1.在app.json文件中,添加自定义tabbar配置:"custom": true
- "tabBar": {
- "custom": true,
- "backgroundColor": "#fafafa",
- "borderStyle": "white",
- "selectedColor": "#40ae36",
- "color": "#666",
- "list": [
- {
- "pagePath": "pages/index/index",
- "iconPath": "static/images/home.png",
- "selectedIconPath": "static/images/home@selected.png",
- "text": "首页"
- },
- {
- "pagePath": "pages/adoptProduct/adoptProduct",
- "iconPath": "static/images/adopt.png",
- "selectedIconPath": "static/images/adopt@selected.png",
- "text": "认养"
- },
- {
- "pagePath": "pages/supermarket/supermarket",
- "iconPath": "static/images/supermarket.png",
- "selectedIconPath": "static/images/supermarket@selected.png",
- "text": "农副超市"
- },
- {
- "pagePath": "pages/ucenter/index/index",
- "iconPath": "static/images/my.png",
- "selectedIconPath": "static/images/my@selected.png",
- "text": "我的"
- }
- ]
- }
复制代码 2.新建根目次文件
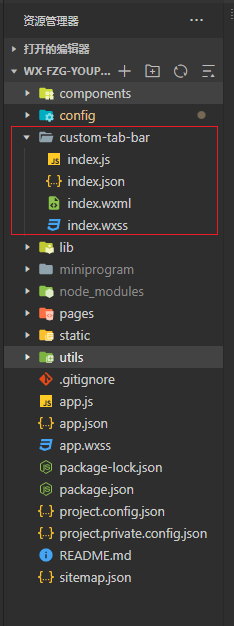
index.wxml
- <view class="tabBar">
- <view class="cont">
- <block wx:for="{{tabBar.list}}" wx:key="index" class="cont-item">
- <view data-path="{{item.pagePath}}" data-index="{{item.pagePath}}" bindtap="switchTab" class="{{item.search?'search':'item'}} {{tabIndex === index ? 'on' : 'off'}}">
- <image src="{{tabIndex === index ? item.selectedIconPath : item.iconPath}}"></image>
- <view class="txt {{tabIndex === index ? 'selectedColor' : ''}}">{{item.text}}</view>
- </view>
- </block>
- </view>
- </view>
复制代码 index.wxss
- .tabBar {
- z-index: 100;
- width: 100%;
- position: fixed;
- bottom: 0;
- font-size: 28rpx;
- background-color: #fff;
- color: #636363;
- }
- .cont {
- z-index: 0;
- height: calc(100rpx + env(safe-area-inset-bottom) / 2);
- padding-bottom: calc(env(safe-area-inset-bottom) / 2);
- padding-bottom: 30rpx;
- display: flex;
- justify-content: space-around;
- }
- .cont .item {
- font-size: 24rpx;
- position: relative;
- width: 15%;
- text-align: center;
- padding: 0;
- display: block;
- height: auto;
- line-height: 1;
- margin: 0;
- background-color: inherit;
- overflow: initial;
- justify-content: center;
- align-items: center;
- padding-top: 20rpx;
- }
- .cont .item image {
- width: 50rpx !important;
- height: 50rpx !important;
- margin: auto
- }
- .cont .selectedColor {
- color: #40ae36;
- }
- .txt{
- font-size: 24rpx;
- }
复制代码 index.js
- //获取应用实例
- const app = getApp();
- Component({
- data: {},
- methods: {
- switchTab(e) {
- const data = e.currentTarget.dataset
- const url = data.path
- wx.switchTab({url})
- }
- }
- })
复制代码 index.json
- {
- "component": true,
- "usingComponents": {}
- }
复制代码 3.在utils添加tab-service.js(通过接口请求,去判断体现谁人tabbar)
- let tabData = {
- tabIndex: 0,//底部按钮高亮下标
- tabBar: {
- custom: true,
- color: "#5F5F5F",
- selectedColor: "#07c160",
- backgroundColor: "#F7F7F7",
- list: []
- }
- }
- // 更新菜单
- const updateRole = (that, type) => {
- if (type === '0') {
- tabData.tabBar.list=[{
- pagePath: "/pages/index/index",
- iconPath: "/static/images/home.png",
- selectedIconPath: "/static/images/home@selected.png",
- text: "首页"
- },
- {
- pagePath: "/pages/adoptProduct/adoptProduct",
- iconPath: "/static/images/adopt.png",
- selectedIconPath: "/static/images/adopt@selected.png",
- text: "认养"
- },
- {
- pagePath: "/pages/supermarket/supermarket",
- iconPath: "/static/images/supermarket.png",
- selectedIconPath: "/static/images/supermarket@selected.png",
- text: "农副超市"
- },
- {
- pagePath: "/pages/ucenter/index/index",
- iconPath: "/static/images/my.png",
- selectedIconPath: "/static/images/my@selected.png",
- text: "我的"
- }]
- }else if (type === '1'){
- tabData.tabBar.list= [{
- pagePath: "/pages/index/index",
- iconPath: "/static/images/home.png",
- selectedIconPath: "/static/images/home@selected.png",
- text: "首页"
- },
- {
- pagePath: "/pages/supermarket/supermarket",
- iconPath: "/static/images/supermarket.png",
- selectedIconPath: "/static/images/supermarket@selected.png",
- text: "农副超市"
- },
- {
- pagePath: "/pages/ucenter/index/index",
- iconPath: "/static/images/my.png",
- selectedIconPath: "/static/images/my@selected.png",
- text: "我的"
- }]
- }
- updateTab(that);
- }
-
- // 更新底部高亮
- const updateIndex = (that, index) => {
- tabData.tabIndex = index;
- updateTab(that);
- }
-
- // 更新Tab状态
- const updateTab = (that) => {
- if (typeof that.getTabBar === 'function' && that.getTabBar()) {
- that.getTabBar().setData(tabData);
- }
- }
-
- // 将可调用的方法抛出让外面调用
- module.exports = {
- updateRole, updateTab, updateIndex,tabBar:tabData.tabBar.list
- }
复制代码 4.在tabbar对应界面添加
- tabService.updateRole(this,'0') // 显示所有tabbar4个
- tabService.updateRole(this,'1') // 显示tabbar中的3个
- tabService.updateIndex(this, 0) // tabbar高亮
复制代码 首页中的index.js
- onShow(){
- this.chooseMenu()
- tabService.updateIndex(this, 0) // tabbar高亮
- }
- chooseMenu(){
- util.request(api.chooseMenu, {
- }, 'GET').then(res => {
- if (res.errno === 0) {
- if(res.data == 1 ) {
- tabService.updateRole(this,'1')
- }else {
- tabService.updateRole(this,'0')
- }
- }
- else{
- util.showErrorToast(res.errmsg);
- }
- })
- }
复制代码 我的页面中的index.js
- onShow(){
- this.chooseMenu()
- tabService.updateIndex(this, 0) // tabbar高亮
- }
- chooseMenu(){
- util.request(api.chooseMenu, {
- }, 'GET').then(res => {
- if (res.errno === 0) {
- if(res.data == 1 ) {
- tabService.updateRole(this,'1')
- tabService.updateIndex(this, 2) //由于tabService.updateRole(this,'1')为1,所有少一个认养tabbar,所有updateIndex应传2高亮
- }else {
- tabService.updateRole(this,'0')
- tabService.updateIndex(this, 3)
- }
- }
- else{
- util.showErrorToast(res.errmsg);
- }
- })
- }
复制代码
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |