马上注册,结交更多好友,享用更多功能,让你轻松玩转社区。
您需要 登录 才可以下载或查看,没有账号?立即注册
x
话不多说,直接上干货:
一、首先去官网注册一个账号百度智能云-登录 (baidu.com),注册完成后等待审核,审核后就可以去控制台操纵啦!
二、根据官网介绍,由于文心一言属于收费产品(也有免费的,但功能限定),因此发起可以充值几块钱,足以做实验用了。我这里用的是“千帆大模型”产品。
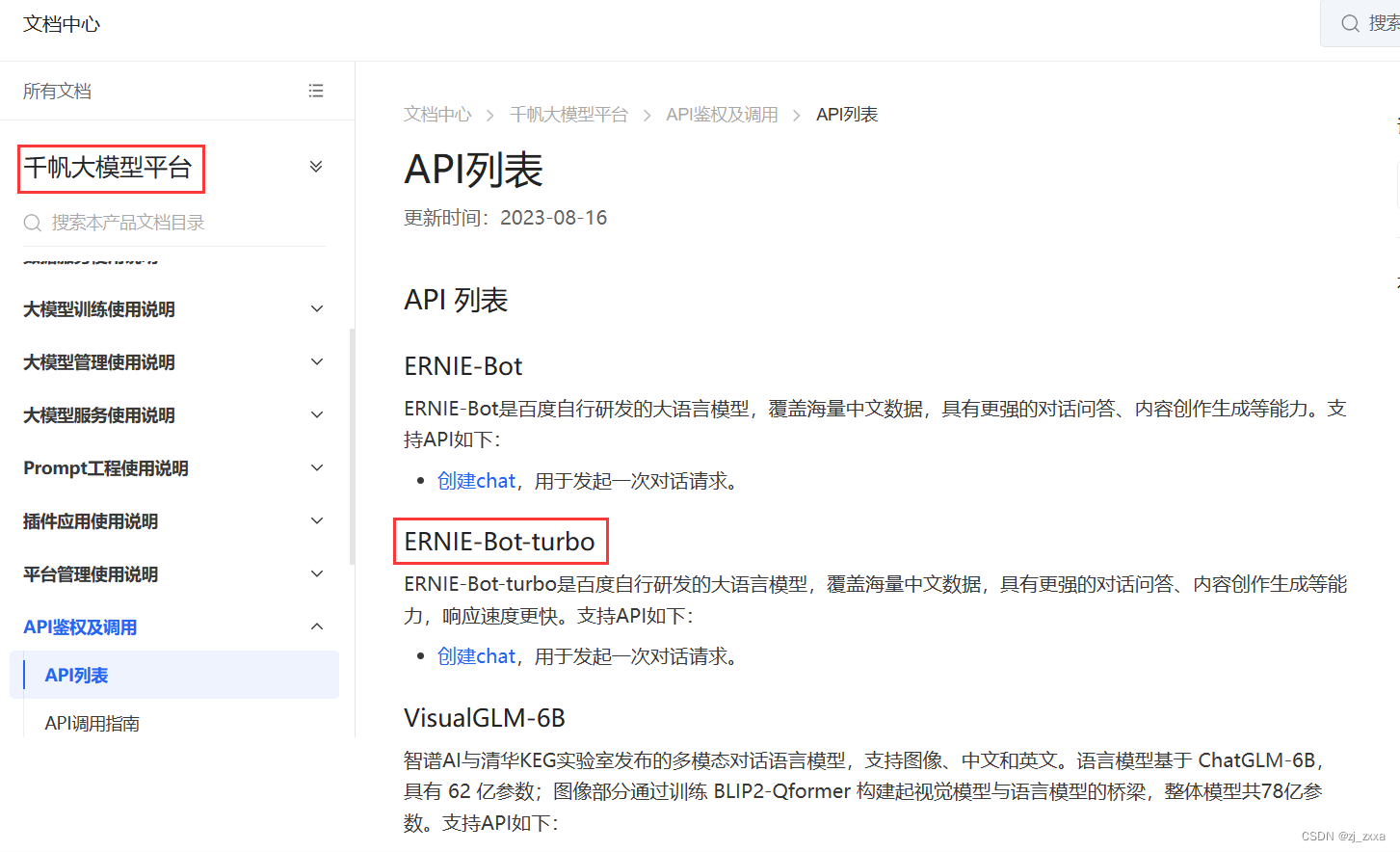
三、代码实现过程:
1)调用工具:
- package utils;
- import com.alibaba.fastjson.JSON;
- import com.alibaba.fastjson.JSONArray;
- import com.alibaba.fastjson.JSONObject;
- import okhttp3.*;
- import java.io.*;
- import java.net.URLEncoder;
- import java.util.ArrayList;
- import java.util.HashMap;
- public class ChineseGPT {
- static final OkHttpClient HTTP_CLIENT = new OkHttpClient().newBuilder().build();
- public static void main(String []args) throws IOException{
- //1、获取token
- String access_token = new ChineseGPT().getWenxinToken();
- //2、访问数据
- String requestMethod = "POST";
- String body = URLEncoder.encode("junshi","UTF-8");//设置要传的信息
- String url = "https://aip.baidubce.com/rpc/2.0/ai_custom/v1/wenxinworkshop/chat/eb-instant?access_token="+access_token;//post请求时格式
- //测试:访问聚合数据的地区新闻api
- HashMap<String, String> msg = new HashMap<>();
- msg.put("role","user");
- msg.put("content", "帮我写一篇关于介绍吉林市的小短文,大概100字左右");
- ArrayList<HashMap> messages = new ArrayList<>();
- messages.add(msg);
- HashMap<String, Object> requestBody = new HashMap<>();
- requestBody.put("messages", messages);
- String outputStr = JSON.toJSONString(requestBody);
- JSON json = HttpRequest.httpRequest(url,requestMethod,outputStr,"application/json");
- System.out.println(json);
- }
- public String getWenxinToken() throws IOException {
- MediaType mediaType = MediaType.parse("application/json");
- RequestBody body = RequestBody.create(mediaType, "");
- Request request = new Request.Builder()
- .url("https://aip.baidubce.com/oauth/2.0/token?client_id=xxxxxxxxxxxxxxxxxx&client_secret=xxxxxxxxxxxxxxxxxx&grant_type=client_credentials") //按官网要求填写你申请的key和相关秘钥
- .method("POST", body)
- .addHeader("Content-Type", "application/json")
- .addHeader("Accept", "application/json")
- .build();
- Response response = HTTP_CLIENT.newCall(request).execute();
- String s = response.body().string();
- JSONObject objects = JSONArray.parseObject(s);
- String msg = objects.getString("access_token");
- System.out.println(msg);
- return msg;
- }
- }
复制代码 2)哀求工具:
- package utils;
- import com.alibaba.fastjson.JSONObject;
- import javax.net.ssl.HttpsURLConnection;
- import java.io.*;
- import java.net.ConnectException;
- import java.net.HttpURLConnection;
- import java.net.URL;
- import java.net.URLConnection;
- import java.util.List;
- import java.util.Map;
- public class HttpRequest {
-
- /**
- * post/GET远程请求接口并得到返回结果
- *
- * @param requestUrl 请求地址
- * @param requestMethod 请求方法post/GET
- * @return
- */
- public static JSONObject httpRequest(String requestUrl, String requestMethod, String outputStr,String contentType) {
- JSONObject jsonObject = null;
- StringBuffer buffer = new StringBuffer();
- try {
- URL url = new URL(requestUrl);
- HttpURLConnection httpUrlConn = (HttpURLConnection)url.openConnection();
- httpUrlConn.setDoOutput(true);
- httpUrlConn.setDoInput(true);
- httpUrlConn.setUseCaches(false);
- // 设置请求方式(GET/POST)
- httpUrlConn.setRequestMethod(requestMethod);
- //设置头信息
- // httpUrlConn.setRequestProperty("Content-Type","application/x-www-form-urlencoded;charset=UTF-8");
- httpUrlConn.setRequestProperty("Content-Type",contentType);
- httpUrlConn.setRequestProperty("Accept","application/json;charset=UTF-8");
- // 设置连接主机服务器的超时时间:15000毫秒
- httpUrlConn.setConnectTimeout(15000);
- // 设置读取远程返回的数据时间:60000毫秒
- httpUrlConn.setReadTimeout(60000);
- if ("GET".equalsIgnoreCase(requestMethod)){
- httpUrlConn.connect();
- }
- // 当有数据需要提交时
- if (null != outputStr) {
- OutputStream outputStream = httpUrlConn.getOutputStream();
- // 注意编码格式,防止中文乱码
- outputStream.write(outputStr.getBytes("UTF-8"));
- outputStream.close();
- }
- // 将返回的输入流转换成字符串
- InputStream inputStream = httpUrlConn.getInputStream();
- InputStreamReader inputStreamReader = new InputStreamReader(inputStream, "utf-8");
- BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
- String str = null;
- while ((str = bufferedReader.readLine()) != null) {
- buffer.append(str);
- }
- bufferedReader.close();
- inputStreamReader.close();
- // 释放资源
- inputStream.close();
- inputStream = null;
- httpUrlConn.disconnect();
- jsonObject = JSONObject.parseObject(buffer.toString());
- } catch (ConnectException ce) {
- } catch (Exception e) {
- }
- return jsonObject;
- }
- }
复制代码 3)执行main方法执行即可。
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |