基础使用
首先引入依赖-
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-data-redis</artifactId>
- </dependency>
- <dependency>
- <groupId>redis.clients</groupId>
- <artifactId>jedis</artifactId>
- <version>2.9.0</version>
- </dependency>
复制代码 然后在application.yml的spring下增加redis配置:
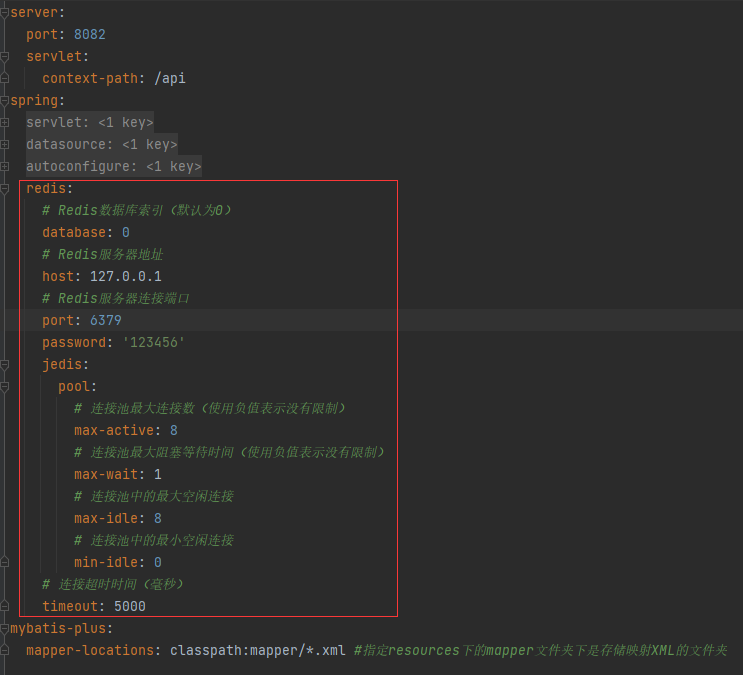
代码如下- redis:
- # Redis数据库索引(默认为0)
- database: 0
- # Redis服务器地址
- host: 127.0.0.1
- # Redis服务器连接端口
- port: 6379
- password: '123456'
- jedis:
- pool:
- # 连接池最大连接数(使用负值表示没有限制)
- max-active: 8
- # 连接池最大阻塞等待时间(使用负值表示没有限制)
- max-wait: 1
- # 连接池中的最大空闲连接
- max-idle: 8
- # 连接池中的最小空闲连接
- min-idle: 0
- # 连接超时时间(毫秒)
- timeout: 5000
复制代码 然后在根包下创建一个service的文件夹加,然后在里面增加redis文件夹,redis文件夹里编写redis的基础操作函数。
编写IRedisService接口,编写增删改查函数,代码如下:- import java.util.Map;
-
- @Service
- public interface IRedisService {
- /**
- * 加入元素
- * @param key
- * @param value
- */
- void setValue(String key, Map<String, Object> value);
- /**
- * 加入元素
- * @param key
- * @param value
- */
- void setValue(String key, String value);
- /**
- * 加入元素
- * @param key
- * @param value
- */
- void setValue(String key, Object value);
- /**
- * 获取元素
- * @param key
- */
- Object getMapValue(String key);
- /**
- * 获取元素
- * @param key
- */
- Object getValue(String key);
-
- }
复制代码 编写RedisServiceImpl实现,实现Redis的增删改查。- package com.example.dynamicdb.service.redis;
-
- import org.springframework.beans.factory.annotation.Autowired;
-
- import org.springframework.data.redis.core.RedisTemplate;
- import org.springframework.data.redis.core.ValueOperations;
- import org.springframework.stereotype.Service;
-
- import java.util.Map;
- import java.util.concurrent.TimeUnit;
-
- @Service("RedisServiceImpl")
- public class RedisServiceImpl implements IRedisService {
-
- public RedisServiceImpl(){}
-
- @Autowired
- private RedisTemplate redisTemplate;
-
-
- @Override
- public void setValue(String key, Map<String, Object> value) {
- ValueOperations<String, Object> vo = redisTemplate.opsForValue();
- vo.set(key, value);
- redisTemplate.expire(key, 1, TimeUnit.HOURS);
- }
-
- @Override
- public Object getValue(String key) {
- ValueOperations<String, String> vo = redisTemplate.opsForValue();
- return vo.get(key);
- }
-
- @Override
- public void setValue(String key, String value) {
- ValueOperations<String, Object> vo = redisTemplate.opsForValue();
- vo.set(key, value);
- redisTemplate.expire(key, 1, TimeUnit.HOURS);
- }
-
- @Override
- public void setValue(String key, Object value) {
- ValueOperations<String, Object> vo = redisTemplate.opsForValue();
- vo.set(key, value);
- redisTemplate.expire(key, 1, TimeUnit.HOURS);
- }
-
- @Override
- public Object getMapValue(String key) {
- ValueOperations<String, String> vo = redisTemplate.opsForValue();
- return vo.get(key);
- }
-
- }
复制代码 然后创建一个RedisController,编写一个测试接口,如下:- @RestController
- public class RedisController {
- @Resource(name = "RedisServiceImpl")//使用resource实例化对象,name是指定实例化的类,用于一个接口多个类继承的情况
- private IRedisService iRedisService;
- @PostMapping(value = "/Redis/TestRedis")
- @ApiOperation(value = "redis测试接口", notes = "redis测试接口", httpMethod = "POST")
- public String TestRedis(){
- iRedisService.setValue("redis", "这是redis的测试数据");
- Object redis = iRedisService.getValue("redis");
- return redis.toString();
- }
- }
复制代码 redis缓存使用
首先创建一个config文件夹,然后创建一个RedisCacheConfig文件,代码如下:-
- import com.fasterxml.jackson.annotation.JsonAutoDetect;
- import com.fasterxml.jackson.annotation.PropertyAccessor;
- import com.fasterxml.jackson.databind.ObjectMapper;
- import org.springframework.cache.annotation.EnableCaching;
- import org.springframework.context.annotation.Bean;
- import org.springframework.context.annotation.Configuration;
- import org.springframework.data.redis.cache.RedisCacheConfiguration;
- import org.springframework.data.redis.cache.RedisCacheManager;
- import org.springframework.data.redis.cache.RedisCacheWriter;
- import org.springframework.data.redis.connection.RedisConnectionFactory;
- import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
- import org.springframework.data.redis.serializer.RedisSerializationContext;
-
- import java.time.Duration;
- import java.util.HashMap;
- import java.util.Map;
-
- @EnableCaching
- @Configuration
- public class RedisCacheConfig {
-
-
- /**
- * 最新版,设置redis缓存过期时间
- */
-
- @Bean
- public RedisCacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) {
- return new RedisCacheManager(
- RedisCacheWriter.nonLockingRedisCacheWriter(redisConnectionFactory),
- this.getRedisCacheConfigurationWithTtl( 60), // 默认策略,未配置的 key 会使用这个
- this.getRedisCacheConfigurationMap() // 指定 key 策略
- );
- }
-
- private Map<String, RedisCacheConfiguration> getRedisCacheConfigurationMap() {
- Map<String, RedisCacheConfiguration> redisCacheConfigurationMap = new HashMap<>();
- //SsoCache和BasicDataCache进行过期时间配置
- redisCacheConfigurationMap.put("messagCache", this.getRedisCacheConfigurationWithTtl(30 * 60));
-
- //自定义设置缓存时间
- redisCacheConfigurationMap.put("studentCache", this.getRedisCacheConfigurationWithTtl(60 ));
-
- return redisCacheConfigurationMap;
- }
-
- private RedisCacheConfiguration getRedisCacheConfigurationWithTtl(Integer seconds) {
- Jackson2JsonRedisSerializer<Object> jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer<>(Object.class);
- ObjectMapper om = new ObjectMapper();
- om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
- om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
- jackson2JsonRedisSerializer.setObjectMapper(om);
- RedisCacheConfiguration redisCacheConfiguration = RedisCacheConfiguration.defaultCacheConfig();
- redisCacheConfiguration = redisCacheConfiguration.serializeValuesWith(
- RedisSerializationContext
- .SerializationPair
- .fromSerializer(jackson2JsonRedisSerializer)
- ).entryTtl(Duration.ofSeconds(seconds));
-
- return redisCacheConfiguration;
- }
- }
复制代码 类名上有注解@Configuration,代表该类会在启动时加入进bean集合。
然后在RedisController下编写测试函数,如下:- @Autowired
- private SqlSession sqlSession;
- @GetMapping(value = "/Redis/TestRedisCache")
- @ResponseBody
- @DS("db2")
- @Cacheable(cacheNames = "userCache", key = "#id")
- @ApiOperation(value="查询单条记录",notes = "查询")
- public List<user> TestRedisCache(Integer id) {
- //读取第二个数据库的值
- UserMapper mapper = sqlSession.getMapper(UserMapper.class);
- List<user> users = mapper.test();
- return users;
- }
复制代码 使用@Cacheable注解缓存接口的返回值,cacheNames的值和key的值,组合起来成为是缓存中的键值对的key值,如下图。
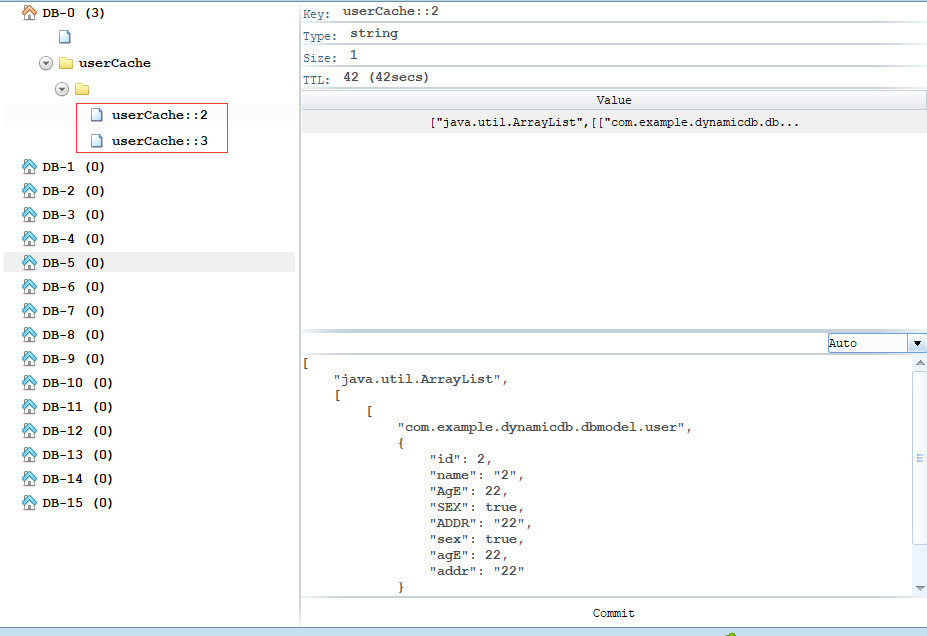
----------------------------------------------------------------------------------------------------
到此,SpringBoot中Redis的基础使用就已经介绍完了。
代码已经传到Github上了,欢迎大家下载。
Github地址:https://github.com/kiba518/dynamicdb
----------------------------------------------------------------------------------------------------
注:此文章为原创,任何形式的转载都请联系作者获得授权并注明出处!
若您觉得这篇文章还不错,请点击下方的【推荐】,非常感谢!
https://www.cnblogs.com/kiba/p/17480377.html
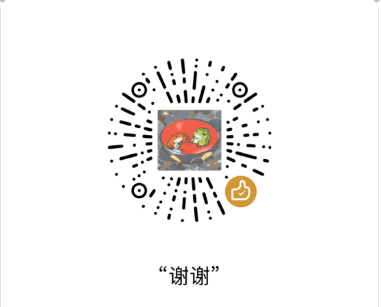
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |