马上注册,结交更多好友,享用更多功能,让你轻松玩转社区。
您需要 登录 才可以下载或查看,没有账号?立即注册
x
概述
技能栈:Vue3+Ts+Vite+Echarts
简述:图文详解,教你如安在Vue项目中引入Echarts,封装Echarts组件,并实现常用Echats图列
文章目次
一,效果图
二,引入Echarts
2.1安装Echarts
2.2main.ts中引入
2.3Echarts组件封装
三,使用
3.1柱形图(为例)
文章正文
一效果图
静态效果
动态效果
2.1安装Echarts
2.1.1 npm
2.2.2 pnpm
2.2main.ts中引入
- //引入echarts
- import * as echarts from 'echarts'
- app.config.globalProperties.$echarts = echarts;
复制代码 2.3Echarts组件封装
在/src/components/echartsComp.vue文件中写入以下代码
- <template>
- <div ref="myChartsRef" :style="{ height: height, width: width }" :option="option" />
- </template>
- <script setup lang="ts">
- import { ECharts, EChartsOption, init } from 'echarts';
- import { ref, watch, onMounted, onBeforeUnmount } from 'vue';
- // 定义props泛型
- interface Props {
- width?: string;
- height?: string;
- option: EChartsOption;
- }
- const props = withDefaults(defineProps<Props>(), {
- width: '100%',
- height: '100%',
- option: () => ({})
- });
- const myChartsRef = ref<HTMLDivElement>();
- let myChart: ECharts;
- // eslint-disable-next-line no-undef
- let timer: string | number | NodeJS.Timeout | undefined;
- // 初始化echarts
- const initChart = (): void => {
- if (myChart !== undefined) {
- myChart.dispose();
- }
- myChart = init(myChartsRef.value as HTMLDivElement);
- // 拿到option配置项,渲染echarts
- myChart?.setOption(props.option, true);
- };
- // 重新渲染echarts
- const resizeChart = (): void => {
- timer = setTimeout(() => {
- if (myChart) {
- myChart.resize();
- }
- }, 50);
- };
- // 挂载
- onMounted(() => {
- initChart();
- window.addEventListener('resize', resizeChart);
- });
- // 挂载前
- onBeforeUnmount(() => {
- window.removeEventListener('resize', resizeChart);
- clearTimeout(timer);
- timer = 0;
- });
- // 监听器
- watch(
- props.option,
- () => {
- initChart();
- },
- {
- deep: true
- }
- );
- </script>
复制代码 三,使用(以柱状图为例)
效果图
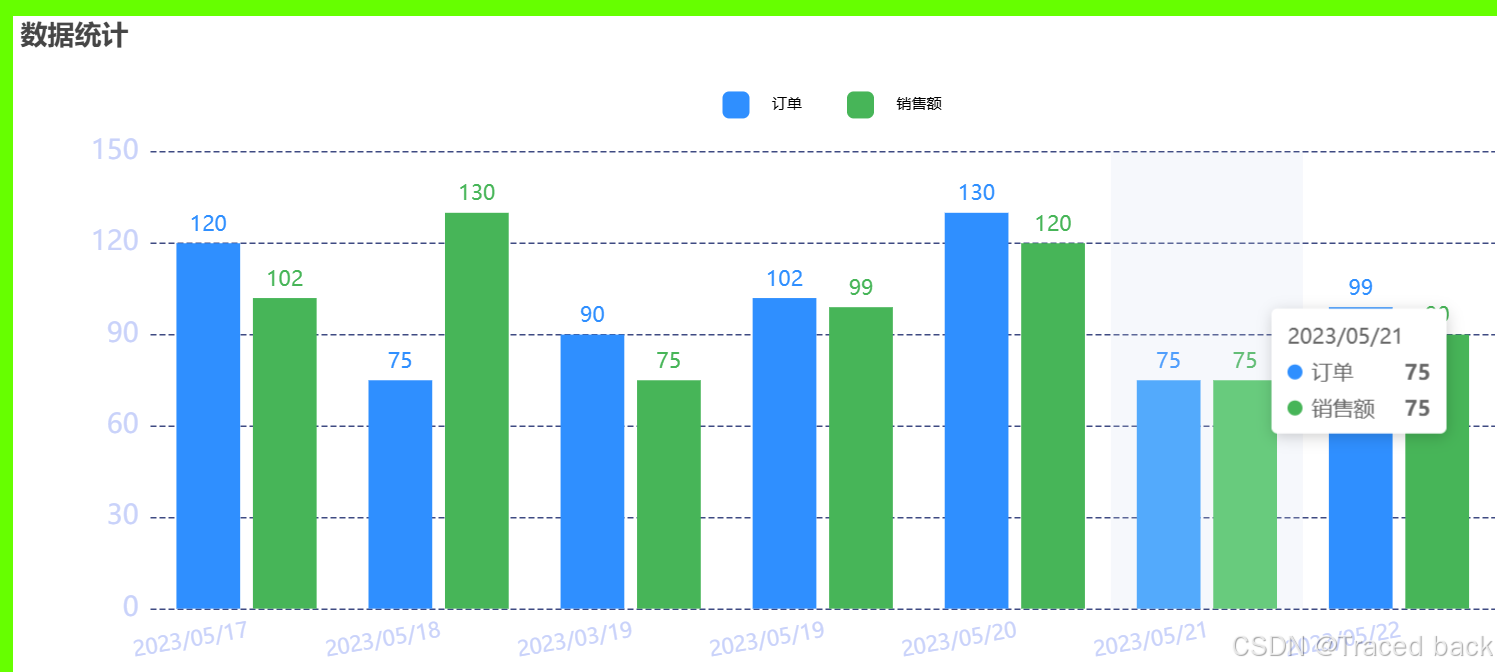
1,在需要的组件中引入该封装的组件
2,在需要的位置引入该组件
- <template>
- <div class="common-layout">
- <el-main>
- <div :style="{ width: '100%', height: '100%' }">
- <Echarts :option="option" />
- </div>
- </el-main>
- </div>
- </template>
- <script setup lang="ts">
- //引入ref实现响应式数据
- import { reactive ,ref} from 'vue';
- // 引入封装好的组件
- import Echarts from '../components/echartsComp.vue';
- const option = reactive(
- {
- backgroundColor: '#fff',
- title:{
- text:'数据统计',
- align: 'center',
- },
- grid: {
- containLabel: true,
- bottom: '5%',
- top: '20%',
- left: '5%',
- right: '5%',
- },
- tooltip: {
- trigger: 'axis',
- axisPointer: {
- type: 'shadow',
- },
- },
- legend: {
- top: '10%',
- right: '40%',
- data: ['订单', '销售额'],
- itemWidth: 18,
- itemHeight: 18,
- itemGap: 30,
- textStyle: {
- fontSize: 10,
- color: 'black',
- padding: [0, 0, 0, 10],
- },
- },
- xAxis: {
- // name: "班级",
- triggerEvent: true,
- data: ['2023/05/17', '2023/05/18', '2023/03/19', '2023/05/19', '2023/05/20', '2023/05/21', '2023/05/22'],
- axisLabel: {
- show: true,
- fontSize: 14,
- color: '#C9D2FA',
- rotate: 10, // 设置旋转角度为30度
- align: 'right',
- verticalAlign: 'top',
- },
- axisLine: {
- show: false,
- lineStyle: {
- show: false,
- color: '#F3F3F3',
- width: 2,
- },
- },
- axisTick: {
- show: false,
- },
- },
- yAxis: [
- {
- // name: '单位:万',
- // type: 'value',
- // nameTextStyle: {
- // color: '#444444',
- // },
- axisLabel: {
- interval: 0,
- show: true,
- fontSize: 18,
- color: '#C9D2FA',
- },
- axisLine: {
- show: false,
- // lineStyle: {
- // color: "#F3F3F3",
- // width: 2
- // }
- },
- axisTick: {
- show: false,
- },
- splitLine: {
- lineStyle: {
- type: 'dashed',
- color: '#3E4A82',
- },
- },
- },
- ],
- series: [
- {
- name: '订单',
- type: 'bar',
- align: 'center',
- silent: true,
- itemStyle: {
- normal: {
- color: '#2F8FFF',
- },
- },
- label: {
- show: true,
- color: '#2F8FFF',
- fontSize: 14,
- position: 'top', // 显示位置,可选值有 'top', 'bottom', 'inside', 'outside'
- formatter: '{c}', // 标签内容格式器,这里表示显示数据值
- },
- data: [120, 75, 90, 102, 130, 75, 99],
- },
- {
- name: '销售额',
- type: 'bar',
- silent: true,
- itemStyle: {
- normal: {
- color: '#47B558',
- },
- },
- label: {
- show: true,
- color: '#47B558',
- fontSize: 14,
- position: 'top', // 显示位置,可选值有 'top', 'bottom', 'inside', 'outside'
- formatter: '{c}', // 标签内容格式器,这里表示显示数据值
- },
- data: [102, 130, 75, 99, 120, 75, 90],
- },
- ],
- }
- )
- </script>
复制代码
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |