.split('/');
- if (parts.length === 0) {
- return '';
- } // 如果没有找到任何斜杠,返回null
- const fileNameWithExtension = parts[parts.length - 1];
- const fileNameParts = fileNameWithExtension.split('.');
- if (fileNameParts.length < 2) {
- return fileNameWithExtension;
- } // 如果没有扩展名,直接返回文件名
- // return fileNameParts[0]; // 返回文件名(不含扩展名)
- return fileNameWithExtension; // 返回文件名,含扩展名
- }
复制代码 9.保存文件到当地文件夹
- /**
- * 拉起picker保存文件
- */
- async saveFile(fileName: string):Promise<string>{
- let saveFileUri = ''
- try {
- let DocumentSaveOptions = new picker.DocumentSaveOptions();
- DocumentSaveOptions.newFileNames = [fileName];
- let documentPicker = new picker.DocumentViewPicker();
- await documentPicker.save(DocumentSaveOptions).then( (DocumentSaveResult) => {
- if (DocumentSaveResult !== null && DocumentSaveResult !== undefined) {
- let uri = DocumentSaveResult[0] as string;
- this.realFileUri = uri
- this.realSaveFile(fileName)
- saveFileUri = uri
- }
- }).catch((err: BusinessError) => {
- Log.error('saveFile-DocumentViewPicker.save failed with err: ' + JSON.stringify(err));
- });
- } catch (err) {
- Log.error('saveFile-DocumentViewPicker failed with err: ' + err);
- }
- return saveFileUri
- }
- realFileUri: string = ''
- /**
- * 真正开始保存文件
- */
- async realSaveFile(fileName: string) {
- let context = getContext(this) as common.UIAbilityContext;
- let pathDir = context.distributedFilesDir;
- // 获取分布式目录的文件路径
- let filePath = pathDir + '/' + fileName;
- // 读取buffer
- const file = fs.openSync(filePath, fs.OpenMode.READ_ONLY);
- let photoSize = fs.statSync(file.fd).size;
- let buffer = new ArrayBuffer(photoSize);
- fs.readSync(file.fd, buffer);
- fs.closeSync(file);
- let saveFile = fs.openSync(this.realFileUri, fs.OpenMode.WRITE_ONLY);
- let writeLen = fs.write(saveFile.fd, buffer)
- Log.info(`save file uri success ~~~~~` + writeLen);
- //保存成功以后删除分布式上面的文件
- this.deleteDistributedFile(filePath)
- }
复制代码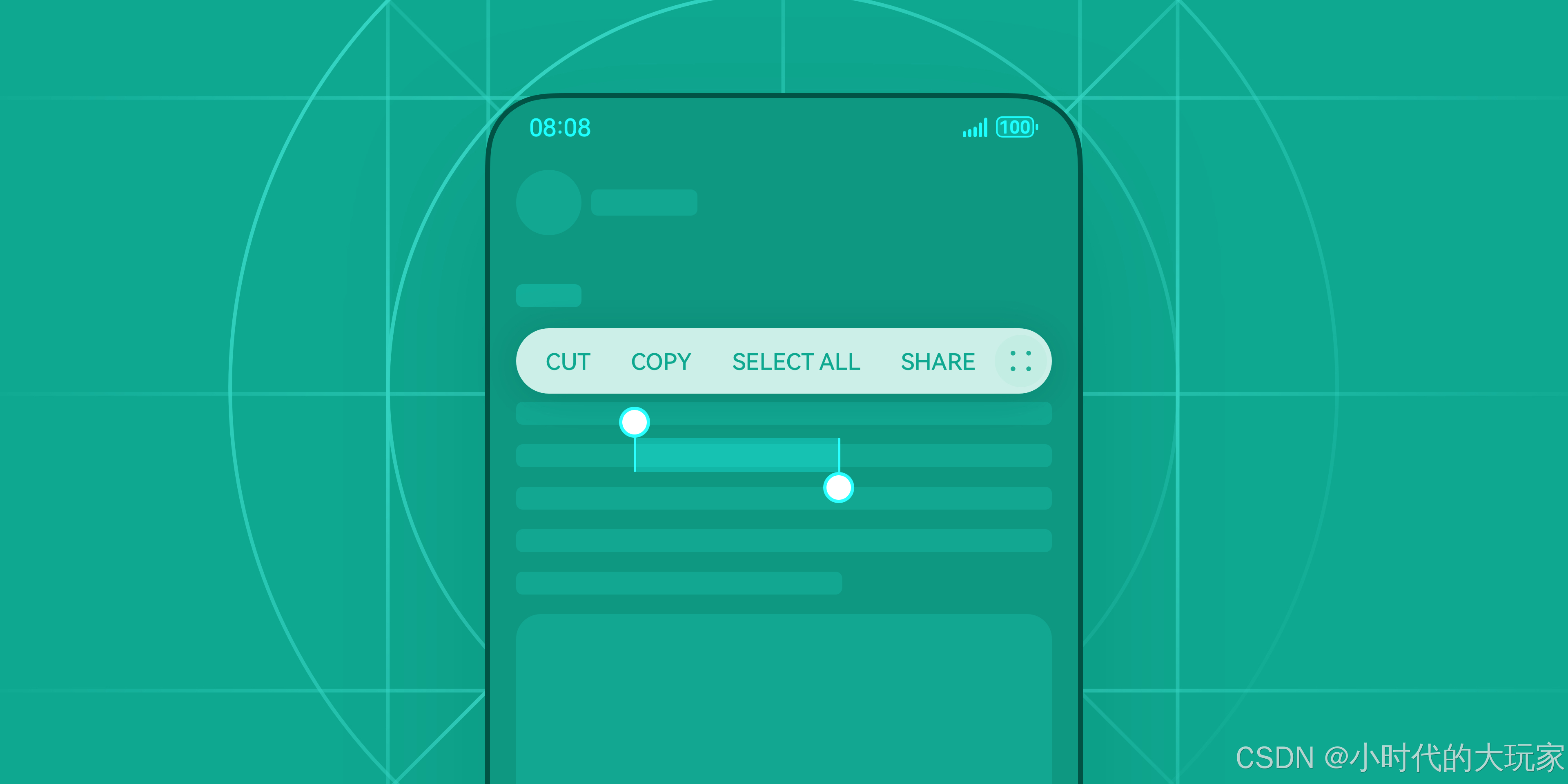
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |