1.My_string类中重载以下的运算符:
+、[] 、>、<、==、>=、<=、!=、+=、输入输出(>>、<<)
主函数:
- #include <iostream>
- #include "my_string.h"
- using namespace std;
- int main()
- {
- My_string s1("cat");
- My_string s2(" fash");
- My_string s3=s1+s2;
- s3.show();
- cout<<"索引:"<<s3[5]<<endl;
- My_string s4("beef");
- My_string s5(" milk");
- s4+=s5;
- s4.show();
- My_string s6("Abc");
- My_string s7("abc");
- cout<<(s6>s7)<<endl;
- cout<<(s6<s7)<<endl;
- return 0;
- }
复制代码 my_string.h
- #ifndef MY_STRING_H
- #define MY_STRING_H
- #include <iostream>
- #include <cstring>
- using namespace std;
- class My_string
- {
- private:
- char *ptr;
- int size;
- int len;
- public:
- //无参构造
- My_string();
- //有参构造
- My_string(const char *src);
- //析构函数
- ~My_string();
- My_string operator+(My_string &S);
- char & operator[](int index);
- bool operator>(const My_string &S) const;
- bool operator<(const My_string &S) const;
- bool operator==(const My_string &S) const;
- bool operator>=(const My_string &S) const;
- bool operator<=(const My_string &S) const;
- bool operator!=(const My_string &S) const;
- My_string & operator+=(const My_string &S);
- friend ostream& operator<<(ostream &out, const My_string &S);
- friend istream& operator>>(istream &in, My_string &S);
- void show();
- };
- #endif // MY_STRING_H
复制代码 my_string.cpp
- #include "my_string.h"
- //无参构造
- My_string::My_string():size(15)
- {
- this->ptr=new char[size];
- this->ptr[0]='\0';
- this->len=0;
- }
- //有参构造
- My_string::My_string(const char *src)
- {
- len=0;
- for(int i=0;src[i]!='\0';i++)
- {
- len++;
- }
- size=len+1;
- this->ptr=new char[size];
- for(int i=0;i<len;i++)
- {
- ptr[i]=src[i];
- }
- ptr[len]='\0';
- }
- //析构函数
- My_string::~My_string()
- {
- delete []ptr;
- //cout<<"析构函数"<<this<<endl;
- }
- //重载 +操作符
- My_string My_string::operator+(My_string &S)
- {
- int newlen=len+S.len;
- char *newptr=new char[newlen+1];//分配新内存
- strcpy(newptr,this->ptr);
- strcat(newptr,S.ptr);
- My_string temp(newptr);//创建临时对象
- delete []newptr; // 释放临时内存
- return temp;
- }
- //重载 [] 操作符
- char & My_string::operator[](int index)
- {
- if(index<0 ||index>=len)
- {
- exit(EXIT_FAILURE);
- }
- else
- {
- return ptr[index-1];
- }
- }
- // 重载 > 操作符
- bool My_string::operator>(const My_string &S) const
- {
- return strcmp(this->ptr, S.ptr) > 0;
- }
- // 重载 < 操作符
- bool My_string::operator<(const My_string &S) const
- {
- return strcmp(this->ptr, S.ptr) < 0;
- }
- // 重载 == 操作符
- bool My_string::operator==(const My_string &S) const
- {
- return strcmp(this->ptr, S.ptr) == 0;
- }
- // 重载 >= 操作符
- bool My_string::operator>=(const My_string &S) const
- {
- return strcmp(this->ptr, S.ptr) >= 0;
- }
- // 重载 <= 操作符
- bool My_string::operator<=(const My_string &S) const
- {
- return strcmp(this->ptr, S.ptr) <= 0;
- }
- // 重载 != 操作符
- bool My_string::operator!=(const My_string &S) const
- {
- return strcmp(this->ptr, S.ptr) != 0;
- }
- // 重载 += 操作符
- My_string & My_string::operator+=(const My_string &S)
- {
- int newlen=len+S.len;
- char *newptr = new char[newlen+1];
- strcpy(newptr, this->ptr);
- strcat(newptr, S.ptr);
- delete[] this->ptr;
- this->ptr = newptr; // 更新 ptr 指向新内存
- this->len += newlen; // 更新长度
- this->size = newlen + 1; // 更新容量
- return *this;
- }
- ostream& operator<<(ostream &out, const My_string &S)
- {
- out << S.ptr;
- return out;
- }
- istream& operator>>(istream &in, My_string &S)
- {
- delete[] S.ptr; // 释放已有内存
- S.ptr = new char[S.size]; // 重新分配内存
- in >> S.ptr; // 读取字符串
- S.len = strlen(S.ptr); // 更新长度
- return in;
- }
- void My_string::show()
- {
- cout<<"*ptr="<<ptr<<endl;
- cout<<"size="<<size<<endl;
- cout<<"len="<<len<<endl;
- }
复制代码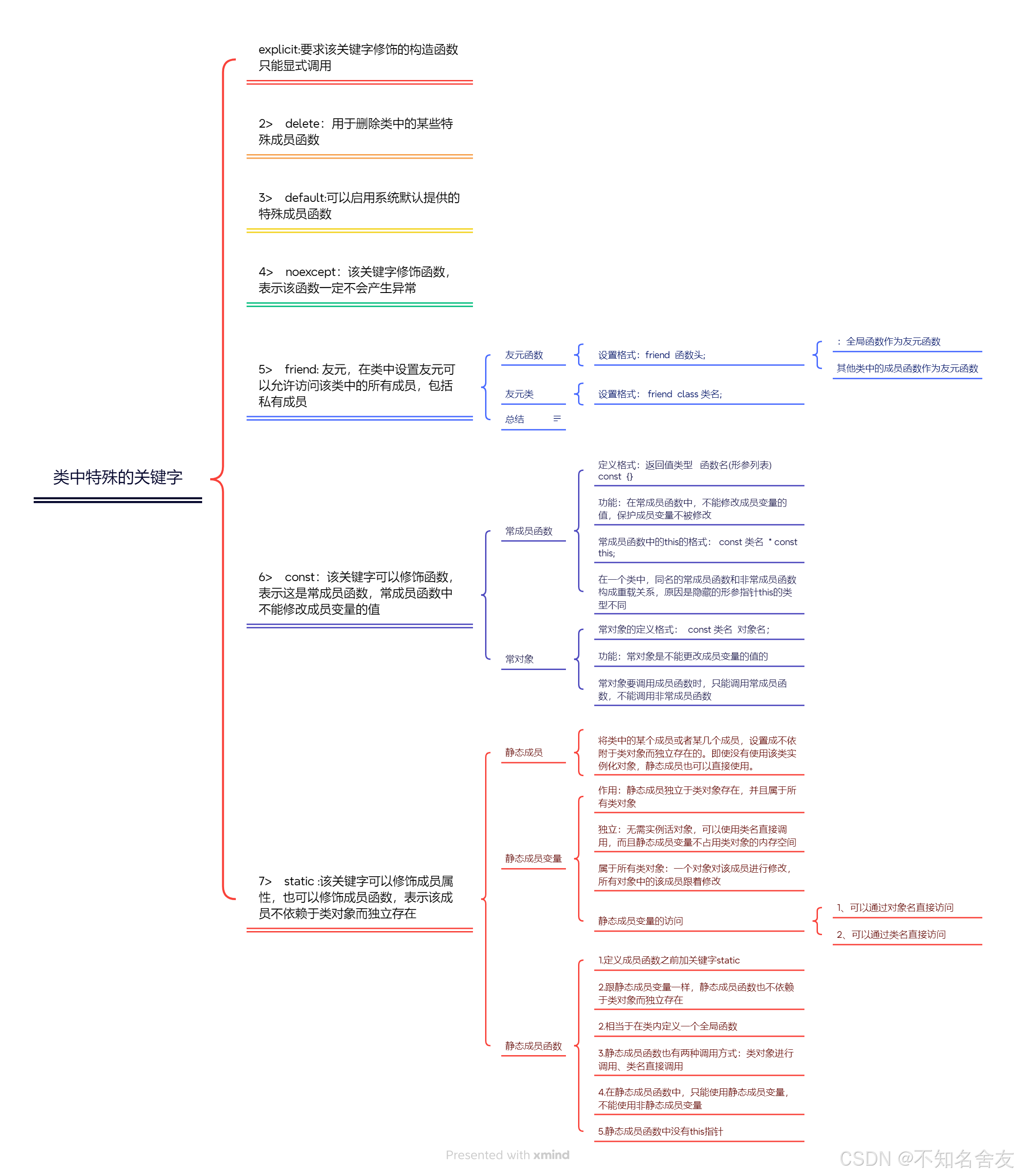 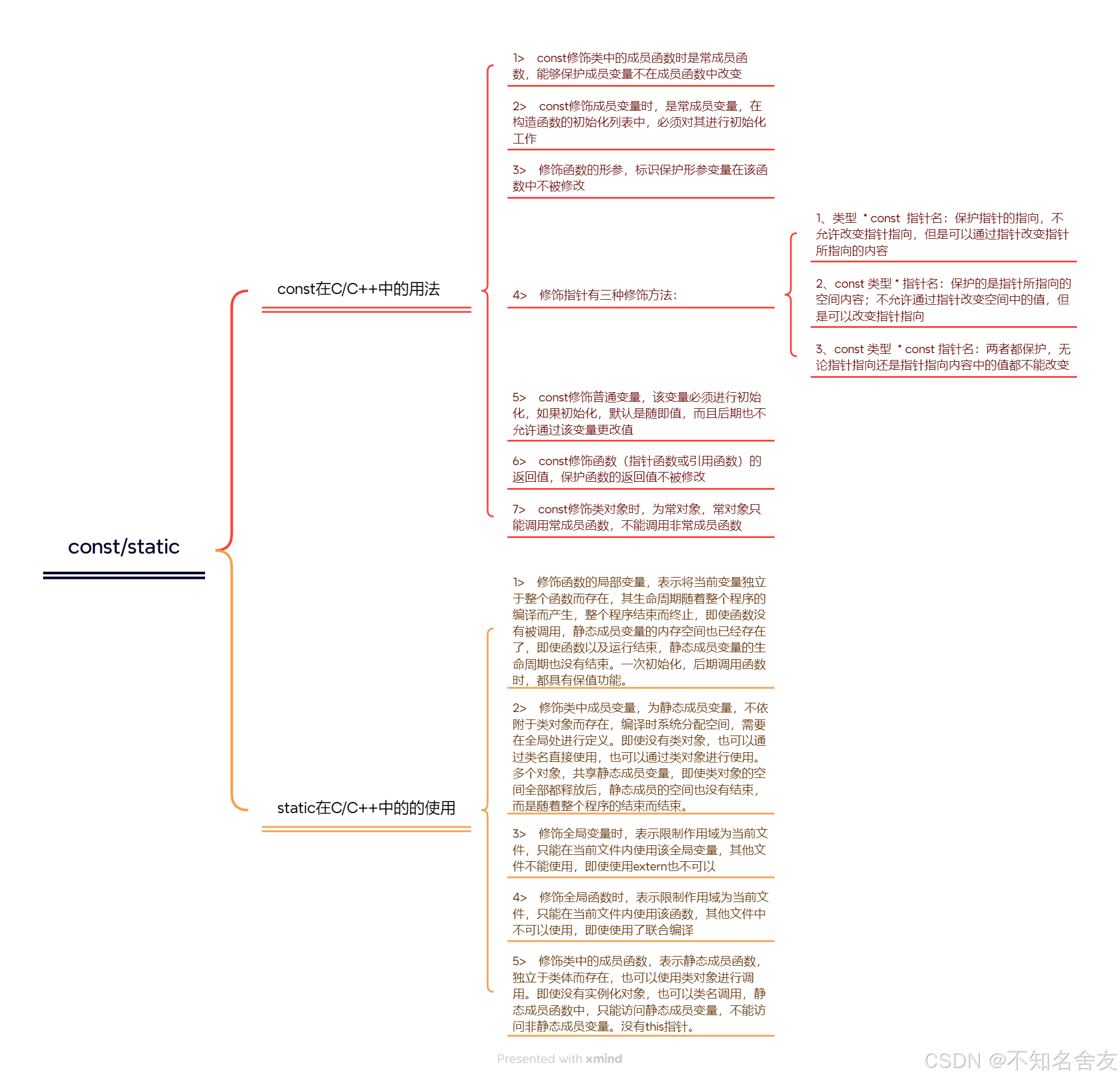
免责声明:如果侵犯了您的权益,请联系站长,我们会及时删除侵权内容,谢谢合作!更多信息从访问主页:qidao123.com:ToB企服之家,中国第一个企服评测及商务社交产业平台。 |