依赖注入就是对类的属性进行赋值
4.1、环境搭建
创建名为spring_ioc_xml的新module,过程参考3.1节
4.1.1、创建spring配置文件
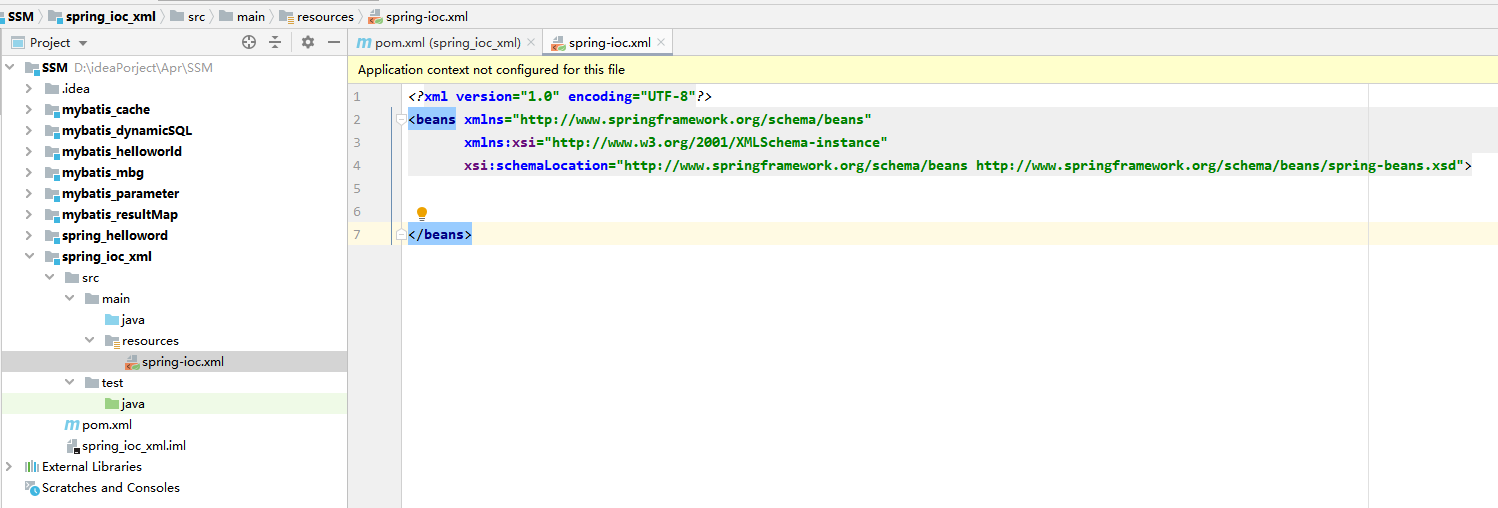 - [/code][size=4]4.1.2、创建学生类Student[/size]
- [img]https://img2023.cnblogs.com/blog/2052479/202307/2052479-20230727223544516-1576170602.png[/img]
- [code]package org.rain.spring.pojo;
- /**
- * @author liaojy
- * @date 2023/7/27 - 22:33
- */
- public class Student {
- private Integer id;
- private String name;
- private Integer age;
- private String sex;
- public Student() {
- }
- public Student(Integer id, String name, Integer age, String sex) {
- this.id = id;
- this.name = name;
- this.age = age;
- this.sex = sex;
- }
- public Integer getId() {
- return id;
- }
- public void setId(Integer id) {
- this.id = id;
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- public Integer getAge() {
- return age;
- }
- public void setAge(Integer age) {
- this.age = age;
- }
- public String getSex() {
- return sex;
- }
- public void setSex(String sex) {
- this.sex = sex;
- }
- @Override
- public String toString() {
- return "Student{" +
- "id=" + id +
- ", name='" + name + '\'' +
- ", age=" + age +
- ", sex='" + sex + '\'' +
- '}';
- }
- }
复制代码 4.2、setter注入(常用)
4.2.1、配置bean
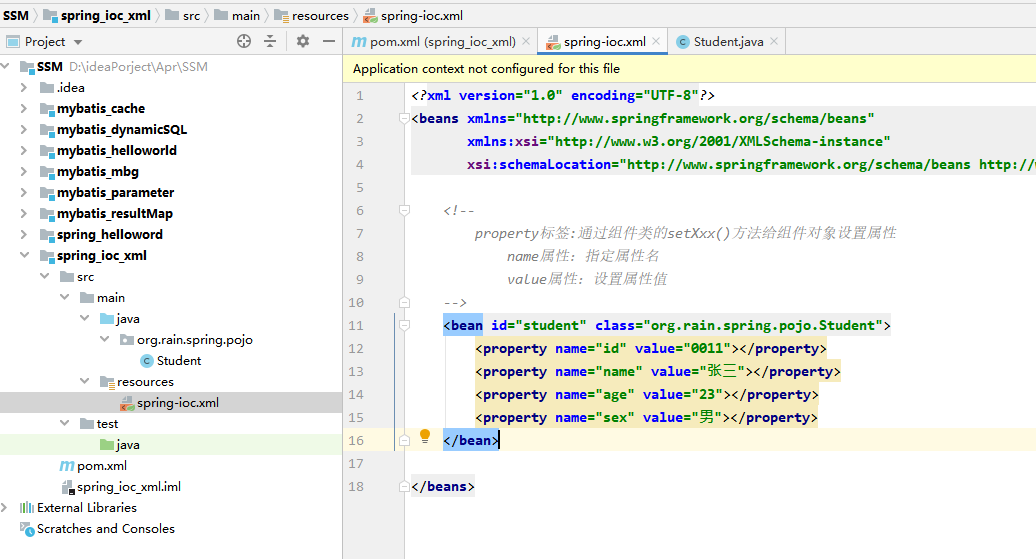 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.2.2、测试
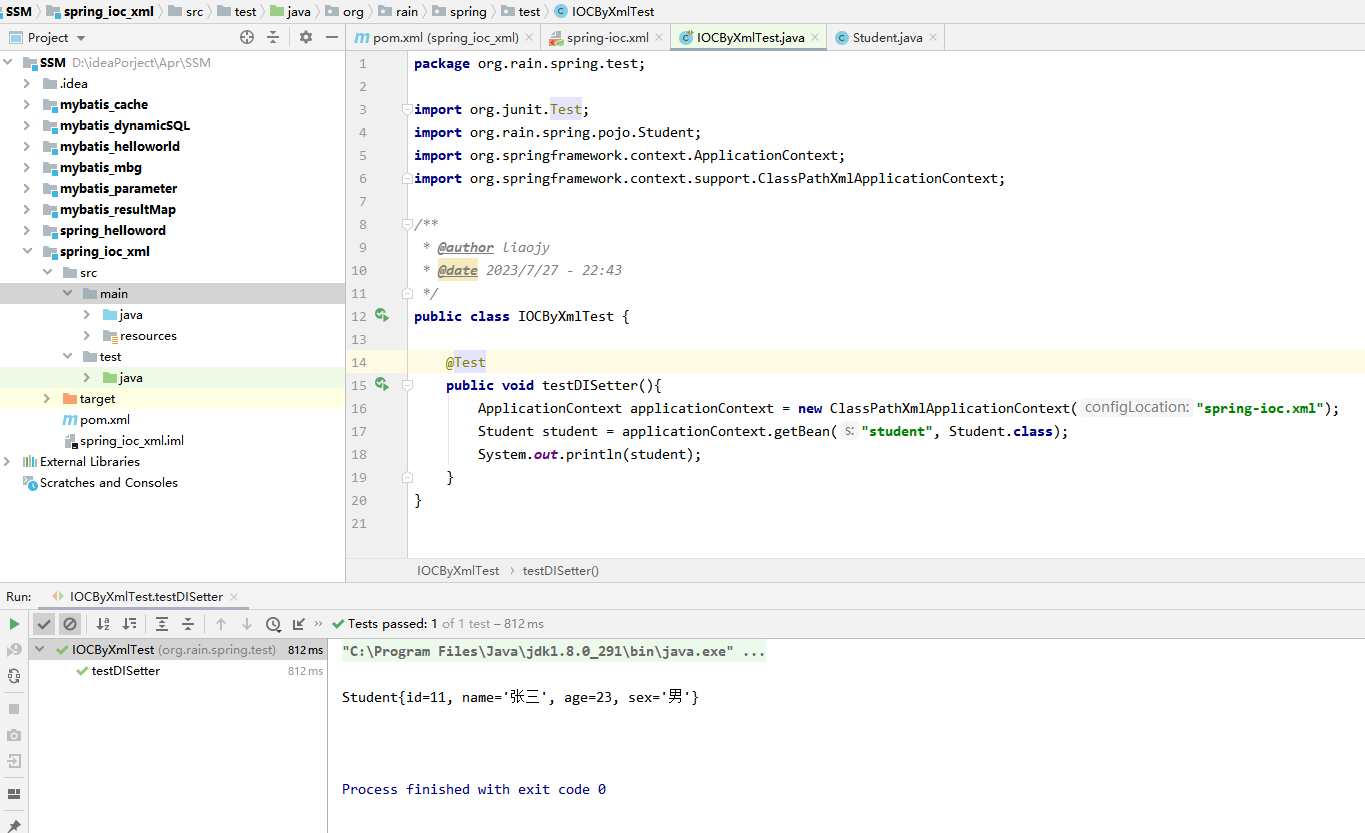 - package org.rain.spring.test;
- import org.junit.Test;
- import org.rain.spring.pojo.Student;
- import org.springframework.context.ApplicationContext;
- import org.springframework.context.support.ClassPathXmlApplicationContext;
- /**
- * @author liaojy
- * @date 2023/7/27 - 22:43
- */
- public class IOCByXmlTest {
- @Test
- public void testDISetter(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("student", Student.class);
- System.out.println(student);
- }
- }
复制代码 4.3、构造器注入
4.3.1、配置bean
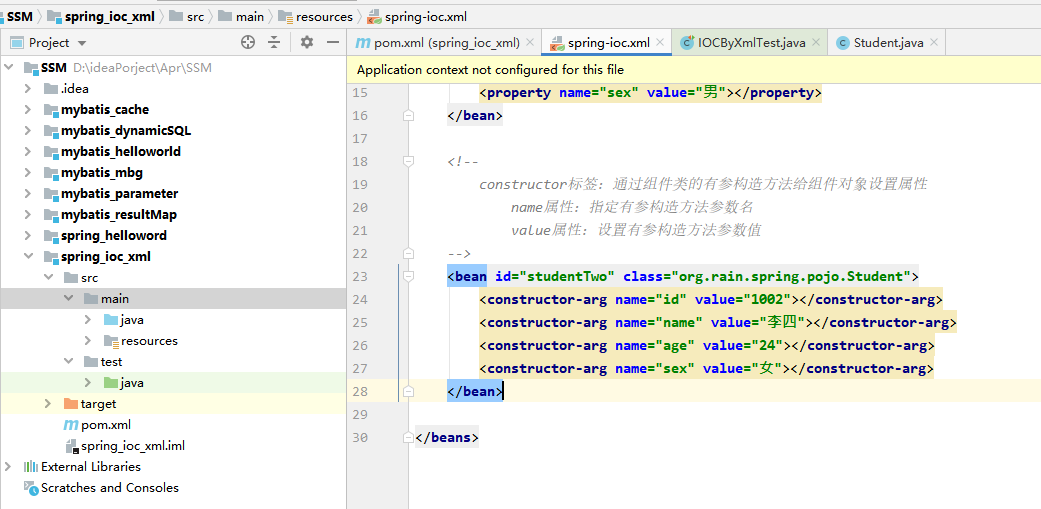
注意:constructor-arg标签的数量,必须和某一个构造器方法的参数数量一致
-
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.3.2、测试
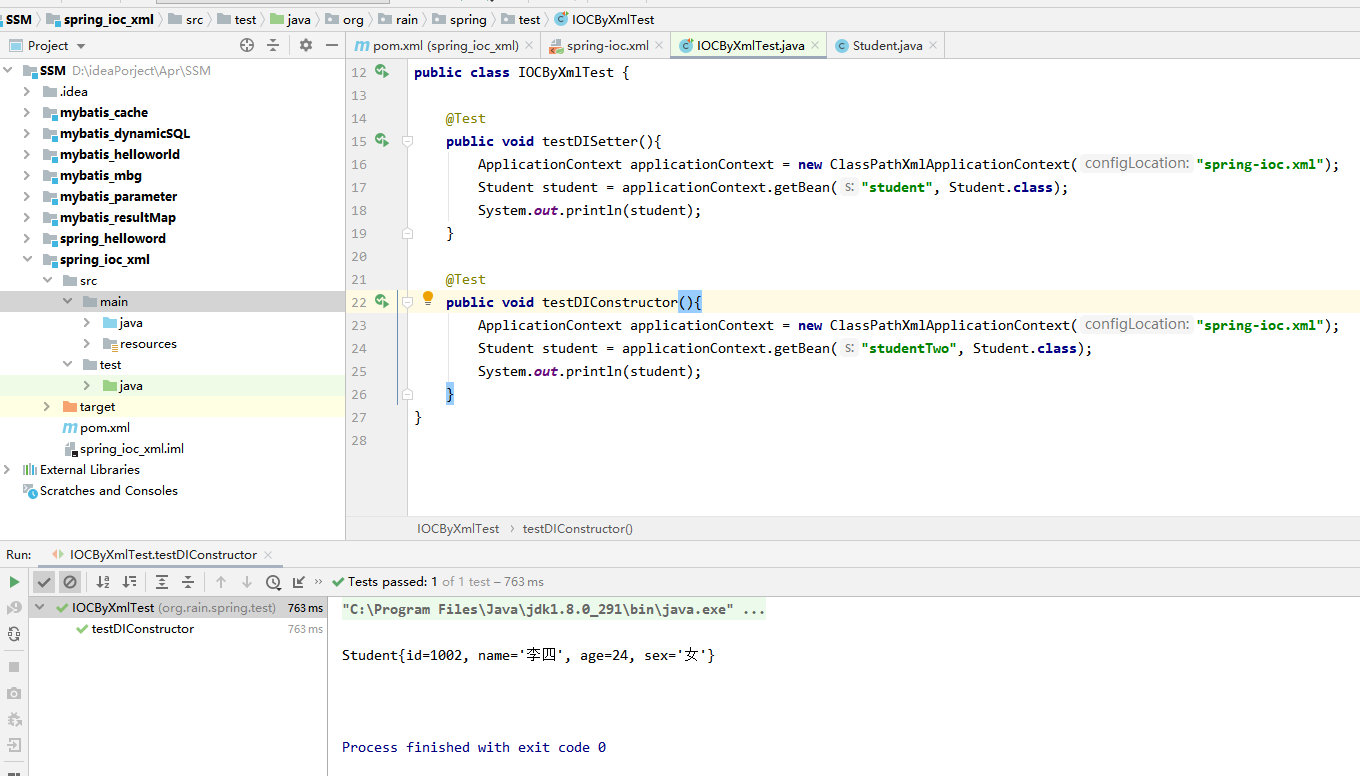 - @Test
- public void testDIConstructor(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentTwo", Student.class);
- System.out.println(student);
- }
复制代码 4.4、特殊值处理
4.4.1、null值
4.4.1.1、配置bean
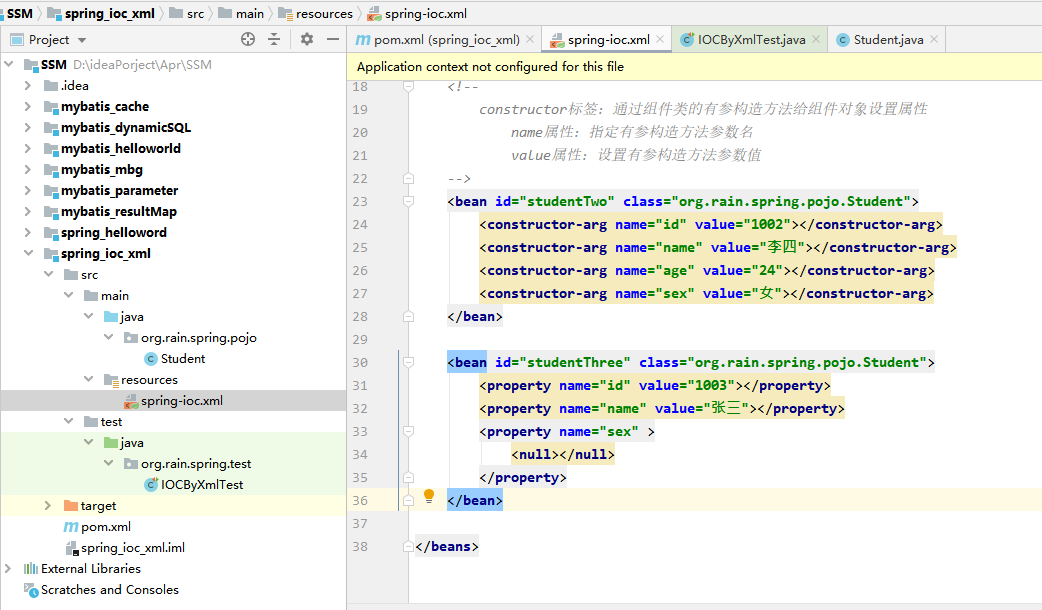
注意:该写法,实际为sex所赋的值是字符串null
-
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.4.1.2、测试
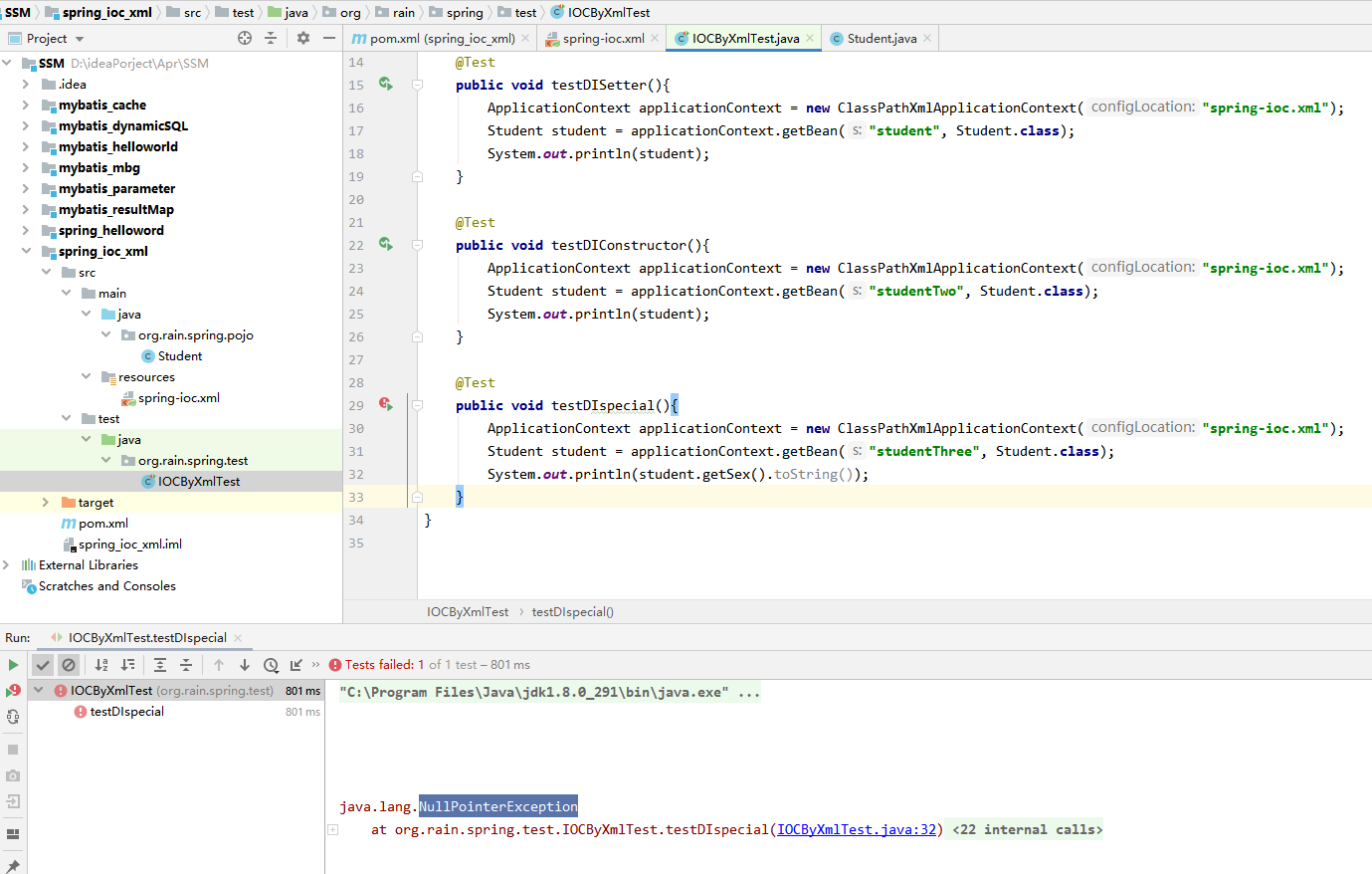
由控制台日志可知,此时sex的值为null
- @Test
- public void testDIspecial(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentThree", Student.class);
- System.out.println(student.getSex().toString());
- }
复制代码 +++++++++++++++++++++++++++++++++++分割线+++++++++++++++++++++++++++++++++++
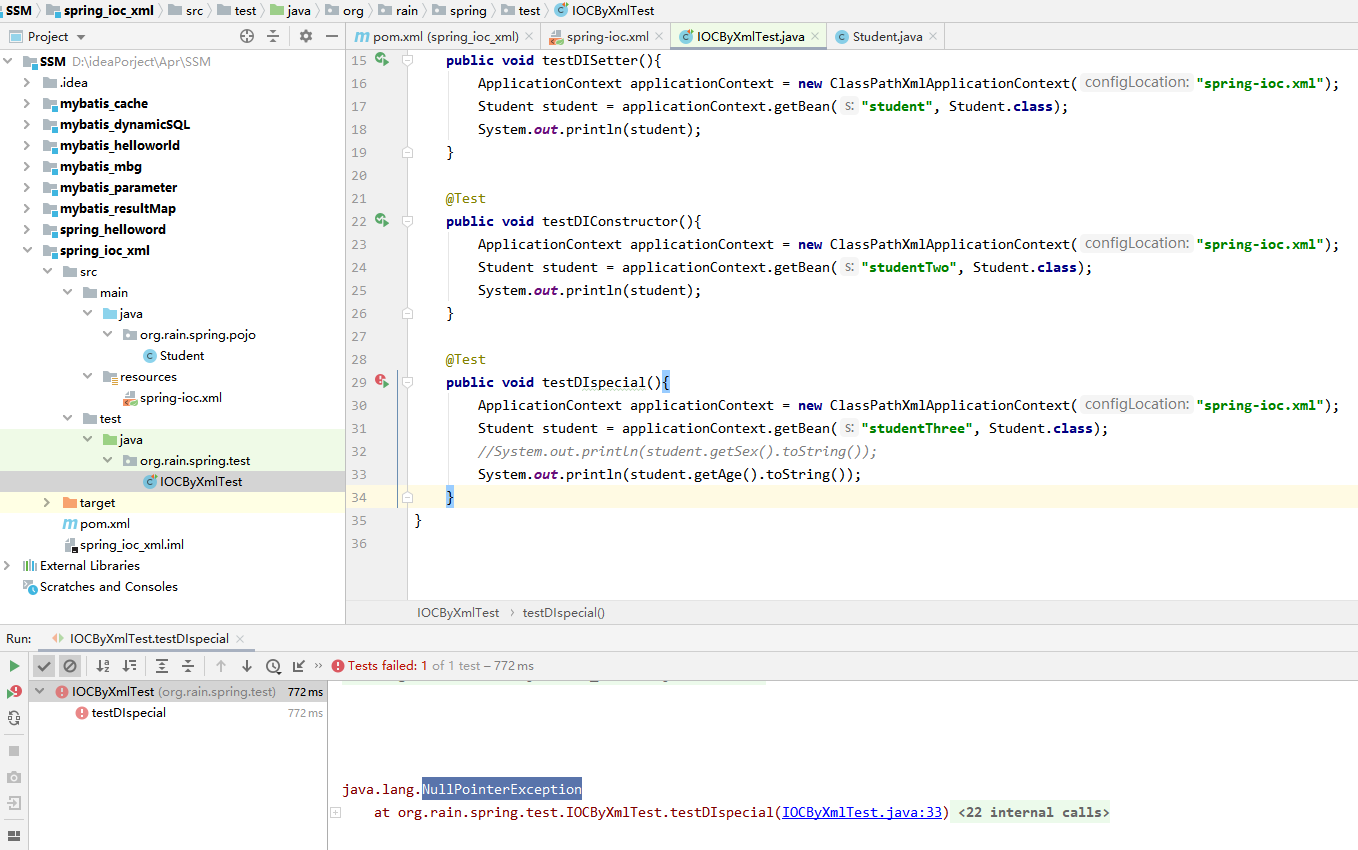
由控制台日志可知,此时age的值也为null;所以不配置属性也能实现同样的效果
4.4.2、xml字符值
4.4.2.1、方式一:实体
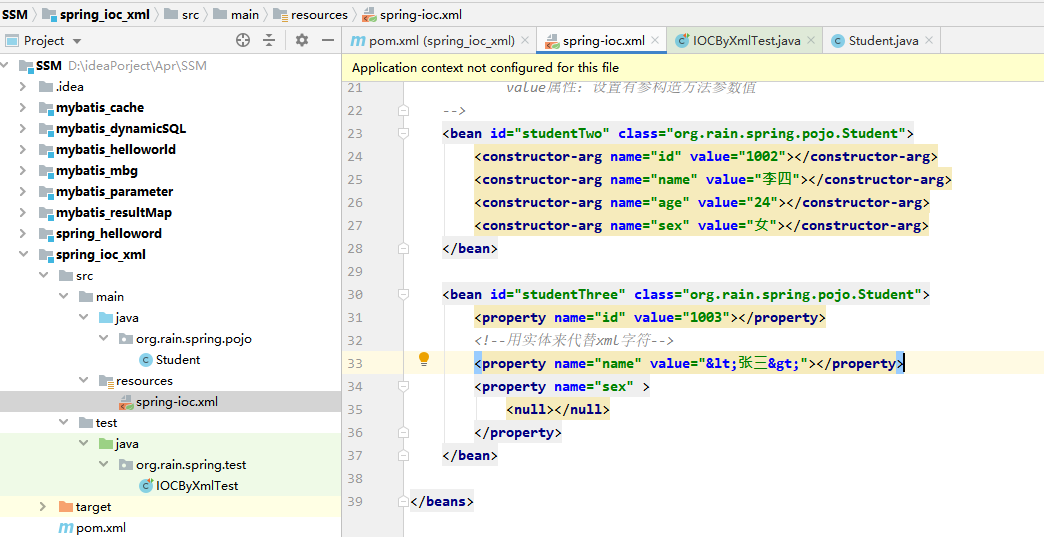 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.4.2.2、方式二:CDATA节
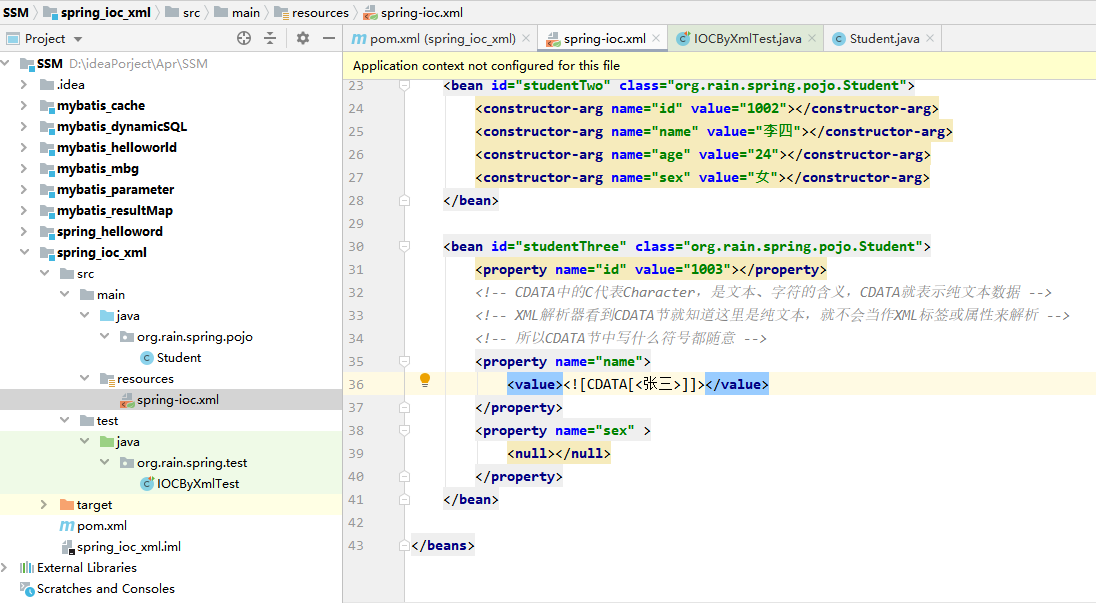 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> ]]> <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.4.2.3、测试
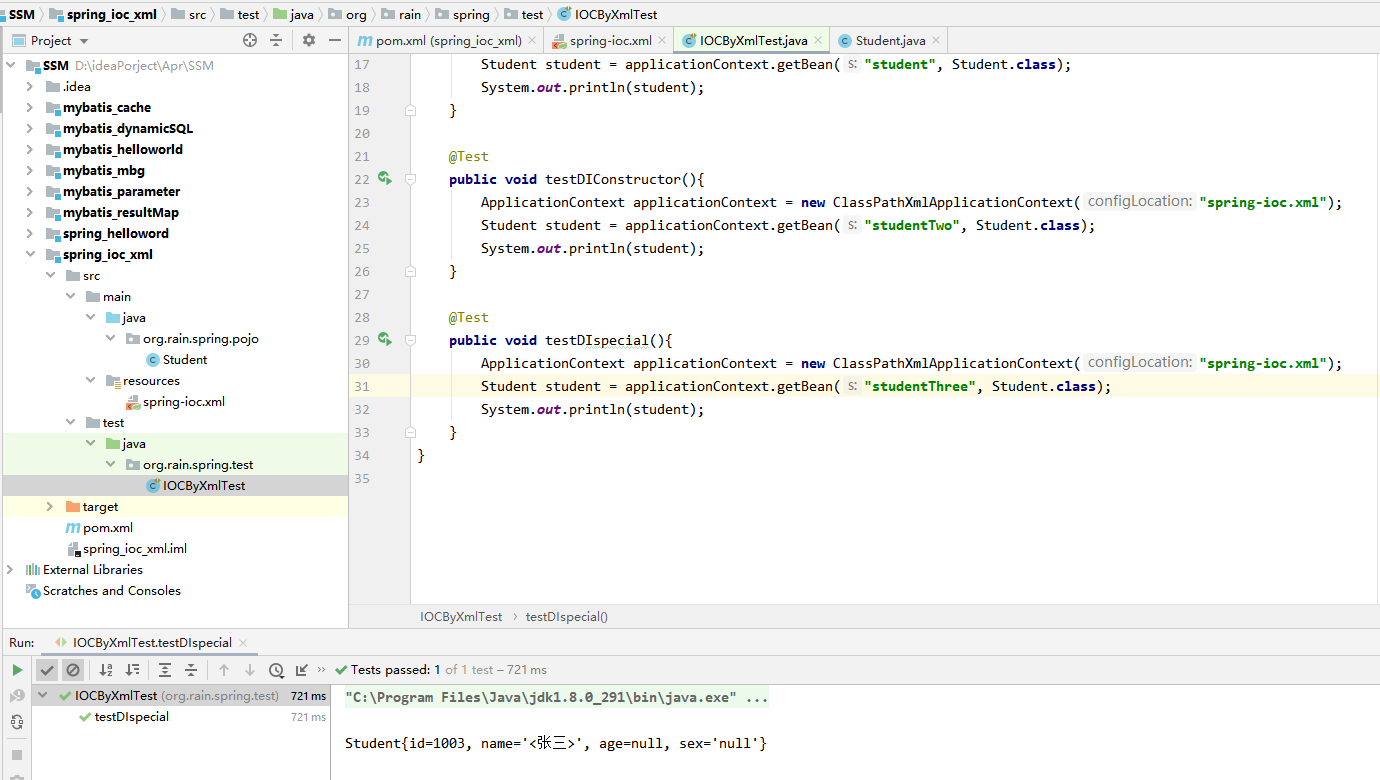
4.5、为类类型的属性赋值
4.5.1、方式一:外部bean(常用)
4.5.1.1、创建班级类Clazz
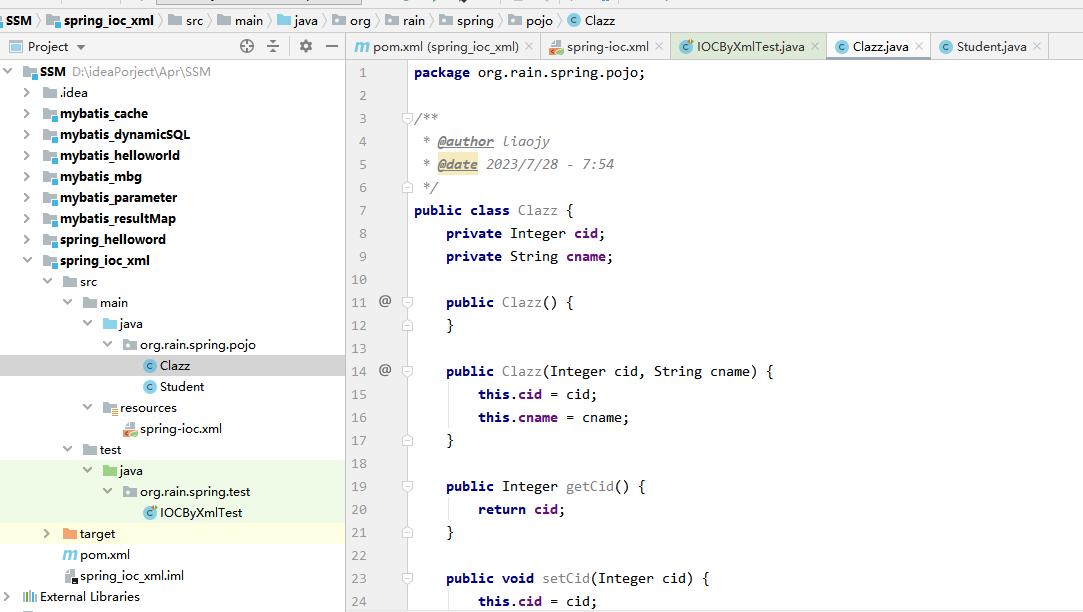 - package org.rain.spring.pojo;
- /**
- * @author liaojy
- * @date 2023/7/28 - 7:54
- */
- public class Clazz {
- private Integer cid;
- private String cname;
- public Clazz() {
- }
- public Clazz(Integer cid, String cname) {
- this.cid = cid;
- this.cname = cname;
- }
- public Integer getCid() {
- return cid;
- }
- public void setCid(Integer cid) {
- this.cid = cid;
- }
- public String getCname() {
- return cname;
- }
- public void setCname(String cname) {
- this.cname = cname;
- }
- @Override
- public String toString() {
- return "Clazz{" +
- "cid=" + cid +
- ", cname='" + cname + '\'' +
- '}';
- }
- }
复制代码 4.5.1.2、修改Student类
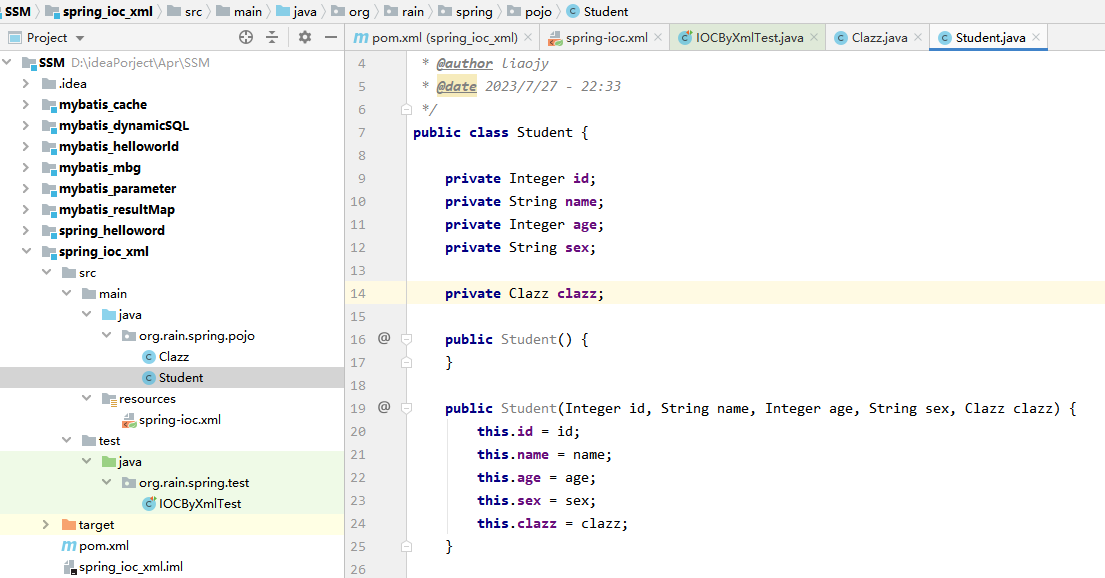 - package org.rain.spring.pojo;
- /**
- * @author liaojy
- * @date 2023/7/27 - 22:33
- */
- public class Student {
- private Integer id;
- private String name;
- private Integer age;
- private String sex;
- private Clazz clazz;
- public Student() {
- }
- public Student(Integer id, String name, Integer age, String sex, Clazz clazz) {
- this.id = id;
- this.name = name;
- this.age = age;
- this.sex = sex;
- this.clazz = clazz;
- }
- public Clazz getClazz() {
- return clazz;
- }
- public void setClazz(Clazz clazz) {
- this.clazz = clazz;
- }
- public Integer getId() {
- return id;
- }
- public void setId(Integer id) {
- this.id = id;
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- public Integer getAge() {
- return age;
- }
- public void setAge(Integer age) {
- this.age = age;
- }
- public String getSex() {
- return sex;
- }
- public void setSex(String sex) {
- this.sex = sex;
- }
- @Override
- public String toString() {
- return "Student{" +
- "id=" + id +
- ", name='" + name + '\'' +
- ", age=" + age +
- ", sex='" + sex + '\'' +
- ", clazz=" + clazz +
- '}';
- }
- }
复制代码 4.5.1.3、配置外部bean
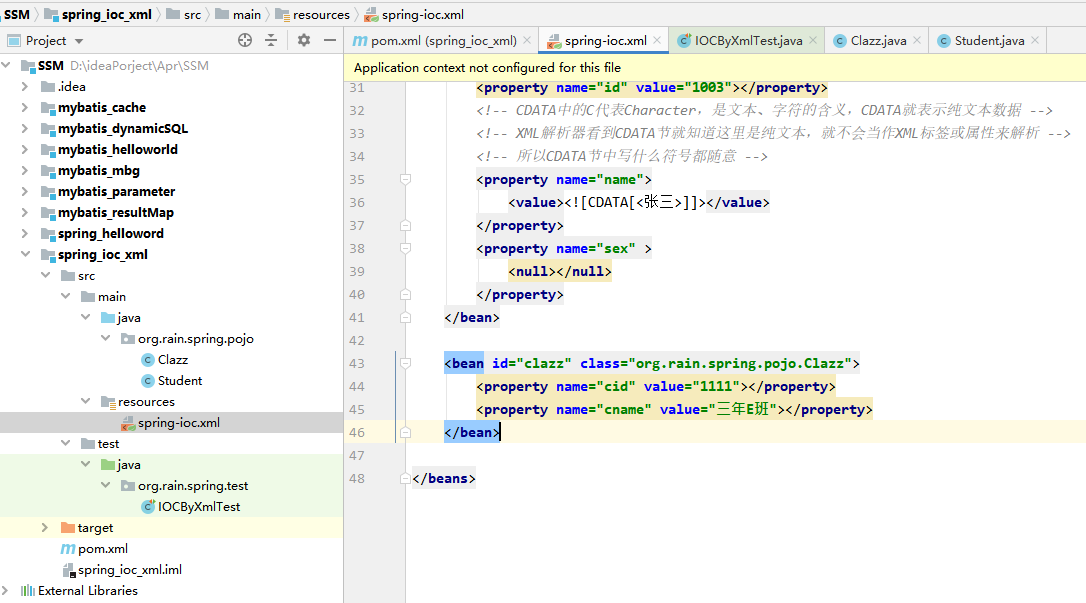 - <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.5.1.4、引用外部bean
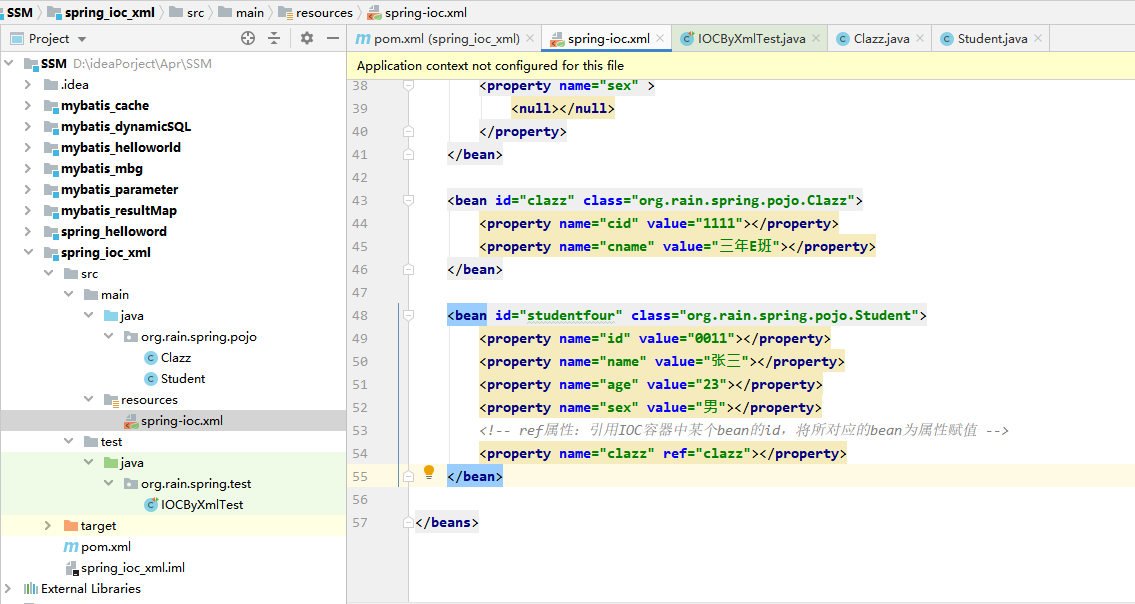 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.5.1.5、测试
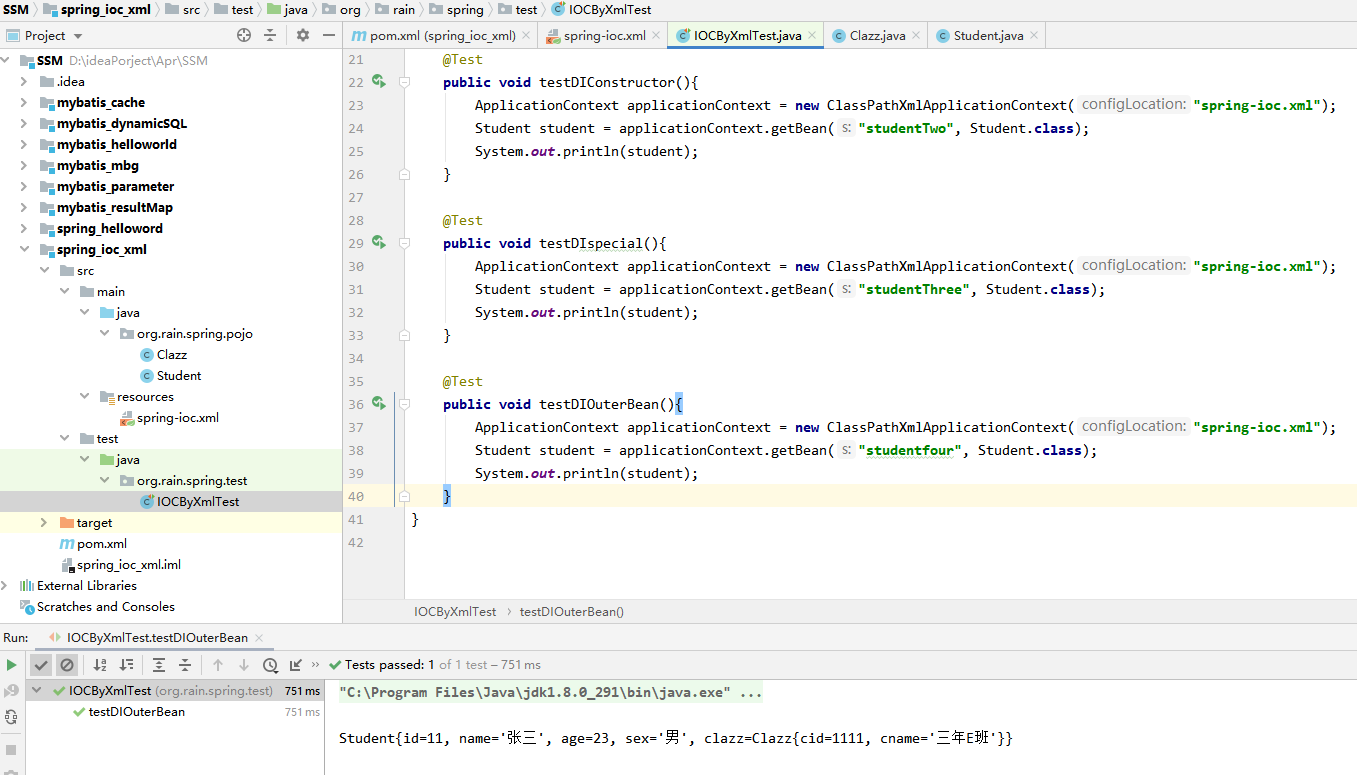 - @Test
- public void testDIOuterBean(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentfour", Student.class);
- System.out.println(student);
- }
复制代码 4.5.2、方式二、级联
4.5.2.1、配置bean
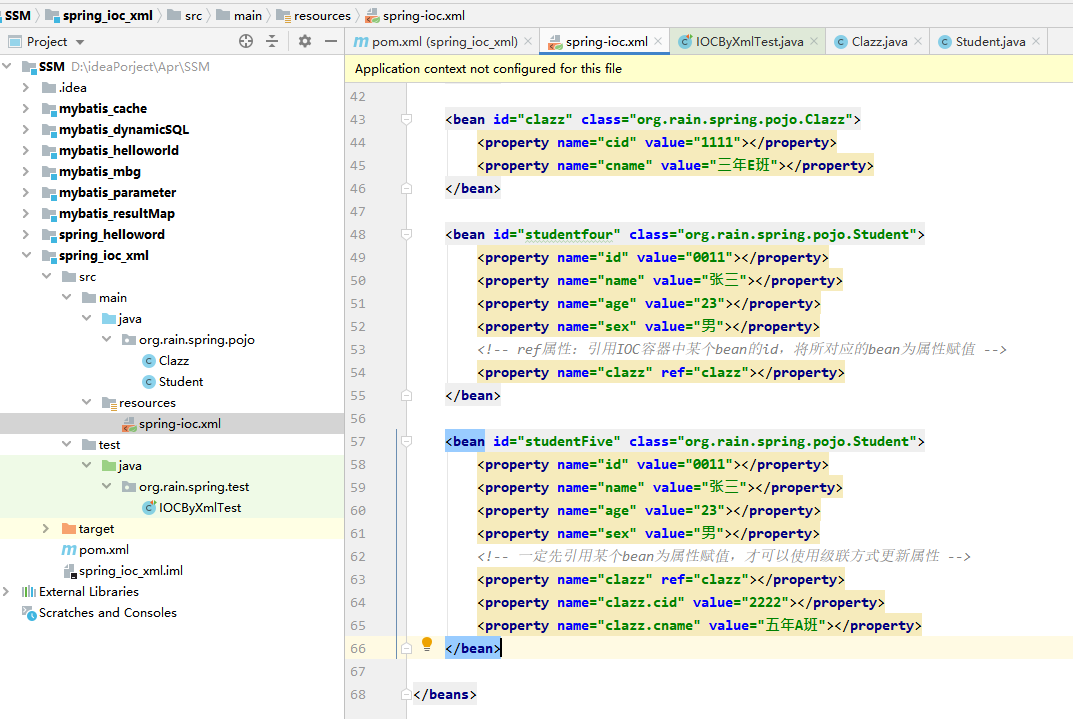 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.5.2.2、测试
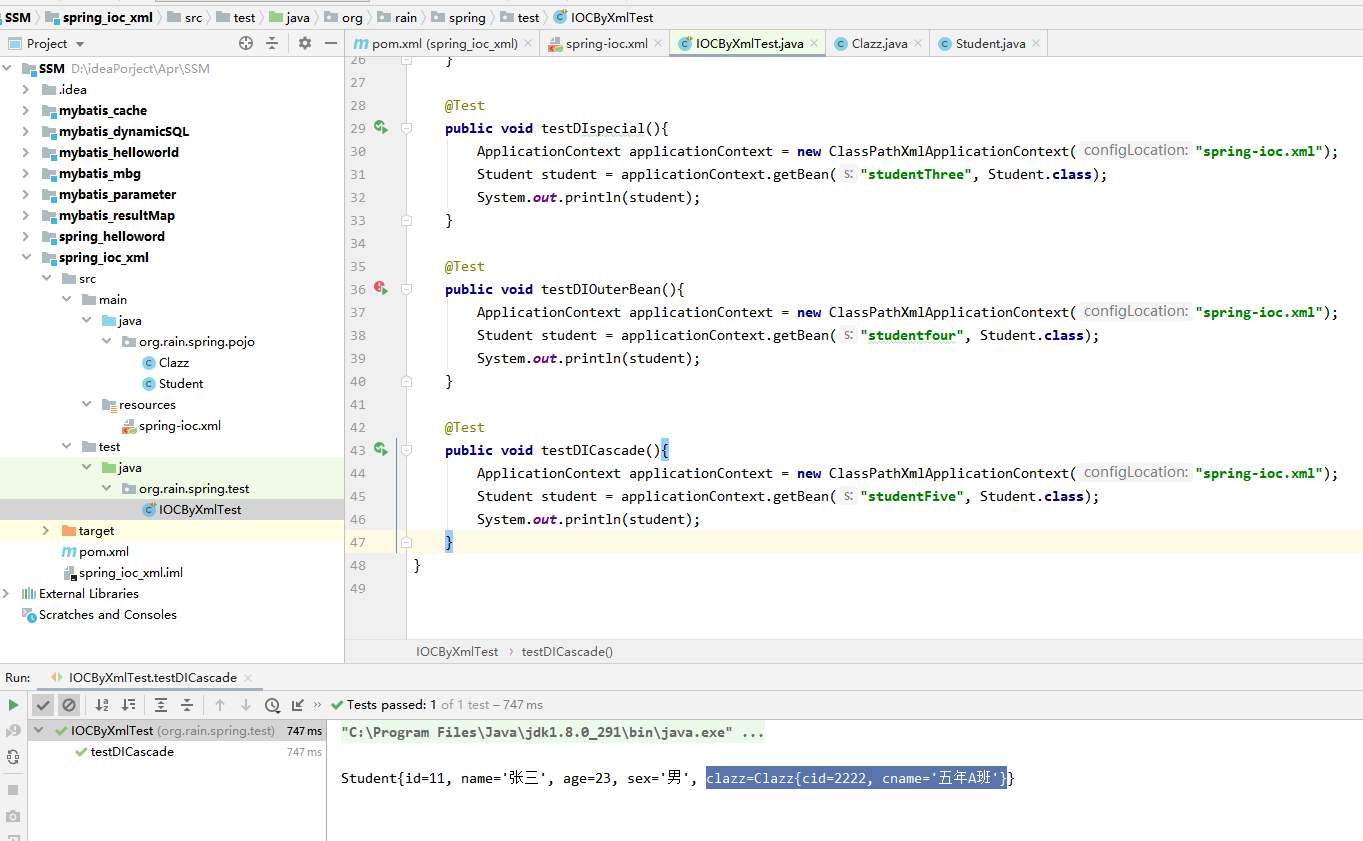 - @Test
- public void testDICascade(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentFive", Student.class);
- System.out.println(student);
- }
复制代码 4.5.3、内部bean(常用)
4.5.3.1、配置bean
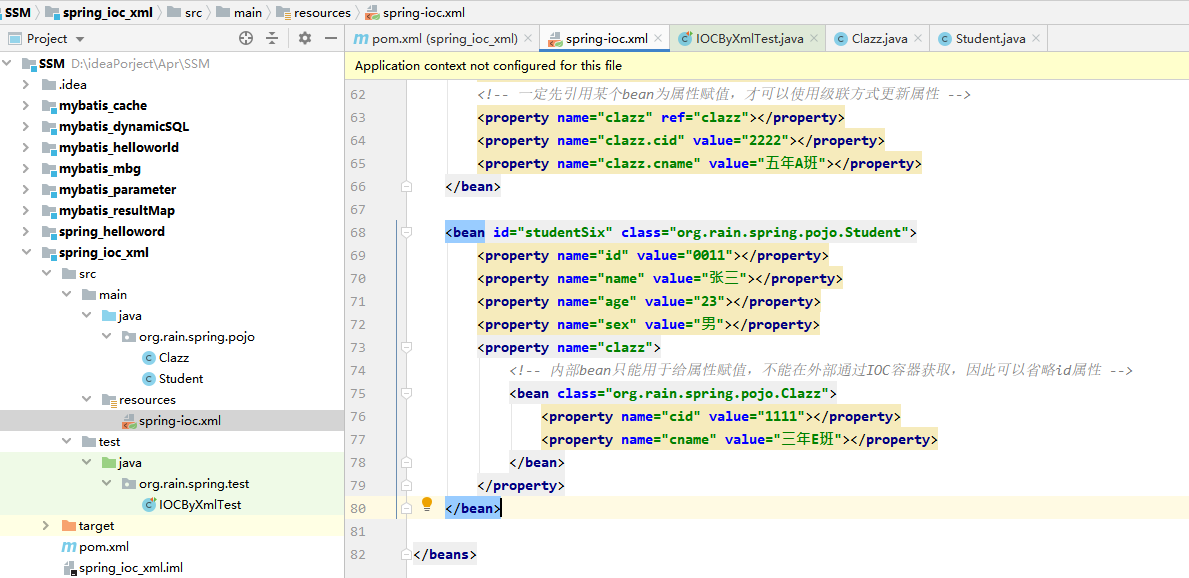 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.5.3.2、测试
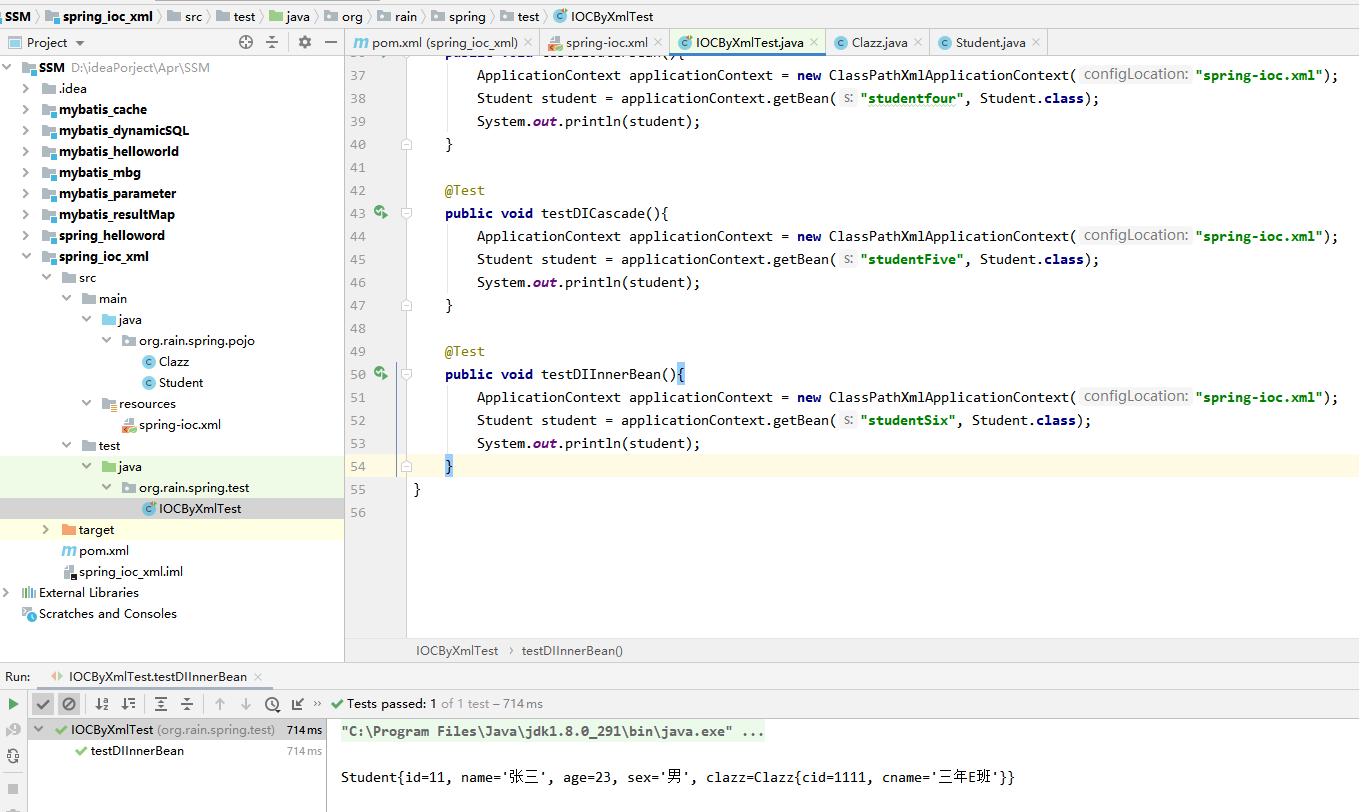 - @Test
- public void testDIInnerBean(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentSix", Student.class);
- System.out.println(student);
- }
复制代码 4.6、为数组类型的属性赋值
4.6.1、修改Student类
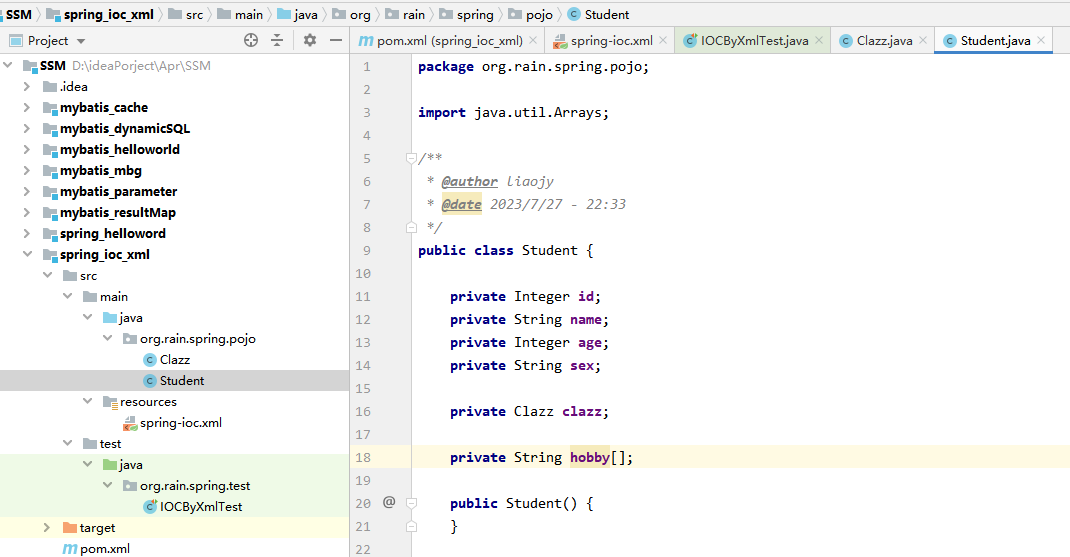
在Student类中添加或修改以下代码:
- private String hobby[];
- public String[] getHobby() {
- return hobby;
- }
- public void setHobby(String[] hobby) {
- this.hobby = hobby;
- }
- @Override
- public String toString() {
- return "Student{" +
- "id=" + id +
- ", name='" + name + '\'' +
- ", age=" + age +
- ", sex='" + sex + '\'' +
- ", clazz=" + clazz +
- ", hobby=" + Arrays.toString(hobby) +
- '}';
- }
复制代码 4.6.2、配置bean
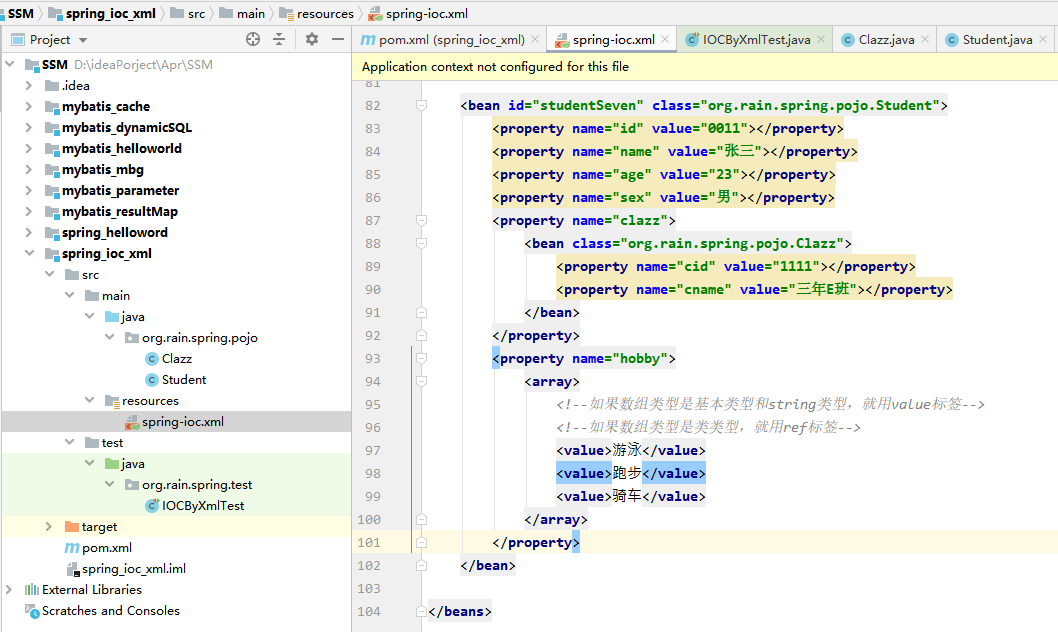 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>游泳 跑步 骑车 <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.6.3、测试
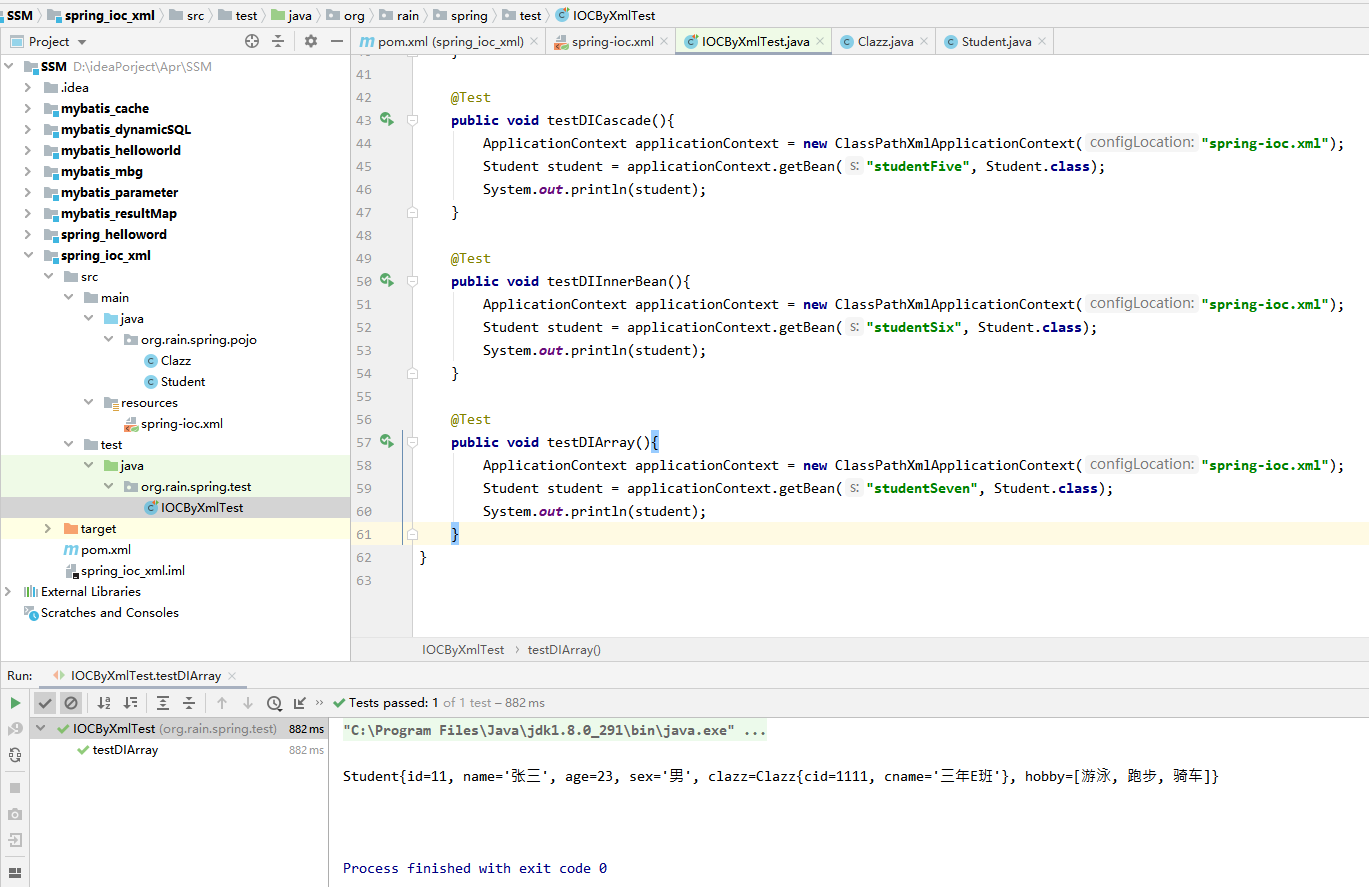 - @Test
- public void testDIArray(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentSeven", Student.class);
- System.out.println(student);
- }
复制代码 4.7、为List集合类型的属性赋值
4.7.1、修改Clazz类
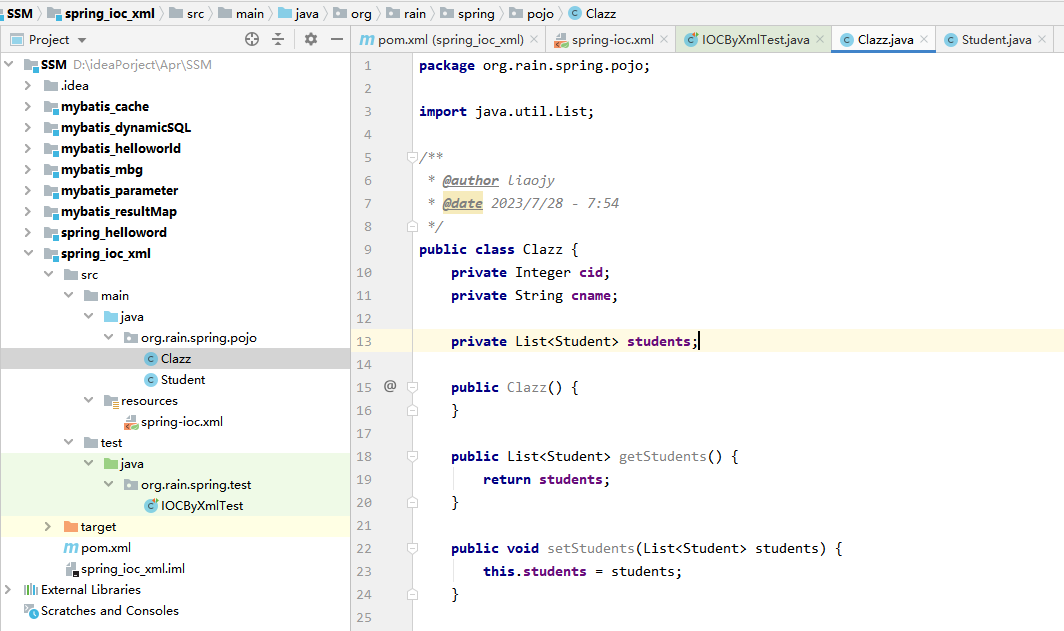
在Clazzt类中添加或修改以下代码:
- private List<Student> students;
- public List<Student> getStudents() {
- return students;
- }
- public void setStudents(List<Student> students) {
- this.students = students;
- }
- @Override
- public String toString() {
- return "Clazz{" +
- "cid=" + cid +
- ", cname='" + cname + '\'' +
- ", students=" + students +
- '}';
- }
复制代码 4.7.2、配置bean
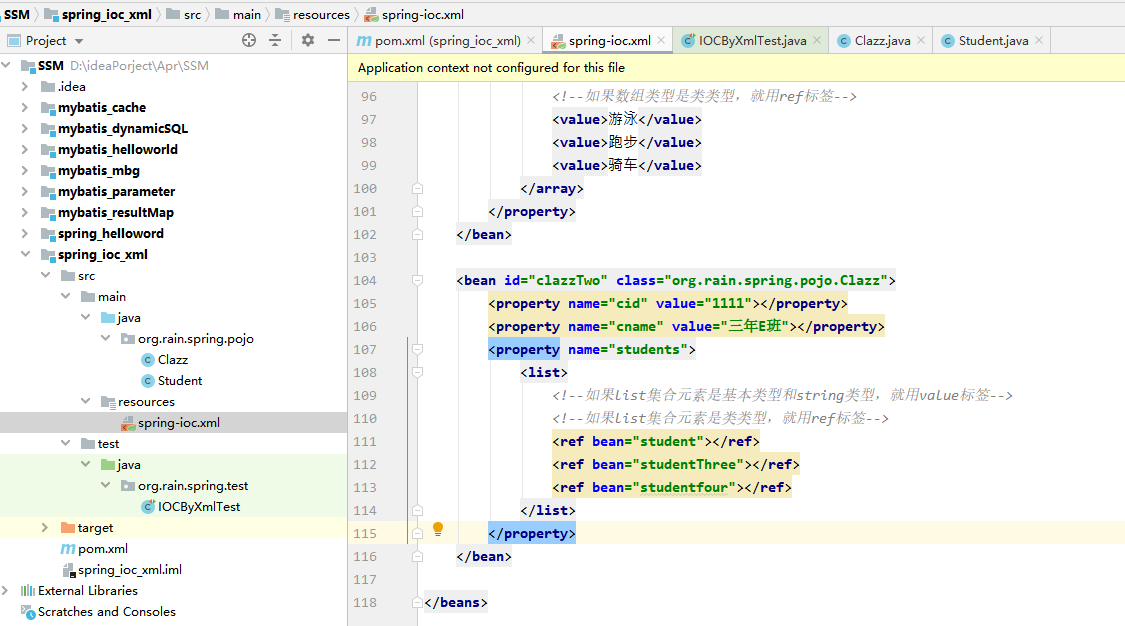 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean> ]]>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.7.3、测试
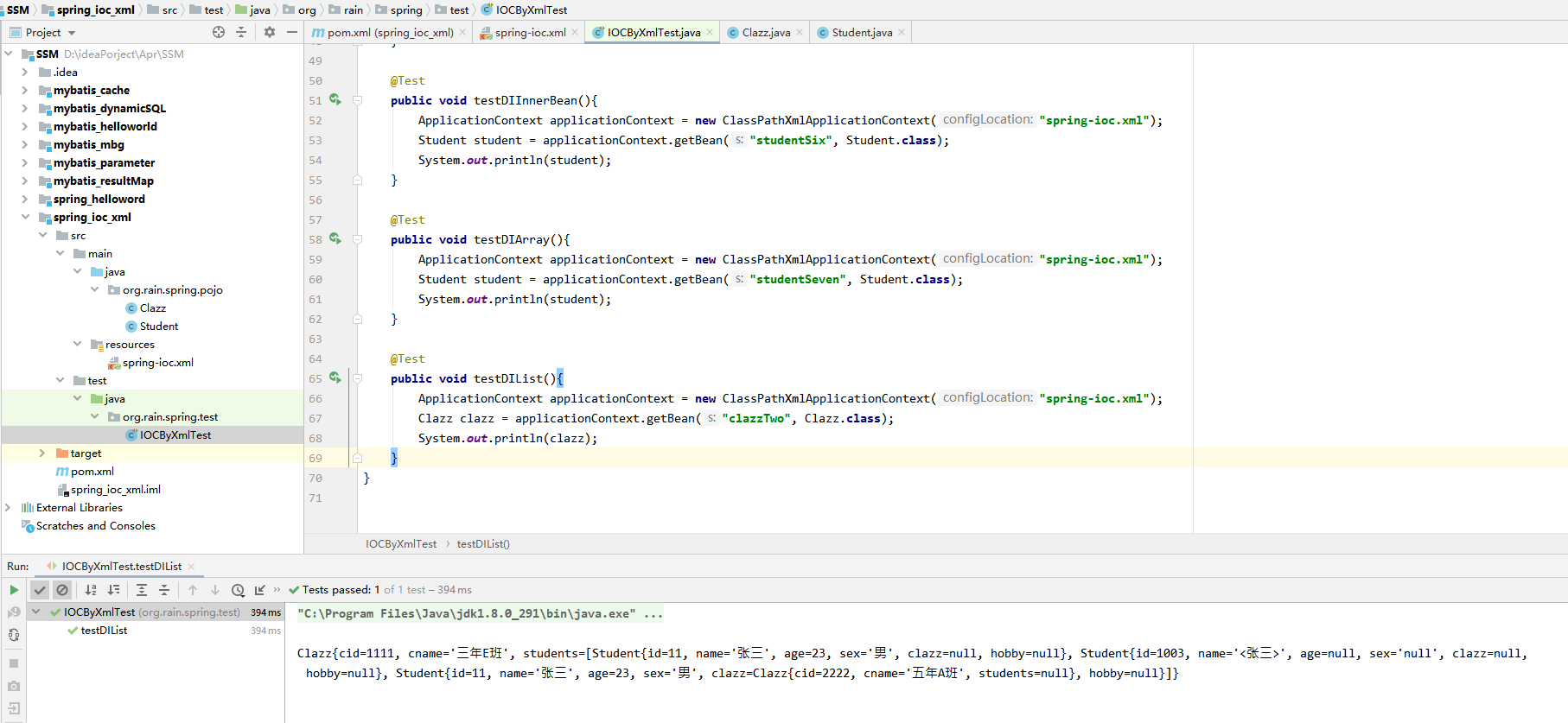 - @Test
- public void testDIList(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Clazz clazz = applicationContext.getBean("clazzTwo", Clazz.class);
- System.out.println(clazz);
- }
复制代码 4.8、为Map集合类型的属性赋值
4.8.1、创建教师类Teacher
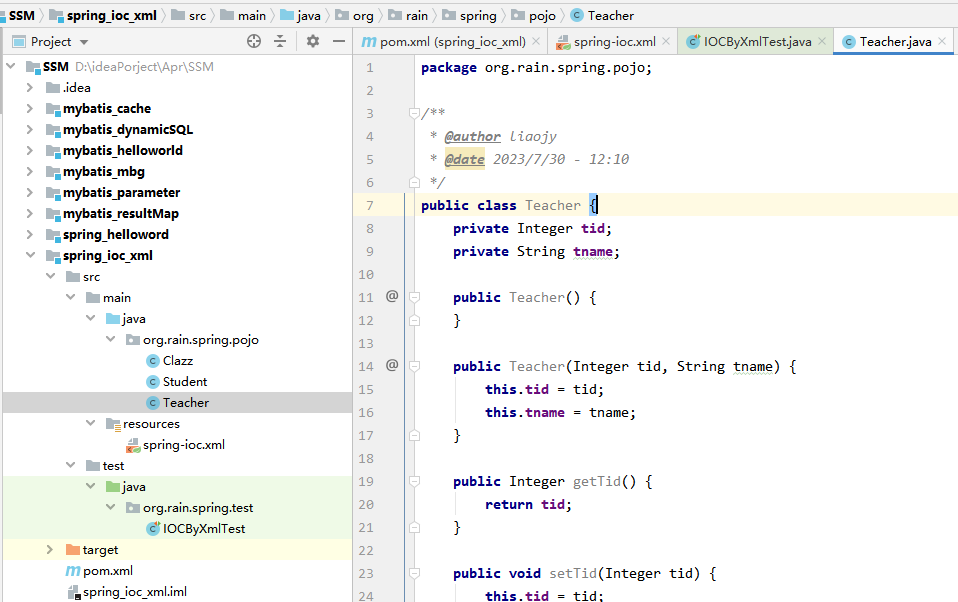 - package org.rain.spring.pojo;
- /**
- * @author liaojy
- * @date 2023/7/30 - 12:10
- */
- public class Teacher {
- private Integer tid;
- private String tname;
- public Teacher() {
- }
- public Teacher(Integer tid, String tname) {
- this.tid = tid;
- this.tname = tname;
- }
- public Integer getTid() {
- return tid;
- }
- public void setTid(Integer tid) {
- this.tid = tid;
- }
- public String getTname() {
- return tname;
- }
- public void setTname(String tname) {
- this.tname = tname;
- }
- @Override
- public String toString() {
- return "Teacher{" +
- "tid=" + tid +
- ", tname='" + tname + '\'' +
- '}';
- }
- }
复制代码 4.8.2、修改Student类
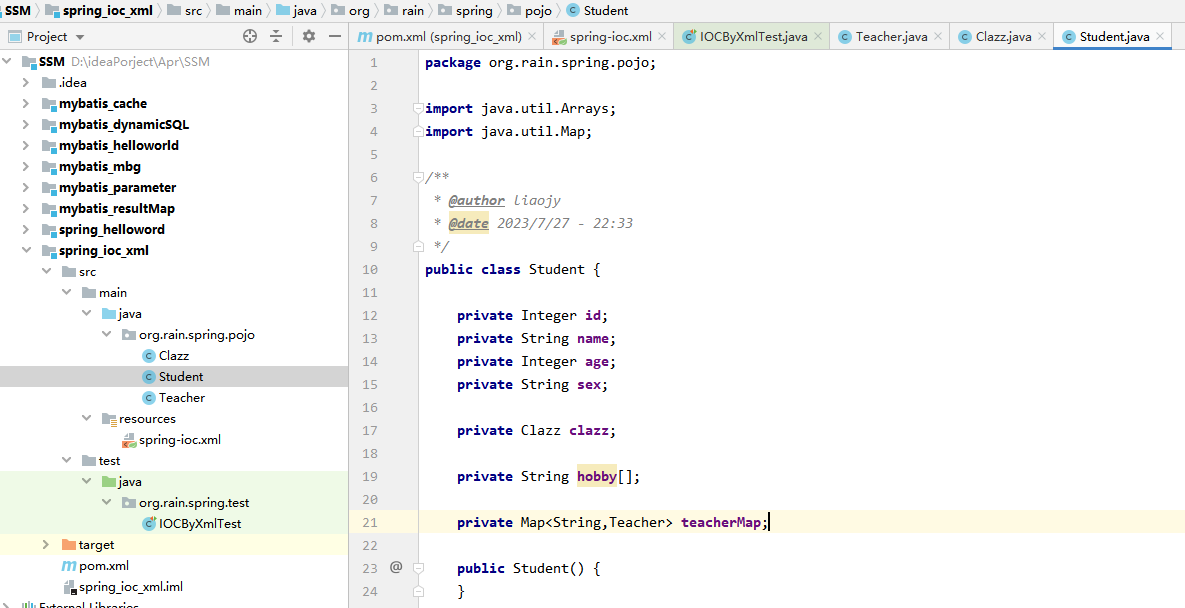
在Student类中添加或修改以下代码:
- private Map<String,Teacher> teacherMap;
- public Map<String, Teacher> getTeacherMap() {
- return teacherMap;
- }
- public void setTeacherMap(Map<String, Teacher> teacherMap) {
- this.teacherMap = teacherMap;
- }
- @Override
- public String toString() {
- return "Student{" +
- "id=" + id +
- ", name='" + name + '\'' +
- ", age=" + age +
- ", sex='" + sex + '\'' +
- ", clazz=" + clazz +
- ", hobby=" + Arrays.toString(hobby) +
- ", teacherMap=" + teacherMap +
- '}';
- }
复制代码 4.8.3、配置bean
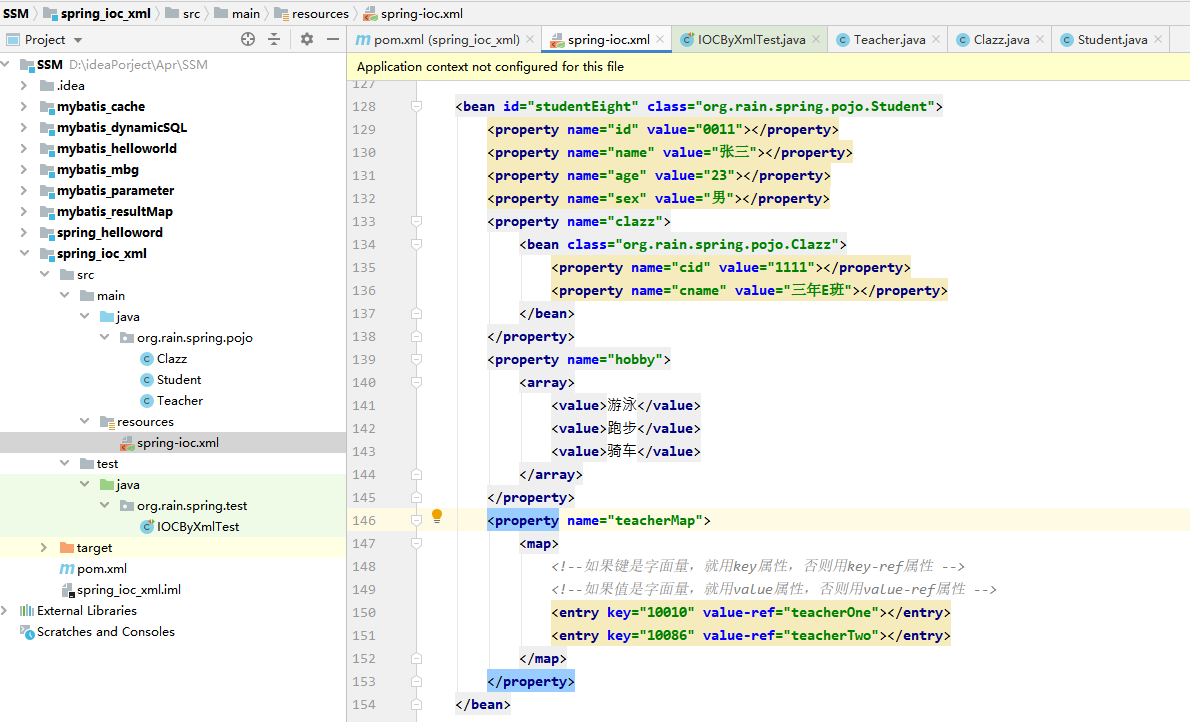 -
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> 游泳 跑步 骑车
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.8.4、测试
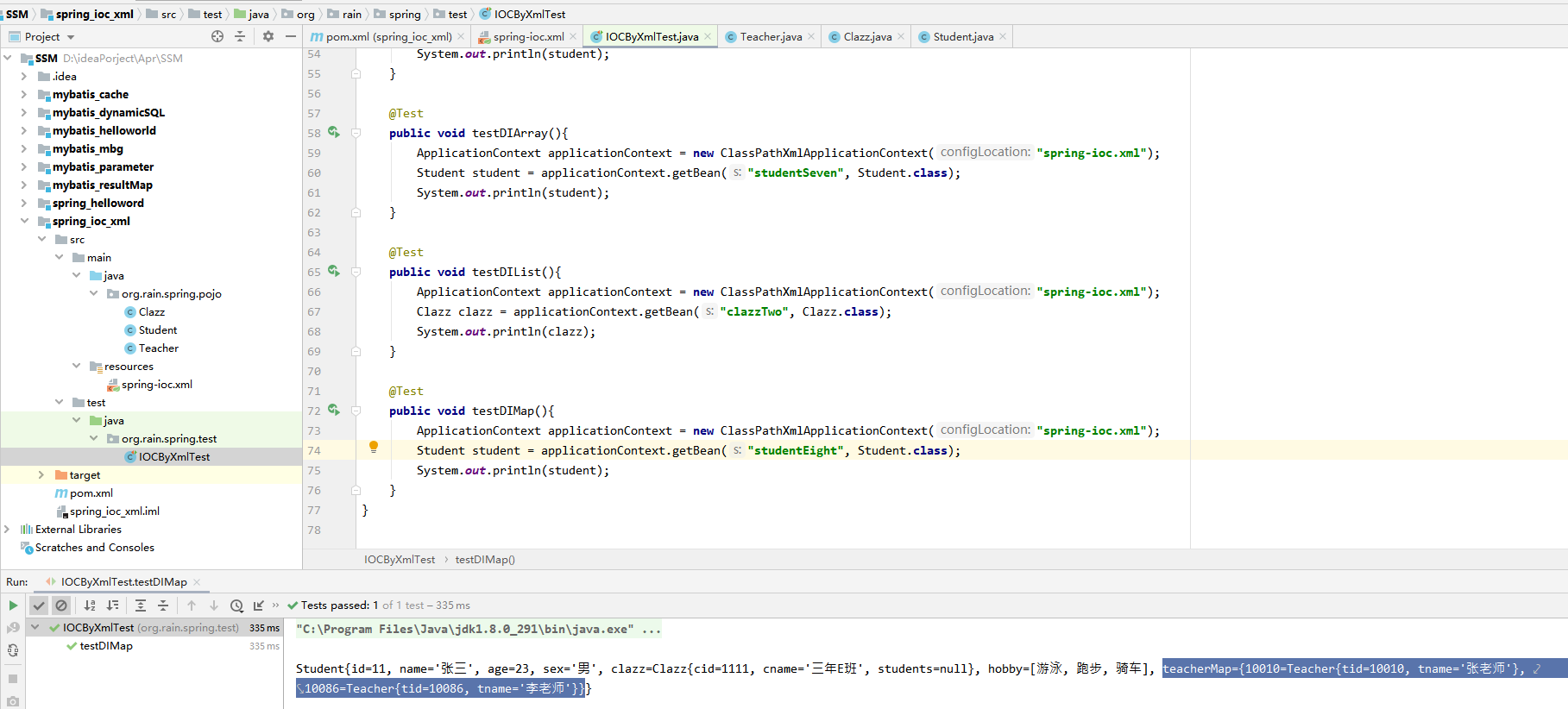 - @Test
- public void testDIMap(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentEight", Student.class);
- System.out.println(student);
- }
复制代码 4.9、为集合类型的属性赋值(解耦引用方式)
4.9.1、配置bean
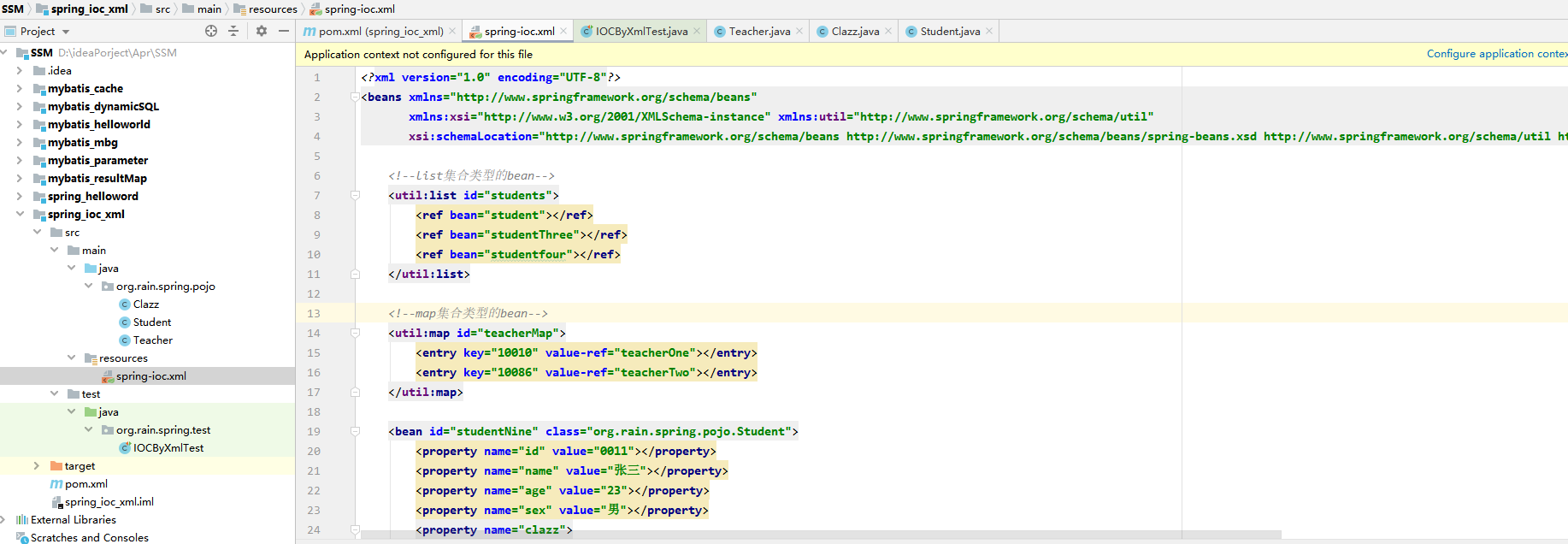
注意:使用util:list、util:map标签必须引入相应的XML命名空间
-
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean> <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean> 游泳 跑步 骑车 <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.9.2、测试
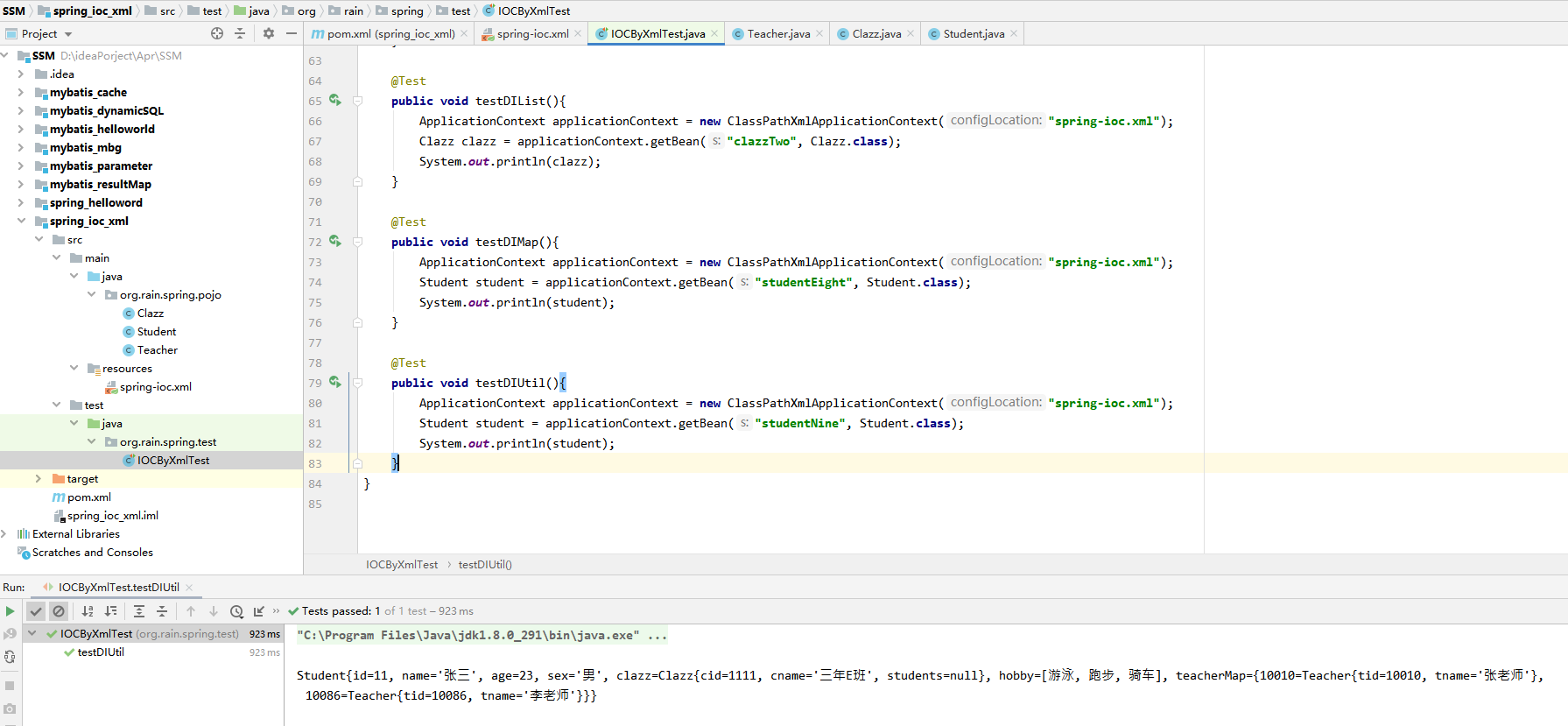 - @Test
- public void testDIUtil(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentNine", Student.class);
- System.out.println(student);
- }
复制代码 4.10、p命名空间(了解)
4.10.1、配置bean
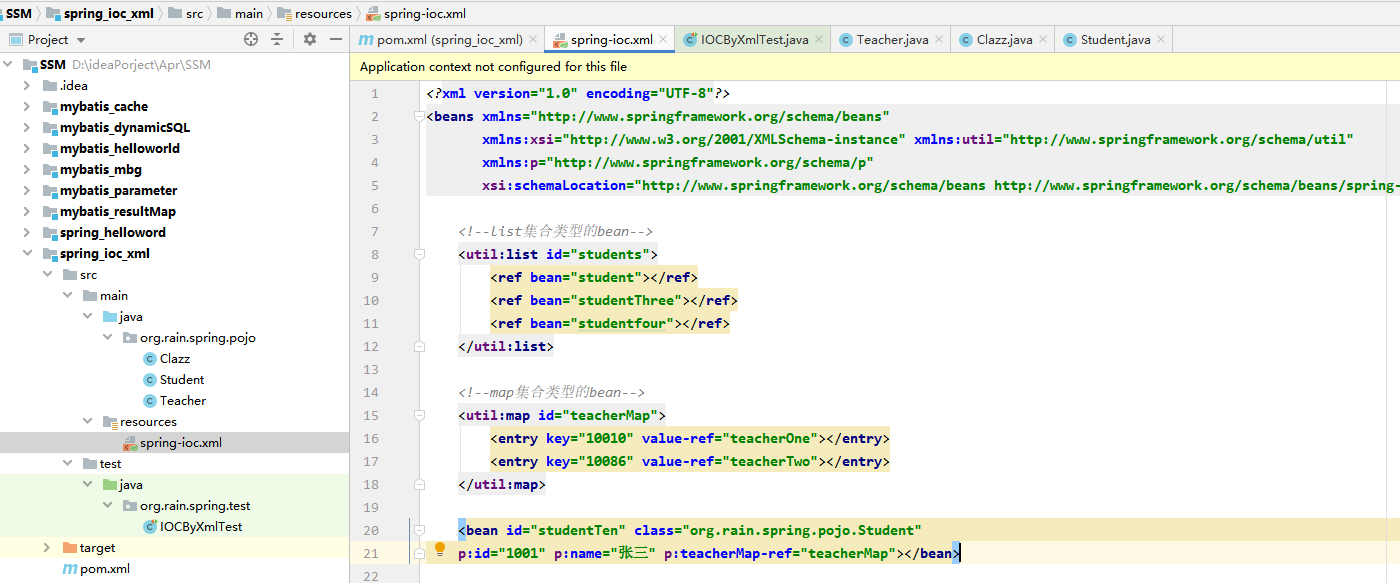
注意:p命名空间的依赖注入,本质上是属性方式(setter方法)的依赖注入
- <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean>
复制代码 4.10.2、测试
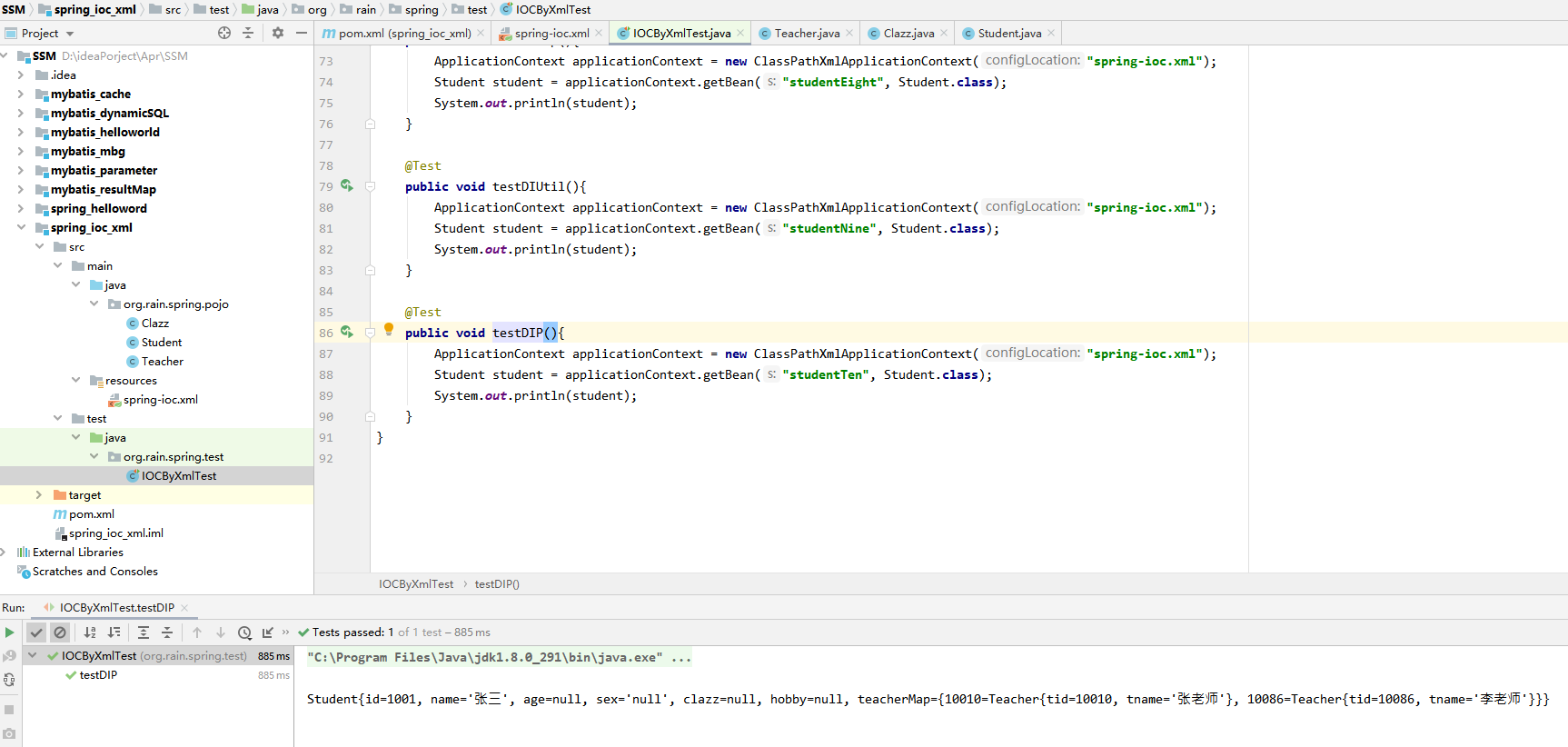 - @Test
- public void testDIP(){
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-ioc.xml");
- Student student = applicationContext.getBean("studentTen", Student.class);
- System.out.println(student);
- }
复制代码 4.11、引入外部属性文件
4.11.1、引入数据库相关依赖
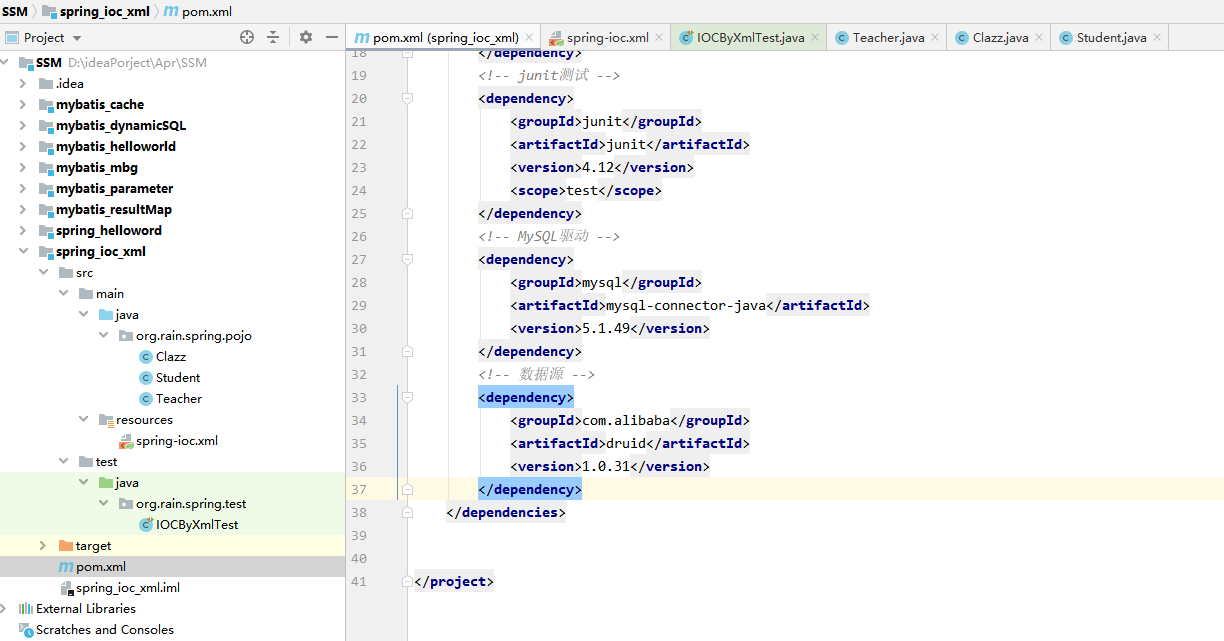 - <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean> mysql mysql-connector-java 5.1.49 <bean id="clazz" >
- <property name="cid" value="1111"></property>
- <property name="cname" value="三年E班"></property>
- </bean> com.alibaba druid 1.0.31
复制代码 4.11.2、创建外部属性文件
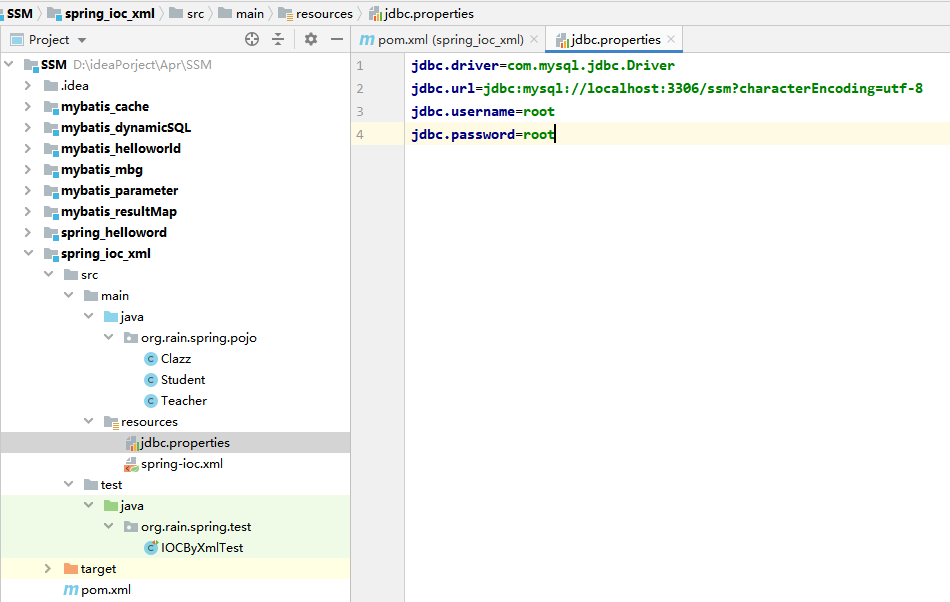 - jdbc.driver=com.mysql.jdbc.Driver
- jdbc.url=jdbc:mysql://localhost:3306/ssm?characterEncoding=utf-8
- jdbc.username=root
- jdbc.password=root
复制代码 4.11.3、配置bean
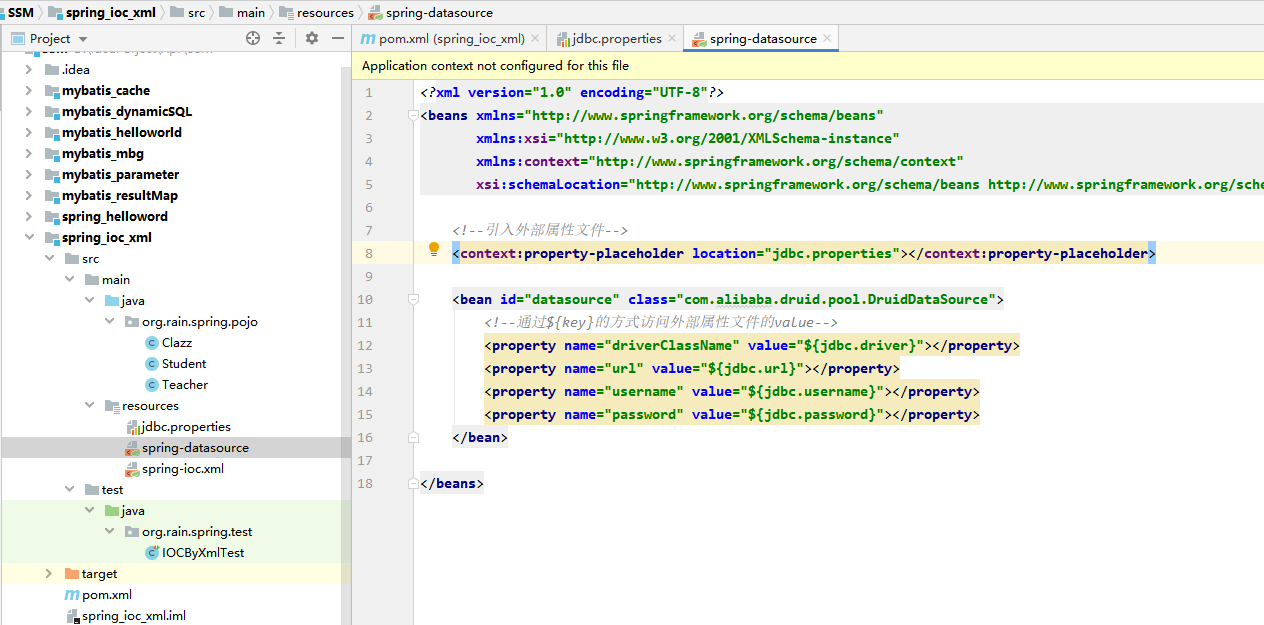
注意:使用context:property-placeholder标签必须引入相应的XML命名空间
-
- <bean id="student" >
- <property name="id" value="0011"></property>
- <property name="name" value="张三"></property>
- <property name="age" value="23"></property>
- <property name="sex" value="男"></property>
- </bean>
复制代码 4.11.4、测试
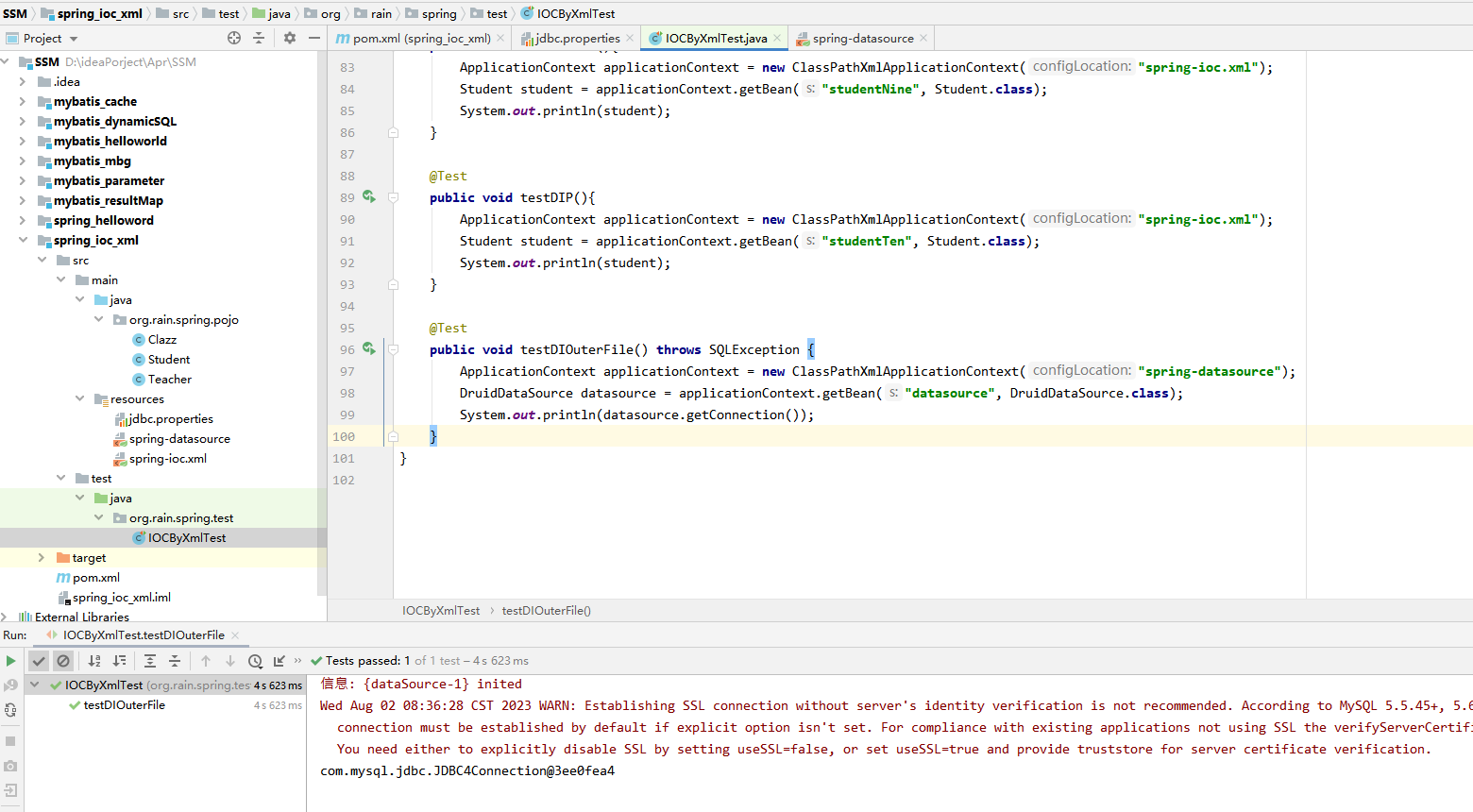 - @Test
- public void testDIOuterFile() throws SQLException {
- ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring-datasource");
- DruidDataSource datasource = applicationContext.getBean("datasource", DruidDataSource.class);
- System.out.println(datasource.getConnection());
- }
复制代码 免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |