创建一个Java Web API涉及多个步骤和技能栈,包括项目设置、依赖管理、数据访问层实现、业务逻辑实现、控制层开辟以及测试和部署。在这篇详解中,我将领导你通过一个完整的Java Web API实现流程,采用Spring Boot和MyBatis-Plus作为主要技能工具。
一、项目初始化
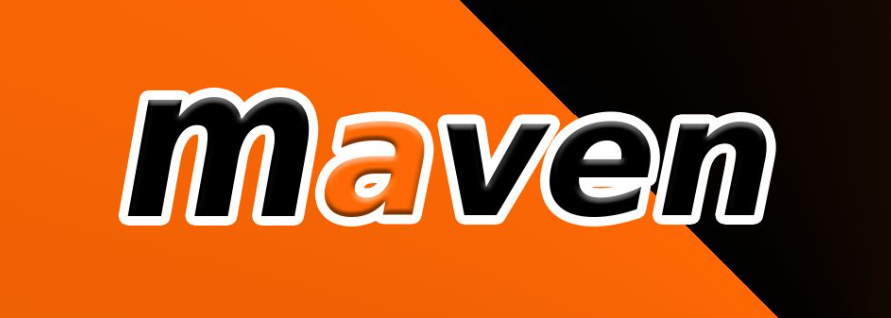
- 选择技能栈:起首,需要确定使用Spring Boot作为框架,MyBatis-Plus作为ORM框架,Spring Web作为Rest接口的实现工具。
- 创建Maven项目:采用Maven举行依赖管理,Spring Initializr是一个很好的工具,可以快速生成基本的Spring Boot项目布局。
- 设置pom.xml文件:
- <dependencyManagement>
- <dependencies>
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-dependencies</artifactId>
- <version>2.6.4</version>
- <type>pom</type>
- <scope>import</scope>
- </dependency>
- </dependencies>
- </dependencyManagement>
- <dependencies>
- <!-- Spring Boot Starter Web for building web, including RESTful applications -->
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-web</artifactId>
- </dependency>
- <!-- MyBatis-Plus for data access layer -->
- <dependency>
- <groupId>com.baomidou</groupId>
- <artifactId>mybatis-plus-boot-starter</artifactId>
- <version>3.4.3.4</version>
- </dependency>
- <!-- MySQL driver -->
- <dependency>
- <groupId>mysql</groupId>
- <artifactId>mysql-connector-java</artifactId>
- <scope>runtime</scope>
- </dependency>
- <!-- Other necessary dependencies -->
- ...
- </dependencies>
复制代码 二、项目目录布局
通常的Spring Boot项目目录布局如下:
- src
- └── main
- ├── java
- │ └── com
- │ └── example
- │ ├── controller
- │ ├── service
- │ ├── serviceImpl
- │ ├── mapper
- │ ├── entity
- │ └── dto
- └── resources
- ├── static
- ├── templates
- └── application.yml
复制代码 三、设置文件
application.yml文件用于设置数据库连接和MyBatis-Plus设置:
- spring:
- datasource:
- url: jdbc:mysql://localhost:3306/yourdb
- username: root
- password: password
- driver-class-name: com.mysql.cj.jdbc.Driver
- mybatis-plus:
- mapper-locations: classpath:/mapper/*Mapper.xml
- type-aliases-package: com.example.entity
复制代码 四、实体层
创建实体类,如User:
- package com.example.entity;
- import com.baomidou.mybatisplus.annotation.TableId;
- import com.baomidou.mybatisplus.annotation.TableName;
- @TableName("user")
- public class User {
- @TableId
- private Long id;
- private String name;
- private Integer age;
- // Getters and Setters
- }
复制代码 五、数据访问层
实现数据访问层接口:
- package com.example.mapper;
- import com.baomidou.mybatisplus.core.mapper.BaseMapper;
- import com.example.entity.User;
- import org.apache.ibatis.annotations.Mapper;
- @Mapper
- public interface UserMapper extends BaseMapper<User> {
- }
复制代码 六、服务层
编写服务接口和实现类:
- package com.example.service;
- import com.example.entity.User;
- import java.util.List;
- public interface UserService {
- List<User> getAllUsers();
- User getUserById(Long id);
- void createUser(User user);
- void updateUser(User user);
- void deleteUser(Long id);
- }
复制代码- package com.example.serviceImpl;
- import com.example.entity.User;
- import com.example.mapper.UserMapper;
- import com.example.service.UserService;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.stereotype.Service;
- import java.util.List;
- @Service
- public class UserServiceImpl implements UserService {
- @Autowired
- private UserMapper userMapper;
- @Override
- public List<User> getAllUsers() {
- return userMapper.selectList(null);
- }
- @Override
- public User getUserById(Long id) {
- return userMapper.selectById(id);
- }
- @Override
- public void createUser(User user) {
- userMapper.insert(user);
- }
- @Override
- public void updateUser(User user) {
- userMapper.updateById(user);
- }
- @Override
- public void deleteUser(Long id) {
- userMapper.deleteById(id);
- }
- }
复制代码 七、控制层
创建RESTful接口:
- package com.example.controller;
- import com.example.entity.User;
- import com.example.service.UserService;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.web.bind.annotation.*;
- import java.util.List;
- @RestController
- @RequestMapping("/api/users")
- public class UserController {
- @Autowired
- private UserService userService;
- @GetMapping
- public List<User> getAllUsers() {
- return userService.getAllUsers();
- }
- @GetMapping("/{id}")
- public User getUserById(@PathVariable Long id) {
- return userService.getUserById(id);
- }
- @PostMapping
- public void createUser(@RequestBody User user) {
- userService.createUser(user);
- }
- @PutMapping
- public void updateUser(@RequestBody User user) {
- userService.updateUser(user);
- }
- @DeleteMapping("/{id}")
- public void deleteUser(@PathVariable Long id) {
- userService.deleteUser(id);
- }
- }
复制代码 八、测试和部署
1. 测试
- 使用JUnit举行单位测试。
- 使用Postman等工具举行API测试。
2. 部署
- 生成.jar文件,通过下令java -jar <jar-file>.jar运行应用。
- 也可以在云服务平台如AWS, Azure, 大概阿里云上举行部署。
九、MyBatis-Plus特性分析
MyBatis-Plus是MyBatis的一个增强工具,设计为简化开辟、提高服从。以下是MyBatis-Plus的一些关键特性和设置:
- CRUD操纵:MyBatis-Plus扩展了MyBatis的基本功能,提供主动生成的CRUD接口,开辟者无需编写XML文件来实现基本的增编削查功能。
- 分页插件:内置分页插件,轻松实现物理分页和信息统计,淘汰了开辟分页逻辑的复杂性。
- 主动填充:支持主动填充计谋,帮助企业主动维护创建时间、更新时间等字段。
- 代码生成器:提供代码生成器,可以通过简朴的设置主动生成符合业务需求的代码,快速搭建基础业务。
- 多种插件支持:插件机制非常机动,如乐观锁插件、防止全表更新操纵插件,满足各种复杂的业务场景。
- 高性能:基于MyBatis,深度优化了单表操纵,兼容MyBatis所有功能。
例如,分页插件的设置使用:
- @Configuration
- public class MybatisPlusConfig {
- @Bean
- public PaginationInterceptor paginationInterceptor() {
- return new PaginationInterceptor();
- }
- }
复制代码 在实现同一的CRUD操纵的时候,
- Page<User> page = new Page<>(1, 10);
- IPage<User> userIPage = userMapper.selectPage(page, null);
复制代码 上述代码可以轻松实现用户数据的分页查询。
将来的改进点可以是结合Spring Cloud举行整个体系微服务化,实现更大的扩展性和机动性。
总结:Java Web API实现结合Spring Boot和MyBatis-Plus可以大大简化开辟中的数据访问复杂性,优化开辟流程并增强体系的可维护性。理解并合理运用MyBatis-Plus中的各种特性,更能使开辟事半功倍。
- //python 因为爱,所以学
- print("Hello, Python!")
复制代码 关注我,不迷路,共学习,同进步
关注我,不迷路,共学习,同进步
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |