1.项目需求
开发一个包罗门生信息、课程信息和成绩信息的门生管理体系。这可以让用户轻松地添加、删除、编辑和搜刮门生信息和课程信息。
(1)要求使用数据库存储信息,数据库中要有3个表 门生信息表(学号 姓名 性别 年龄 专业 班级) 和 课程信息表(学号、姓名、课程、学分)用户表用于登录(账号 密码 权限)
(2)GUI界面实现 登录和增编削查4个功能
(3)用户权限设置,普通同砚可以查看全部和改自己的信息,管理员可以更改全部。
2.项目框架
bean包:此中包罗了门生信息类StudentInfo,课程信息类CourseInfo,用户信息类Users;
JDBCUtil包:工具包,此中的JDBCUtil类包罗了连接数据库,资源关闭等基础功能的方法。
Function包:功能包,此中的Function类涵盖了实现指定界面的功能方法。此中还是调用了JDBCUtil包中的方法。
MyFrame包:界面包,此中有每一个界面的界面类
UunitDemo包:测试包,里面有单元测试类JuniTestDemo、JunitTestFrame,以及测试类TestDemo
该项目主要实现流程是:通过界面类中获取文本框中的数据,在根据获得的数据通过调用功能包中的方法与数据进行交互并返回一个结果(或对象),再将返回的数据填入文本框中。当然在实现每一个模块后,都可以在测试包中进行单独模块的测试,好比单独测试功能类中的某一个方法是否有用,或者单独测试某一个界面是否合理,在每一个模块都测试乐成后,就可以将整个项目进行运行了。大多数情况下是没有太大的题目的,如许就可以制止运行项目时出现奇奇怪怪的错误。
3.项目概述
利用门生学号将三张表接洽起来。即学号同样也是账号,那么这三张表都有学号这一个共同的属性,利用学号可同时操作这三张表。具体实现思路如下:
1、在数据库创建相对应的三张表
2、利用JDBC操作数据库
(1)基于三张表创建三个对应的类。
(2)创建工具类。工具类分为实现对表进行增编削、查询、连接数据库、关闭资源等基本操作。
(3)基于工具类从而创建对应的功能类。每个功能类相对应一个操作界面(如增长门生信息界面)。功能类 通过界面传入的数据进行实现相对应的功能。
(4)基于GUI设计门生管理体系界面。每一个界面创建一个界面类,该界面利用输入的数据从而调用对应该 界面功能的功能类,从而实现每一个界面的功能。
- (5)创建单元测试类。创建多个单元测试类,每一个单元测试类都测试每一个模块的功能类以及每一个界面 功能的实现。比如:单独测试增加学生信息功能类、修改课程信息界面类等等。
- (6)创建测试类。通过测试类进行整体地测试该项目。
复制代码 4.项目设计
1.建立数据库
在Navicat或SQLyog上使用以下SQL语句创建三张表
同时,先声明我的数据库账号为root,密码为root,数据库名为db01
建立门生信息表
- # 创建学生信息表
- CREATE TABLE studentinfo(
- num INT(11),
- NAME VARCHAR(11),
- gender VARCHAR(11),
- age INT(11)
- );
- # 插入学生信息
- INSERT INTO studentinfo VALUE(1,'麦当','男',16);
- INSERT INTO studentinfo VALUE(2,'笛亚','女',16);
- INSERT INTO studentinfo VALUE(3,'柯南','男',7);
- INSERT INTO studentinfo VALUE(4,'小哀','女',7);
- INSERT INTO studentinfo VALUE(5,'新一','男',17);
- INSERT INTO studentinfo VALUE(6,'小兰','女',17);
- INSERT INTO studentinfo VALUE(7,'沸羊羊','男',4);
- INSERT INTO studentinfo VALUE(8,'美羊羊','女',4);
复制代码
建立课程信息表
- # 创建课程信息表
- CREATE TABLE courseinfo(
- num INT(11),
- NAME VARCHAR(11),
- course VARCHAR(11),
- credit VARCHAR(11)
- );
- #插入课程信息
- INSERT INTO courseinfo VALUE(1,'麦当','java',5);
- INSERT INTO courseinfo VALUE(2,'笛亚','c++',5);
- INSERT INTO courseinfo VALUE(3,'柯南','c#',4);
- INSERT INTO courseinfo VALUE(4,'小哀','c',3);
- INSERT INTO courseinfo VALUE(5,'新一','python',3);
- INSERT INTO courseinfo VALUE(6,'小兰','php',4);
- INSERT INTO courseinfo VALUE(7,'沸羊羊','SQL',3);
- INSERT INTO courseinfo VALUE(8,'美羊羊','HTML',5);
复制代码
建立用户信息表
- #创建用户信息表
- CREATE TABLE users(
- USER VARCHAR(11),
- passworld VARCHAR(11),
- Privilege VARCHAR(11)
- );
- #插入用户信息
- INSERT INTO users VALUE('1','555','管理员');
- INSERT INTO users VALUE('2','666','普通用户');
- INSERT INTO users VALUE('3','444','普通用户');
- INSERT INTO users VALUE('4','444','管理员');
- INSERT INTO users VALUE('5','444','管理员');
- INSERT INTO users VALUE('6','444','普通用户');
- INSERT INTO users VALUE('7','444','管理员');
- INSERT INTO users VALUE('8','444','普通用户');
复制代码
注:字段名大小写需要保持同等,否则使用JDBC操作过程中无法获取与属性同名的字段数据。
2.项目功能
1.创建门生类、课程类和用户类
- //创建学生类
- package bean;
- public class StudentInfo {
- private int num;
- private String name;
- private String gender;
- private int age;
- private String major;
- private String classes;
- public StudentInfo() {
- }
- public StudentInfo(int num, String name, String gender, int age) {
- this.num = num;
- this.name = name;
- this.gender = gender;
- this.age = age;
- }
- public int getNum() {
- return num;
- }
- public String getName() {
- return name;
- }
- public String getGender() {
- return gender;
- }
- public int getAge() {
- return age;
- }
- public String getMajor() {
- return major;
- }
- public String getClasses() {
- return classes;
- }
- public void setNum(int num) {
- this.num = num;
- }
- public void setName(String name) {
- this.name = name;
- }
- public void setGender(String gender) {
- this.gender = gender;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setMajor(String major) {
- this.major = major;
- }
- public void setClasses(String classes) {
- this.classes = classes;
- }
- @Override
- public boolean equals(Object o) {
- if (this == o) return true;
- if (o == null || getClass() != o.getClass()) return false;
- StudentInfo that = (StudentInfo) o;
- if (num != that.num) return false;
- if (age != that.age) return false;
- if (!name.equals(that.name)) return false;
- if (!gender.equals(that.gender)) return false;
- if (!major.equals(that.major)) return false;
- return classes.equals(that.classes);
- }
- @Override
- public String toString() {
- return "StudentInfo{" +
- "num=" + num +
- ", name='" + name + '\'' +
- ", gender='" + gender + '\'' +
- ", age=" + age +
- ", major='" + major + '\'' +
- ", classes='" + classes + '\'' +
- '}';
- }
- }
复制代码- //创建课程信息表
- package bean;
- public class CourseInfo {
- private int num;
- private String name;
- private String course;
- private String credit;
- public CourseInfo() {
- }
- public CourseInfo(int num, String name, String course, String credit) {
- this.num = num;
- this.name = name;
- this.course = course;
- this.credit = credit;
- }
- public int getNum() {
- return num;
- }
- public void setNum(int num) {
- this.num = num;
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- public String getCourse() {
- return course;
- }
- public void setCourse(String course) {
- this.course = course;
- }
- public String getCredit() {
- return credit;
- }
- public void setCredit(String credit) {
- this.credit = credit;
- }
- @Override
- public boolean equals(Object o) {
- if (this == o) return true;
- if (o == null || getClass() != o.getClass()) return false;
- CourseInfo that = (CourseInfo) o;
- if (num != that.num) return false;
- if (!name.equals(that.name)) return false;
- if (!course.equals(that.course)) return false;
- return credit.equals(that.credit);
- }
- @Override
- public String toString() {
- return "CourseInfo{" +
- "num=" + num +
- ", name='" + name + '\'' +
- ", course='" + course + '\'' +
- ", credit='" + credit + '\'' +
- '}';
- }
- }
复制代码- //创建用户信息表
- package bean;
- public class Users {
- private String user;
- private String password;
- private String privilege;
- public Users() {
- }
- public Users(String user, String password, String privilege) {
- this.user = user;
- this.password = password;
- this.privilege = privilege;
- }
- public String getUser() {
- return user;
- }
- public void setUser(String user) {
- this.user = user;
- }
- public String getPassword() {
- return password;
- }
- public void setPassword(String password) {
- this.password = password;
- }
- public String getPrivilege() {
- return privilege;
- }
- public void setPrivilege(String privilege) {
- this.privilege = privilege;
- }
- @Override
- public boolean equals(Object o) {
- if (this == o) return true;
- if (o == null || getClass() != o.getClass()) return false;
- Users users = (Users) o;
- if (!user.equals(users.user)) return false;
- if (!password.equals(users.password)) return false;
- return privilege.equals(users.privilege);
- }
- @Override
- public String toString() {
- return "Users{" +
- "user='" + user + '\'' +
- ", password='" + password + '\'' +
- ", privilege='" + privilege + '\'' +
- '}';
- }
- }
复制代码 2.创建基础工具类
- package JDBCUtil;
- import bean.CourseInfo;
- import bean.StudentInfo;
- import java.lang.reflect.Field;
- import java.sql.*;
- //工具类。其中包含:连接数据库、关闭资源、对数据库增删该查操作的一些通用基础的操作方法,没有限制条件。可由传入的sql语句包装该工具类,实现特定的功能。
- public class JDBCUtil {
- //封装获取连接的方法
- public static Connection getConnection() throws Exception {
- Class.forName("com.mysql.jdbc.Driver");
- String url = "jdbc:mysql://localhost:3306/db01";
- String user = "root";
- String password = "root";
- Connection connection = DriverManager.getConnection(url, user, password);
- return connection;
- }
- //封装资源关闭的方法
- public static void closeResource(Connection connection, PreparedStatement ps){
- if (connection != null){
- try {
- connection.close();
- } catch (SQLException e) {
- throw new RuntimeException(e);
- }
- }
- if (ps != null){
- try {
- ps.close();
- } catch (SQLException e) {
- throw new RuntimeException(e);
- }
- }
- }
- //封装资源关闭的方法(重载)
- public static void closeResource(Connection connection, PreparedStatement ps, ResultSet rs){
- if (connection != null){
- try {
- connection.close();
- } catch (SQLException e) {
- throw new RuntimeException(e);
- }
- }
- if (ps != null){
- try {
- ps.close();
- } catch (SQLException e) {
- throw new RuntimeException(e);
- }
- }
- if (rs != null){
- try {
- rs.close();
- } catch (SQLException e) {
- throw new RuntimeException(e);
- }
- }
- }
- //封装查询studentinfo
- public static StudentInfo queryForStudentinfo(String sql, Object...args) throws Exception {
- Connection connection = getConnection();
- PreparedStatement ps = connection.prepareStatement(sql);
- for (int i = 0;i < args.length;i++){
- ps.setObject(i+1,args[i]);
- }
- ResultSet rs = ps.executeQuery();
- ResultSetMetaData md = rs.getMetaData();
- int columnCount = md.getColumnCount();
- if (rs.next()){
- StudentInfo studentInfo = new StudentInfo();
- for (int i = 0;i < columnCount;i++){
- Object columnValue = rs.getObject(i + 1);
- String columnName = md.getColumnLabel(i + 1);
- Field field = StudentInfo.class.getDeclaredField(columnName);
- field.setAccessible(true);
- field.set(studentInfo,columnValue);
- }
- closeResource(connection,ps,rs);
- return studentInfo;
- }
- closeResource(connection,ps, rs);
- return null;
- }
- //封装查询courseinfo
- public static CourseInfo queryForCourseInfo(String sql, Object...args) throws Exception {
- Connection connection = getConnection();
- PreparedStatement ps = connection.prepareStatement(sql);
- for (int i = 0;i < args.length;i++){
- ps.setObject(i+1,args[i]);
- }
- ResultSet rs = ps.executeQuery();
- ResultSetMetaData md = rs.getMetaData();
- int columnCount = md.getColumnCount();
- if (rs.next()){
- CourseInfo courseInfo = new CourseInfo();
- for (int i = 0;i < columnCount;i++){
- Object columnValue = rs.getObject(i + 1);
- String columnName = md.getColumnLabel(i + 1);
- Field field = CourseInfo.class.getDeclaredField(columnName);
- field.setAccessible(true);
- field.set(courseInfo,columnValue);
- }
- closeResource(connection,ps,rs);
- return courseInfo;
- }
- closeResource(connection,ps, rs);
- return null;
- }
- //封装增删改操作的方法
- public static void update(String sql,Object...args) throws Exception {
- Connection connection = getConnection();
- PreparedStatement ps = connection.prepareStatement(sql);
- if (args != null) {
- for (int i = 0; i < args.length; i++) {
- ps.setObject(i + 1, args[i]);
- }
- }
- ps.execute();
- JDBCUtil.closeResource(connection,ps);
- }
- }
复制代码 该工具类可直接通过类名.方法调用,不需要创建对象,减少空间,使调用更简便。关于它的使用,好比:获取数据库的连接可通过:JDBCUtil.getConnection(),从而获取连接。
详情请看jdbc康师傅第14个视频链接:https://www.bilibili.com/video/BV1eJ411c7rf?p=14&vd_source=4cbd87668bb6192f3c7017d0ef336e63
详情请看jdbc康师傅第14个视频
3.创立功能类
- package Function;
- import JDBCUtil.JDBCUtil;
- import bean.CourseInfo;
- import bean.StudentInfo;
- import bean.Users;
- import java.sql.Connection;
- import java.sql.PreparedStatement;
- import java.sql.ResultSet;
- /*
- 该功能类作用:以封装好相关的sql语句(起到限制作用),只允许通过学号进行增删改查操作。通过该功能类对工具类进行一定的包装,可通过以下的方法具体实现某些特定的功能
- */
- public class Function {
- //通过学号实现查询功能
- public static StudentInfo queryForStudenInfoFunction(int num) throws Exception {
- //根据num搜索查询studentInfo功能的测试
- String sql = "select * from studentinfo where num=?";
- StudentInfo studentInfo = JDBCUtil.queryForStudentinfo(sql, num);
- return studentInfo;
- }
- public static CourseInfo queryForCourseInfoFunction(int num) throws Exception {
- //根据学号num,搜索查询courseInfo功能的测试
- String sql = "select * from courseinfo where num=?";
- CourseInfo courseInfo = JDBCUtil.queryForCourseInfo(sql, num);;
- return courseInfo;
- }
- //根据学号num,增删改
- //对studentInfo实现增加的功能
- public static Boolean createForStudentInfoFunction(int num,String name,String gender,int age,String major,String classes) throws Exception {
- String sql = "insert into studentinfo value(?,?,?,?,?,?)";
- JDBCUtil.update(sql,num,name,gender,age,major,classes);
- return true;
- }
- //对studentInfo实现删除的功能
- public static Boolean deleteForStudentInfoFunction(int num) throws Exception {
- String sql = "delete from studentinfo where num=?";
- JDBCUtil.update(sql,num);
- return true;
- }
- //对studentInfo实现改的功能 --可利用重载进行单个的修改
- public static void updateForStudentInfoFunction(String major,String classes,int num) throws Exception {
- String sql = "update studentinfo set major=?,classes=? where num=?"; //只能该专业和班级
- JDBCUtil.update(sql,major,classes,num);
- }
- public static Boolean updateForStudentInfoFunctionOfMajor(String major,int num) throws Exception {
- String sql = "update studentinfo set major=? where num=?"; //只能该专业和班级
- JDBCUtil.update(sql,major,num);
- return true;
- }
- public static boolean updateForStudentInfoFunctionOfClasses(String classes,int num) throws Exception {
- String sql = "update studentinfo set classes=? where num=?"; //只能该专业和班级
- JDBCUtil.update(sql,classes,num);
- return true;
- }
- //对courseinfo实现增加的功能
- public static boolean createForCourseInfoFunction(int num,String name,String course,String credit) throws Exception {
- String sql = "insert into courseinfo value(?,?,?,?)";
- JDBCUtil.update(sql,num,name,course,credit);
- return true;
- }
- //对courseinfo实现删除的功能
- public static boolean deleteForCourseInfoFunction(int num) throws Exception {
- String sql = "update courseinfo set course='',credit='' where num=?";
- JDBCUtil.update(sql,num);
- return true;
- }
- //对courseinfo实现修改的功能
- public static void updateForCourseInfoFunction(String course,String credit,int num) throws Exception {
- String sql = "update courseinfo set course=?,credit=? where num=?"; //改课程和学分
- JDBCUtil.update(sql,course,credit,num);
- }
- public static boolean updateForCourseInfoFunctionOfCourse(String course,int num) throws Exception {
- String sql = "update courseinfo set course=? where num=?"; //改课程和学分
- JDBCUtil.update(sql,course,num);
- return true;
- }
- public static boolean updateForCourseInfoFunctionOfCredit(String credit,int num) throws Exception {
- String sql = "update courseinfo set credit=? where num=?"; //改课程和学分
- JDBCUtil.update(sql,credit,num);
- return true;
- }
- //验证是否已经存在该学生---验证学号即可 --true 表示已经存在该学号的学生
- public static boolean queryIsExitForStuFunction(int num) throws Exception {
- Connection connection = JDBCUtil.getConnection();
- String sql = "select * from studentinfo";
- PreparedStatement ps = connection.prepareStatement(sql);
- ResultSet rs = ps.executeQuery();
- while (rs.next()){
- int numValue = rs.getInt(1);
- if (num == numValue){
- JDBCUtil.closeResource(connection,ps,rs);
- return true;
- }
- }
- JDBCUtil.closeResource(connection,ps,rs);
- return false;
- }
- //实现登入的功能,将从界面获得的账号和密码与数据库中的数据进行验证
- public static Boolean registerFunction(String user,String password) throws Exception {
- //测试登入功能
- String sql = "select * from users";
- Connection connection = JDBCUtil.getConnection();
- PreparedStatement ps = connection.prepareStatement(sql);
- ResultSet rs = ps.executeQuery();
- while (rs.next()){
- String userValue = rs.getString(1);
- String passwordValue = rs.getString(2);
- if (user.equals(userValue) && password.equals(passwordValue)){
- JDBCUtil.closeResource(connection,ps,rs);
- return true;
- }
- }
- JDBCUtil.closeResource(connection,ps,rs);
- return false;
- }
- //查询用户信息
- public static Users queryForUserFunction(String user,String password) {
- //从前端得到用户名和密码,传入后端
- Connection connection = null;
- PreparedStatement ps = null;
- ResultSet rs = null;
- try {
- String sql = "select * from users";
- connection = JDBCUtil.getConnection();
- ps = connection.prepareStatement(sql);
- rs = ps.executeQuery();
- Users users = new Users();
- while (rs.next()){
- String userValue = rs.getString(1);
- String passwordValue = rs.getString(2);
- String privilegeValue = rs.getString(3);
- if (user.equals(userValue) && password.equals(passwordValue)){
- users.setUser(userValue);
- users.setPassword(passwordValue);
- users.setPrivilege(privilegeValue);
- JDBCUtil.closeResource(connection,ps,rs);
- return users;
- }
- }
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- JDBCUtil.closeResource(connection,ps,rs);
- return null;
- }
- //增加用户信息
- public static Boolean createForUsersFunction(String user,String password,String privilege) {
- String sql = "insert into users value(?,?,?)";
- try {
- JDBCUtil.update(sql,user,password,privilege);
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- return true;
- }
- //删除用户信息
- public static Boolean deleteForUsersFunction(String user) {
- String sql = "delete from users where user=?";
- try {
- JDBCUtil.update(sql, user);
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- return true;
- }
- //修改用户密码
- public static Boolean updateForUsersFunction(String user,String prePassword,String curPassword) {
- Boolean flag = null;
- try {
- flag = registerFunction(user, prePassword);
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- if (flag){
- String sql = "update users set password=? where user=?";
- try {
- JDBCUtil.update(sql,curPassword,user);
- } catch (Exception e) {
- throw new RuntimeException(e);
- }
- return true;
- }
- return false;
- }
- }
复制代码 该功能类将传入的参数添补到实现该功能的sql语句中,在调用基础的工具类,进而实现特定的功能。(主要依赖于在该类中所写的sql语句,通过特定的sql语句对工具类进行特定的封装,从而实现特定的功能)这些功能类的实现,有利于后面的界面类调用该功能类并将读取的数据作为实参传入,最终即可获取所想要的数据结果。
4.单元测试
- package JunitDemo;
- import Function.Function;
- import bean.CourseInfo;
- import bean.StudentInfo;
- import org.junit.Test;
-
- //分别对每一个后端板块进行单元测试,逐一测试各个板块是否正确,测试时各个板块之间无关联。
- public class JunitTestDemo {
- @Test
- public void testRegister() throws Exception {
- //从前端界面获取账号和密码,实现登入功能
- String user = "520";
- String password = "1314";
- Boolean flag = Function.registerFunction(user, password);//true表示登入成功,前端进入新的界面
- System.out.println(flag);
- }
- @Test
- public void testQuery() throws Exception {
- //从前端界面获取学号,实现对studentinfo和courseinfo的查询功能
- int num = 1;
- StudentInfo studentInfo = Function.queryForStudenInfoFunction(num);
- CourseInfo courseInfo = Function.queryForCourseInfoFunction(num);//将这两个结果重新传入前端界面
- System.out.println(studentInfo);
- System.out.println(courseInfo);
- }
- @Test
- public void testCreateForStudentInfo() throws Exception {
- //从前端界面获得学号,实现对studentinfo增加的功能
- int num = 9;
- String name = "关羽";
- String gender = "男";
- int age = 35;
- String major = "太极";
- String classes = "五虎上将";
- Function.createForStudentInfoFunction(num,name,gender,age,major,classes);
- }
- @Test
- public void testDeleteForStudentInfo() throws Exception {
- //从前端界面获得学号,实现对studentinfo删除的功能
- int num = 0;
- Function.deleteForStudentInfoFunction(num);
- }
- @Test
- public void testUpdateForStudentInfo() throws Exception {
- //从前端界面获得学号,实现对studentinfo修改的功能----修改major和classes
- String major = "阴阳五行";
- String classes = "五子良将";
- int num = 9;
- Function.updateForStudentInfoFunction(major,classes,num);
- }
- @Test
- public void testCreateForCourseInfo() throws Exception {
- //从前端界面获得学号,实现对courseinfo增加的功能
- int num = 11;
- String name = "关羽";
- String course = "法学";
- String credit = "5";
- Function.createForCourseInfoFunction(num,name,course,credit);
- }
- @Test
- public void testDeleteForCourseInfo() throws Exception {
- //从前端界面获得学号,实现对courseinfo删除的功能
- int num = 11;
- Function.deleteForCourseInfoFunction(num);
- }
- @Test
- public void testUpdateForCourseInfo() throws Exception {
- //从前端界面获得学号,实现对courseinfo修改的功能---修改course和credit
- int num = 11;
- String course = "哲学";
- String credit = "4";
- Function.updateForCourseInfoFunction(course,credit,num);
- }
- @Test
- public void testCreateForUsers() throws Exception {
- //从前端获得账号和密码,实现增加用户的功能
- String user = "11";
- String password = "890";
- String privilege = "管理员";
- Function.createForUsersFunction(user,password,privilege);
- }
- @Test
- public void testDeleteForUsers() throws Exception {
- //从前端获得账号和密码,实现删除的功能
- String user = "11";
- Function.deleteForUsersFunction(user);
- }
- }
复制代码 单元测试方法:鼠标左键双击方法名,再鼠标右键点击运行即可运行所双击的方法,与其他的无关。
该单元测试方法有利于对每一个功能进行单个逐一地测试,确保每一个功能的实现,使测试省时省力。
注:测试时,对于需要从界面获取的数据,我们采取自动赋值的方式传入数据,主要观察功能是否有用精确。
5.创建界面类
1.界面管理类
- package MyFrame;
- import bean.Users;
- //界面管理类。负责包装所有的界面。只要调用该类中的方法,即可创建出相应的界面并且监听该界面。
- public class FramePackaging {
- public static void getHandleFrame(Users users){
- HandleFrame handleFrame = new HandleFrame(users);
- handleFrame.init();
- handleFrame.jbtListener();
- }
- public static void getCSFrame(Users users){
- CreateForStuFrame createForStuFrame = new CreateForStuFrame(users);
- createForStuFrame.init();
- createForStuFrame.jbtListener();
- }
- public static void getCCFrame(Users users){
- CreateForCouFrame createForCouFrame = new CreateForCouFrame(users);
- createForCouFrame.init();
- createForCouFrame.jbtListener();
- }
- public static void getDSFrame(Users users){
- DeleteForStuFrame deleteForStuFrame = new DeleteForStuFrame(users);
- deleteForStuFrame.init();
- deleteForStuFrame.jbtListener();
- }
- public static void getDCFrame(Users users){
- DeleteForCouFrame deleteForCouFrame = new DeleteForCouFrame(users);
- deleteForCouFrame.init();
- deleteForCouFrame.jbtListener();
- }
- public static void getQSFrame(Users users){
- QueryForStuFrame queryForStuFrame = new QueryForStuFrame(users);
- queryForStuFrame.init();
- queryForStuFrame.jbtLister();
- }
- public static void getQCFrame(Users users){
- QueryForCouFrame queryForCouFrame = new QueryForCouFrame(users);
- queryForCouFrame.init();
- queryForCouFrame.jbtListener();
- }
- public static void getUSFrame(Users users){
- UpdateForStuFrame updateForStuFrame = new UpdateForStuFrame(users);
- updateForStuFrame.init();
- updateForStuFrame.jbtListener();
- }
- public static void getUCFrame(Users users){
- UpdateForCouFrame updateForCouFrame = new UpdateForCouFrame(users);
- updateForCouFrame.init();
- updateForCouFrame.jbtListener();
- }
- }
复制代码 2.登录界面
- package MyFrame;
- import Function.Function;
- import bean.Users;
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- public class RegisterFrame extends JFrame {
- Users users = new Users();
- //创建组件
- JFrame jFrame = new JFrame("登录系统");
- JPanel jpUser = new JPanel();
- JLabel jlbUser = new JLabel("账号");
- JTextField jtfUser = new JTextField(33);
- JPanel jpPassword = new JPanel();
- JLabel jlbPassWord = new JLabel("密码");
- JTextField jtfPassWord = new JTextField(33);
- JButton jbt = new JButton("登录");
- public void init() {
- jpUser.add(jlbUser);
- jpUser.add(jtfUser);
-
- jpPassword.add(jlbPassWord);
- jpPassword.add(jtfPassWord);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpUser);
- vbox.add(jpPassword);
- jFrame.add(vbox);
- jFrame.add(jbt, BorderLayout.SOUTH);
- jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- jFrame.setSize(600, 400);
- jFrame.setVisible(true);
- jFrame.setLocationRelativeTo(null);
- }
- public void buttonListenerForRegister() {
- jbt.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- String user = jtfUser.getText();
- String password = jtfPassWord.getText();
- Boolean flag = false;
- try {
- //将账号和密码与数据库进行匹配
- flag = Function.registerFunction(user, password);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- //若账号密码匹配正确:
- if (flag) {
- //返回当前用户信息(账号、密码和权限)
- users = Function.queryForUserFunction(user, password);
- //切换新的页面--操作页面---并将该用户信息传入下一个界面
- JOptionPane.showMessageDialog(jFrame, "登录成功" + "\n"+ "你好!"+ users.getPrivilege() + "!", "消息对话框", JOptionPane.INFORMATION_MESSAGE);、
- //转入下一个界面
- FramePackaging.getHandleFrame(users);
- //转入到下一个界面后,关闭该界面
- jFrame.setVisible(false);
- }else{
- JOptionPane.showMessageDialog(jFrame, "账号或密码错误", "消息对话框", JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- }
- }
复制代码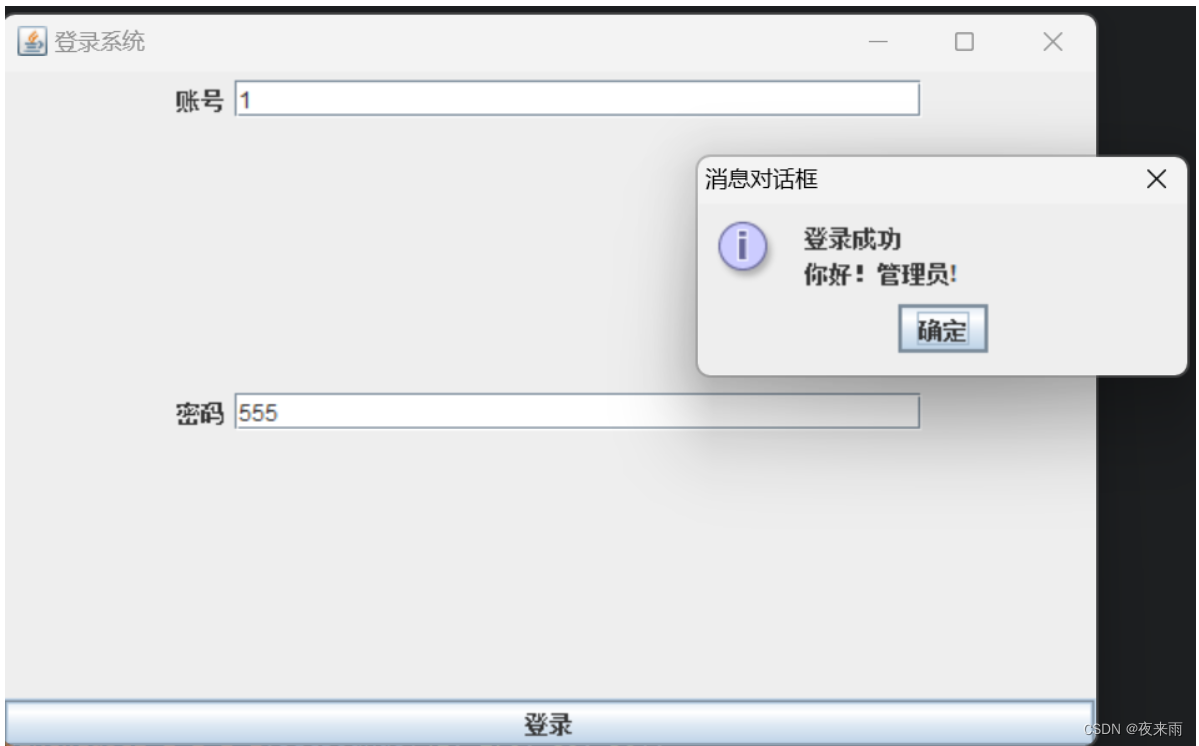
界面类的思路大要同等,这里以登录界面为例进行着重讲解:
属性:一种是用户类属性(users),该users属性中储存这登录时使用的账号密码权限等。另一种是界面组件属性,由这些组件构成界面。
方法:init():即初始化方法,该方法主要的作用就是将组件组装成一个界面。
buttonListenerForRegister();即监听方法,该方法主要是监听各个按钮,实现该界面的功能。该方法也是整个界面运转的核心,很多逻辑思路都在这里。该方法涉及到:获取文本框数据,与数据库交互,弹出消息对话框,将获取数据写入文本框,连接下一个界面这五大方面。要实现这些主要通过调用刚才所写的功能类中的方法,一一对应即可。
界面的思路讲解:通过登录界面获取的账号和密码调用registerFunction()方法与数据库进行匹配,若匹配精确则返回一个users对象,并将该对象包罗了账号、密码和权限。接着,通过下一个界面的构造器,将uses对象传给下一个界面的uses对象。
3.菜单界面
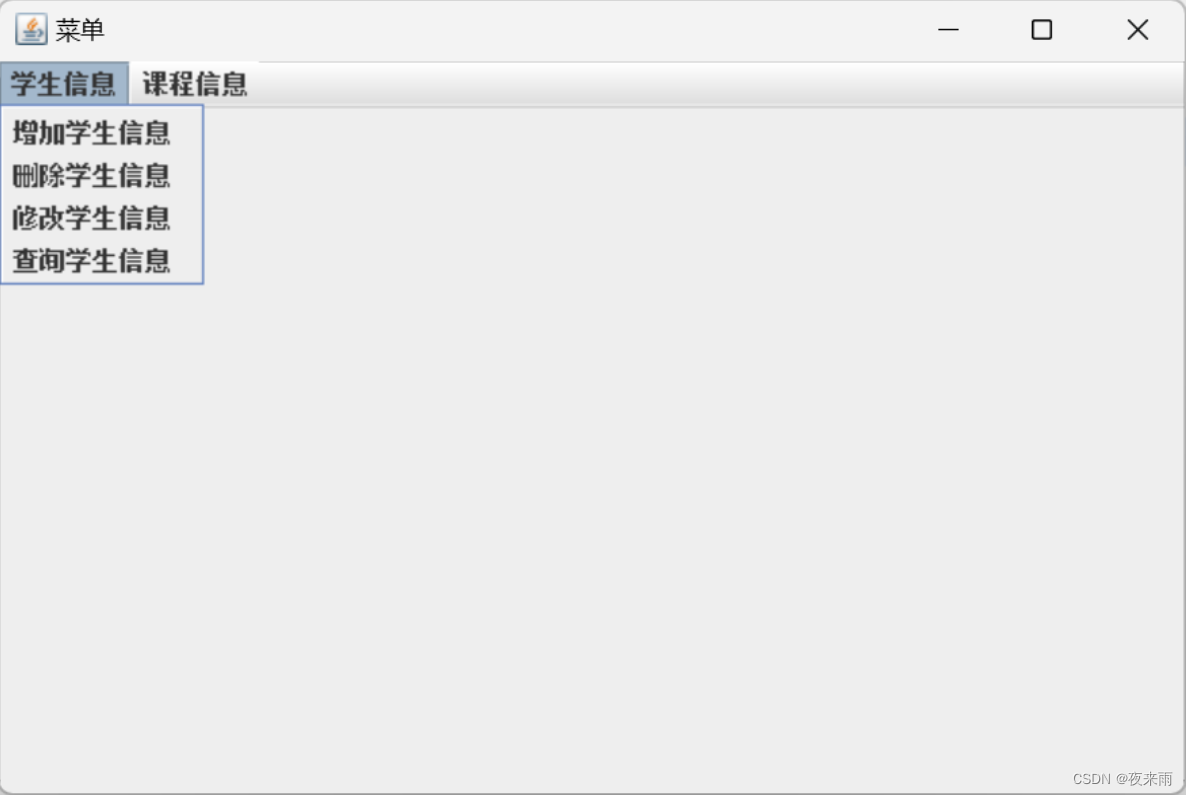
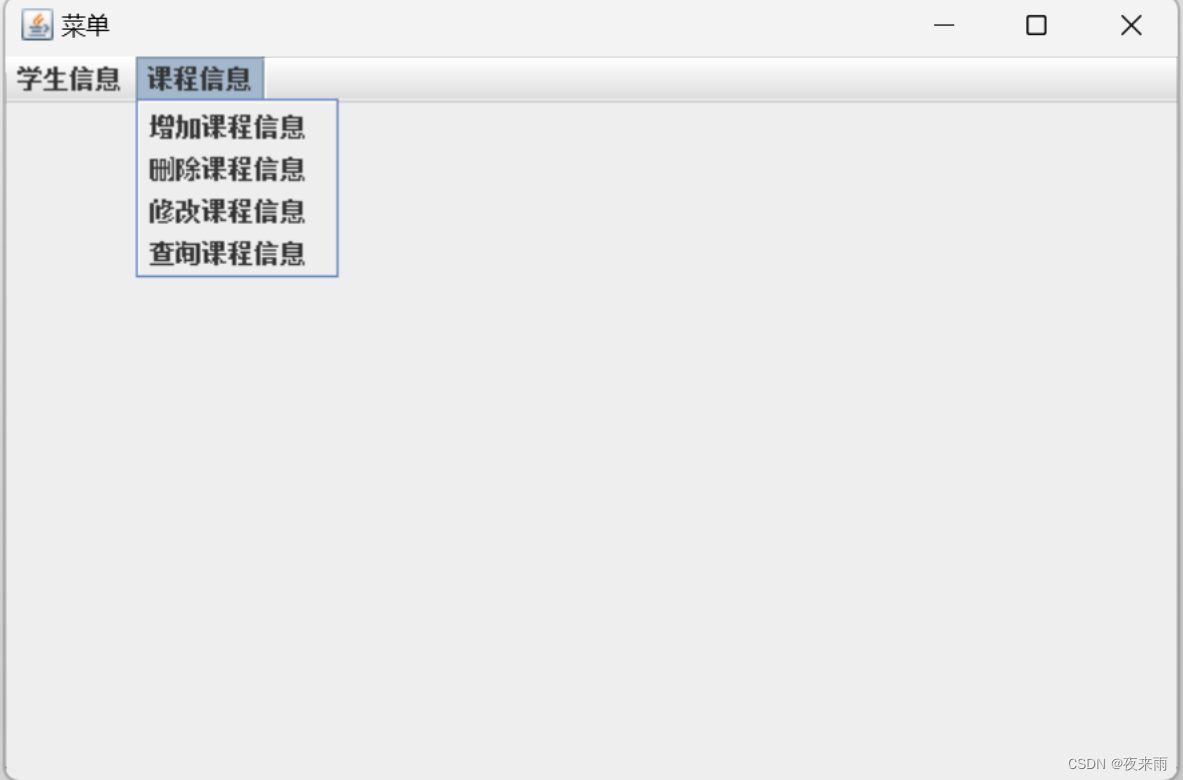
菜单界面通过构造器获得了登录时的用户信息,该界面最大的区别就是在监听方法中,监听每一个菜单项,并且当菜单项被点击后将登录时的users对象传入并弹出对应功能的界面。
4.增长门生信息界面
- package MyFrame;
- import Function.Function;
- import bean.StudentInfo;
- import bean.Users;
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.WindowAdapter;
- import java.awt.event.WindowEvent;
- //添加学生信息界面
- public class CreateForStuFrame {
- JFrame createForStuFrame = new JFrame("增加学生信息");
- JButton jbt = new JButton("确认添加");
- JLabel jlbNum = new JLabel("学号:");
- JLabel jlbName = new JLabel("姓名:");
- JLabel jlbGender = new JLabel("性别:");
- JLabel jlbAge = new JLabel("年龄:");
- JLabel jlbMajor = new JLabel("专业:");
- JLabel jlbClass = new JLabel("班级:");
- JTextField jtfNum = new JTextField(11);
- JTextField jtfName = new JTextField(11);
- JTextField jtfGender = new JTextField(11);
- JTextField jtfAge = new JTextField(11);
- JTextField jtfMajor = new JTextField(11);
- JTextField jtfClass = new JTextField(11);
- JPanel jpNum = new JPanel();
- JPanel jpName = new JPanel();
- JPanel jpGender = new JPanel();
- JPanel jpAge = new JPanel();
- JPanel jpMajor = new JPanel();
- JPanel jpClass = new JPanel();
- Users users = null;
- public CreateForStuFrame(Users users){
- this.users = users;
- }
- //组装界面
- public void init(){
- jpNum.add(jlbNum);
- jpNum.add(jtfNum);
- jpName.add(jlbName);
- jpName.add(jtfName);
- jpGender.add(jlbGender);
- jpGender.add(jtfGender);
- jpAge.add(jlbAge);
- jpAge.add(jtfAge);
- jpMajor.add(jlbMajor);
- jpMajor.add(jtfMajor);
- jpClass.add(jlbClass);
- jpClass.add(jtfClass);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpNum);
- vbox.add(jpName);
- vbox.add(jpAge);
- vbox.add(jpGender);
- vbox.add(jpMajor);
- vbox.add(jpClass);
- createForStuFrame.add(vbox);
- createForStuFrame.add(jbt,BorderLayout.SOUTH);
- createForStuFrame.setVisible(true);
- createForStuFrame.setSize(600, 400);
- createForStuFrame.setLocationRelativeTo(null);
- }
- //对按钮进行监听----在主线程中调用了该方法,该按钮被点击时自动调用该方法内的设置好的监听器
- public void jbtListener(){
- jbt.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege())){
- String user = null;
- String privilege = null;
- if (Math.random() > 0.5) {
- privilege = "管理员";
- }else {
- privilege = "普通用户";
- }
- boolean flag = false;
- try {
- String textNum = jtfNum.getText();
- int num = Integer.parseInt(textNum);
- String name = jtfName.getText();
- String gender = jtfGender.getText();
- String textAge = jtfAge.getText();
- int age = Integer.parseInt(textAge);
- String major = jtfMajor.getText();
- String classes = jtfClass.getText();
- user = textNum;
- //从界面获得信息,传入后端操作,进而导入数据库内
- //查询是否有该学生,有则添加失败
- StudentInfo info = Function.queryForStudenInfoFunction(num);
- if (info == null)
- flag = Function.createForStudentInfoFunction(num, name, gender, age, major, classes);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag){
- JOptionPane.showMessageDialog(createForStuFrame,"添加成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- //添加学生信息的同时,添加用户账号和密码
- Function.createForUsersFunction(user,"123",privilege);
- emptyTextField();
- }else {
- JOptionPane.showMessageDialog(createForStuFrame,"添加失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(createForStuFrame,"非管理员,添加失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- createForStuFrame.addWindowListener(new WindowAdapter() {
- @Override
- public void windowClosing(WindowEvent e) {
- //关闭该界面,系统未关闭
- createForStuFrame.setVisible(false);
- }
- });
- }
- //封装清空所有文本框
- public void emptyTextField(){
- jtfNum.setText("");
- jtfName.setText("");
- jtfGender.setText("");
- jtfAge.setText("");
- jtfMajor.setText("");
- jtfClass.setText("");
- }
- }
复制代码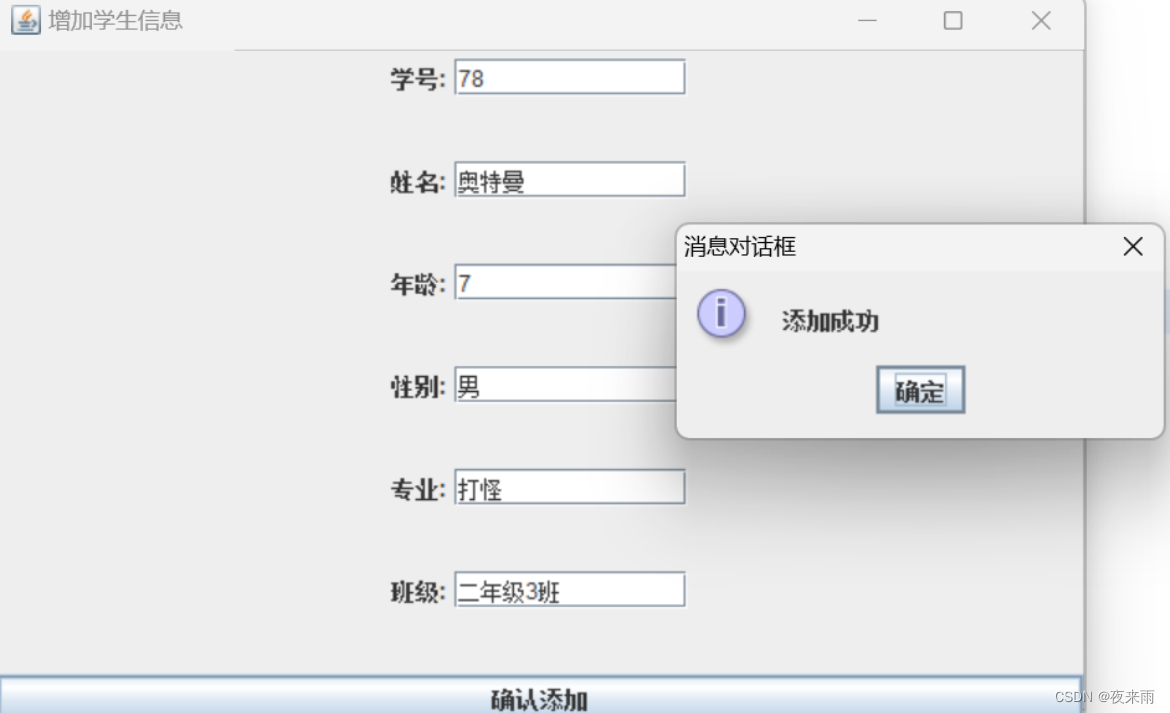
init()没有太大的变革,依然是组装界面。该界面主要讲解的是监听方法,即jbtListener()。
由于只能管理员能增长门生,并且我们通过构造器获得了users对象,所以我们可以先匹配一下该users对象的权限,假如是管理员则可以进行下面的操作。
5.增长课程信息界面
- package MyFrame;
- import Function.Function;
- import bean.CourseInfo;
- import bean.StudentInfo;
- import bean.Users;
- import javax.swing.*;
- import java.awt.BorderLayout;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.WindowAdapter;
- import java.awt.event.WindowEvent;
- //添加课程信息界面
- public class CreateForCouFrame {
- JFrame createForCouFrame = new JFrame("增加课程信息");
- JButton jbt = new JButton("确认添加");
- JPanel jpQuery = new JPanel();
- JLabel jlbQueryNum = new JLabel("输入添加的学号");
- JTextField jtfQueryNum = new JTextField(6);
- JButton jbtQuery = new JButton("查询");
-
- JPanel jpNUm = new JPanel();
- JLabel jlbNum = new JLabel("学号:");
- JTextField jtfNum = new JTextField(11);
- JPanel jpName = new JPanel();
- JLabel jlbName = new JLabel("姓名:");
- JTextField jtfName = new JTextField(11);
- JPanel jpCourse = new JPanel();
- JLabel jlbCourse = new JLabel("课程:");
- JTextField jtfCourse = new JTextField(11);
- JPanel jpCredit = new JPanel();
- JLabel jlbCredit = new JLabel("学分");
- JTextField jtfCredit = new JTextField(11);
- Users users = null;
- StudentInfo studentInfo = null;
- public CreateForCouFrame() {}
- public CreateForCouFrame(Users users){
- this.users = users;
- }
- public void init(){
- jpQuery.add(jlbQueryNum);
- jpQuery.add(jtfQueryNum);
- jpQuery.add(jbtQuery);
-
- jpNUm.add(jlbNum);
- jpNUm.add(jtfNum);
- jtfNum.setEditable(false);
-
- jpName.add(jlbName);
- jpName.add(jtfName);
- jtfName.setEditable(false);
-
- jpCourse.add(jlbCourse);
- jpCourse.add(jtfCourse);
-
- jpCredit.add(jlbCredit);
- jpCredit.add(jtfCredit);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpQuery);
- vbox.add(jpNUm);
- vbox.add(jpName);
- vbox.add(jpCourse);
- vbox.add(jpCredit);
-
- createForCouFrame.add(vbox);
- createForCouFrame.add(jbt,BorderLayout.SOUTH);
- createForCouFrame.setVisible(true);
- createForCouFrame.setSize(600, 400);
- createForCouFrame.setLocationRelativeTo(null);
- }
- //对按钮进行监听----在主线程中调用了该方法,该按钮被点击时自动调用该方法内的设置好的监听器
- public void jbtListener(){
- //查询是否有该学生
- jbtQuery.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- String text = jtfQueryNum.getText();
- int num = Integer.parseInt(text);
- try {
- studentInfo = Function.queryForStudenInfoFunction(num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- if (studentInfo != null){
- JOptionPane.showMessageDialog(createForCouFrame,"查询成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- int stu_num = studentInfo.getNum();
- String stu_name = studentInfo.getName();
- jtfNum.setText(""+stu_num);
- jtfName.setText(stu_name);
- jtfQueryNum.setText("");
- }else {
- JOptionPane.showMessageDialog(createForCouFrame,"查询失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
-
- jbt.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege())){
- boolean flag = false;
- try {
- String textNum = jtfNum.getText();
- int num = Integer.parseInt(textNum);
- String name = jtfName.getText();
- String course = jtfCourse.getText();
- String credit = jtfCredit.getText();
- //查询是否有该学生,有该学生则添加
- CourseInfo courseInfo = Function.queryForCourseInfoFunction(num);
- if (jtfNum != null && courseInfo.getCourse() == null)
- flag = Function.createForCourseInfoFunction(num, name, course,credit);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag){
- JOptionPane.showMessageDialog(createForCouFrame,"添加成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- emptyTextField();
- }else {
- JOptionPane.showMessageDialog(createForCouFrame,"添加失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else{
- JOptionPane.showMessageDialog(createForCouFrame,"非管理员,添加失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- createForCouFrame.addWindowListener(new WindowAdapter() {
- @Override
- public void windowClosing(WindowEvent e) {
- createForCouFrame.setVisible(false);
- }
- });
- }
- //封装清空所有文本框
- public void emptyTextField(){
- jtfNum.setText("");
- jtfName.setText("");
- jtfCourse.setText("");
- jtfCredit.setText("");
- }
- }
复制代码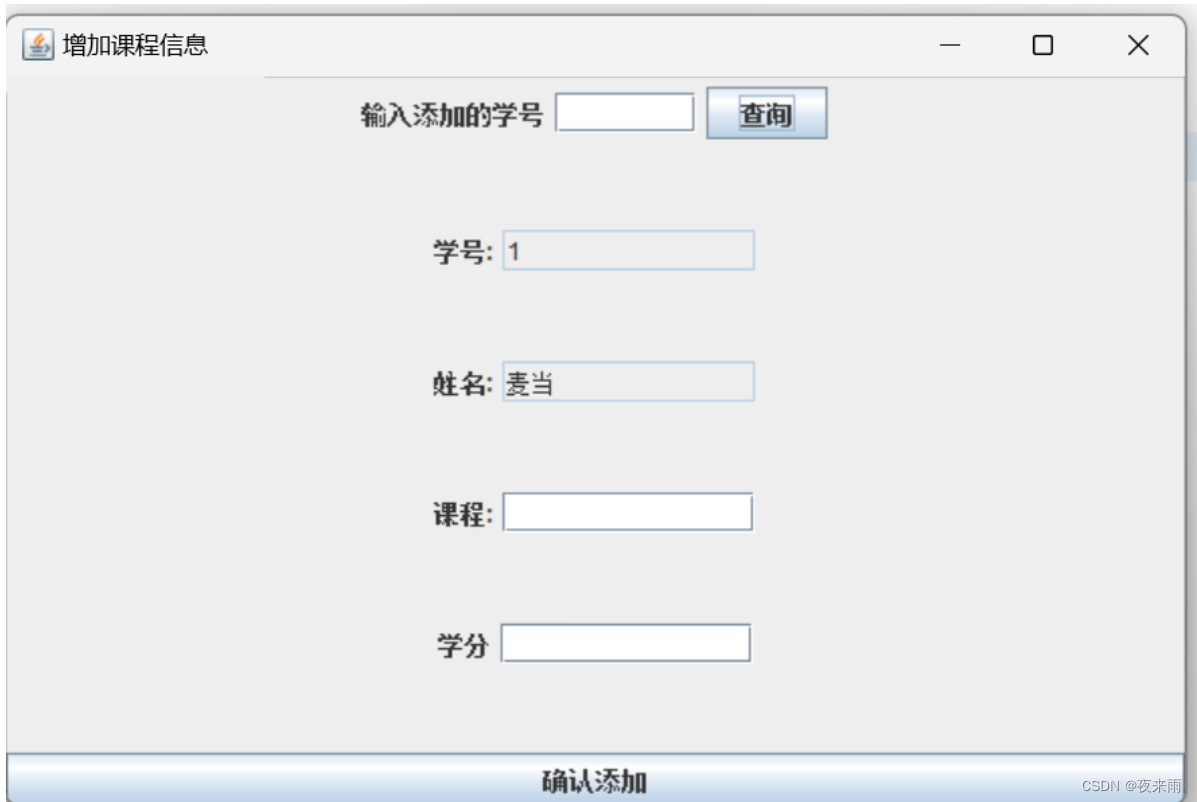
该界面的思路基本与上一个界面的思路同等。将得到的users对象中的权限进行匹配,管理员则可以进行添加。添加必须先有这个门生否则添加失败。
6.删除门生信息界面
- package MyFrame;
- import Function.Function;
- import bean.StudentInfo;
- import bean.Users;
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.WindowAdapter;
- import java.awt.event.WindowEvent;
- //学生信息界面
- public class DeleteForStuFrame {
- StudentInfo studentInfo = null;
- JFrame deleteForStuFrame = new JFrame("删除学生信息");
- JPanel jpQuery = new JPanel();
- JLabel jlbQueryNum = new JLabel("学号");
- JTextField jtfQueryNum = new JTextField(11);
- JButton jbtQuery = new JButton("查询");
- JPanel jpNum = new JPanel();
- JLabel jlbNum = new JLabel("学号");
- JTextField jtfNum = new JTextField(11);
- JPanel jpName = new JPanel();
- JLabel jlbName = new JLabel("姓名");
- JTextField jtfName = new JTextField(11);
- JPanel jpGender = new JPanel();
- JLabel jlbGender = new JLabel("性别");
- JTextField jtfGender = new JTextField(11);
- JPanel jpAge = new JPanel();
- JLabel jlbAge = new JLabel("年龄");
- JTextField jtfAge = new JTextField(11);
- JPanel jpMajor = new JPanel();
- JLabel jlbMajor = new JLabel("专业");
- JTextField jtfMajor = new JTextField(11);
- JPanel jpClass = new JPanel();
- JLabel jlbClass = new JLabel("班级");
- JTextField jtfClass = new JTextField(11);
- JButton jbtDelete = new JButton("确认删除");
- Users users = null;
- String textNum = null;
- public DeleteForStuFrame(Users users){
- this.users = users;
- }
- //组装组件
- public void init(){
- jpQuery.add(jlbQueryNum);
- jpQuery.add(jtfQueryNum);
- jpQuery.add(jbtQuery);
-
- jpNum.add(jlbNum);
- jpNum.add(jtfNum);
-
- jpName.add(jlbName);
- jpName.add(jtfName);
-
- jpGender.add(jlbGender);
- jpGender.add(jtfGender);
-
- jpAge.add(jlbAge);
- jpAge.add(jtfAge);
-
- jpMajor.add(jlbMajor);
- jpMajor.add(jtfMajor);
-
- jpClass.add(jlbClass);
- jpClass.add(jtfClass);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpQuery);
- vbox.add(jpNum);
- vbox.add(jpName);
- vbox.add(jpGender);
- vbox.add(jpAge);
- vbox.add(jpMajor);
- vbox.add(jpClass);
- vbox.add(jbtDelete);
- deleteForStuFrame.add(vbox);
- deleteForStuFrame.setVisible(true);
- deleteForStuFrame.setSize(600, 400);
- deleteForStuFrame.setLocationRelativeTo(null);
- }
- //监听按钮分别进行查询和删除
- public void jbtListener(){
- //监听查询按钮
- jbtQuery.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- try {
- textNum = jtfQueryNum.getText();
- int num = Integer.parseInt(textNum);
- studentInfo = Function.queryForStudenInfoFunction(num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (studentInfo != null){
- JOptionPane.showMessageDialog(deleteForStuFrame,"查询成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- //将获取的数据填充文本框
- setTextField();
- //查询成功的同时,清空刚才手动输入的文本框
- jtfQueryNum.setText("");
- }else {
- JOptionPane.showMessageDialog(deleteForStuFrame,"查询失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }
- });
- //监听删除按钮
- jbtDelete.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege()) || users.getUser().equals(textNum)){
- String user = null;
- Boolean flag = false;
- try {
- user = "" + studentInfo.getNum();
- flag = Function.deleteForStudentInfoFunction(studentInfo.getNum());
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag && studentInfo != null){
- JOptionPane.showMessageDialog(deleteForStuFrame,"删除成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- emptyTextField();
- //学生信息被删除的同时,该学生的课程信息和用户信息也被删除
- try {
- Function.deleteForCourseInfoFunction(studentInfo.getNum());
- Function.deleteForUsersFunction(user);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- }else{
- JOptionPane.showMessageDialog(deleteForStuFrame,"删除失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(deleteForStuFrame,"非管理员或非本人操作,删除失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- deleteForStuFrame.addWindowListener(new WindowAdapter() {
- @Override
- public void windowClosing(WindowEvent e) {
- deleteForStuFrame.setVisible(false);
- }
- });
- }
- //封装填充文本框
- public void setTextField(){
- int infoNum = studentInfo.getNum();
- String num1 = ""+infoNum;
- String infoName = studentInfo.getName();
- String infoGender = studentInfo.getGender();
- int infoAge = studentInfo.getAge();
- String age = "" + infoAge;
- String infoMajor = studentInfo.getMajor();
- String infoClasses = studentInfo.getClasses();
- jtfNum.setText(num1);
- jtfName.setText(infoName);
- jtfGender.setText(infoGender);
- jtfAge.setText(age);
- jtfMajor.setText(infoMajor);
- jtfClass.setText(infoClasses);
- }
- //封装清空所有文本框
- public void emptyTextField(){
- jtfNum.setText("");
- jtfName.setText("");
- jtfGender.setText("");
- jtfAge.setText("");
- jtfMajor.setText("");
- jtfClass.setText("");
- }
- }
复制代码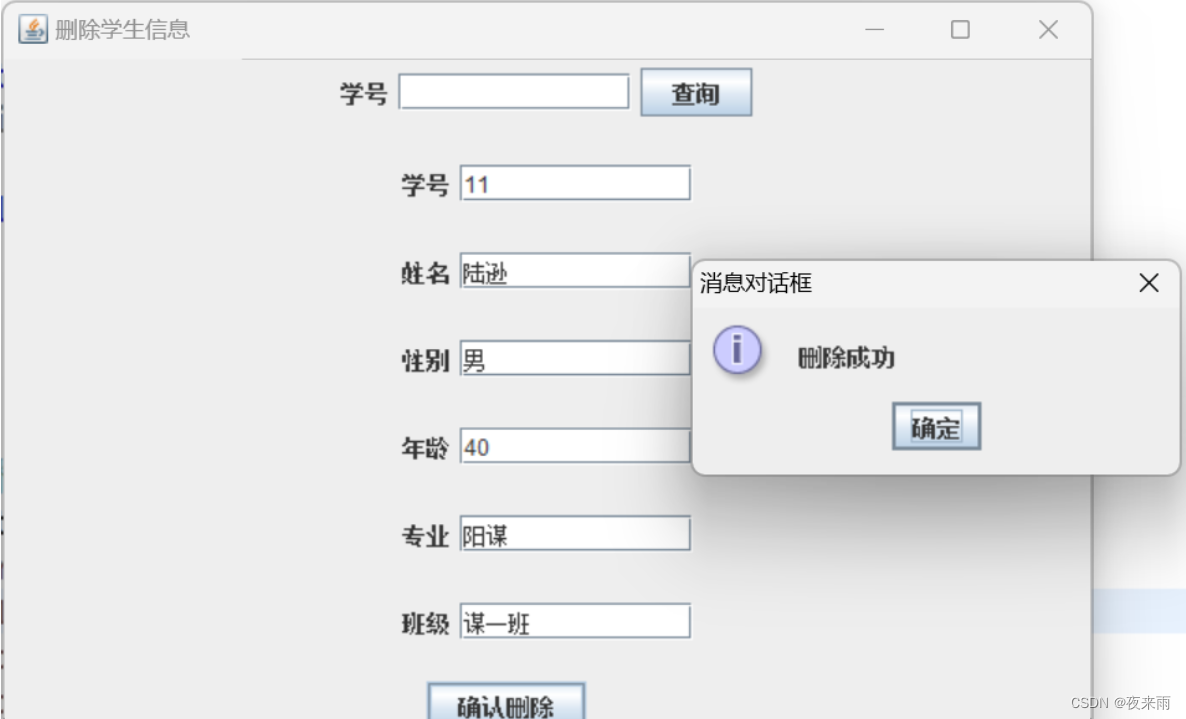
7.删除课程信息界面
- package MyFrame;
- import Function.Function;
- import bean.CourseInfo;
- import bean.Users;
- import javax.swing.*;
- import java.awt.Dimension;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.WindowAdapter;
- import java.awt.event.WindowEvent;
-
- //删除课程信息界面
- public class DeleteForCouFrame {
- CourseInfo courseInfo = null;
- JFrame deleteForCouFrame = new JFrame("删除课程");
- JLabel jlbQueryNum = new JLabel("学号");
- JTextField jtfdeleNum = new JTextField(11);
- JButton jbtQuery = new JButton("查询");
- JPanel jpQuery = new JPanel();
- JPanel jpNum = new JPanel();
- JPanel jpName = new JPanel();
- JPanel jpCourse = new JPanel();
- JPanel jpCredit = new JPanel();
- JLabel jlbNum = new JLabel("学号:");
- JLabel jlbName = new JLabel("姓名:");
- JLabel jlbCourse = new JLabel("课程:");
- JLabel jlbCredit = new JLabel("学分");
- JTextField jtfNum = new JTextField(11);
- JTextField jtfName = new JTextField(11);
- JTextField jtfCourse = new JTextField(11);
- JTextField jtfCredit = new JTextField(11);
- JButton jbtDelete = new JButton("确认删除");
- Users users = null;
- String textNum = null;
- public DeleteForCouFrame(Users users){
- this.users = users;
- }
- public void init(){
-
- jpQuery.add(jlbQueryNum);
- jpQuery.add(jtfdeleNum);
- jpQuery.add(jbtQuery);
-
- jpNum.add(jlbNum);
- jpNum.add(jtfNum);
-
- jpName.add(jlbName);
- jpName.add(jtfName);
-
- jpCourse.add(jlbCourse);
- jpCourse.add(jtfCourse);
-
- jpCredit.add(jlbCredit);
- jpCredit.add(jtfCredit);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpQuery);
- vbox.add(jpNum);
- vbox.add(jpName);
- vbox.add(jpCourse);
- vbox.add(jpCredit);
- vbox.add(jbtDelete);
- deleteForCouFrame.add(vbox);
- deleteForCouFrame.setVisible(true);
- deleteForCouFrame.setSize(600, 400);
- deleteForCouFrame.setLocationRelativeTo(null);
- }
- //对按钮进行监听
- public void jbtListener(){
- jbtQuery.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- try {
- textNum = jtfdeleNum.getText();
- int num = Integer.parseInt(textNum);
- courseInfo = Function.queryForCourseInfoFunction(num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }finally {
- if (courseInfo != null){
- JOptionPane.showMessageDialog(deleteForCouFrame,"查询成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- jtfdeleNum.setText("");
- setTextField();
- }else{
- JOptionPane.showMessageDialog(deleteForCouFrame,"查询失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }
- });
- jbtDelete.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege()) || users.getUser().equals(textNum)){
- boolean flag = false;
- try {
- flag = Function.deleteForCourseInfoFunction(courseInfo.getNum());
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag && courseInfo != null){
- JOptionPane.showMessageDialog(deleteForCouFrame,"删除成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- emptyTextField();
- }else{
- JOptionPane.showMessageDialog(deleteForCouFrame,"删除失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(deleteForCouFrame,"非管理员或非本人操作,删除失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- deleteForCouFrame.addWindowListener(new WindowAdapter() {
- @Override
- public void windowClosing(WindowEvent e) {
- deleteForCouFrame.setVisible(false);
- }
- });
- }
- //封装清空所有文本框
- public void emptyTextField(){
- jtfNum.setText("");
- jtfName.setText("");
- jtfCourse.setText("");
- jtfCredit.setText("");
- }
- //封装输入文本框
- public void setTextField(){
- int num1 = courseInfo.getNum();
- String num2= "" + num1;
- String name = courseInfo.getName();
- String course = courseInfo.getCourse();
- String credit = courseInfo.getCredit();
- jtfNum.setText(num2);
- jtfName.setText(name);
- jtfCourse.setText(course);
- jtfCredit.setText(credit);
- }
- }
复制代码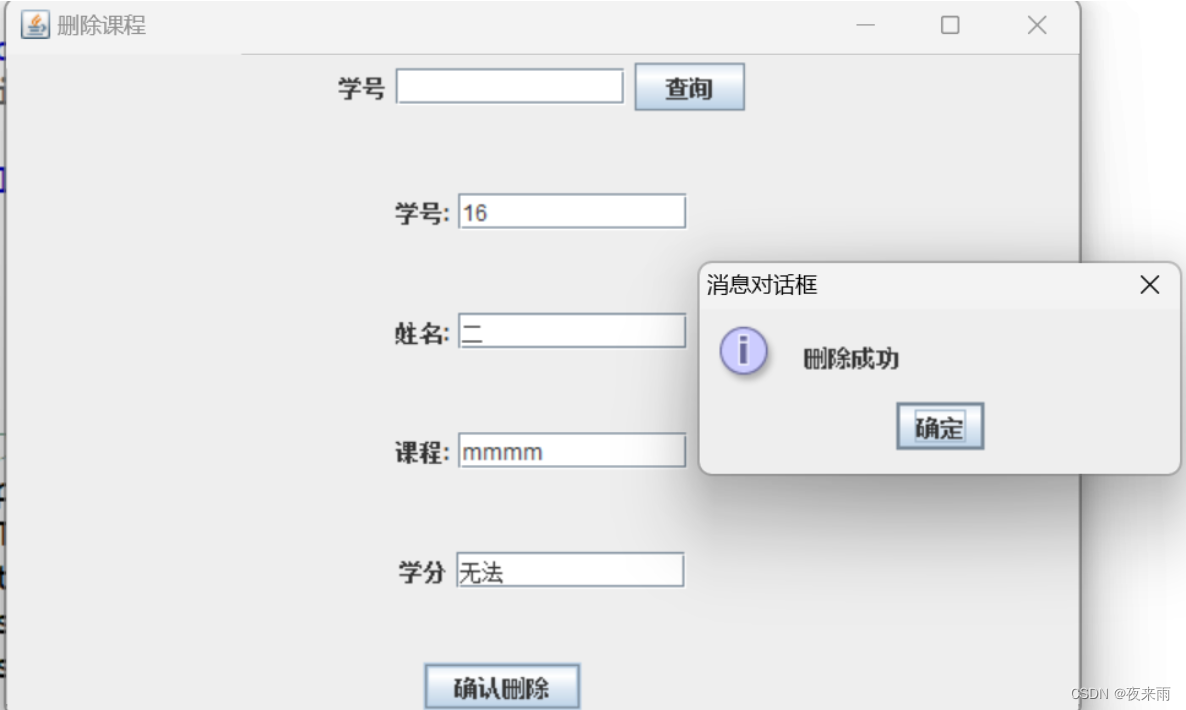
8.修改门生信息界面
- package MyFrame;
- import Function.Function;
- import bean.StudentInfo;
- import bean.Users;
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.WindowAdapter;
- import java.awt.event.WindowEvent;
- //修改学生信息界面
- public class UpdateForStuFrame {
- StudentInfo studentInfo = null;
- JFrame updateForStuFrame = new JFrame("修改学生信息");
- JPanel jpQuery = new JPanel();
- JLabel jlbQueryNum = new JLabel("学号");
- JTextField jtfQueryNum = new JTextField();
- JButton jbtQuery = new JButton("查询");
- JPanel jp = new JPanel();
- JLabel jlbEmpty = new JLabel("");
- JLabel jlbStu = new JLabel("学生信息");
- JLabel jlbUd = new JLabel("修改为");
- JLabel jlbIsUd = new JLabel("是否修改");
- JLabel jlbNum = new JLabel("学号");
- JTextField jtfNum = new JTextField(11);
- JTextField jtfUpToNum = new JTextField(11);
- JLabel jlbIsNumUd = new JLabel();
- JLabel jlbName = new JLabel("姓名");
- JTextField jtfName = new JTextField(11);
- JTextField jtfUpToName = new JTextField(11);
- JLabel jlbIsNameUd = new JLabel();
- JLabel jlbGender = new JLabel("性别");
- JTextField jtfGender = new JTextField(11);
- JTextField jtfUpToGender = new JTextField(11);
- JLabel jlbIsGenderUd = new JLabel();
- JLabel jlbAge = new JLabel("年龄");
- JTextField jtfAge = new JTextField(11);
- JTextField jtfUpToAge = new JTextField(11);
- JLabel jlbIsAgeUd = new JLabel();
- JLabel jlbMajor = new JLabel("专业");
- JTextField jtfMajor = new JTextField(11);
- JTextField jtfUpToMajor = new JTextField(11);
- JButton jbtMajor = new JButton("修改");
- JLabel jlbClass = new JLabel("班级");
- JTextField jtfClass = new JTextField(11);
- JTextField jtfUpToClass = new JTextField(11);
- JButton jbtClass = new JButton("修改");
- Users users = null;
- String textNum = null;
- public UpdateForStuFrame(Users users){
- this.users = users;
- }
- public void init(){
-
- jpQuery.add(jlbQueryNum);
- jpQuery.add(jtfQueryNum);
- jpQuery.add(jbtQuery);
- jp.setLayout(new GridLayout(7,4));
- jp.add(jlbEmpty);
- jp.add(jlbStu);
- jp.add(jlbUd);
- jp.add(jlbIsUd);
- jp.add(jlbNum);
- jp.add(jtfNum);
- jp.add(jtfUpToNum);
- jp.add(jlbIsNumUd);
- jp.add(jlbName);
- jp.add(jtfName);
- jp.add(jtfUpToName);
- jp.add(jlbIsNameUd);
- jp.add(jlbGender);
- jp.add(jtfGender);
- jp.add(jtfUpToGender);
- jp.add(jlbIsGenderUd);
- jp.add(jlbAge);
- jp.add(jtfAge);
- jp.add(jtfUpToAge);
- jp.add(jlbIsAgeUd);
- jp.add(jlbMajor);
- jp.add(jtfMajor);
- jp.add(jtfUpToMajor);
- jp.add(jbtMajor);
- jp.add(jlbClass);
- jp.add(jtfClass);
- jp.add(jtfUpToClass);
- jp.add(jbtClass);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpQuery);
- vbox.add(jp);
- updateForStuFrame.add(vbox);
-
- jtfQueryNum.setPreferredSize(new Dimension(100, 20));
- updateForStuFrame.setSize(600, 400);
- updateForStuFrame.setVisible(true);
- updateForStuFrame.setLocationRelativeTo(null);
- }
- //监听查询和修改按钮
- public void jbtListener(){
- //监听查询按钮
- jbtQuery.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- try {
- textNum = jtfQueryNum.getText();
- int num = Integer.parseInt(textNum);
- studentInfo = Function.queryForStudenInfoFunction(num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (studentInfo != null){
- JOptionPane.showMessageDialog(updateForStuFrame,"查询成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- setTextField();
- jtfQueryNum.setText("");
- }else {
- JOptionPane.showMessageDialog(updateForStuFrame,"查询失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }
- });
- //监听修改专业按钮
- jbtMajor.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege()) || users.getUser().equals(textNum)){
- String major = jtfUpToMajor.getText();
- Boolean flag = false;
- try {
- if (!major.equals(""))
- flag = Function.updateForStudentInfoFunctionOfMajor(major, studentInfo.getNum());
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag && !major.equals("")){
- JOptionPane.showMessageDialog(updateForStuFrame,"修改成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- jtfMajor.setText(major);
- jtfUpToMajor.setText("");
- }else{
- JOptionPane.showMessageDialog(updateForStuFrame,"修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(updateForStuFrame,"非管理员或非本人操作,修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- //监听修改班级按钮
- jbtClass.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege()) || users.getUser().equals(textNum)){
- String classes = jtfUpToClass.getText();
- boolean flag = false;
- try {
- if (!classes.equals(""))
- flag = Function.updateForStudentInfoFunctionOfClasses(classes,studentInfo.getNum());
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag && !classes.equals("")){
- JOptionPane.showMessageDialog(updateForStuFrame,"修改成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- jtfClass.setText(classes);
- jtfUpToClass.setText("");
- }else {
- JOptionPane.showMessageDialog(updateForStuFrame,"修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(updateForStuFrame,"非管理员或非本人操作,修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- updateForStuFrame.addWindowListener(new WindowAdapter() {
- @Override
- public void windowClosing(WindowEvent e) {
- updateForStuFrame.setVisible(false);
- }
- });
- }
- //封装填充查询后的信息
- public void setTextField(){
- int infoNum = studentInfo.getNum();
- String num1 = ""+infoNum;
- String infoName = studentInfo.getName();
- String infoGender = studentInfo.getGender();
- int infoAge = studentInfo.getAge();
- String age = "" + infoAge;
- String infoMajor = studentInfo.getMajor();
- String infoClasses = studentInfo.getClasses();
- jtfNum.setText(num1);
- jtfName.setText(infoName);
- jtfGender.setText(infoGender);
- jtfAge.setText(age);
- jtfMajor.setText(infoMajor);
- jtfClass.setText(infoClasses);
- }
- }
复制代码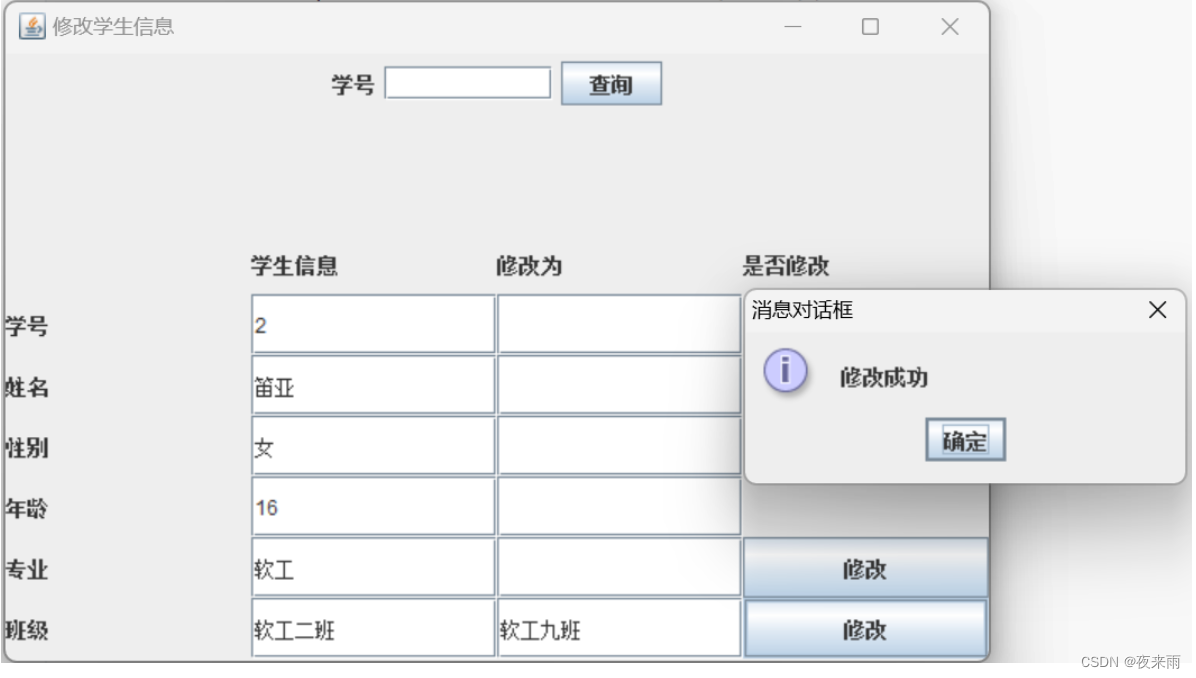
9.修改课程信息界面
- package MyFrame;
- import Function.Function;
- import bean.CourseInfo;
- import bean.Users;
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.WindowAdapter;
- import java.awt.event.WindowEvent;
- //修改课程信息界面
- public class UpdateForCouFrame {
- CourseInfo courseInfo = null;
- JFrame updateForCouFrame = new JFrame("修改课程信息");
- JPanel jpQuery = new JPanel();
- JLabel jlbQurtyNum = new JLabel("学号");
- JTextField jtfQueryNum = new JTextField(11);
- JButton jbtQuery = new JButton("查询");
- JPanel jp = new JPanel();
- JLabel jlbEmpty = new JLabel("");
- JLabel jlbStu = new JLabel("课程信息");
- JLabel jlbUd = new JLabel("修改为");
- JLabel jlbIsUd = new JLabel("是否修改");
- JLabel jlbNum = new JLabel("学号");
- JTextField jtfNum = new JTextField(11);
- JTextField jtfUpToNum = new JTextField(11);
- JLabel jlbIsNum = new JLabel();
- JLabel jlbName = new JLabel("姓名");
- JTextField jtfName = new JTextField(11);
- JTextField jtfUpToName = new JTextField(11);
- JLabel jlbIsName = new JLabel();
- JLabel jlbCourse = new JLabel("课程");
- JTextField jtfCourse = new JTextField(11);
- JTextField jtfUpToCourse = new JTextField(11);
- JButton jbtIsCourse = new JButton("修改");
- JLabel jlbCredit = new JLabel("学分");
- JTextField jtfCredit = new JTextField(11);
- JTextField jtfUpToCredit = new JTextField(11);
- JButton jbtIsCredit = new JButton("修改");
- Users users = null;
- String textNum = null;
- public UpdateForCouFrame(Users users){
- this.users = users;
- }
- public void init(){
- jpQuery.add(jlbQurtyNum);
- jpQuery.add(jtfQueryNum);
- jpQuery.add(jbtQuery);
- jp.setLayout(new GridLayout(5,4));
- jp.add(jlbEmpty);
- jp.add(jlbStu);
- jp.add(jlbUd);
- jp.add(jlbIsUd);
- jp.add(jlbNum);
- jp.add(jtfNum);
- jp.add(jtfUpToNum);
- jp.add(jlbIsNum);
- jp.add(jlbName);
- jp.add(jtfName);
- jp.add(jtfUpToName);
- jp.add(jlbIsName);
- jp.add(jlbCourse);
- jp.add(jtfCourse);
- jp.add(jtfUpToCourse);
- jp.add(jbtIsCourse);
- jp.add(jlbCredit);
- jp.add(jtfCredit);
- jp.add(jtfUpToCredit);
- jp.add(jbtIsCredit);
- Box vbox = Box.createVerticalBox();
- vbox.add(jpQuery);
- vbox.add(jp);
- updateForCouFrame.add(vbox);
- updateForCouFrame.setSize(600, 400);
- updateForCouFrame.setVisible(true);
- updateForCouFrame.setLocationRelativeTo(null);
- }
- //监听查询修改按钮
- public void jbtListener(){
- jbtQuery.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- try {
- textNum = jtfQueryNum.getText();
- int num = Integer.parseInt(textNum);
- courseInfo = Function.queryForCourseInfoFunction(num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (courseInfo != null){
- JOptionPane.showMessageDialog(updateForCouFrame,"查询成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- setTextField();
- }else {
- JOptionPane.showMessageDialog(updateForCouFrame,"查询失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }
- });
- //监听修改课程的按钮
- jbtIsCourse.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege()) || users.getUser().equals(textNum)){
- int num = courseInfo.getNum();
- String course = jtfUpToCourse.getText();
- Boolean flag = false;
- try {
- if(!course.equals(""))
- flag = Function.updateForCourseInfoFunctionOfCourse(course,num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag && courseInfo != null && !course.equals("")) {
- JOptionPane.showMessageDialog(updateForCouFrame,"修改成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- jtfCourse.setText(course);
- jtfUpToCourse.setText("");
- }else {
- JOptionPane.showMessageDialog(updateForCouFrame,"修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(updateForCouFrame,"非管理员或非本人操作,修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- //监听修改学分的按钮
- jbtIsCredit.addActionListener(new ActionListener() {
- @Override
- public void actionPerformed(ActionEvent e) {
- if ("管理员".equals(users.getPrivilege()) || users.getUser().equals(textNum)){
- int num = courseInfo.getNum();
- String credit = jtfUpToCredit.getText();
- Boolean flag = false;
- try {
- if (!credit.equals(""))
- flag = Function.updateForCourseInfoFunctionOfCredit(credit,num);
- } catch (Exception ex) {
- throw new RuntimeException(ex);
- }
- finally {
- if (flag && courseInfo != null && !credit.equals("")) {
- JOptionPane.showMessageDialog(updateForCouFrame,"修改成功","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- jtfCredit.setText(credit);
- jtfUpToCredit.setText("");
- }else {
- JOptionPane.showMessageDialog(updateForCouFrame,"修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- }else {
- JOptionPane.showMessageDialog(updateForCouFrame,"非管理员或非本人操作,修改失败","消息对话框",JOptionPane.INFORMATION_MESSAGE);
- }
- }
- });
- updateForCouFrame.addWindowListener(new WindowAdapter() {
- @Override
- public void windowClosing(WindowEvent e) {
- updateForCouFrame.setVisible(false);
- }
- });
- }
- public void setTextField(){
- int num1 = courseInfo.getNum();
- String num2= "" + num1;
- String name = courseInfo.getName();
- String course = courseInfo.getCourse();
- String credit = courseInfo.getCredit();
- jtfNum.setText(num2);
- jtfName.setText(name);
- jtfCourse.setText(course);
- jtfCredit.setText(credit);
- jtfQueryNum.setText("");
- }
- }
复制代码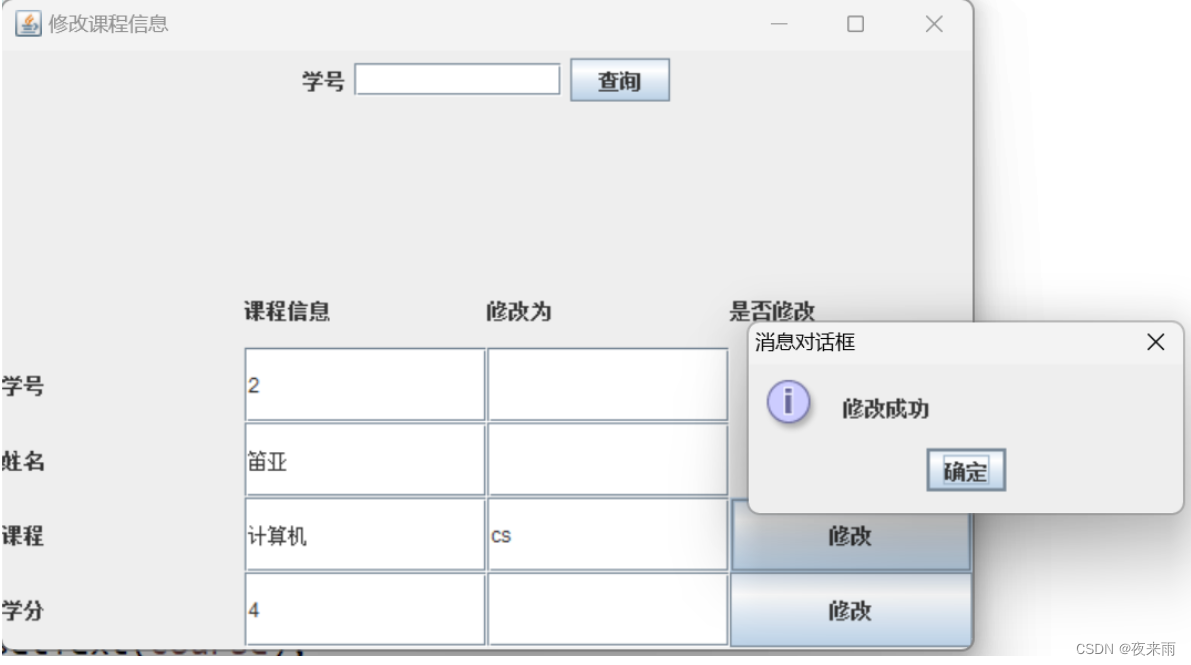
10.查询门生信息界面
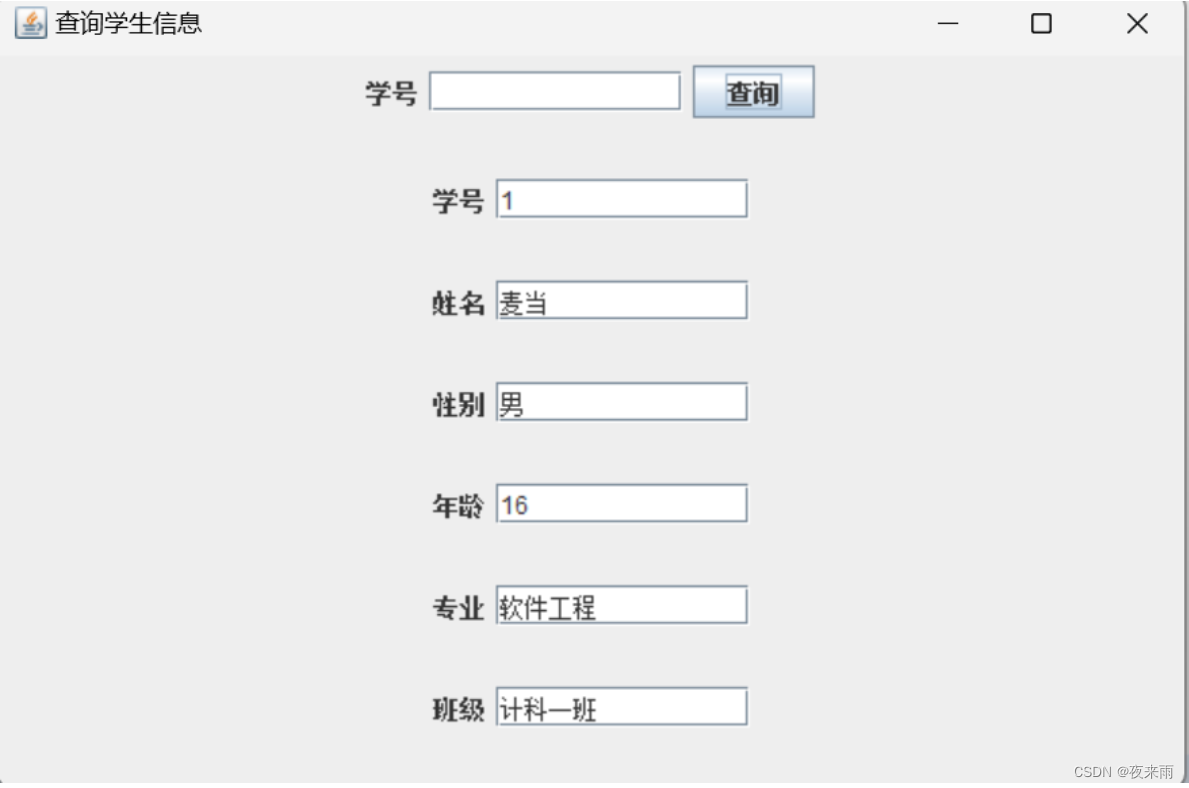
11.查询课程信息界面
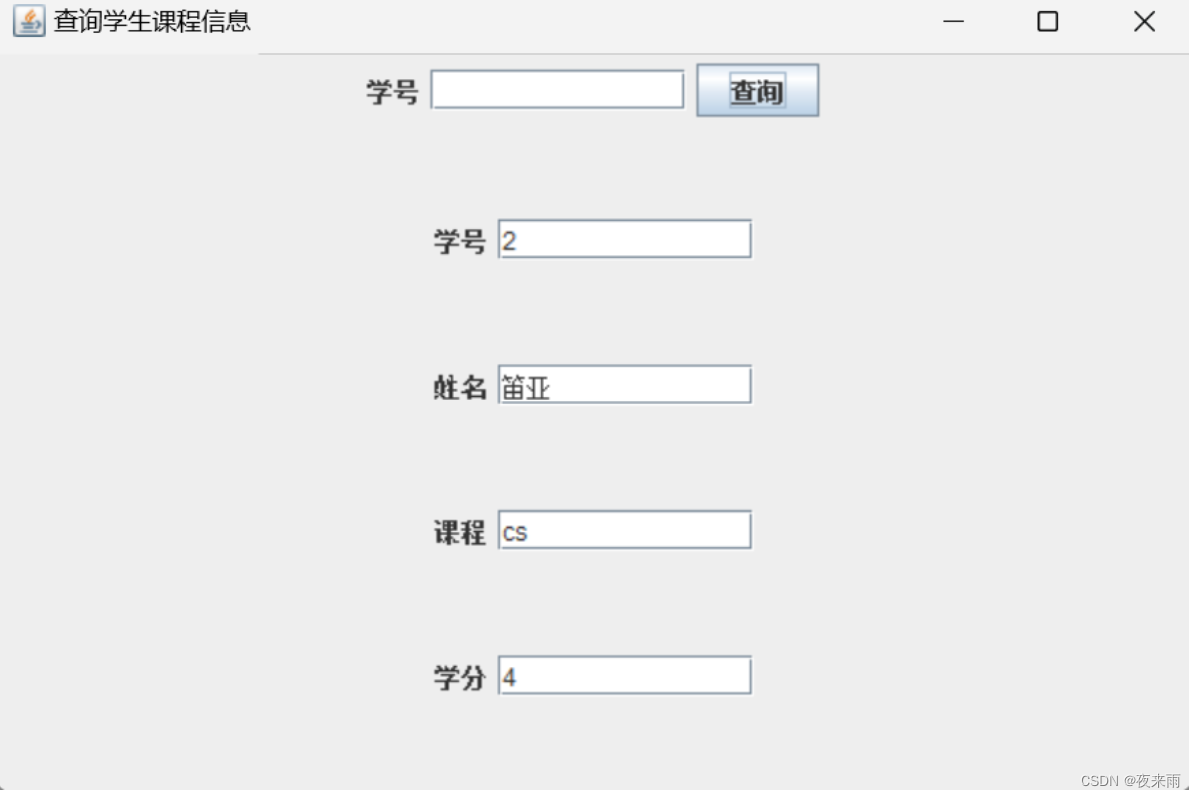
6.创建测试类
- package JunitDemo;
- import MyFrame.RegisterFrame;
- //学生信息管理系统的测试类
- public class TestDemo {
- public static void main(String[] args) {
- //创建登录界面对象
- RegisterFrame registerFrame = new RegisterFrame();
- //登录界面初试化
- registerFrame.init();
- //登录界面相关监听器
- registerFrame.buttonListenerForRegister();
- }
- }
复制代码 5.项目总结
这个项目涵盖了GUI,MySQL,JDBC等方面,固然这个项目没有太大的技能,但也算是一个小小的期末课程设计作业。当然了,这此中也有很多的不足,欢迎大家批评指正。
另外,附上源码:
百度网盘源码,点击获取源码
导入源码的小同伴们请注意了:我的MySQL是5.7的,所以我的项目中导入了顺应5.7版本的驱动。
同时将eclipse的字符集换成utf-8,并且运行前记得打开数据库哦。
假如有小同伴的MySQL不是5.7的,那么请将驱动换成相对应的即可。
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |