大家好!最近学习完数据库系统,一直在写实战项目-网上书店管理系统。其功能一般包括:图书信息管理、用户信息管理、图书购买、图书订单查看、图书添加、图书维护等等。现在做一个总结。 **源码及搭建教程已经上传至资源!仅供下载学习。下载
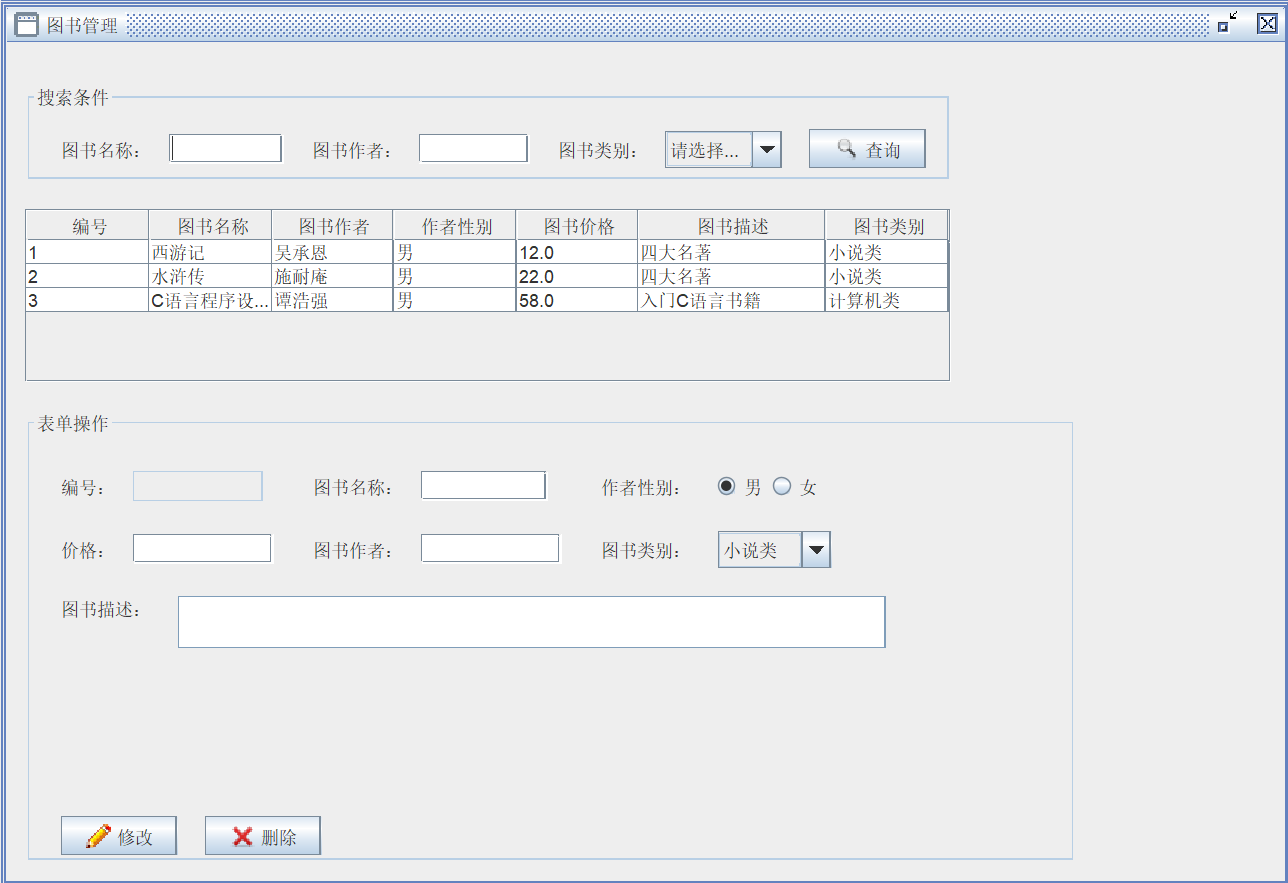
文章目录
1.效果展示
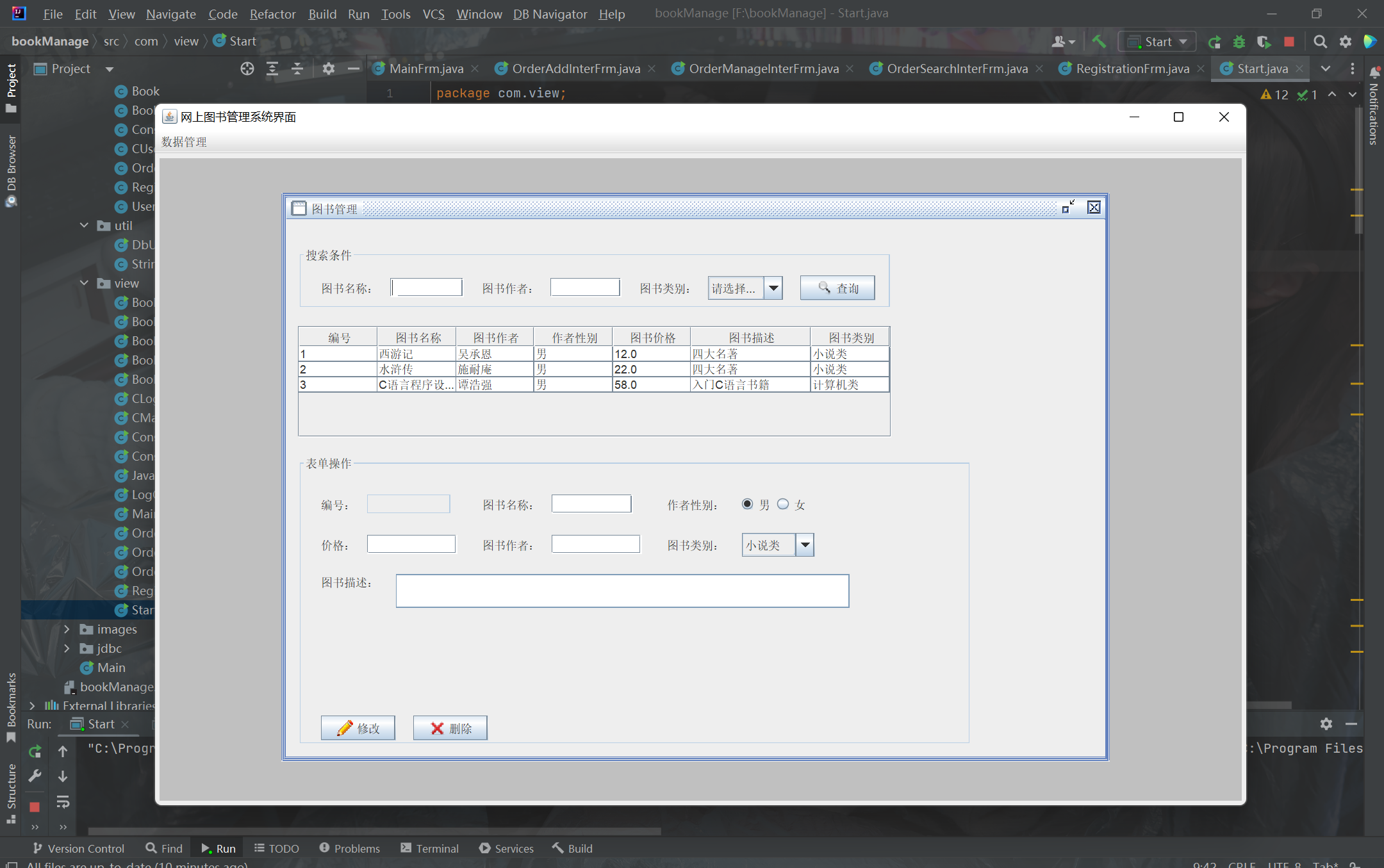 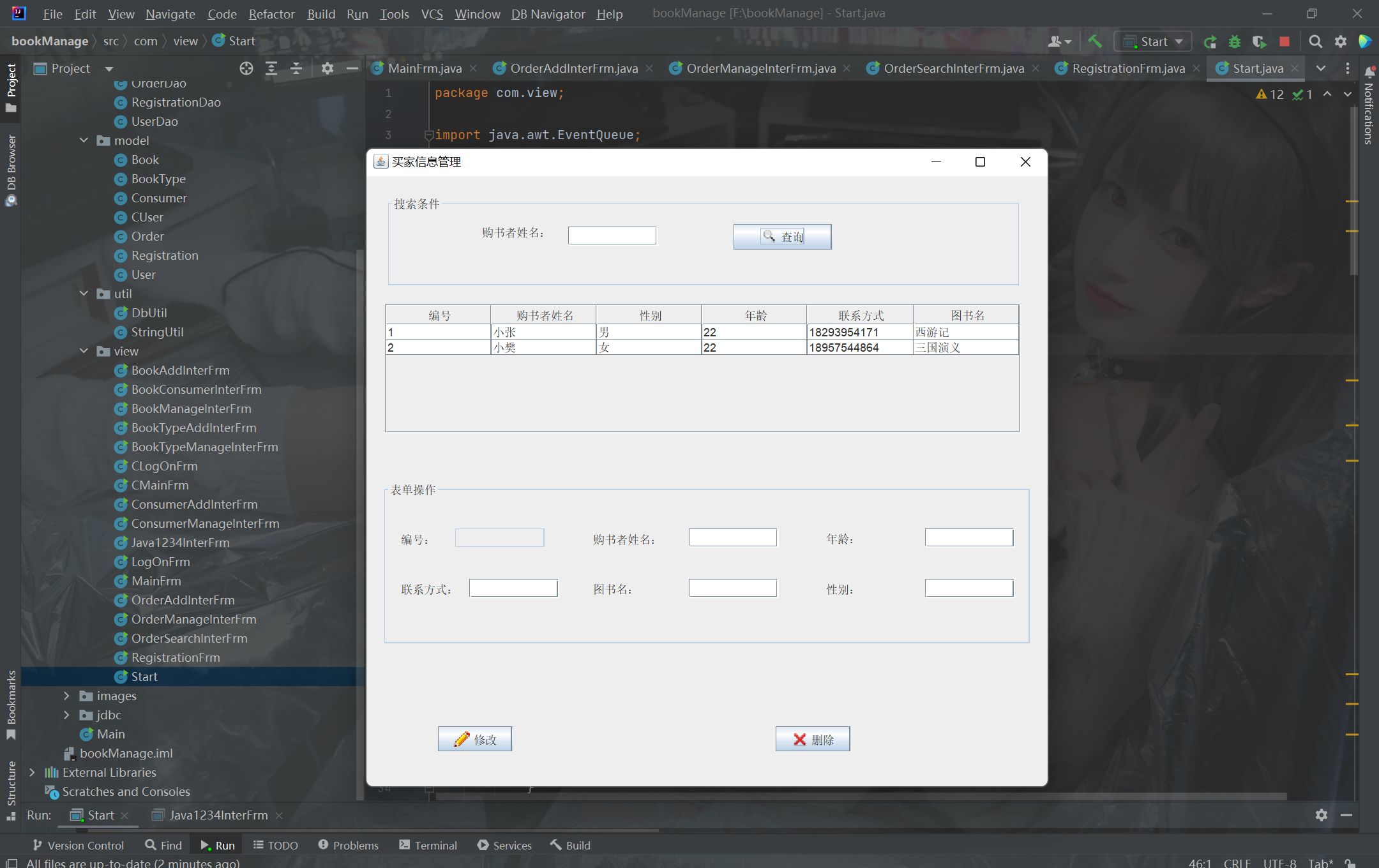
2.需求功能
用户可以进行注册登陆系统,在用户的界面上,其可以进行查看网上书店里的图书类别和所在类别下的图书,根据自己的需求可在订单项目里添加订单购买自己喜欢的图书;
管理员可以通过自己的账号登录到管理员系统对书店进行管理,其可实现对图书的添加,修改,查询,和删除功能,可以查看用户的订单,修改和维护订单。添家客户的信息用以统计数据。
在构造系统时,首先从需求出发构造数据库,然后再由数据库表结合需求划分系统功能模块。这样,就把一个大的系统解成了几个小系统。这里把系统划分为了三个模块:用户登录模块,管理员模块,用户购买模块。模块分别能够实现以下功能:
- 登录模块:实现登录,注册功能。
- 管理员模块:实现对图书的添加修改和删除以及对订单的添加修改和删除功能。
- 用户购买模块:实现对图书的查找以及对所需图书的下单功能。
3.系统总体设计及部分代码
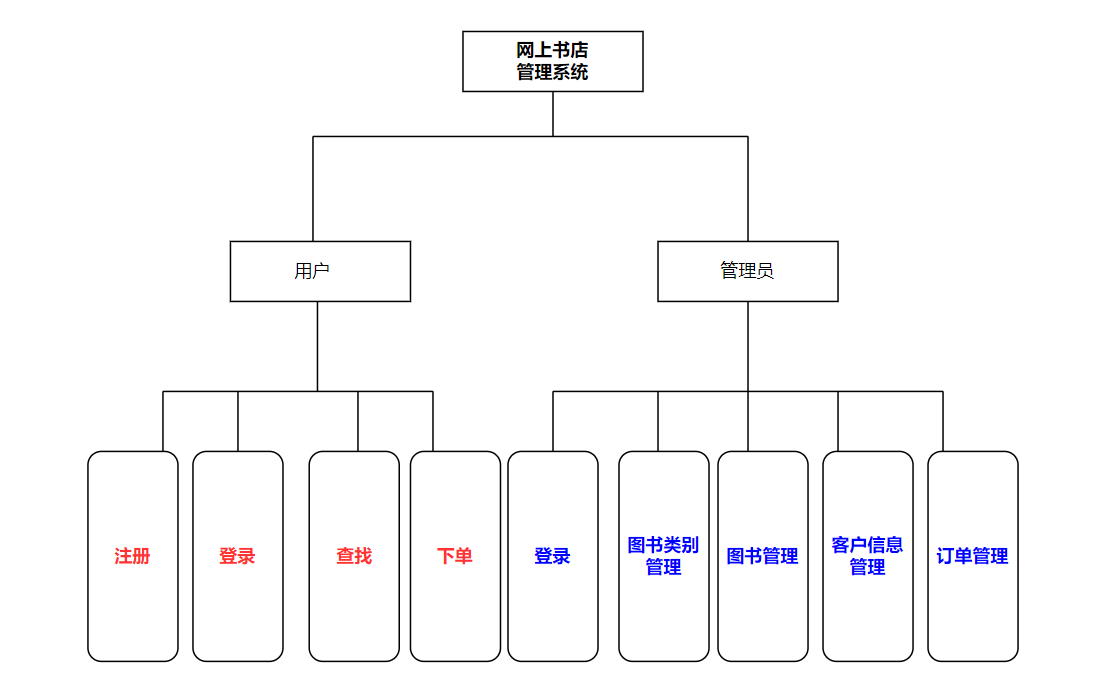
3.1登录模块设计
用户正确输入用户名和密码,连接到数据库,登录成功!
- private void loginActionPerformed(ActionEvent evt) {
- String userName=this.userNameTxt.getText();
- String password=new String(this.passwordTxt.getPassword());
- if(StringUtil.isEmpty(userName)){
- JOptionPane.showMessageDialog(null, "用户名不能为空!");
- return;
- }
- if(StringUtil.isEmpty(password)){
- JOptionPane.showMessageDialog(null, "密码不能为空!");
- return;
- }
- CUser cuser=new CUser(userName,password);
- Connection con=null;
- try {
- con=dbUtil.getCon();
- CUser currentCUser =cuserDao.login(con,cuser);
- if(currentCUser!=null){
- dispose();
- new CMainFrm().setVisible(true);
- }else{
- JOptionPane.showMessageDialog(null, "用户名或者密码错误!");
- }
-
- } catch (Exception e) {
- // TODO 自动生成的 catch 块
- e.printStackTrace();
- }
复制代码 3.2新用户的注册
此模块的核心是创建实例化对象。
- private void registrationActionPerformed(ActionEvent evt) {
- String userName=this.userNameTxt.getText();
- String password=this.passwordTxt.getText();
- if(StringUtil.isEmpty(userName)){
- JOptionPane.showMessageDialog(null, "用户名不能为空!");
- return;
- }
- if(StringUtil.isEmpty(password)){
- JOptionPane.showMessageDialog(null, "密码不能为空!");
- return;
- }
- Registration registration= new Registration(userName,password);
- Connection con= null;
- try {
- con=dbUtil.getCon();
- int n= registrationDao.add(con, registration);
- if(n==1){
- JOptionPane.showMessageDialog(null, "注册成功!");
- resetValue();
- }else{
- JOptionPane.showMessageDialog(null, "注册失败!");
- }
- }catch(Exception e) {
- }finally {
- try {
- dbUtil.closeCon(con);
- } catch (Exception e) {
- // TODO 自动生成的 catch 块
- e.printStackTrace();
- JOptionPane.showMessageDialog(null, "注册失败!");
- }
- }
- }
复制代码 3.3图书添加模块
管理员在此界面上可对系统里的图书进行查询修改和删除。
- public static void main(String[] args) {
- EventQueue.invokeLater(new Runnable() {
- public void run() {
- try {
- BookAddInterFrm frame = new BookAddInterFrm();
- frame.setVisible(true);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
- });
- }
复制代码 3.4图书添加事件
此界面主要实现图书的添加功能。
- /**
- *图书添加事件
- */
- private void bookAddActionPerformed(ActionEvent evt) {
- String bookName=this.bookNameTxt.getText();
- String author=this.authorTxt.getText();
- String price=this.priceTxt.getText();
- String bookDesc=this.bookDescTxt.getText();
- if(StringUtil.isEmpty(bookName)){
- JOptionPane.showMessageDialog(null, "图书名称不能为空!");
- return;
- }
- if(StringUtil.isEmpty(author)){
- JOptionPane.showMessageDialog(null, "图书作者不能为空!");
- return;
- }
- if(StringUtil.isEmpty(price)){
- JOptionPane.showMessageDialog(null, "图书价格不能为空!");
- return;
- }
- String sex="";
- if(manJrb.isSelected()){
- sex="男";
- }else if(femaleJrb.isSelected()){
- sex="女";
- }
- BookType bookType=(BookType) bookTypeJcb.getSelectedItem();
- int bookTypeId=bookType.getId();
- Book book=new Book(bookName,author, sex, Float.parseFloat(price) , bookTypeId, bookDesc);
- Connection con=null;
- try{
- con=dbUtil.getCon();
- int addNum=bookDao.add(con, book);
- if(addNum==1){
- JOptionPane.showMessageDialog(null, "图书添加成功!");
- resetValue();
- }else{
- JOptionPane.showMessageDialog(null, "图书添加失败!");
- }
- }catch(Exception e){
- e.printStackTrace();
- JOptionPane.showMessageDialog(null, "图书添加失败!");
- }finally{
- try {
- dbUtil.closeCon(con);
- } catch (Exception e) {
- // TODO Auto-generated catch block
- e.printStackTrace();
- }
- }
- }
- /**
- * 重置表单
- */
- private void resetValue(){
- this.bookNameTxt.setText("");
- this.authorTxt.setText("");
- this.priceTxt.setText("");
- this.manJrb.setSelected(true);
- this.bookDescTxt.setText("");
- if(this.bookTypeJcb.getItemCount()>0){
- this.bookTypeJcb.setSelectedIndex(0);
- }
- }
- /**
- * 初始化图书类别下拉框
- */
- private void fillBookType(){
- Connection con=null;
- BookType bookType=null;
- try{
- con=dbUtil.getCon();
- ResultSet rs=bookTypeDao.list(con, new BookType());
- while(rs.next()){
- bookType=new BookType();
- bookType.setId(rs.getInt("id"));
- bookType.setBookTypeName(rs.getString("bookTypeName"));
- this.bookTypeJcb.addItem(bookType);
- }
- }catch(Exception e){
- e.printStackTrace();
- }finally{
- }
- }
- }
复制代码 3.5买家信息维护
此模块主要用于对买家信息的查找和维护。
- /**
- * 买家信息搜索事件处理
- */
- protected void consumerSerachActionPerformed(ActionEvent evt) {
- String s_consumerName= this.s_consumerNameTxt.getText();
- Consumer consumer=new Consumer();
- consumer.setConsumerName(s_consumerName);
- this.fillTable(consumer);
- }
- private void fillTable(Consumer consumer){
- DefaultTableModel dtm=(DefaultTableModel) consumerTable.getModel();
- dtm.setRowCount(0); // 设置成0行
- Connection con=null;
- try{
- con=dbUtil.getCon();
- ResultSet rs=consumerDao.list(con, consumer);
- while(rs.next()){
- Vector v=new Vector();
- v.add(rs.getString("id"));
- v.add(rs.getString("consumerName"));
- v.add(rs.getString("sex"));
- v.add(rs.getString("age"));
- v.add(rs.getString("number"));
- v.add(rs.getString("bookName"));
- dtm.addRow(v);
- }
- }catch(Exception e){
- e.printStackTrace();
- }finally {
- try {
- dbUtil.closeCon(con);
- } catch (Exception e) {
- // TODO 自动生成的 catch 块
- e.printStackTrace();
- }
- }
- }
- /**
- * 买家信息修改
- */
- private void consumerUpdateActionEvet(ActionEvent evt) {
- String id=idTxt.getText();
- String consumerName=consumerNameTxt.getText();
- String sex=sexTxt.getText();
- String age=ageTxt.getText();
- String number=numberTxt.getText();
- String bookName=bookNameTxt.getText();
- if(StringUtil.isEmpty(id)){
- JOptionPane.showMessageDialog(null, "请选择要修改的记录");
- return;
- }
- if(StringUtil.isEmpty(consumerName)){
- JOptionPane.showMessageDialog(null, "购书者名称不能为空");
- return;
- }
- if(StringUtil.isEmpty(age)){
- JOptionPane.showMessageDialog(null, "年龄不能为空");
- return;
- }
- if(StringUtil.isEmpty(number)){
- JOptionPane.showMessageDialog(null, "联系方式不能为空");
- return;
- }
- if(StringUtil.isEmpty(bookName)){
- JOptionPane.showMessageDialog(null, "图书名称不能为空");
- return;
- }
- if(StringUtil.isEmpty(sex)){
- JOptionPane.showMessageDialog(null, "性别不能为空");
- return;
- }
- Consumer consumer=new Consumer(Integer.parseInt(id),consumerName,sex,age,number,bookName);
- Connection con=null;
- try {
- con=dbUtil.getCon();
- con=dbUtil.getCon();
- int modifyNum=consumerDao.update(con, consumer);
- if(modifyNum==1){
- JOptionPane.showMessageDialog(null, "修改成功");
- this.resetValue();
- this.fillTable(new Consumer());
- }else{
- JOptionPane.showMessageDialog(null, "修改失败");
- }
- }catch(Exception e) {
- e.printStackTrace();
- JOptionPane.showMessageDialog(null, "修改失败");
- }finally {
- try {
- dbUtil.closeCon(con);
- } catch (Exception e) {
- // TODO 自动生成的 catch 块
- e.printStackTrace();
- }
- }
- }
复制代码 3.6订单管理模块
此模块用于图书订单管理,查找,修改,删除等功能的实现。
- /**
- * 订单修改事件
- */
- protected void orderUpdateActionPerformed(ActionEvent evt) {
- String id=this.idTxt.getText();
- if(StringUtil.isEmpty(id)){
- JOptionPane.showMessageDialog(null, "请选择要修改的记录");
- return;
- }
- String addressee=this.addresseeTxt.getText();
- String number=this.numberTxt.getText();
- String deliveryMent=this.deliveryMentTxt.getText();
- String paymentMethod=this.paymentMethodTxt.getText();
- String shippingAddress=this.shippingAddressTxt.getText();
- if(StringUtil.isEmpty(addressee)){
- JOptionPane.showMessageDialog(null, "收件人不能为空!");
- return;
- }
- if(StringUtil.isEmpty(number)){
- JOptionPane.showMessageDialog(null, "购买数量不能为空!");
- return;
- }
- if(StringUtil.isEmpty(deliveryMent)){
- JOptionPane.showMessageDialog(null, "运送方式不能为空!");
- return;
- }
- if(StringUtil.isEmpty(paymentMethod)){
- JOptionPane.showMessageDialog(null, "支付方式不能为空!");
- return;
- }
- if(StringUtil.isEmpty(paymentMethod)){
- JOptionPane.showMessageDialog(null, "收件地址不能为空!");
- return;
- }
- Book book=(Book) this.bookNameJcb.getSelectedItem();
- int bookId=book.getId();
- Order order =new Order(Integer.parseInt(id), addressee, number, deliveryMent, paymentMethod, shippingAddress,
- bookId);
- Connection con =null;
- try {
- con=dbUtil.getCon();
- int addNum=orderDao.update(con, order);
- if(addNum==1) {
- JOptionPane.showMessageDialog(null, "订单修改成功!");
- resetValue();
- this.fillTable(new Order());
- }else {
- JOptionPane.showMessageDialog(null, "订单修改失败!");
- }
- }catch(Exception e) {
- e.printStackTrace();
- }finally {
- try {
- dbUtil.closeCon(con);
- } catch (Exception e) {
- // TODO 自动生成的 catch 块
- e.printStackTrace();
- JOptionPane.showMessageDialog(null, "订单添加失败!");
- }
- }
- }
复制代码 4.数据库设计
4.1系统数据库设计
使用sql语句查询项目存储数据用到的数据库表格:
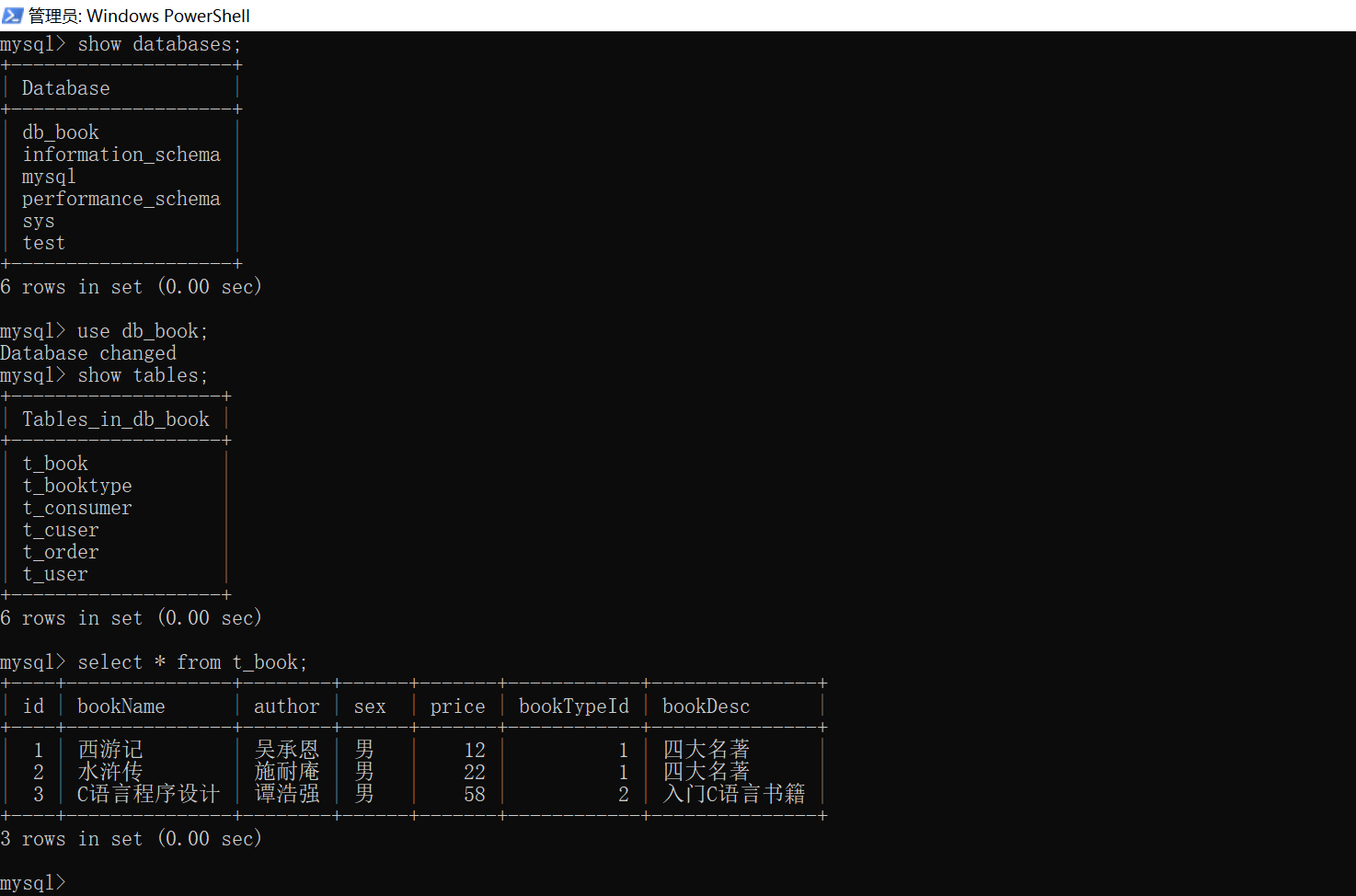
1.管理员信息表
列名数据类型长度主键非空自增IdInt11√√√usenamevarchar20passwordvarchar202.图书类型信息表
列名数据类型长度主键非空自增idInt11√√√BookTypeNameVarchar20bookTypeDesVarchar203.图书信息表
列名数据类型长度主键非空自增BooknameInt11√√√AuthorVarchar20SexVarchar10PriceFloat10bookTypeIdInt11bookDescVarchar10004.订单信息表
列名数据类型长度主键非空自增BuyidInt11√√√NameVarchar20SexVarchar20BuybooknamtelVarchar20WayVarchar20AddressVarchar205.买家信息表
列名数据类型长度主键非空自增IdInt11√√√ConsumernameVarchar50SexVarchar50AgeVarchar50NumberVarchar50BooknameVarchar504.2系统E-R图设计
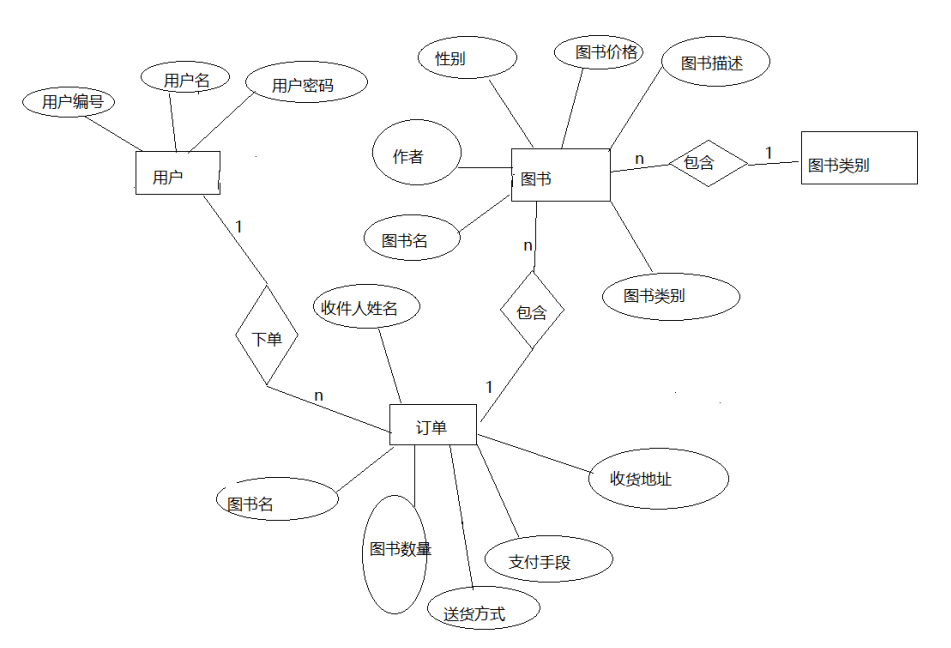
5.JDBC连接数据库
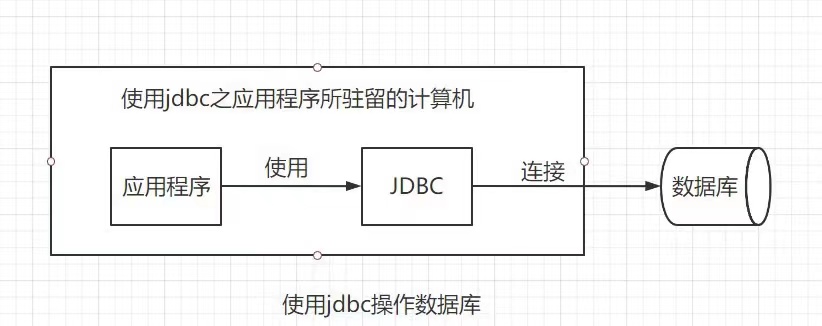
一定要安装数据库jdbc驱动包!
代码展示:
- package com.util;
- import java.sql.Connection;
- import java.sql.DriverManager;
- /**
- * 数据库工具类
- */
- public class DbUtil {
- private String jdbcName="com.mysql.cj.jdbc.Driver"; // 驱动名称
- 数据库连接地址 由于数据库为最新版本 导致驱动名称已改为com.mysql.cj.jdbc.Driver
- //由于时区错乱 执行命令给MySQL服务器设置时区为东八区 serverTimezone=GMT%2B8
- private String dbUrl="jdbc:mysql://localhost:3306/db_book?serverTimezone=GMT%2B8";// 数据库连接地址
- private String dbuserName = "root"; // 用户名
- private String dbpassWord = "abc123"; // 密码
- /**
- * 获取数据库连接
- */
- public Connection getCon()throws Exception{
- Class.forName(jdbcName);
- Connection con=DriverManager.getConnection(dbUrl, dbuserName, dbpassWord);
- return con;
- }
- /**
- * 关闭数据库连接
- */
- public void closeCon(Connection con)throws Exception{
- if(con!=null){
- con.close();
- }
- }
- public static void main(String[] args) {
- DbUtil dbUtil=new DbUtil();
- try {
- dbUtil.getCon();
- System.out.println("数据库连接成功!");
- } catch (Exception e) {
- // TODO Auto-generated catch block
- e.printStackTrace();
- System.out.println("数据库连接失败");
- }
- }
- }
复制代码 6.总结
两周的课程设计圆满结束,在整个项目搭建的过程中也解决了很多的问题,对整体的思路有了把握,进一步理解了Java数据库编程,GUI图形用户界面,JDBC技术。虽然项目设计的时间匆忙,但是Java学习任重而道远,一个人走的更快,一群人走的更远,共勉!
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |