1、先配置spring、springmvc通用xml文件的头部信息
spring、springmvc通用xml文件的模板内容如下:
  - 1 <?xml version="1.0" encoding="UTF-8"?>
- 2 <beans xmlns="http://www.springframework.org/schema/beans"
- 3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- 4 xmlns:aop="http://www.springframework.org/schema/aop"
- 5 xmlns:context="http://www.springframework.org/schema/context"
- 6 xmlns:tx="http://www.springframework.org/schema/tx"
- 7 xmlns:mvc="http://www.springframework.org/schema/mvc"
- 8 xsi:schemaLocation="http://www.springframework.org/schema/beans
- 9 http://www.springframework.org/schema/beans/spring-beans.xsd
- 10 http://www.springframework.org/schema/aop
- 11 http://www.springframework.org/schema/aop/spring-aop.xsd
- 12 http://www.springframework.org/schema/context
- 13 http://www.springframework.org/schema/context/spring-context.xsd
- 14 http://www.springframework.org/schema/tx
- 15 http://www.springframework.org/schema/tx/spring-tx.xsd
- 16 http://www.springframework.org/schema/mvc
- 17 http://www.springframework.org/schema/mvc/spring-mvc.xsd
- 18 ">
- 19
- 20 </beans>
复制代码 spring、springmvc文件通用内容
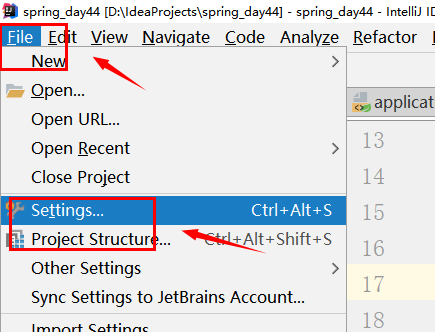
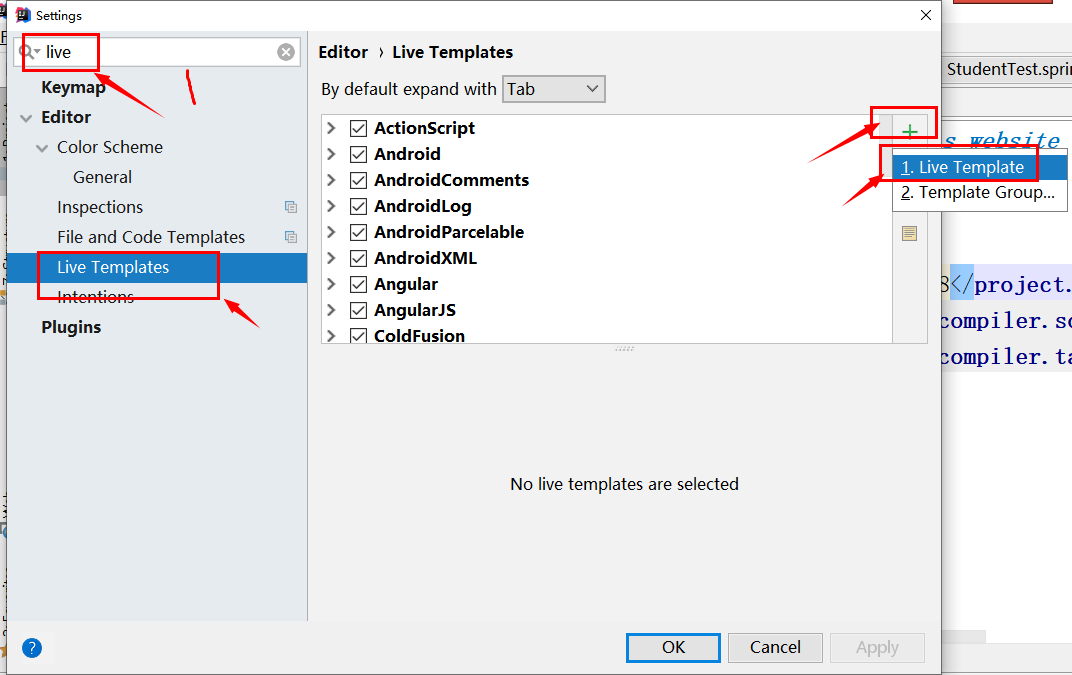
spring、spring
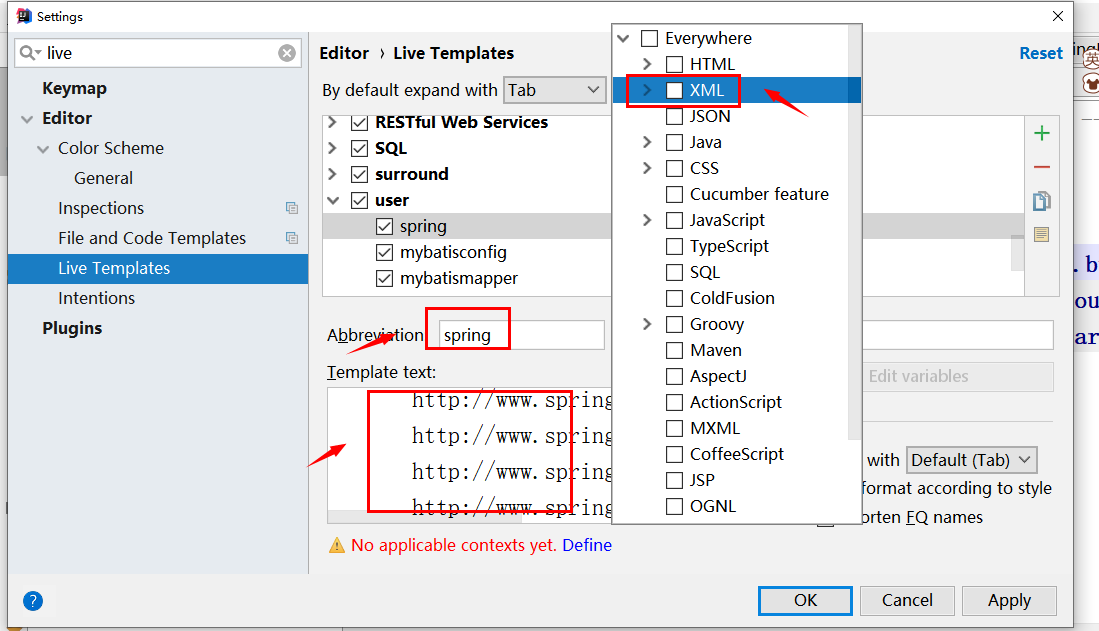
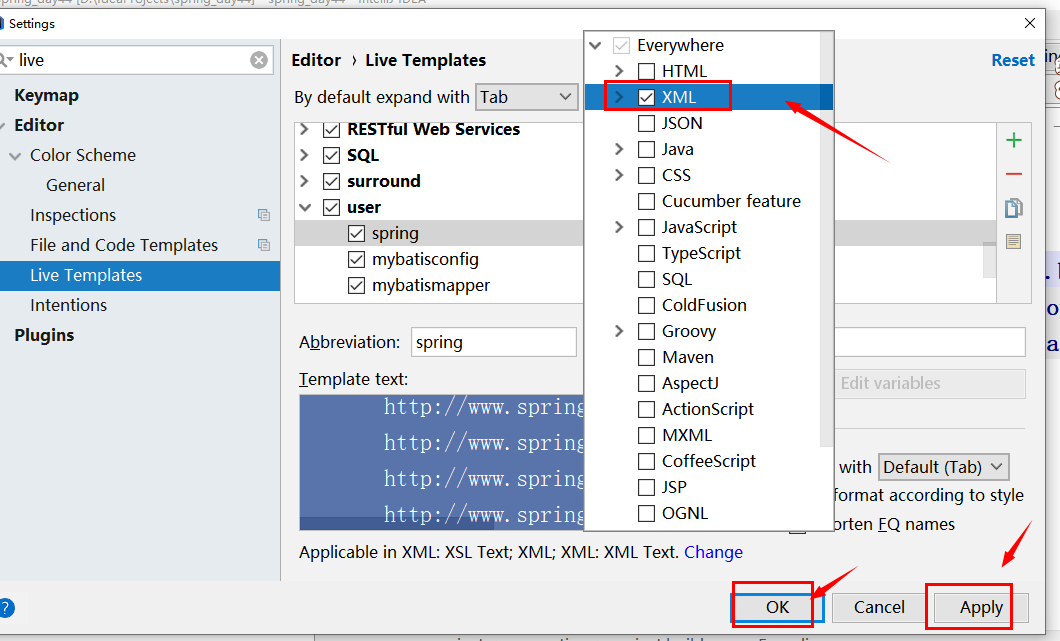
2、构建spring的第一个工程
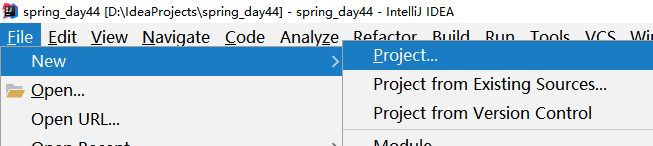
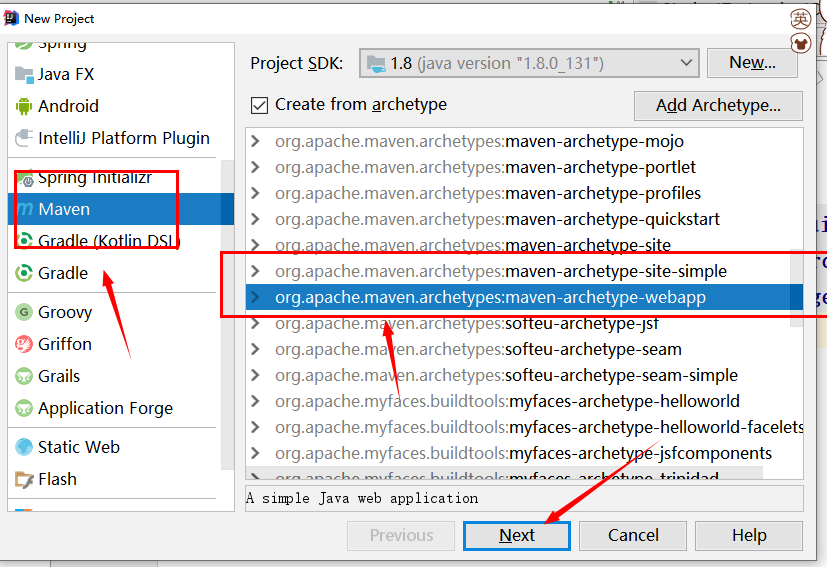
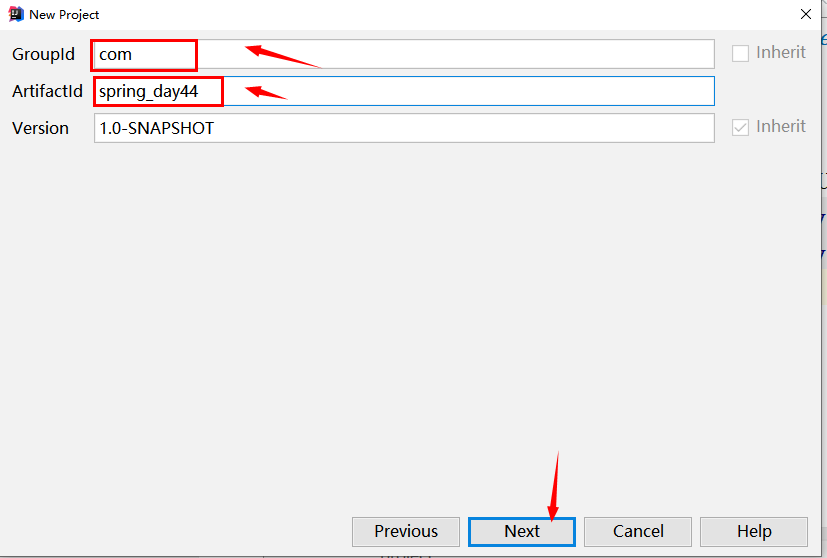
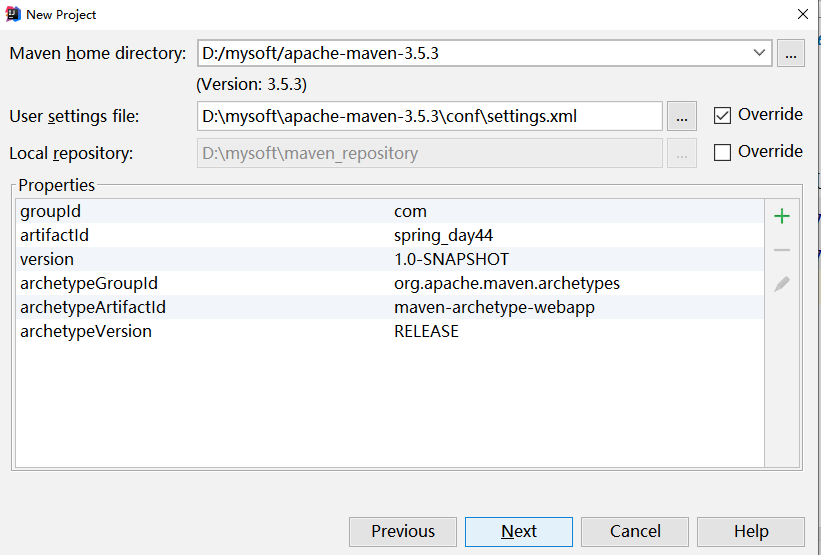
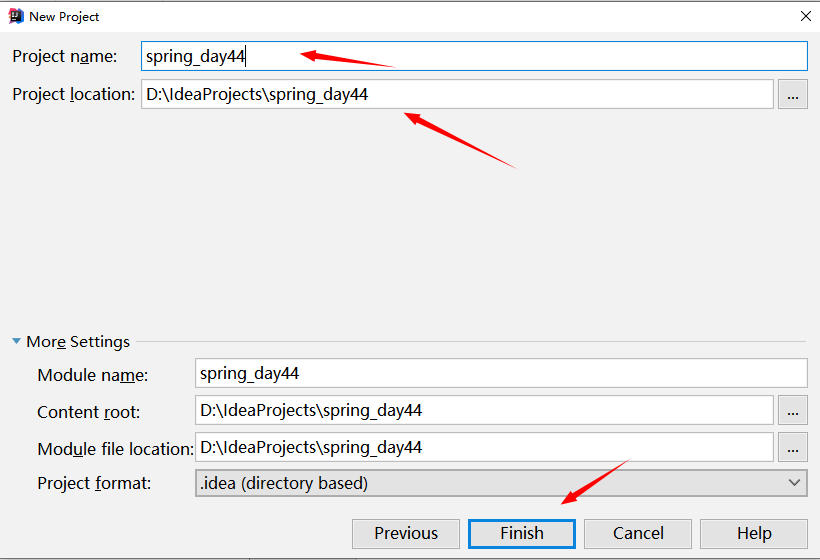
3、配置项目中的pom.xml文件依赖
 pom.xml4、在项目的main下创建java和resouces目录
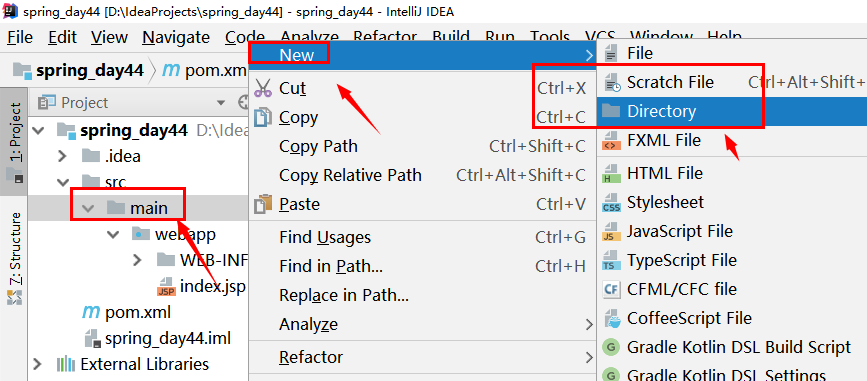
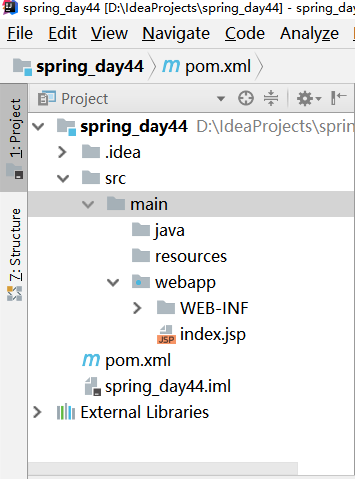
5、将java目录变为编译目录
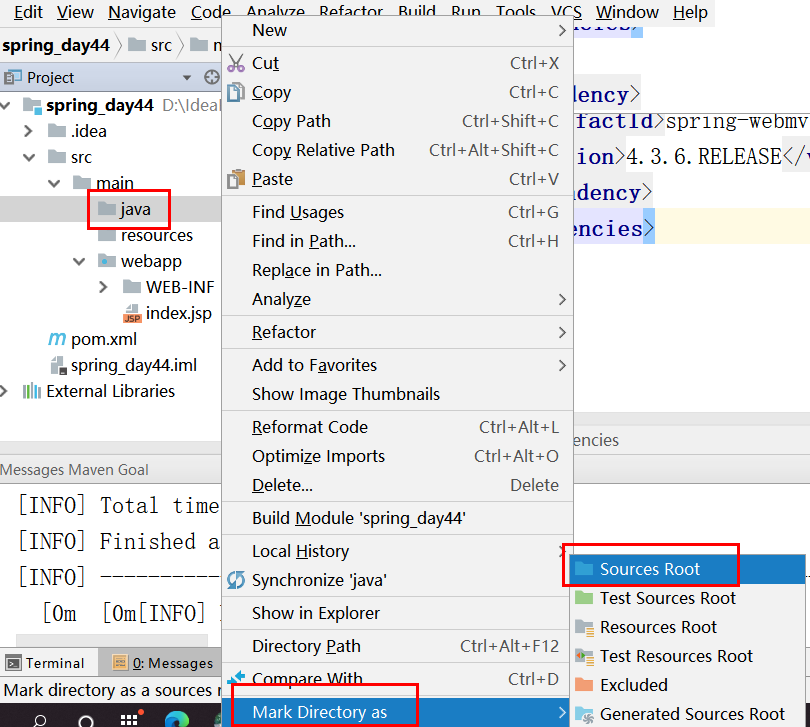 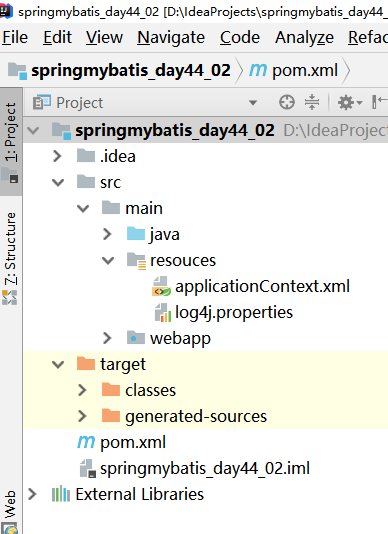
6、将resources目录变为存放xml脚本的目录
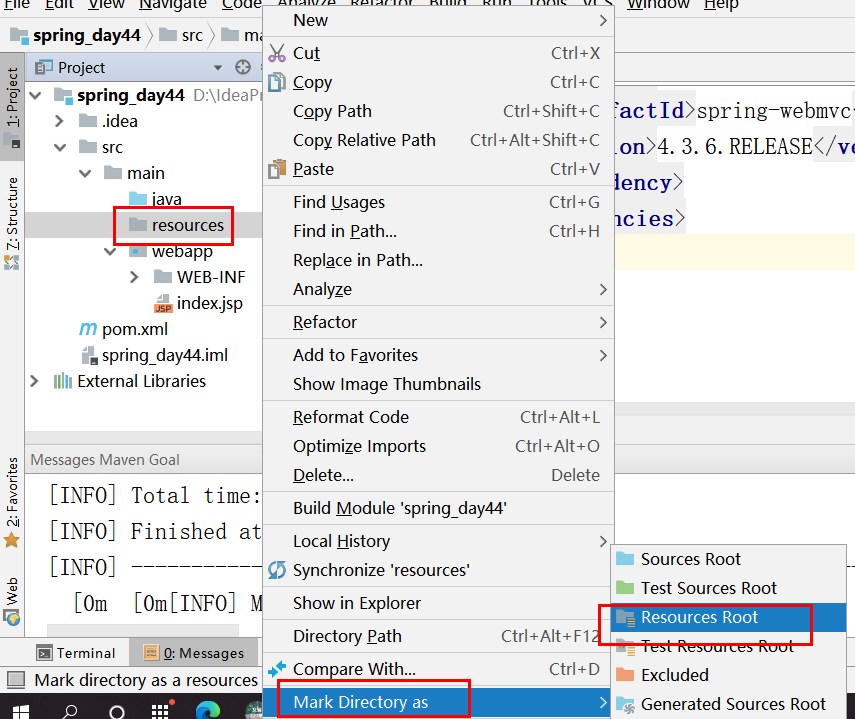 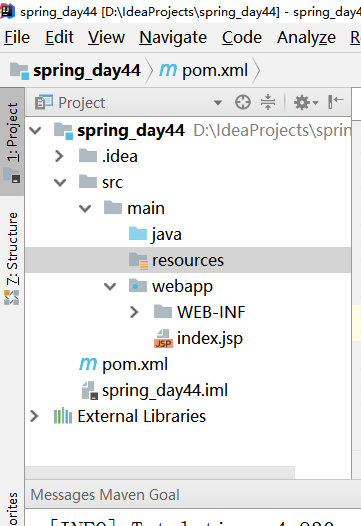
7、在java的com.pojo包下构建Student和Classes两个类
  - 1 package com.pojo;
- 2
- 3 public class Classes {
- 4 private Integer cid;
- 5 private String cname;
- 6
- 7 public Classes() {
- 8 }
- 9
- 10 public Classes(Integer cid, String cname) {
- 11 this.cid = cid;
- 12 this.cname = cname;
- 13 }
- 14
- 15 public Integer getCid() {
- 16 return cid;
- 17 }
- 18
- 19 public void setCid(Integer cid) {
- 20 this.cid = cid;
- 21 }
- 22
- 23 public String getCname() {
- 24 return cname;
- 25 }
- 26
- 27 public void setCname(String cname) {
- 28 this.cname = cname;
- 29 }
- 30
- 31 @Override
- 32 public String toString() {
- 33 return "Classes{" +
- 34 "cid=" + cid +
- 35 ", cname='" + cname + '\'' +
- 36 '}';
- 37 }
- 38 }
复制代码 Classes.java  - 1 package com.pojo;
- 2
- 3 public class Student {
- 4 private Integer sid;
- 5 private String sname;
- 6 private String course;
- 7 private Double score;
- 8
- 9 private Classes classes;
- 10
- 11 public Student() {
- 12 }
- 13
- 14 public Student(Integer sid, String sname, String course, Double score, Classes classes) {
- 15 this.sid = sid;
- 16 this.sname = sname;
- 17 this.course = course;
- 18 this.score = score;
- 19 this.classes = classes;
- 20 }
- 21
- 22 public Integer getSid() {
- 23 return sid;
- 24 }
- 25
- 26 public void setSid(Integer sid) {
- 27 this.sid = sid;
- 28 }
- 29
- 30 public String getSname() {
- 31 return sname;
- 32 }
- 33
- 34 public void setSname(String sname) {
- 35 this.sname = sname;
- 36 }
- 37
- 38 public String getCourse() {
- 39 return course;
- 40 }
- 41
- 42 public void setCourse(String course) {
- 43 this.course = course;
- 44 }
- 45
- 46 public Double getScore() {
- 47 return score;
- 48 }
- 49
- 50 public void setScore(Double score) {
- 51 this.score = score;
- 52 }
- 53
- 54 public Classes getClasses() {
- 55 return classes;
- 56 }
- 57
- 58 public void setClasses(Classes classes) {
- 59 this.classes = classes;
- 60 }
- 61
- 62 @Override
- 63 public String toString() {
- 64 return "Student{" +
- 65 "sid=" + sid +
- 66 ", sname='" + sname + '\'' +
- 67 ", course='" + course + '\'' +
- 68 ", score=" + score +
- 69 ", classes=" + classes +
- 70 '}';
- 71 }
- 72 }
复制代码 Student.java8、在resouces下创建spring的applicationContext.xml文件
  - 1 <?xml version="1.0" encoding="UTF-8"?>
- 2 <beans xmlns="http://www.springframework.org/schema/beans"
- 3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- 4 xmlns:aop="http://www.springframework.org/schema/aop"
- 5 xmlns:context="http://www.springframework.org/schema/context"
- 6 xmlns:tx="http://www.springframework.org/schema/tx"
- 7 xmlns:mvc="http://www.springframework.org/schema/mvc"
- 8 xsi:schemaLocation="http://www.springframework.org/schema/beans
- 9 http://www.springframework.org/schema/beans/spring-beans.xsd
- 10 http://www.springframework.org/schema/aop
- 11 http://www.springframework.org/schema/aop/spring-aop.xsd
- 12 http://www.springframework.org/schema/context
- 13 http://www.springframework.org/schema/context/spring-context.xsd
- 14 http://www.springframework.org/schema/tx
- 15 http://www.springframework.org/schema/tx/spring-tx.xsd
- 16 http://www.springframework.org/schema/mvc
- 17 http://www.springframework.org/schema/mvc/spring-mvc.xsd
- 18 ">
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26 <bean id="classes" class="com.pojo.Classes">
- 27
- 28
- 29
- 30
- 31 <property name="cid" value="1"/>
- 32 <property name="cname" value="教学部"/>
- 33 </bean>
- 34
- 35 <bean id="student" class="com.pojo.Student">
- 36 <property name="sid" value="10"/>
- 37 <property name="sname" value="holly"/>
- 38 <property name="course" value="java"/>
- 39 <property name="score" value="65.5"/>
- 40
- 41 <property name="classes" ref="classes"/>
- 42 </bean>
- 43 </beans>
复制代码 applicationContext.xml9、在java的com.test包下构建StudentTest.java测试类
  - 1 package com.test;
- 2
- 3 import com.pojo.Classes;
- 4 import com.pojo.Student;
- 5 import org.junit.Test;
- 6 import org.springframework.context.ApplicationContext;
- 7 import org.springframework.context.support.ClassPathXmlApplicationContext;
- 8
- 9 //常规:需要对象时,主动创建,主动赋值,
- 10 //springIOC:需要对象时,被动接受完整对象(spring对我们所需要的对象进行赋值)
- 11 public class StudentTest {
- 12 @Test
- 13 public void normal(){
- 14 Classes classes=new Classes();
- 15 classes.setCid(1);
- 16 classes.setCname("教学部");
- 17
- 18 Student student=new Student();
- 19 student.setSid(10);
- 20 student.setSname("holly");
- 21 student.setCourse("java");
- 22 student.setScore(65.5);
- 23 student.setClasses(classes);
- 24
- 25 System.out.println(classes);
- 26 System.out.println(student);
- 27 }
- 28
- 29 @Test
- 30 public void springIOC(){
- 31 //加载spring的xml文件
- 32 ApplicationContext ac=new ClassPathXmlApplicationContext("applicationContext.xml");
- 33
- 34 //spring给我们对象注入需要的值,我们根据bean 的id去查找赋值
- 35 Classes classes=(Classes) ac.getBean("classes");
- 36 Student student =(Student) ac.getBean("student");
- 37
- 38 System.out.println(classes);
- 39 System.out.println(student);
- 40 }
- 41 }
复制代码 StudentTest.java10、运行我们的测试方法且查看我们的打印效果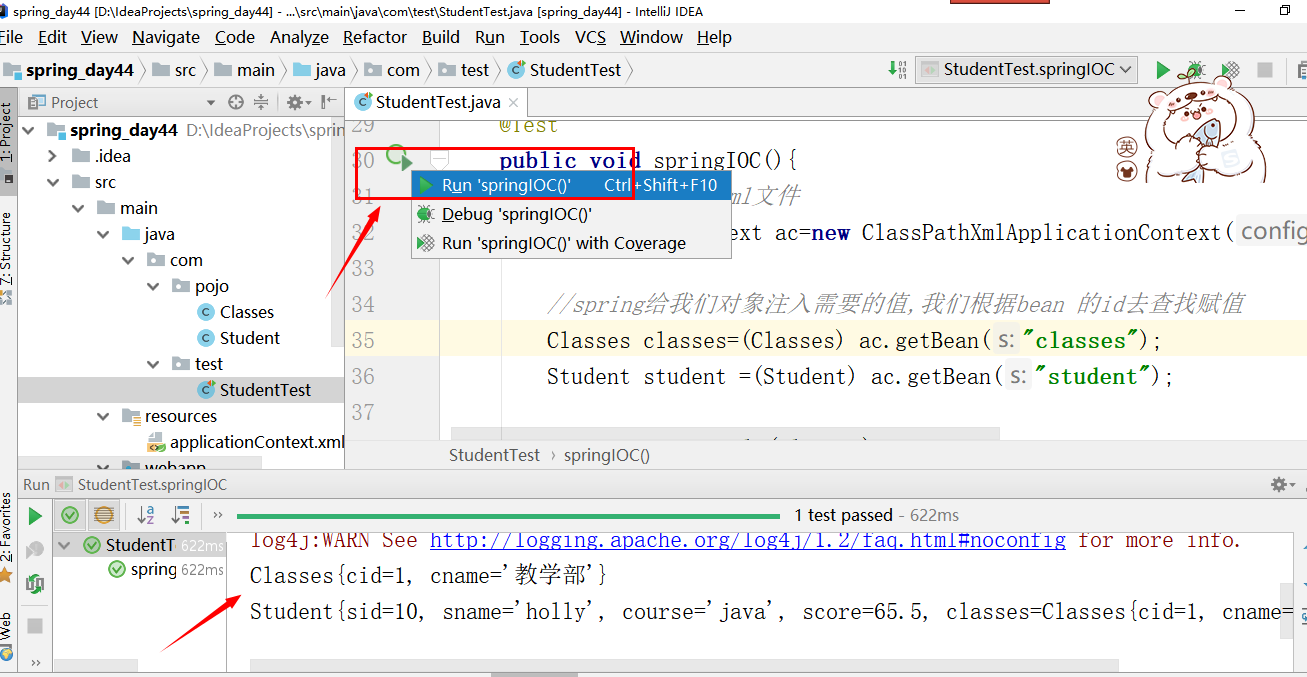
声明:此为原创,源码+理论讲解视频+操作视频,请私信作者!
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作! |