〇、参考资料
1、Spring Boot 中文乱码问题解决方案汇总
https://blog.51cto.com/u_15236724/5372824
2、spring boot读取自定义配置properties文件★
https://www.yisu.com/zixun/366877.html
3、spring boot通过配置工厂类,实现读取指定位置的yml文件★
https://blog.csdn.net/weixin_45168162/article/details/125427465
4、springBoot 读取yml 配置文件的三种方式(包含以及非component下)★
https://blog.csdn.net/weixin_44131922/article/details/126866040
5、SpringBoot集成Swagger的详细步骤
https://blog.csdn.net/m0_67788957/article/details/123670244
一、项目介绍
1、项目框架
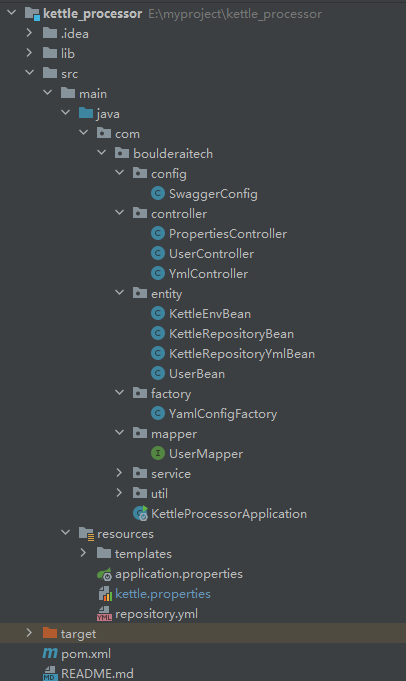
2、技术栈
Spring Boot+Swagger+Lombok+Hutool
3、项目地址
https://gitee.com/ljhahu/kettle_processor.git
需要权限请联系:liujinhui-ahu@foxmail.com
二、properties配置与使用
1、默认配置文件
(1)配置-application.properties
- # Spring Boot端口配置
- server.port=9088
- # spring数据源配置
- spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
- spring.datasource.password=qaz123
- spring.datasource.username=root
- spring.datasource.url=jdbc:mysql://192.168.40.111:3306/visualization?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&useSSL=true
- spring.thymeleaf.prefix=classpath:/templates/
复制代码 (2)读取
Spring Boot自己会读取,配置数据源、thymeleaf等信息
2、自定义配置文件
(1)配置-kettle.properties
- # properties https://blog.csdn.net/weixin_42352733/article/details/121830775
- environment=xuelei-www
- kettle.repository.type=database
- kettle.repository.username=admin
- kettle.repository.password=admin
复制代码 (2)使用-读取单个值-PropertiesController.java
- package com.boulderaitech.controller;
- import com.boulderaitech.entity.KettleRepositoryBean;
- import io.swagger.annotations.Api;
- import io.swagger.annotations.ApiImplicitParam;
- import io.swagger.annotations.ApiOperation;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.beans.factory.annotation.Value;
- import org.springframework.context.annotation.PropertySource;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RestController;
- @Api("properties测试")
- @RestController //Controller和RestController的区别
- @PropertySource("classpath:kettle.properties") //默认是application.properties
- //可以将PropertySource注解加入entity,也可以加入controller将bean注入
- public class PropertiesController {
- @Value("${environment}")
- private String envName;
- @Autowired
- private KettleRepositoryBean kettleRepositoryBean;
- @RequestMapping("/getEnv")
- @ApiOperation("properties方式获取当前的环境")
- public String getEnv() {
- return "hello " + envName;
- }
- @ApiOperation("properties方式获取当前的环境")
- @RequestMapping("/getRepoInfo")
- public String getRepoInfo() {
- return "hello " + kettleRepositoryBean.toString();
- }
- }
复制代码 (3)使用-读取多个值到对象-KettleRepositoryBean.java
- package com.boulderaitech.entity;
- import lombok.AllArgsConstructor;
- import lombok.Data;
- import lombok.NoArgsConstructor;
- import org.springframework.boot.context.properties.ConfigurationProperties;
- import org.springframework.stereotype.Component;
- @Data
- @Component
- @NoArgsConstructor
- @AllArgsConstructor
- @ConfigurationProperties(prefix = "kettle.repository")
- public class KettleRepositoryBean {
- private String type;
- private String username;
- private String password;
- }
复制代码 三、yml配置
1、默认配置文件
application.yml
2、自定义配置文件
(1)配置-repository.yml
- kettle:
- repository:
- repo1:
- type: postgresql
- ip_addr: 192.168.4.68
- port: 5432
- username: admin
- password: admin
- db_name: kettle
- version: 8.0.0
复制代码 (2)实现任意位置读取的工厂类-YamlConfigFactory.java
- package com.boulderaitech.factory;
- import org.springframework.beans.factory.config.YamlPropertiesFactoryBean;
- import org.springframework.core.env.PropertiesPropertySource;
- import org.springframework.core.env.PropertySource;
- import org.springframework.core.io.support.DefaultPropertySourceFactory;
- import org.springframework.core.io.support.EncodedResource;
- import java.io.IOException;
- import java.util.Properties;
- /**
- * 在任意位置读取指定的yml文件
- * 参考:https://blog.csdn.net/weixin_45168162/article/details/125427465
- */
- public class YamlConfigFactory extends DefaultPropertySourceFactory {
- //继承父类,可以重载父类方法@Override
- //实现接口,重写方法@Override
- @Override
- public PropertySource<?> createPropertySource(String name, EncodedResource resource) throws IOException {
- String sourceName = name != null ? name : resource.getResource().getFilename();
- if (!resource.getResource().exists()) {
- return new PropertiesPropertySource(sourceName, new Properties());
- } else if (sourceName.endsWith(".yml") || sourceName.endsWith(".yaml")) {
- Properties propertiesFromYaml = loadYml(resource);
- return new PropertiesPropertySource(sourceName, propertiesFromYaml);
- } else {
- return super.createPropertySource(name, resource);
- }
- }
- private Properties loadYml(EncodedResource resource) throws IOException {
- YamlPropertiesFactoryBean factory = new YamlPropertiesFactoryBean();
- factory.setResources(resource.getResource());
- factory.afterPropertiesSet();
- return factory.getObject();
- }
- }
复制代码 (3)使用-读取单个值-KettleEnvBean.java
- package com.boulderaitech.entity;
- import com.boulderaitech.factory.YamlConfigFactory;
- import lombok.AllArgsConstructor;
- import lombok.Data;
- import lombok.NoArgsConstructor;
- import org.springframework.beans.factory.annotation.Value;
- import org.springframework.context.annotation.PropertySource;
- import org.springframework.stereotype.Component;
- @Data
- @Component
- @AllArgsConstructor
- @NoArgsConstructor
- @PropertySource(value = "classpath:repository.yml",factory = YamlConfigFactory.class)//需要通过工厂加载指定的配置文件
- public class KettleEnvBean {
- @Value("${kettle.version}")
- private String kettleVersion;
- }
复制代码 (4)使用-读取多个值到对象-KettleRepositoryYmlBean.java
- package com.boulderaitech.entity;
- import com.boulderaitech.factory.YamlConfigFactory;
- import lombok.AllArgsConstructor;
- import lombok.Data;
- import lombok.NoArgsConstructor;
- import org.springframework.boot.context.properties.ConfigurationProperties;
- import org.springframework.context.annotation.PropertySource;
- import org.springframework.stereotype.Component;
- @Data
- @AllArgsConstructor
- @NoArgsConstructor
- @PropertySource(value = "classpath:repository.yml",factory = YamlConfigFactory.class)//需要通过工厂加载指定的配置文件
- @ConfigurationProperties(prefix = "kettle.repository.repo1") //需要添加spring boot的注解,才可以使用
- //思考:能否通过反射操作修改注解的参数
- @Component()
- public class KettleRepositoryYmlBean {
- private String type;
- private String ip_addr; //命名只能用下划线
- private String username;
- private String password;
- private String port;
- private String db_name;
- }
复制代码 (5)使用-YmlController.java
- package com.boulderaitech.controller;
- import com.boulderaitech.entity.KettleEnvBean;
- import com.boulderaitech.entity.KettleRepositoryYmlBean;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.beans.factory.annotation.Value;
- import org.springframework.context.annotation.PropertySource;
- import org.springframework.stereotype.Controller;
- import org.springframework.web.bind.annotation.GetMapping;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RequestMethod;
- import org.springframework.web.bind.annotation.RestController;
- @RestController //使用controller返回的结果会按照template进行解析
- //使用RestController则会返回对应的字符串
- public class YmlController {
- @Autowired
- private KettleRepositoryYmlBean kettleRepositoryYmlBean;
- @Autowired
- private KettleEnvBean kettleEnvBean;
- @RequestMapping(value = "/getKettleVersion") //默认是get
- public String getKettleVersion() {
- return "hello " + kettleEnvBean.getKettleVersion();
- }
- // @GetMapping("/getRepoInfoYml"),不支持get方法
- //@RequestMapping(value = "/getRepoInfoYml", method = RequestMethod.POST)
- @RequestMapping(value = "/getRepoInfoYml")
- public String getRepoInfoYml() {
- return "hello " + kettleRepositoryYmlBean.toString();
- }
- }
复制代码
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |