马上注册,结交更多好友,享用更多功能,让你轻松玩转社区。
您需要 登录 才可以下载或查看,没有账号?立即注册
x
- #include <stdio.h>
- #include <Windows.h>
- #include <process.h>
- #include <conio.h>
- #include "MvCameraControl.h"
- bool g_bExit = false;
- // ch:等待按键输入 | en:Wait for key press
- void WaitForKeyPress(void)
- {
- while(!_kbhit())
- {
- Sleep(10);
- }
- _getch();
- }
- //相机参数
- bool PrintDeviceInfo(MV_CC_DEVICE_INFO* pstMVDevInfo)
- {
- if (NULL == pstMVDevInfo)
- {
- printf("The Pointer of pstMVDevInfo is NULL!\n");
- return false;
- }
- if (pstMVDevInfo->nTLayerType == MV_GIGE_DEVICE)
- {
- int nIp1 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0xff000000) >> 24);
- int nIp2 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x00ff0000) >> 16);
- int nIp3 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x0000ff00) >> 8);
- int nIp4 = (pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x000000ff);
- // ch:打印当前相机ip和用户自定义名字 | en:print current ip and user defined name
- printf("CurrentIp: %d.%d.%d.%d\n" , nIp1, nIp2, nIp3, nIp4);
- printf("UserDefinedName: %s\n\n" , pstMVDevInfo->SpecialInfo.stGigEInfo.chUserDefinedName);
- }
- else if (pstMVDevInfo->nTLayerType == MV_USB_DEVICE)
- {
- printf("UserDefinedName: %s\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.chUserDefinedName);
- printf("Serial Number: %s\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.chSerialNumber);
- printf("Device Number: %d\n\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.nDeviceNumber);
- }
- else
- {
- printf("Not support.\n");
- }
- return true;
- }
- static unsigned int __stdcall WorkThread(void* pUser)
- {
- int nRet = MV_OK;
- MV_FRAME_OUT stOutFrame = {0};
- MV_DISPLAY_FRAME_INFO stDisplayInfo = { 0 };
-
- MV_DISPLAY_FRAME_INFO 成员变量
- //void * hWnd //窗口句柄
- //unsigned char * pData //显示的数据
- //unsigned int nDataLen//数据长度
- //unsigned short nWidth//图像宽
- //unsigned short nHeight//图像高
- //enum MvGvspPixelType enPixelType//像素格式
- //unsigned int nRes[4]//保留
- HWND g_hwnd = GetForegroundWindow();//获取当前DOS窗口句柄
- while(true)
- {
- nRet = MV_CC_GetImageBuffer(pUser, &stOutFrame, 1000);
- if (nRet == MV_OK)
- {
- printf("Get Image Buffer: Width[%d], Height[%d], FrameNum[%d]\n",
- stOutFrame.stFrameInfo.nWidth, stOutFrame.stFrameInfo.nHeight, stOutFrame.stFrameInfo.nFrameNum);
- //将图片显示在DOS窗口上
- if (g_hwnd)
- {
- stDisplayInfo.hWnd = g_hwnd;
- stDisplayInfo.pData = stOutFrame.pBufAddr;// 指向图像数据的指针
- stDisplayInfo.nDataLen = stOutFrame.stFrameInfo.nFrameLen;//数据长度
- stDisplayInfo.nWidth = stOutFrame.stFrameInfo.nWidth; // 图像的宽度(像素)
- stDisplayInfo.nHeight = stOutFrame.stFrameInfo.nHeight; // 图像的高度(像素)
- stDisplayInfo.enPixelType = stOutFrame.stFrameInfo.enPixelType; // 像素类型,如黑白、RGB、Bayer等
- MV_CC_DisplayOneFrame(pUser, &stDisplayInfo);
- }
- nRet = MV_CC_FreeImageBuffer(pUser, &stOutFrame);
- if(nRet != MV_OK)
- {
- printf("Free Image Buffer fail! nRet [0x%x]\n", nRet);
- }
- }
- else
- {
- printf("Get Image fail! nRet [0x%x]\n", nRet);
- }
- if(g_bExit)
- {
- break;
- }
- }
- return 0;
- }
- int main()
- {
- int nRet = MV_OK;
- void* handle = NULL;
- do
- {
- // ch:枚举设备 | en:Enum device
- MV_CC_DEVICE_INFO_LIST stDeviceList;
- memset(&stDeviceList, 0, sizeof(MV_CC_DEVICE_INFO_LIST));
- nRet = MV_CC_EnumDevices(MV_GIGE_DEVICE | MV_USB_DEVICE, &stDeviceList);
- if (MV_OK != nRet)
- {
- printf("Enum Devices fail! nRet [0x%x]\n", nRet);
- break;
- }
- if (stDeviceList.nDeviceNum > 0)
- {
- for (unsigned int i = 0; i < stDeviceList.nDeviceNum; i++)
- {
- printf("[device %d]:\n", i);
- MV_CC_DEVICE_INFO* pDeviceInfo = stDeviceList.pDeviceInfo[i];
- if (NULL == pDeviceInfo)
- {
- break;
- }
- PrintDeviceInfo(pDeviceInfo);
- }
- }
- else
- {
- printf("Find No Devices!\n");
- break;
- }
- printf("Please Input camera index(0-%d):", stDeviceList.nDeviceNum-1);
- unsigned int nIndex = 0;
- scanf_s("%d", &nIndex);
- if (nIndex >= stDeviceList.nDeviceNum)
- {
- printf("Input error!\n");
- break;
- }
- // ch:选择设备并创建句柄 | en:Select device and create handle
- nRet = MV_CC_CreateHandle(&handle, stDeviceList.pDeviceInfo[nIndex]);
- if (MV_OK != nRet)
- {
- printf("Create Handle fail! nRet [0x%x]\n", nRet);
- break;
- }
- // ch:打开设备 | en:Open device
- nRet = MV_CC_OpenDevice(handle);
- if (MV_OK != nRet)
- {
- printf("Open Device fail! nRet [0x%x]\n", nRet);
- break;
- }
- // ch:探测网络最佳包大小(只对GigE相机有效) | en:Detection network optimal package size(It only works for the GigE camera)
- if (stDeviceList.pDeviceInfo[nIndex]->nTLayerType == MV_GIGE_DEVICE)
- {
- int nPacketSize = MV_CC_GetOptimalPacketSize(handle);
- if (nPacketSize > 0)
- {
- nRet = MV_CC_SetIntValue(handle,"GevSCPSPacketSize",nPacketSize);
- if(nRet != MV_OK)
- {
- printf("Warning: Set Packet Size fail nRet [0x%x]!", nRet);
- }
- }
- else
- {
- printf("Warning: Get Packet Size fail nRet [0x%x]!", nPacketSize);
- }
- }
- // ch:设置触发模式为off | en:Set trigger mode as off
- nRet = MV_CC_SetEnumValue(handle, "TriggerMode", 0);
- if (MV_OK != nRet)
- {
- printf("Set Trigger Mode fail! nRet [0x%x]\n", nRet);
- break;
- }
- // ch:开始取流 | en:Start grab image
- nRet = MV_CC_StartGrabbing(handle);
- if (MV_OK != nRet)
- {
- printf("Start Grabbing fail! nRet [0x%x]\n", nRet);
- break;
- }
- unsigned int nThreadID = 0;
- void* hThreadHandle = (void*) _beginthreadex( NULL , 0 , WorkThread , handle, 0 , &nThreadID );
- if (NULL == hThreadHandle)
- {
- break;
- }
- //在C/C++编程中,_beginthreadex 是一个用于在Microsoft Visual Studio环境下创建线程的函数。
- //这个函数定义在<process.h>头文件中,它允许你在一个独立的线程中启动一个新的执行路径。下面是对给定代码行的解释:
- //NULL:这是线程属性指针,通常设置为NULL表示使用默认属性。
- //0:这是线程堆栈大小。如果设置为0,那么系统会根据默认值或编译器设置来决定堆栈大小。
- //WorkThread:这是指向线程函数的指针,当线程开始运行时,将从这里开始执行。
- //handle:这是传递给WorkThread函数的参数。当WorkThread被调用时,它的第一个参数将接收这个值。
- //0:这是线程的优先级增量。通常设置为0,意味着线程将具有默认的优先级。
- //&nThreadID:这是一个指向整型变量的指针,_beginthreadex函数将返回创建的新线程的ID到这个变量中。
- printf("Press a key to stop grabbing.\n");
- WaitForKeyPress();
- g_bExit = true;
- Sleep(1000);
- // ch:停止取流 | en:Stop grab image
- nRet = MV_CC_StopGrabbing(handle);
- if (MV_OK != nRet)
- {
- printf("Stop Grabbing fail! nRet [0x%x]\n", nRet);
- break;
- }
- // ch:关闭设备 | Close device
- nRet = MV_CC_CloseDevice(handle);
- if (MV_OK != nRet)
- {
- printf("ClosDevice fail! nRet [0x%x]\n", nRet);
- break;
- }
- // ch:销毁句柄 | Destroy handle
- nRet = MV_CC_DestroyHandle(handle);
- if (MV_OK != nRet)
- {
- printf("Destroy Handle fail! nRet [0x%x]\n", nRet);
- break;
- }
- } while (0);
- if (nRet != MV_OK)
- {
- if (handle != NULL)
- {
- MV_CC_DestroyHandle(handle);
- handle = NULL;
- }
- }
- printf("Press a key to exit.\n");
- WaitForKeyPress();
- return 0;
- }
复制代码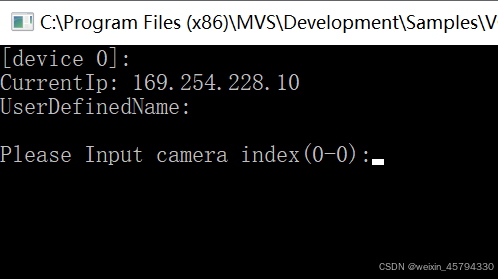
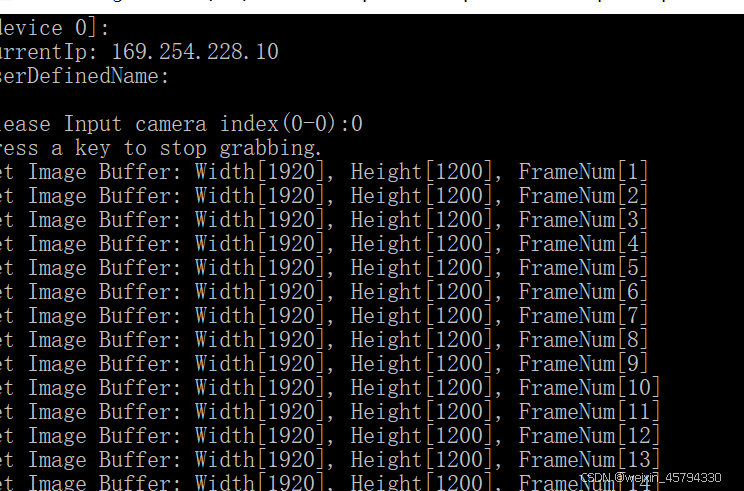
本代码将会把工业相机拍摄图像显示在DOS窗体上
本文仅供学习交流,严禁用于商业用途,如本文涉及侵权请及时接洽将于24小时内删除
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |