一、Schema 文件简介
使用 Room Migration 升级数据库 , 需要根据当前数据库版本和目标版本编写一系列 Migration 迁移类 , 并生成一个升级的 Schema 文件 , 该文件是 json 格式的文件 , 此中包含如下内容 :
- 版本信息 : 包括 当前版本 和 目标版本 ;
- 创建表语句 : 包括 新增的表的 定义 和 字段信息 ;
- 删除表语句 : 包括 需要删除的 表的名称 ;
- 修改表语句 : 包括 需要修改的表的名称 和 需要修改的字段的定义信息 ;
- 插入数据语句 : 包括 需要插入数据的表的名称 和 插入的数据 ;
- 删除数据语句 : 包括 需要删除数据的表的名称 和 删除的条件 ;
Schema 文件是 描述 Room 数据库布局的文件 , 通过该文件 , 可以 很方便地开发者了解数据库的汗青变动记录 , 方便排盘问题 ;
Schema 文件 定义了数据库中的表、列、索引等元素的布局 , 并包含了创建和升级数据库的 SQL 脚本 ;
使用 Room 的 Migration 升级数据库 , 生成的 Schema 文件的方式通常是通过 Gradle 构建脚本中的 roomExportSchema 使命,它会将 Schema 文件导出到指定的目次中 , 该目次需要在 build.gradle 构建脚本中配置 ;
二、生成 Schema 文件配置
在进行 Room 数据库升级时 , 可以使用 Room Migration 工具生成 Schema 文件 ;
如果想要 导出 Schema 文件 , 需要在 RoomDatabase 实现类的 @Database 注解中 , 设置 exportSchema = true 参数 ;
- @Database(entities = [Student::class], version = 3, exportSchema = true)
- abstract class StudentDatabase: RoomDatabase() {
复制代码 在之前的博客中 , exportSchema 参数都设置为了 false , 没有导出 Schema 文件 ;
此外 , 还要在 build.gradle 构建脚本中 配置 Schema 文件的生成位置 , 在 " android / defaultConfig / javaCompileOptions / annotationProcessorOptions " 层级中的 arguments 中 , 配置 "room.schemaLocation": "$projectDir/schemas".toString() 参数 ;
完整的配置层级如下 :
- android {
- namespace 'kim.hsl.rvl'
- compileSdk 32
- defaultConfig {
- applicationId "kim.hsl.rvl"
- minSdk 21
- targetSdk 32
- javaCompileOptions {
- annotationProcessorOptions {
- // 指定 Room Migration 升级数据库导出的 Schema 文件位置
- arguments = ["room.schemaLocation": "$projectDir/schemas".toString()]
- }
- }
- }
- }
复制代码 projectDir 是 Module 模块的根目次 , 生成的 schemas 目次 , 与 src , build , build.gradle 是一个级别的文件 ;
三、生成 Schema 文件过程
1、数据库版本 1 - 首次运行应用
运行数据库版本 1 的应用 , 首次运行 ,
- fun inst(context: Context): StudentDatabase {
- if (!::instance.isInitialized) {
- synchronized(StudentDatabase::class) {
- // 创建数据库
- instance = Room.databaseBuilder(
- context.applicationContext,
- StudentDatabase::class.java,
- "student_database.db")
- //.addMigrations(MIGRATION_1_2)
- //.addMigrations(MIGRATION_2_3)
- //.fallbackToDestructiveMigration()
- .allowMainThreadQueries() // Room 原则上不允许在主线程操作数据库
- // 如果要在主线程操作数据库需要调用该函数
- .build()
- }
- }
- return instance;
- }
复制代码 将 Entity 实体类中 新增的字段 注释掉 ;
- /**
- * 性别字段
- * 数据库表中的列名为 sex
- * 数据库表中的类型为 INTEGER 文本类型
- */
- /*@ColumnInfo(name = "sex", typeAffinity = ColumnInfo.INTEGER)
- var sex: Int = 0*/
- /**
- * degree字段
- * 数据库表中的列名为 sex
- * 数据库表中的类型为 INTEGER 文本类型
- */
- /*@ColumnInfo(name = "degree", typeAffinity = ColumnInfo.INTEGER)
- var degree: Int = 0*/
复制代码 将数据块版本设置为 1 :
- @Database(entities = [Student::class], version = 1, exportSchema = true)
- abstract class StudentDatabase: RoomDatabase() {
复制代码 首次运行应用 , 生成 数据库版本 1 的 Schema 文件 1.json ;
Schema 文件的生成位置是 " app/schemes/包名/1.json " 路径 ;
- {
- "formatVersion": 1,
- "database": {
- "version": 1,
- "identityHash": "acca4b709e6c8b9b88d8328be36b9032",
- "entities": [
- {
- "tableName": "student",
- "createSql": "CREATE TABLE IF NOT EXISTS `${TABLE_NAME}` (`id` INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, `name` TEXT, `age` INTEGER NOT NULL)",
- "fields": [
- {
- "fieldPath": "id",
- "columnName": "id",
- "affinity": "INTEGER",
- "notNull": true
- },
- {
- "fieldPath": "name",
- "columnName": "name",
- "affinity": "TEXT",
- "notNull": false
- },
- {
- "fieldPath": "age",
- "columnName": "age",
- "affinity": "INTEGER",
- "notNull": true
- }
- ],
- "primaryKey": {
- "columnNames": [
- "id"
- ],
- "autoGenerate": true
- },
- "indices": [],
- "foreignKeys": []
- }
- ],
- "views": [],
- "setupQueries": [
- "CREATE TABLE IF NOT EXISTS room_master_table (id INTEGER PRIMARY KEY,identity_hash TEXT)",
- "INSERT OR REPLACE INTO room_master_table (id,identity_hash) VALUES(42, 'acca4b709e6c8b9b88d8328be36b9032')"
- ]
- }
- }
复制代码
2、数据库版本 1 升级至 数据库版本 2 - 第二次运行应用
起首 , 设置 Entity 实体类中的字段 , 在 数据库版本 1 的根本上 , 添加 sex 字段 ;
- /**
- * 性别字段
- * 数据库表中的列名为 sex
- * 数据库表中的类型为 INTEGER 文本类型
- */
- @ColumnInfo(name = "sex", typeAffinity = ColumnInfo.INTEGER)
- var sex: Int = 0
复制代码 然后 , 定义 数据库 版本 1 升级为 数据库 版本 2 的 Migration 迁移类 ,
- /**
- * 数据库版本 1 升级到 版本 2 的迁移类实例对象
- */
- val MIGRATION_1_2: Migration = object : Migration(1, 2) {
- override fun migrate(database: SupportSQLiteDatabase) {
- Log.i("Room_StudentDatabase", "数据库版本 1 升级到 版本 2")
- database.execSQL("alter table student add column sex integer not null default 1")
- }
- }
复制代码 再后 , 调用 RoomDatabase.Builder#addMigrations 添加上述定义的 Migration 迁移类 ;
- fun inst(context: Context): StudentDatabase {
- if (!::instance.isInitialized) {
- synchronized(StudentDatabase::class) {
- // 创建数据库
- instance = Room.databaseBuilder(
- context.applicationContext,
- StudentDatabase::class.java,
- "student_database.db")
- .addMigrations(MIGRATION_1_2)
- //.addMigrations(MIGRATION_2_3)
- //.fallbackToDestructiveMigration()
- .allowMainThreadQueries() // Room 原则上不允许在主线程操作数据库
- // 如果要在主线程操作数据库需要调用该函数
- .build()
- }
- }
- return instance;
- }
复制代码 末了 , 修改数据库版本 为 2 ,
- @Database(entities = [Student::class], version = 2, exportSchema = true)
- abstract class StudentDatabase: RoomDatabase() {
复制代码
生成的 数据库 版本 1 升级到 数据库 版本 2 的 Schema 文件如下 :
- {
- "formatVersion": 1,
- "database": {
- "version": 2,
- "identityHash": "84fb235f8062b0a6b0c8d1a6d1035c4f",
- "entities": [
- {
- "tableName": "student",
- "createSql": "CREATE TABLE IF NOT EXISTS `${TABLE_NAME}` (`id` INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, `name` TEXT, `age` INTEGER NOT NULL, `sex` INTEGER NOT NULL)",
- "fields": [
- {
- "fieldPath": "id",
- "columnName": "id",
- "affinity": "INTEGER",
- "notNull": true
- },
- {
- "fieldPath": "name",
- "columnName": "name",
- "affinity": "TEXT",
- "notNull": false
- },
- {
- "fieldPath": "age",
- "columnName": "age",
- "affinity": "INTEGER",
- "notNull": true
- },
- {
- "fieldPath": "sex",
- "columnName": "sex",
- "affinity": "INTEGER",
- "notNull": true
- }
- ],
- "primaryKey": {
- "columnNames": [
- "id"
- ],
- "autoGenerate": true
- },
- "indices": [],
- "foreignKeys": []
- }
- ],
- "views": [],
- "setupQueries": [
- "CREATE TABLE IF NOT EXISTS room_master_table (id INTEGER PRIMARY KEY,identity_hash TEXT)",
- "INSERT OR REPLACE INTO room_master_table (id,identity_hash) VALUES(42, '84fb235f8062b0a6b0c8d1a6d1035c4f')"
- ]
- }
- }
复制代码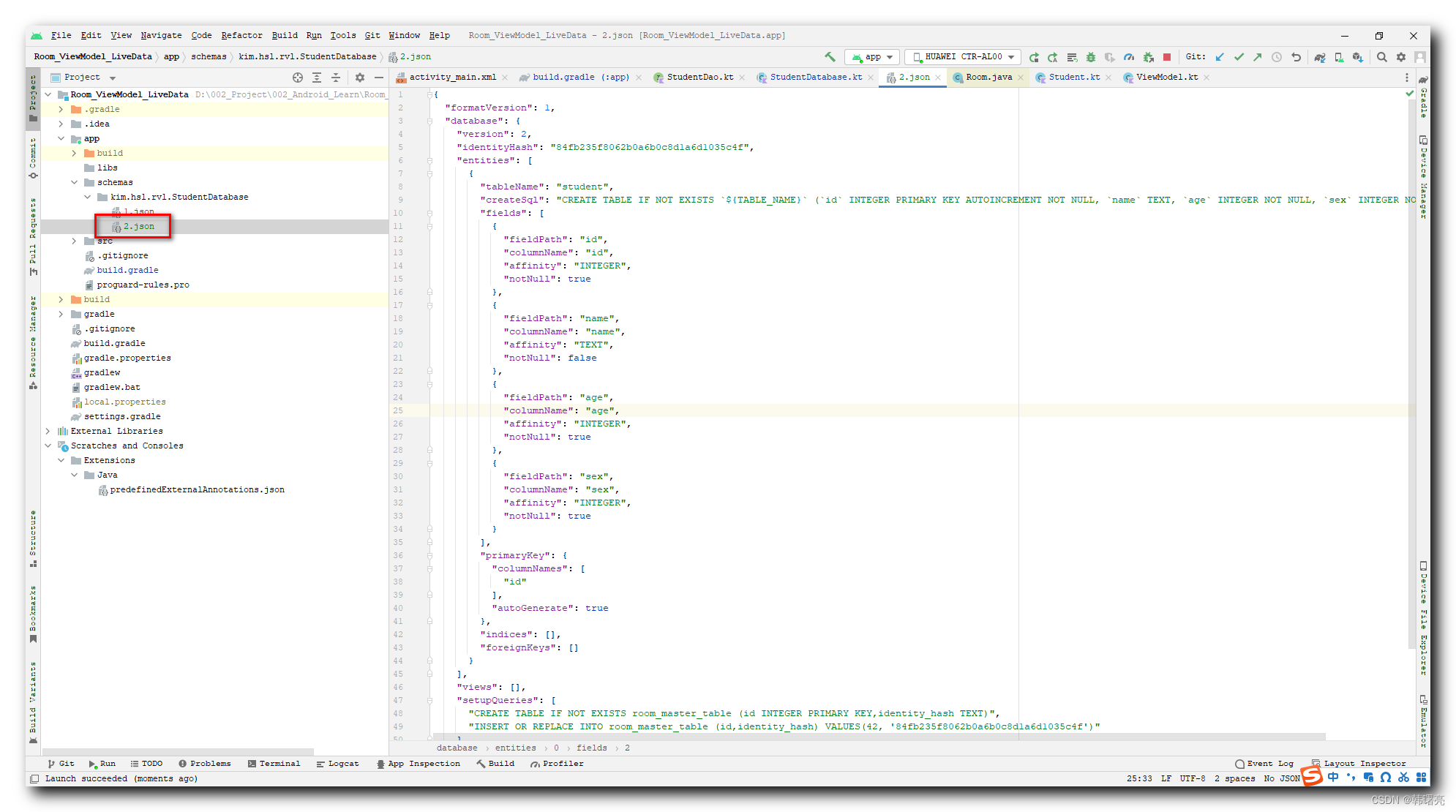
3、数据库版本 2 升级至 数据库版本 3 - 第三次运行应用
起首 , 设置 Entity 实体类中的字段 , 在 数据库版本 2 的根本上 , 添加 degree 字段 ;
- /**
- * 性别字段
- * 数据库表中的列名为 sex
- * 数据库表中的类型为 INTEGER 文本类型
- */
- @ColumnInfo(name = "sex", typeAffinity = ColumnInfo.INTEGER)
- var sex: Int = 0
- /** * degree字段 * 数据库表中的列名为 sex * 数据库表中的类型为 INTEGER 文本类型 */ @ColumnInfo(name = "degree", typeAffinity = ColumnInfo.INTEGER) var degree: Int = 0
复制代码 然后 , 定义 数据库 版本 2 升级为 数据库 版本 3 的 Migration 迁移类 ,
- /**
- * 数据库版本 2 升级到 版本 3 的迁移类实例对象
- */
- val MIGRATION_2_3: Migration = object : Migration(2, 3) {
- override fun migrate(database: SupportSQLiteDatabase) {
- Log.i("Room_StudentDatabase", "数据库版本 2 升级到 版本 3")
- database.execSQL("alter table student add column degree integer not null default 1")
- }
- }
复制代码 再后 , 调用 RoomDatabase.Builder#addMigrations 添加上述定义的 Migration 迁移类 ;
- fun inst(context: Context): StudentDatabase {
- if (!::instance.isInitialized) {
- synchronized(StudentDatabase::class) {
- // 创建数据库
- instance = Room.databaseBuilder(
- context.applicationContext,
- StudentDatabase::class.java,
- "student_database.db")
- .addMigrations(MIGRATION_1_2)
- .addMigrations(MIGRATION_2_3)
- //.fallbackToDestructiveMigration()
- .allowMainThreadQueries() // Room 原则上不允许在主线程操作数据库
- // 如果要在主线程操作数据库需要调用该函数
- .build()
- }
- }
- return instance;
- }
复制代码 末了 , 修改数据库版本 为 3 ,
- @Database(entities = [Student::class], version = 2, exportSchema = true)
- abstract class StudentDatabase: RoomDatabase() {
复制代码
生成的 数据库 版本 2 升级到 数据库 版本 3 的 Schema 文件如下 :
- {
- "formatVersion": 1,
- "database": {
- "version": 3,
- "identityHash": "d88859680a038f5155613d94de7888cc",
- "entities": [
- {
- "tableName": "student",
- "createSql": "CREATE TABLE IF NOT EXISTS `${TABLE_NAME}` (`id` INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, `name` TEXT, `age` INTEGER NOT NULL, `sex` INTEGER NOT NULL, `degree` INTEGER NOT NULL)",
- "fields": [
- {
- "fieldPath": "id",
- "columnName": "id",
- "affinity": "INTEGER",
- "notNull": true
- },
- {
- "fieldPath": "name",
- "columnName": "name",
- "affinity": "TEXT",
- "notNull": false
- },
- {
- "fieldPath": "age",
- "columnName": "age",
- "affinity": "INTEGER",
- "notNull": true
- },
- {
- "fieldPath": "sex",
- "columnName": "sex",
- "affinity": "INTEGER",
- "notNull": true
- },
- {
- "fieldPath": "degree",
- "columnName": "degree",
- "affinity": "INTEGER",
- "notNull": true
- }
- ],
- "primaryKey": {
- "columnNames": [
- "id"
- ],
- "autoGenerate": true
- },
- "indices": [],
- "foreignKeys": []
- }
- ],
- "views": [],
- "setupQueries": [
- "CREATE TABLE IF NOT EXISTS room_master_table (id INTEGER PRIMARY KEY,identity_hash TEXT)",
- "INSERT OR REPLACE INTO room_master_table (id,identity_hash) VALUES(42, 'd88859680a038f5155613d94de7888cc')"
- ]
- }
- }
复制代码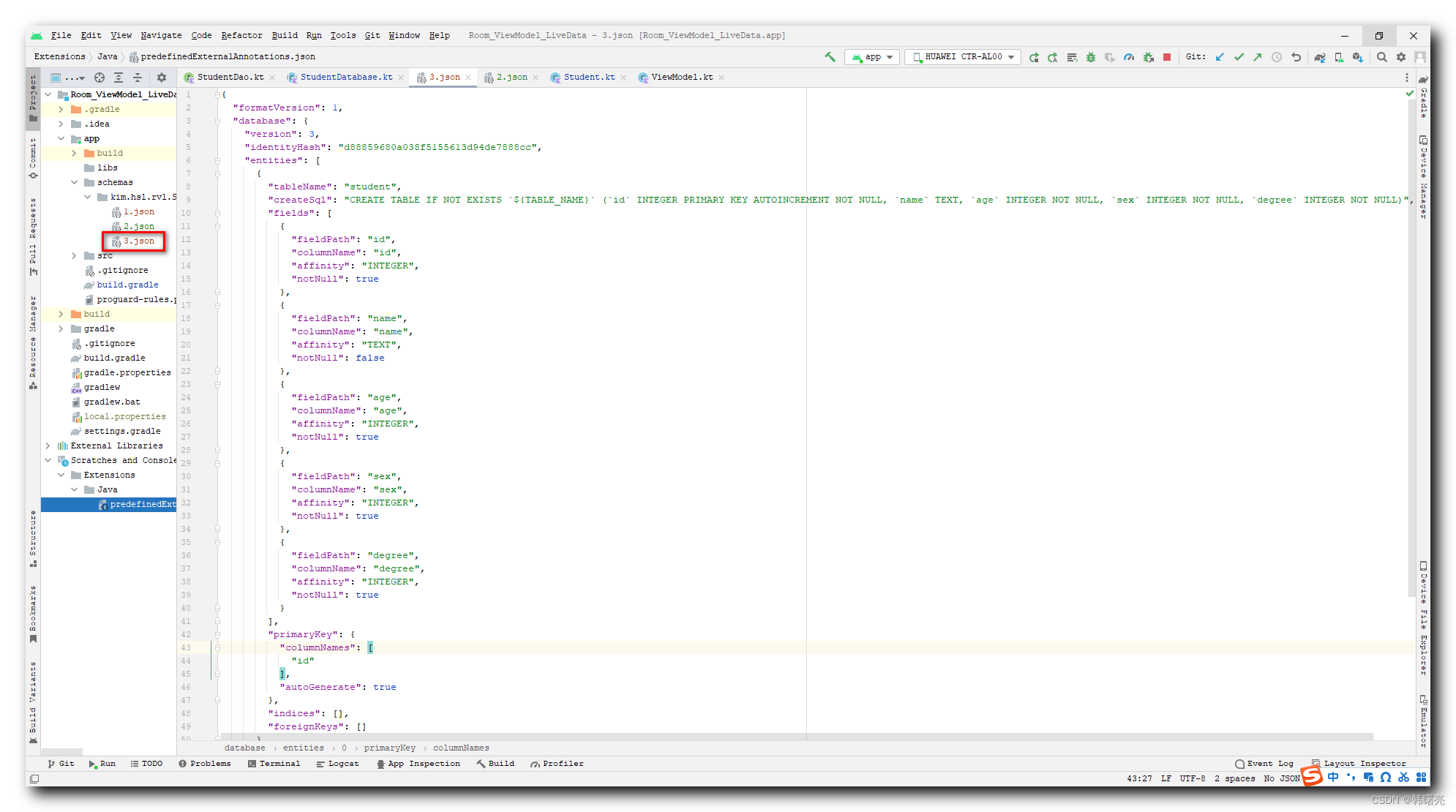
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |