- #include <iostream>
- #include <cstring>
- #include <cstdlib>
- #include <unistd.h>
- #include <sstream>
- #include <vector>
- #include <memory>
- using namespace std;
- // 前向声明武器类
- class Weapon;
- // 英雄基类
- class Hero {
- private:
- int hp; // 生命值
- int atk; // 攻击力
- int def; // 防御力
- int spd; // 速度
- string profession; // 职业类型
- public:
- // 构造函数(默认职业为战士)
- Hero(int h = 0, int a = 0, int d = 0, int s = 0, string p = "Warrior")
- : hp(h), atk(a), def(d), spd(s), profession(p) {}
- // 属性设置方法
- void setAtk(int a) { atk = a; }
- void setDef(int d) { def = d; }
- void setSpd(int s) { spd = s; }
- void setHp(int h) { hp = h; }
- void setProfession(string p) { profession = p; }
- // 属性获取方法
- int getAtk() { return atk; }
- int getDef() { return def; }
- int getSpd() { return spd; }
- int getHp() { return hp; }
- string getProfession() const { return profession; }
- // 装备武器方法(通过多态调用不同武器的属性加成)
- void equipWeapon(Weapon* w);
- };
- // 武器基类(抽象类)
- class Weapon {
- private:
- int atk; // 基础攻击力
- public:
- // 构造函数(默认攻击力为1)
- Weapon(int atk = 1) : atk(atk) {}
- // 属性设置方法
- void setAtk(int a) { atk = a; }
- int getAtk() { return atk; }
- // 基础属性加成方法(所有武器都增加攻击力)
- virtual void getAttr(Hero& hero) {
- int new_atk = hero.getAtk() + atk;
- hero.setAtk(new_atk);
- }
- // 纯虚函数,用于获取武器类型
- virtual string getType() = 0;
- virtual ~Weapon() {} // 虚析构函数
- };
- // 长剑类(继承自武器)
- class Sword : public Weapon {
- private:
- int hp; // 额外生命加成
- public:
- // 构造函数
- Sword(int atk = 1, int hp = 1) : Weapon(atk), hp(hp) {}
- // 设置/获取额外生命值
- void setHp(int h) { hp = h; }
- int getHp() { return hp; }
- // 重写属性加成方法(攻击+生命)
- virtual void getAttr(Hero& hero) {
- Weapon::getAttr(hero); // 调用基类攻击加成
- int new_hp = hero.getHp() + hp;
- hero.setHp(new_hp);
- }
- // 重写获取武器类型
- string getType() override { return "Sword"; }
- };
- // 匕首类(继承自武器)
- class Blade : public Weapon {
- private:
- int spd; // 额外速度加成
- public:
- // 构造函数
- Blade(int atk = 1, int spd = 1) : Weapon(atk), spd(spd) {}
- // 设置/获取额外速度值
- void setSpd(int s) { spd = s; }
- int getSpd() { return spd; }
- // 重写属性加成方法(攻击+速度)
- virtual void getAttr(Hero& hero) {
- Weapon::getAttr(hero); // 调用基类攻击加成
- int new_spd = hero.getSpd() + spd;
- hero.setSpd(new_spd);
- }
- // 重写获取武器类型
- string getType() override { return "Blade"; }
- };
- // 英雄装备武器的具体实现
- void Hero::equipWeapon(Weapon* w) {
- w->getAttr(*this); // 多态调用对应武器的属性加成
- }
- // 怪物类
- class Monster {
- private:
- int hp; // 怪物生命值
- int atk; // 怪物攻击力
- int level; // 怪物等级
- public:
- // 构造函数
- Monster(int h = 50, int a = 10, int l = 1) : hp(h), atk(a), level(l) {}
- // 受到伤害方法
- void takeDamage(int damage) {
- hp -= damage;
- if (hp < 0) hp = 0; // 确保生命值不为负
- }
- // 判断是否死亡
- bool isDead() const { return hp <= 0; }
- // 根据英雄职业掉落武器
- Weapon* dropWeapon(const Hero& hero) {
- if (!isDead()) return nullptr; // 未死亡不掉落
- int baseAtk = 5 + level * 2; // 基础攻击力计算
-
- // 根据英雄职业掉落不同武器
- if (hero.getProfession() == "Warrior") {
- return new Sword(baseAtk, 10 + level * 3); // 战士掉落长剑
- } else { // 默认假设为刺客
- return new Blade(baseAtk, 5 + level * 2); // 刺客掉落匕首
- }
- }
- };
- int main(int argc, const char** argv) {
- // 创建英雄实例
- Hero warrior(100, 15, 10, 5, "Warrior"); // 战士:高血量高防御
- Hero assassin(80, 20, 5, 15, "Assassin"); // 刺客:高攻速低血量
- // 创建怪物实例
- Monster goblin(50, 8, 1); // 绿色史莱姆(弱小怪物)
- Monster orc(100, 15, 2); // 兽人(较强怪物)
- // 战斗过程
- goblin.takeDamage(warrior.getAtk()); // 战士攻击哥布林
- orc.takeDamage(assassin.getAtk()); // 刺客攻击兽人
- // 掉落武器处理
- if (goblin.isDead()) {
- Weapon* weapon = goblin.dropWeapon(warrior);
- cout << "哥布林掉落了 " << weapon->getType() << endl;
- warrior.equipWeapon(weapon); // 战士装备武器
- delete weapon; // 释放内存
- }
- if (orc.isDead()) {
- Weapon* weapon = orc.dropWeapon(assassin);
- cout << "兽人掉落了 " << weapon->getType() << endl;
- assassin.equipWeapon(weapon); // 刺客装备武器
- delete weapon;
- }
- // 显示最终属性
- cout << "
- 战士属性 - 攻击力:" << warrior.getAtk()
- << " 生命值:" << warrior.getHp() << endl;
- cout << "刺客属性 - 攻击力:" << assassin.getAtk()
- << " 速度:" << assassin.getSpd() << endl;
- return 0;
- }
复制代码
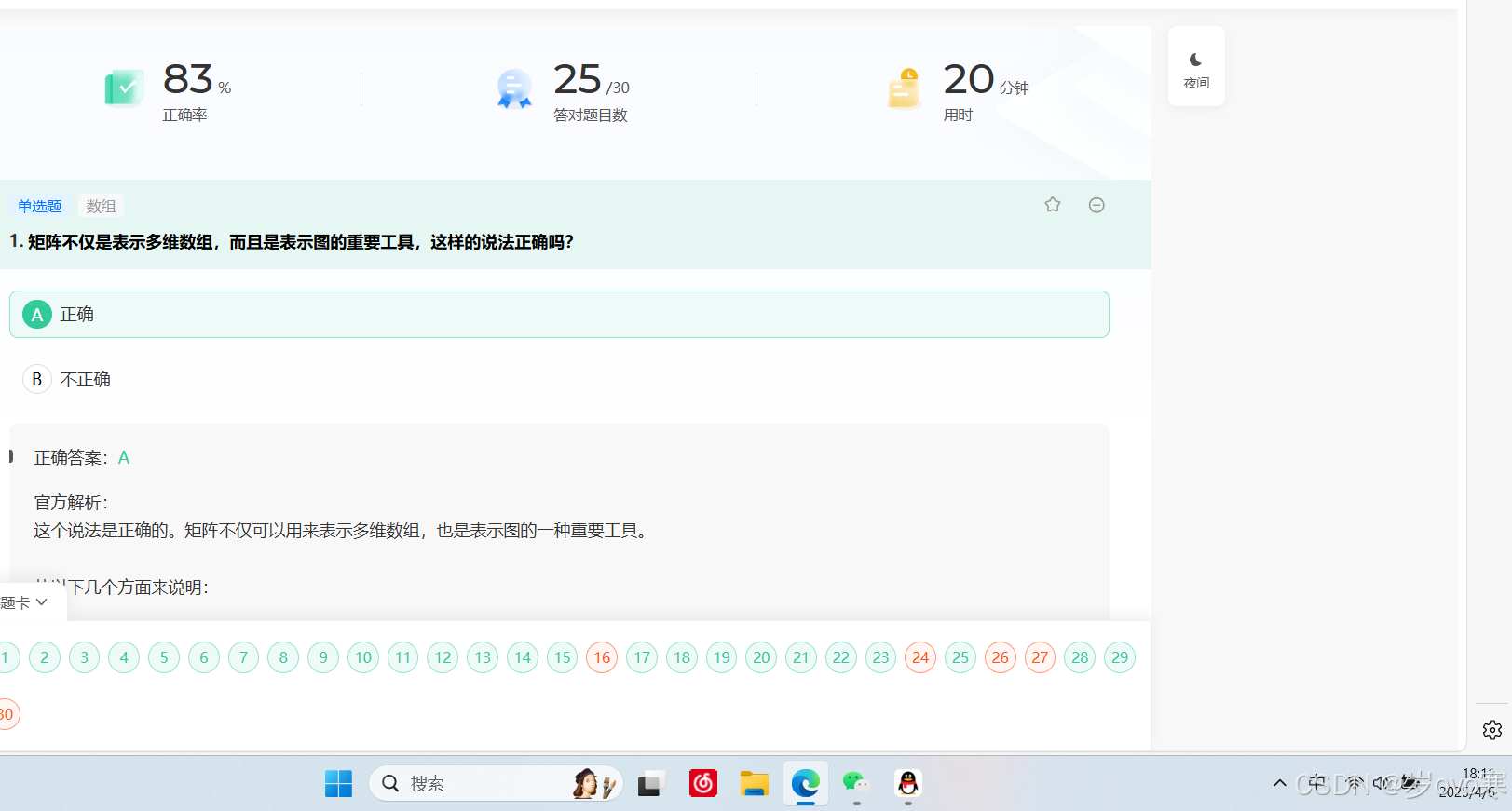
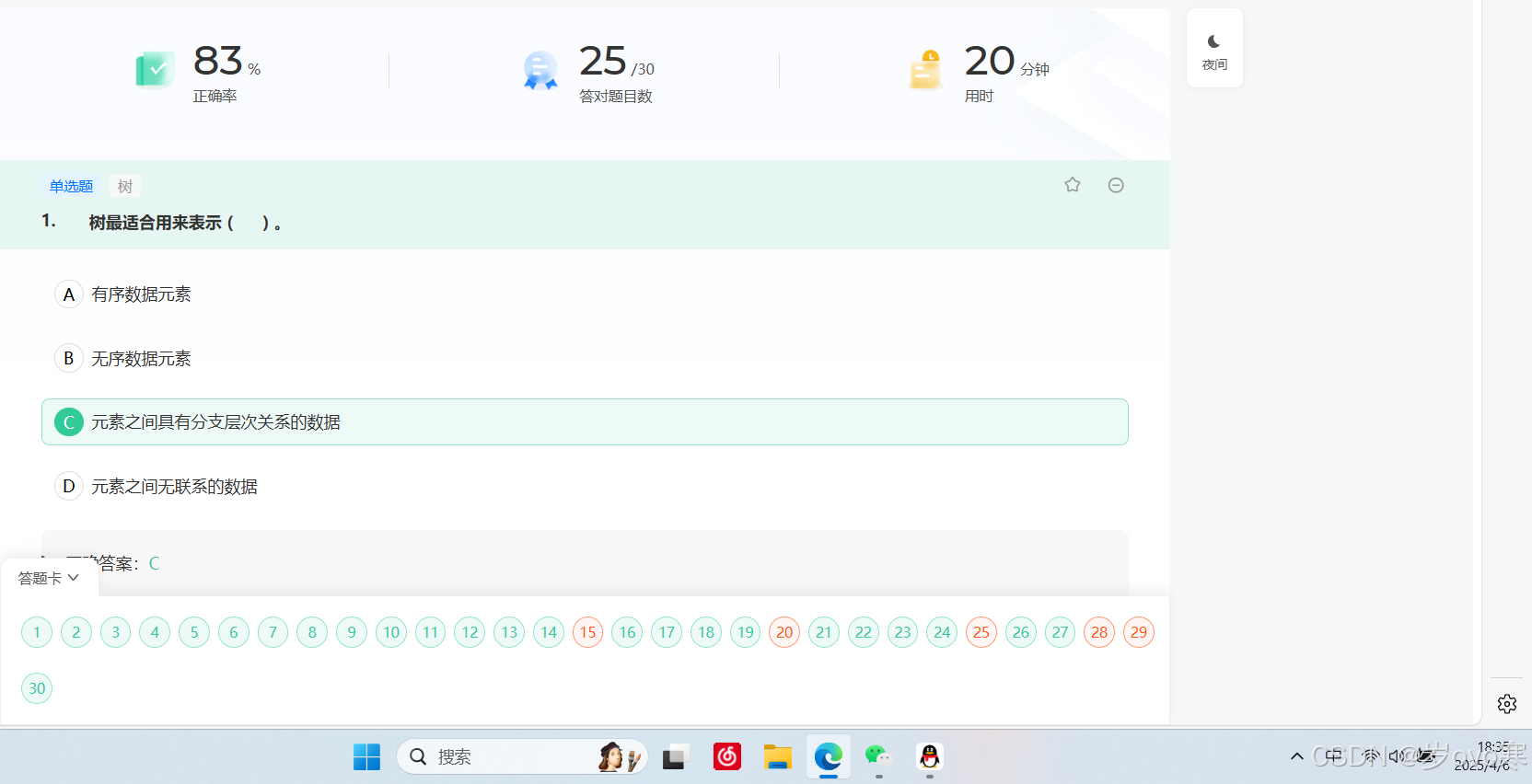
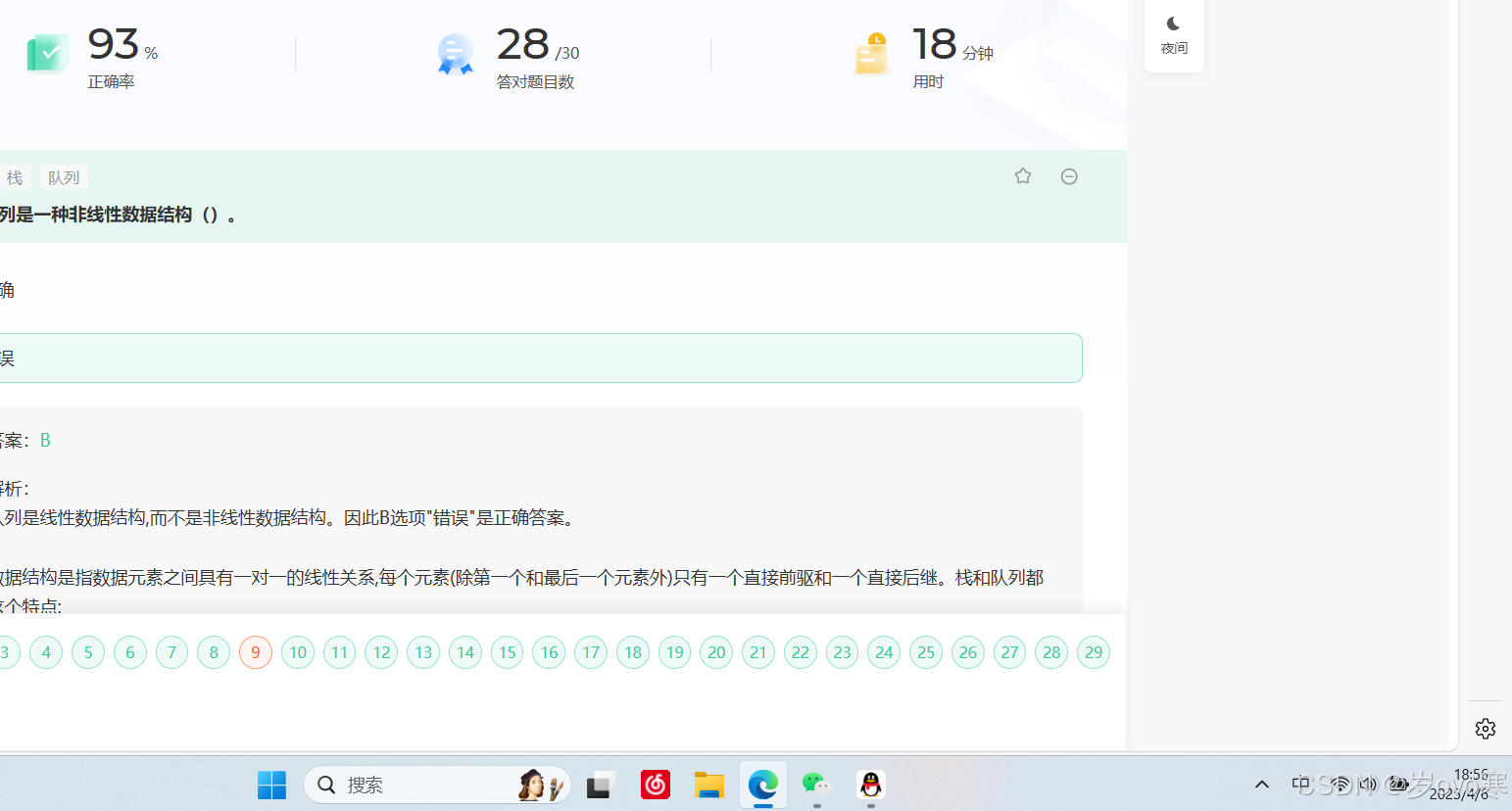
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |